Opencv数据类型(二):Rect类和RotatedRect类
文章目录1、Rect类的一些别名,如Rect2i,Rect2f, Rect2d等等typedef Rect_<int> Rect2i;typedef Rect_<float> Rect2f;typedef Rect_<double> Rect2d;typedef Rect2i Rect;2、Rect_类的构造函数,Rect类包含Point类的成员x...
·
一、cv::Rect类对象
1、Rect类的一些别名,如Rect2i,Rect2f, Rect2d等等
typedef Rect_<int> Rect2i;
typedef Rect_<float> Rect2f;
typedef Rect_<double> Rect2d;
typedef Rect2i Rect;
2、Rect_类的构造函数,Rect类包含Point类的成员x和y(矩形左上角)和Size类的成员width和heigh。
template<typename _Tp> inline
Rect_<_Tp>::Rect_()
: x(0), y(0), width(0), height(0) {}
template<typename _Tp> inline
Rect_<_Tp>::Rect_(_Tp _x, _Tp _y, _Tp _width, _Tp _height)
: x(_x), y(_y), width(_width), height(_height) {}
template<typename _Tp> inline
Rect_<_Tp>::Rect_(const Rect_<_Tp>& r)
: x(r.x), y(r.y), width(r.width), height(r.height) {}
template<typename _Tp> inline
Rect_<_Tp>::Rect_(const Point_<_Tp>& org, const Size_<_Tp>& sz)
: x(org.x), y(org.y), width(sz.width), height(sz.height) {}
template<typename _Tp> inline
Rect_<_Tp>::Rect_(const Point_<_Tp>& pt1, const Point_<_Tp>& pt2)
{
x = std::min(pt1.x, pt2.x);
y = std::min(pt1.y, pt2.y);
width = std::max(pt1.x, pt2.x) - x;
height = std::max(pt1.y, pt2.y) - y;
}
3、Rect类函数
template<typename _Tp> class Rect_
{
public:
typedef _Tp value_type;
//! default constructor
Rect_();
Rect_(_Tp _x, _Tp _y, _Tp _width, _Tp _height);
Rect_(const Rect_& r);
Rect_(const Point_<_Tp>& org, const Size_<_Tp>& sz);
Rect_(const Point_<_Tp>& pt1, const Point_<_Tp>& pt2);
Rect_& operator = ( const Rect_& r );
//! the top-left corner
// 返回左上点的坐标,Point类型
Point_<_Tp> tl() const;
//! the bottom-right corner
// 返回右下点的坐标,Pont类型
Point_<_Tp> br() const;
//! size (width, height) of the rectangle
//返回Size(width,height)
Size_<_Tp> size() const;
//! area (width*height) of the rectangle
_Tp area() const;
//! true if empty
// 是否是空,空返回1,非空返回0
//template<typename _Tp> inline
//bool Rect_<_Tp>::empty() const
//{
// return width <= 0 || height <= 0;
//}
bool empty() const;
//! conversion to another data type
template<typename _Tp2> operator Rect_<_Tp2>() const;
//! checks whether the rectangle contains the point
//Point点是否在Rect范围类 是返回1,否返回0
bool contains(const Point_<_Tp>& pt) const;
_Tp x; //!< x coordinate of the top-left corner
_Tp y; //!< y coordinate of the top-left corner
_Tp width; //!< width of the rectangle
_Tp height; //!< height of the rectangle
};
4、Rect对象的覆写操作符
5、举例
int main() {
cv::Rect Rt(0,5,4,8);
cv::Rect Rt2(1, 2, 5, 8);
Rt2 &= Rt;
std::cout << Rt.tl() << std::endl; // cout [0,5] Point
std::cout << Rt.br() << std::endl; // cout [4,13]
std::cout << Rt.size().width << " " <<Rt.size().height << std::endl; // cout 4 8
std::cout << Rt.area() << std::endl; //cout 32
std::cout << Rt.empty() << std::endl; //cout 0
cv::Point p1(1, 6);
std::cout << Rt.contains(p1) << std::endl; //cout 1
cv::Point p2(100, 100);
std::cout << Rt.contains(p2) << std::endl; //cout 0
std::cout << "hello world" << std::endl;
return 0;
}
二、cv::RotatedRect类
1、cv::RotatedRect 类是少数没有使用模板的C++接口类
包含 一个中心点 cv::Point2f,一个大小cv::Size2f和一个额外的角度float的容器,其中float的角度代表图形绕中心店旋转的角度。和cv::Rect不同的是,RotatedRect是以中心为原点的,Rect是以左上角为原点的。
class CV_EXPORTS RotatedRect
{
public:
//! default constructor
//构造函数
RotatedRect();
/** full constructor
@param center The rectangle mass center.
@param size Width and height of the rectangle.
@param angle The rotation angle in a clockwise direction. When the angle is 0, 90, 180, 270 etc.,
the rectangle becomes an up-right rectangle.
*/
RotatedRect(const Point2f& center, const Size2f& size, float angle);
/**
Any 3 end points of the RotatedRect. They must be given in order (either clockwise or
anticlockwise).
*/
RotatedRect(const Point2f& point1, const Point2f& point2, const Point2f& point3);
/** returns 4 vertices of the rectangle
@param pts The points array for storing rectangle vertices. The order is bottomLeft, topLeft, topRight, bottomRight.
*/
// 返回矩形的四个顶点
void points(Point2f pts[]) const;
//! returns the minimal up-right integer rectangle containing the rotated rectangle
//返回包含旋转矩形的最小矩形
Rect boundingRect() const;
//! returns the minimal (exact) floating point rectangle containing the rotated rectangle, not intended for use with images
//
Rect_<float> boundingRect2f() const;
//! returns the rectangle mass center
// //矩形的质心
Point2f center;
//! returns width and height of the rectangle
Size2f size;
//! returns the rotation angle. When the angle is 0, 90, 180, 270 etc., the rectangle becomes an up-right rectangle.
//旋转角度,当角度为0、90、180、270等时,矩形就成了一个直立的矩形
float angle;
};
2、图解RotatedRect
感谢 https://blog.csdn.net/u011574296/article/details/71405239 提供的图
3、举例
int main() {
cv::RotatedRect rr(cv::Point2f(2.5,2.5), cv::Size(10,10), 90);
cv::Point2f p2[4];
std::cout << rr.center << " " << rr.size.width << " " << rr.size.height << " " << rr.angle << std::endl; // [2.5, 2.5] [10 x 10] 0.5
// cout [2.5, 2.5] 10 10 90
rr.points(p2);
std::cout << p2[0] << " " << p2[1] << " " << p2[2] << " " << p2[3]<< std::endl;
//cout [-2.5, -2.5] [7.5, -2.5] [7.5, 7.5] [-2.5, 7.5]
// cv::RotatedRect rr2(cv::Point2f(2.5,2.5), cv::Point2f(5.5,5.5));
std::cout << "hello world" << std::endl;
return 0;
}
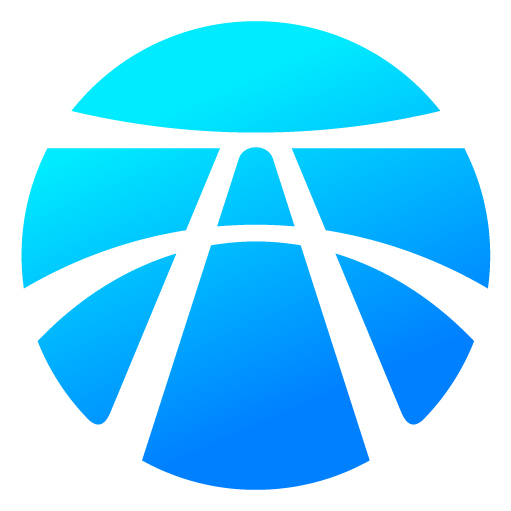
开放原子开发者工作坊旨在鼓励更多人参与开源活动,与志同道合的开发者们相互交流开发经验、分享开发心得、获取前沿技术趋势。工作坊有多种形式的开发者活动,如meetup、训练营等,主打技术交流,干货满满,真诚地邀请各位开发者共同参与!
更多推荐
所有评论(0)