HttpClient详解及如何设置忽略SSL
一、简介HttpClient 是Apache Jakarta Common 下的子项目,可以用来提供高效的、最新的、功能丰富的支持 HTTP 协议的客户端编程工具包,并且它支持 HTTP 协议最新的版本和建议。HttpClient 是 Apache Jakarta Common 下的子项目,用来提供高效的、最新的、功能丰富的支持 HTTP 协议的客户端编程工具包,并且它支持 HTTP 协议...
一、简介
HttpClient 是Apache Jakarta Common 下的子项目,可以用来提供高效的、最新的、功能丰富的支持 HTTP 协议的客户端编程工具包,并且它支持 HTTP 协议最新的版本和建议。
HttpClient 是 Apache Jakarta Common 下的子项目,用来提供高效的、最新的、功能丰富的支持 HTTP 协议的客户端编程工具包,并且它支持 HTTP 协议最新的版本和建议。HttpClient 已经应用在很多的项目中,比如 Apache Jakarta 上很著名的另外两个开源项目 Cactus 和 HTMLUnit 都使用了 HttpClient。现在HttpClient最新版本为 HttpClient 4.5.x
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpclient</artifactId>
<version>4.5.6</version>
</dependency>
二、基本功能
- 实现了所有 HTTP 的方法(GET,POST,PUT,HEAD 等)
- 支持自动转向
- 支持 HTTPS 协议
- 支持代理服务器等
- 2.1 GET方法
/**
* 发送 get请求
*/
public void get() {
CloseableHttpClient httpclient = HttpClients.createDefault();
try {
// 创建httpget.
HttpGet httpget = new HttpGet("http://www.baidu.com/");
System.out.println("executing request " + httpget.getURI());
// 执行get请求.
CloseableHttpResponse response = httpclient.execute(httpget);
try {
// 获取响应实体
HttpEntity entity = response.getEntity();
System.out.println("--------------------------------------");
// 打印响应状态
System.out.println(response.getStatusLine());
if (entity != null) {
// 打印响应内容长度
System.out.println("Response content length: " + entity.getContentLength());
// 打印响应内容
System.out.println("Response content: " + EntityUtils.toString(entity));
}
System.out.println("------------------------------------");
} finally {
response.close();
}
} catch (ClientProtocolException e) {
e.printStackTrace();
} catch (ParseException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
} finally {
// 关闭连接,释放资源
try {
httpclient.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
- 2.2 POST方法
/**
* 发送 post请求访问本地应用并根据传递参数不同返回不同结果
*/
public void post() {
// 创建默认的httpClient实例.
CloseableHttpClient httpclient = HttpClients.createDefault();
// 创建httppost
HttpPost httppost = new HttpPost("http://localhost:8080/myDemo/Ajax/serivceJ.action");
// 创建参数队列
List<NameValuePair> formparams = new ArrayList<NameValuePair>();
formparams.add(new BasicNameValuePair("type", "house"));
UrlEncodedFormEntity uefEntity;
try {
uefEntity = new UrlEncodedFormEntity(formparams, "UTF-8");
httppost.setEntity(uefEntity);
System.out.println("executing request " + httppost.getURI());
CloseableHttpResponse response = httpclient.execute(httppost);
try {
HttpEntity entity = response.getEntity();
if (entity != null) {
System.out.println("--------------------------------------");
System.out.println("Response content: " + ntityUtils.toString(entity, "UTF-8"));
System.out.println("--------------------------------------");
}
} finally {
response.close();
}
} catch (ClientProtocolException e) {
e.printStackTrace();
} catch (UnsupportedEncodingException e1) {
e1.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
} finally {
// 关闭连接,释放资源
try {
httpclient.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
参考资料:
https://www.kancloud.cn/longxuan/httpclient-arron/117499 https://blog.csdn.net/xiaoxian8023 https://blog.csdn.net/w372426096/article/details/82713315
三、常见问题之一:如何设置忽略SSL
有时我们需要访问一些采用Https安全协议的网站,我们可以定制SSL/TLS
的方式来访问该网站,HttpClient是支持的。定制SSL/TLS下节记录。采用定制SSL/TLS的方式需要导出对应网站的证书,证书还有可能过期,需要重新导入,这时可以采用绕过证书的方式。当然,有的网站是Https但是浏览器还是显示不安全,就没办法导出证书,比如12306。
解决常见报错:
方式一:
HttpHost proxy = new HttpHost("10.31.16.216",18103);
CloseableHttpClient httpClient = HttpClients.custom()
.setProxy(proxy)
.setHostnameVerifier(new AllowAllHostnameVerifier())
.setSslcontext(new SSLContextBuilder().loadTrustMaterial(null, new TrustStrategy()
{
public boolean isTrusted(X509Certificate[] arg0, String arg1) throws CertificateException
{
return true;
}
}).build()).build();
HttpURLConnection configurator =new HttpURLConnection(null) {
@Override
public void connect() throws IOException {
// TODO Auto-generated method stub
}
@Override
public boolean usingProxy() {
// TODO Auto-generated method stub
return false;
}
@Override
public void disconnect() {
// TODO Auto-generated method stub
}
};
@PostConstruct
public void init() throws Exception{
RequestConfig httpConfig=RequestConfig.custom()
.setExpectContinueEnabled(false)
.setConnectionRequestTimeout(Integer.valueOf("configurator.getTransportConfig().getConnectionTimeout()"))
.setConnectTimeout(Integer.valueOf("configurator.getTransportConfig().getConnectionTimeout()"))
.setSocketTimeout(Integer.valueOf("configurator.getTransportConfig().getsocketTimeout()"))
.build();
SSLContext sslContextIgnore=SSLContexts.custom().loadTrustMaterial((cert,para)->true).build();
//SSLContext sslContextIgnore=SSLContexts.custom().build();
SSLConnectionSocketFactory socketFactoryIgnore=new SSLConnectionSocketFactory(sslContextIgnore,NoopHostnameVerifier.INSTANCE);
HttpClient httpClient= HttpClients.custom()
.setDefaultRequestConfig(httpConfig)
.setMaxConnPerRoute(Integer.valueOf("configurator.getTransportConfig().getPoolMmaxSizePerRoute()"))
.setSSLContext(sslContextIgnore)
.setSSLSocketFactory(socketFactoryIgnore)
.build();
}
}
方式二:
SSLContextBuilder builder = new SSLContextBuilder();
builder.loadTrustMaterial(null, new TrustSelfSignedStrategy());
SSLConnectionSocketFactory sslsf = new SSLConnectionSocketFactory(
builder.build());
CloseableHttpClient httpclient = HttpClients.custom().setSSLSocketFactory(
sslsf).build();
HttpGet httpGet = new HttpGet("https://www.baidu.com");
CloseableHttpResponse response = httpclient.execute(httpGet);
try {
System.out.println(response.getStatusLine());
HttpEntity entity = response.getEntity();
EntityUtils.consume(entity);
}
finally {
response.close();
}
方式三:
SSLContextBuilder builder = SSLContexts.custom();
builder.loadTrustMaterial(null, new TrustStrategy() {
@Override
public boolean isTrusted(X509Certificate[] chain, String authType)
throws CertificateException {
return true;
}
});
SSLContext sslContext = builder.build();
SSLConnectionSocketFactory sslsf = new SSLConnectionSocketFactory(
sslContext, new X509HostnameVerifier() {
@Override
public void verify(String host, SSLSocket ssl)
throws IOException {
}
@Override
public void verify(String host, X509Certificate cert)
throws SSLException {
}
@Override
public void verify(String host, String[] cns,
String[] subjectAlts) throws SSLException {
}
@Override
public boolean verify(String s, SSLSession sslSession) {
return true;
}
});
Registry<ConnectionSocketFactory> socketFactoryRegistry = RegistryBuilder
.<ConnectionSocketFactory> create().register("https", sslsf)
.build();
PoolingHttpClientConnectionManager cm = new PoolingHttpClientConnectionManager(
socketFactoryRegistry);
CloseableHttpClient httpclient = HttpClients.custom()
.setConnectionManager(cm).build();
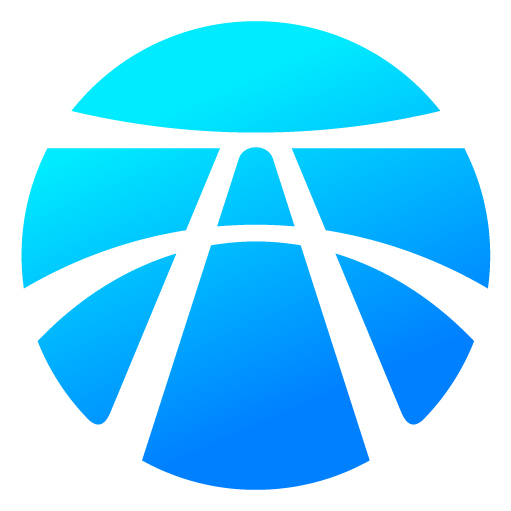
开放原子开发者工作坊旨在鼓励更多人参与开源活动,与志同道合的开发者们相互交流开发经验、分享开发心得、获取前沿技术趋势。工作坊有多种形式的开发者活动,如meetup、训练营等,主打技术交流,干货满满,真诚地邀请各位开发者共同参与!
更多推荐
所有评论(0)