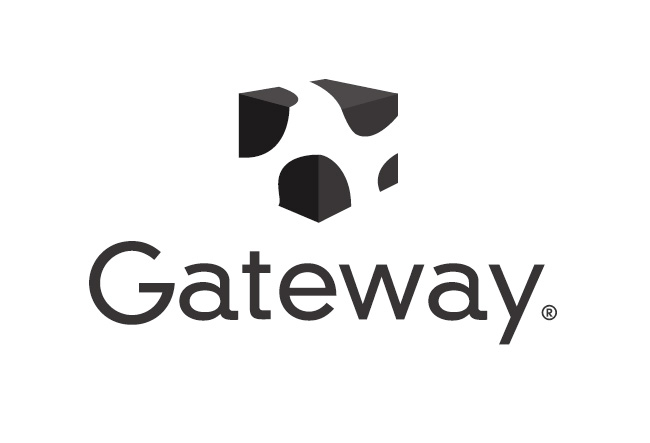
【gateway 入门】5、创建一个简单的Gateway服务
"Gateway" 是一个多义词,在不同的领域有不同的意义。它在计算机网络中是连接不同网络的设备或节点,能在不同协议、数据格式和体系结构之间进行转换,使得不同网络之间能够互相通信;在电子商务中是处理和授权在线支付的服务,确保交易安全顺利进行;在旅游和交通中指的是主要的出入口或枢纽,如国际机场。无论在哪个领域,Gateway 的核心概念都是作为“连接”和“通道”,它是实现两个不同系统或区域之间互动的
【gateway 入门】创建一个简单的Gateway服务
系列文章目录
【gateway 入门】1、什么是Gateway?
【gateway 入门】2、Gateway的基本概念和术语
【gateway 入门】3、安装和配置Gateway
【gateway 入门】4、Gateway的架构和组件介绍
【gateway 入门】5、创建一个简单的Gateway服务(本文)
【gateway 入门】6、路由配置基础
【gateway 入门】7、请求和响应的处理流程
【gateway 入门】8、使用Gateway进行API管理
【gateway 入门】9、基础安全设置:认证和授权
【gateway 入门】10、日志和监控基础
在前面的文章中,我们已经了解了Gateway的基本概念、术语、安装配置以及架构和组件。现在,我们将动手创建一个简单的Gateway服务,以便更好地理解和应用这些知识。
环境准备
1. 创建Spring Boot项目
首先,我们需要创建一个Spring Boot项目。你可以使用Spring Initializr来快速生成项目:
- 打开 Spring Initializr
- 选择项目类型为 Maven Project
- 选择Spring Boot版本(通常选择最新稳定版)
- 填写项目元数据(如Group、Artifact等)
- 在依赖中选择 Gateway 和 Spring Web
点击“Generate”按钮下载项目压缩包,解压后导入你喜欢的IDE中(如IntelliJ IDEA或Eclipse)。
2. 添加依赖
在 pom.xml
文件中,确保包含以下依赖:
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-webflux</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-gateway</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
</dependencies>
配置Gateway
1. 创建配置文件
在 src/main/resources
目录下,创建或编辑 application.yml
文件,添加基本的Gateway配置。
spring:
application:
name: gateway-service
cloud:
gateway:
routes:
- id: user-service
uri: http://localhost:8081
predicates:
- Path=/users/**
- id: order-service
uri: http://localhost:8082
predicates:
- Path=/orders/**
- id: product-service
uri: http://localhost:8083
predicates:
- Path=/products/**
default-filters:
- AddRequestHeader=X-Global-Header, Global
server:
port: 8080
2. 编写主应用程序类
在 src/main/java/com/example/gateway
目录下,创建一个主应用程序类 GatewayServiceApplication.java
:
package com.example.gateway;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class GatewayServiceApplication {
public static void main(String[] args) {
SpringApplication.run(GatewayServiceApplication.class, args);
}
}
启动后端服务
为了测试Gateway服务,我们需要一些后端服务。我们将创建三个简单的Spring Boot服务,分别代表用户服务、订单服务和产品服务。
1. 用户服务
创建一个新的Spring Boot项目 user-service
并添加以下依赖:
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
</dependencies>
创建主应用程序类:
package com.example.userservice;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@SpringBootApplication
@RestController
public class UserServiceApplication {
public static void main(String[] args) {
SpringApplication.run(UserServiceApplication.class, args);
}
@GetMapping("/users")
public String getUsers() {
return "List of users";
}
}
在 application.yml
中配置:
server:
port: 8081
2. 订单服务
创建一个新的Spring Boot项目 order-service
并添加以下依赖:
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
</dependencies>
创建主应用程序类:
package com.example.orderservice;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@SpringBootApplication
@RestController
public class OrderServiceApplication {
public static void main(String[] args) {
SpringApplication.run(OrderServiceApplication.class, args);
}
@GetMapping("/orders")
public String getOrders() {
return "List of orders";
}
}
在 application.yml
中配置:
server:
port: 8082
3. 产品服务
创建一个新的Spring Boot项目 product-service
并添加以下依赖:
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
</dependencies>
创建主应用程序类:
package com.example.productservice;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@SpringBootApplication
@RestController
public class ProductServiceApplication {
public static void main(String[] args) {
SpringApplication.run(ProductServiceApplication.class, args);
}
@GetMapping("/products")
public String getProducts() {
return "List of products";
}
}
在 application.yml
中配置:
server:
port: 8083
启动和测试
- 启动用户服务、订单服务和产品服务:
# 启动用户服务
java -jar user-service.jar
# 启动订单服务
java -jar order-service.jar
# 启动产品服务
java -jar product-service.jar
- 启动Gateway服务:
java -jar gateway-service.jar
- 测试路由:
- 访问
http://localhost:8080/users
应该返回 “List of users” - 访问
http://localhost:8080/orders
应该返回 “List of orders” - 访问
http://localhost:8080/products
应该返回 “List of products”
添加更多功能
1. 日志和监控
通过集成Spring Boot Actuator,Spring Cloud Gateway可以提供一组生产就绪的监控端点。
在 application.yml
中启用Actuator端点:
management:
endpoints:
web:
exposure:
include: "*"
你可以通过以下URL访问Actuator端点:
/actuator/health
/actuator/metrics
/actuator/gateway/routes
2. 安全配置
你可以使用Spring Security对Gateway进行安全配置。
在 pom.xml
中添加依赖:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
在 application.yml
中配置基本认证:
spring:
security:
user:
name: user
password: password
创建一个安全配置类:
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.security.config.annotation.web.reactive.EnableWebFluxSecurity;
import org.springframework.security.config.web.server.ServerHttpSecurity;
import org.springframework.security.web.server.SecurityWebFilterChain;
@Configuration
@EnableWebFluxSecurity
public class SecurityConfig {
@Bean
public SecurityWebFilterChain securityWebFilterChain(ServerHttpSecurity http) {
http
.authorizeExchange()
.anyExchange().authenticated()
.and()
.httpBasic()
.and()
.formLogin();
return http.build();
}
}
3. 自定义过滤器
你可以根据特定需求编写自定义过滤器。
创建一个自定义过滤器类:
import org.springframework.cloud.gateway.filter.GatewayFilter;
import org.springframework.cloud.gateway.filter.GatewayFilterChain;
import org.springframework.cloud.gateway.filter.factory.AbstractGatewayFilterFactory;
import org.springframework.http.HttpHeaders;
import org.springframework.stereotype.Component;
import org.springframework.web.server.ServerWebExchange;
import reactor.core.publisher.Mono;
@Component
public class CustomFilter extends AbstractGatewayFilterFactory<CustomFilter.Config> {
public CustomFilter() {
super(Config.class);
}
@Override
public GatewayFilter apply(Config config) {
return (exchange, chain) -> {
// 自定义过滤逻辑
ServerWebExchange modifiedExchange = exchange.mutate()
.request(exchange.getRequest().mutate()
.header(HttpHeaders.AUTHORIZATION, "Bearer my-token")
.build())
.build();
return chain.filter(modifiedExchange);
};
}
public static class Config {
// 配置属性
}
}
在 application.yml
中应用自定义过滤器:
spring:
cloud:
gateway:
routes:
- id: custom-filter-service
uri: http://localhost:8085
predicates:
- Path=/custom/**
filters:
- name: CustomFilter
结论
通过本文的讲解,你应该已经学会了如何创建一个简单的Gateway服务。我们详细介绍了如何配置路由、启动后端服务、测试Gateway服务以及添加日志、监控、安全配置和自定义过滤器等功能。
希望这篇文章能帮助你顺利创建和配置Gateway服务。如果你有任何问题或建议,欢迎在评论中与我们分享。
这些就是关于【gateway 入门】创建一个简单的Gateway服务的详细介绍。
这里是爪磕,感谢您的到来与关注,我们将持续为您带来优质的文章。
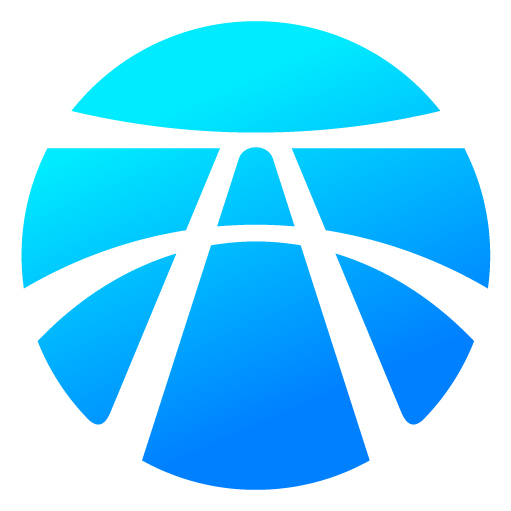
开放原子开发者工作坊旨在鼓励更多人参与开源活动,与志同道合的开发者们相互交流开发经验、分享开发心得、获取前沿技术趋势。工作坊有多种形式的开发者活动,如meetup、训练营等,主打技术交流,干货满满,真诚地邀请各位开发者共同参与!
更多推荐
所有评论(0)