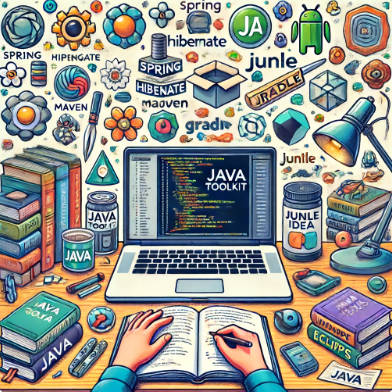
Java工具库系列(八):JUnit
JUnit 是一个开源的 Java 单元测试框架,它提供了一组注解和断言,用于编写和运行测试。JUnit 的目标是简化单元测试的编写和执行,使开发者能够更容易地发现和修复代码中的问题。JUnit 是一个强大的单元测试框架,通过提供丰富的注解和断言方法,极大地简化了 Java 应用程序的测试工作。在本篇文章中,我们介绍了 JUnit 的核心功能和使用方法。在接下来的文章中,我们将继续探讨更多的 Ja
Java工具库系列(八):JUnit
在软件开发过程中,编写测试代码是保证代码质量的重要手段。JUnit 是 Java 中最流行的单元测试框架之一,它为测试驱动开发(TDD)和行为驱动开发(BDD)提供了强有力的支持。本文将介绍 JUnit 的核心功能及其使用方法,帮助你在项目中更高效地进行单元测试。
一、JUnit 简介
JUnit 是一个开源的 Java 单元测试框架,它提供了一组注解和断言,用于编写和运行测试。JUnit 的目标是简化单元测试的编写和执行,使开发者能够更容易地发现和修复代码中的问题。
二、JUnit 的安装
要在项目中使用 JUnit,你需要在项目的构建工具中添加 JUnit 的依赖。例如,如果你使用 Maven,你可以在 pom.xml
文件中添加以下依赖:
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter-api</artifactId>
<version>5.8.2</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter-engine</artifactId>
<version>5.8.2</version>
<scope>test</scope>
</dependency>
对于 Gradle,你可以添加以下内容到 build.gradle
文件:
testImplementation 'org.junit.jupiter:junit-jupiter-api:5.8.2'
testRuntimeOnly 'org.junit.jupiter:junit-jupiter-engine:5.8.2'
三、JUnit 的核心功能
1. 基本测试
JUnit 提供了一组注解用于定义测试方法和生命周期方法。以下是一个简单的测试类示例:
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class SimpleTest {
@Test
public void testAddition() {
assertEquals(2, 1 + 1, "1 + 1 should equal 2");
}
}
2. 生命周期方法
JUnit 提供了多种生命周期注解,用于在测试方法执行前后进行初始化和清理工作:
@BeforeEach
:在每个测试方法执行之前执行@AfterEach
:在每个测试方法执行之后执行@BeforeAll
:在所有测试方法执行之前执行@AfterAll
:在所有测试方法执行之后执行
import org.junit.jupiter.api.BeforeEach;
import org.junit.jupiter.api.AfterEach;
import org.junit.jupiter.api.BeforeAll;
import org.junit.jupiter.api.AfterAll;
import org.junit.jupiter.api.Test;
public class LifecycleTest {
@BeforeAll
static void setupAll() {
System.out.println("Before all tests");
}
@BeforeEach
void setup() {
System.out.println("Before each test");
}
@Test
void testOne() {
System.out.println("Test one");
}
@Test
void testTwo() {
System.out.println("Test two");
}
@AfterEach
void teardown() {
System.out.println("After each test");
}
@AfterAll
static void teardownAll() {
System.out.println("After all tests");
}
}
3. 断言
JUnit 提供了一组断言方法,用于验证测试结果。常用的断言方法包括:
assertEquals
:验证两个值是否相等assertNotEquals
:验证两个值是否不相等assertTrue
:验证条件是否为真assertFalse
:验证条件是否为假assertNull
:验证对象是否为 nullassertNotNull
:验证对象是否不为 null
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.*;
public class AssertionTest {
@Test
public void testAssertions() {
assertEquals(5, 2 + 3, "2 + 3 should equal 5");
assertTrue(3 > 2, "3 is greater than 2");
assertFalse(2 > 3, "2 is not greater than 3");
assertNull(null, "Object should be null");
assertNotNull("Hello", "Object should not be null");
}
}
4. 异常测试
你可以使用 assertThrows
方法来验证代码是否抛出预期的异常。
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertThrows;
public class ExceptionTest {
@Test
public void testException() {
assertThrows(ArithmeticException.class, () -> {
int result = 1 / 0;
}, "Division by zero should throw ArithmeticException");
}
}
5. 参数化测试
JUnit 提供了参数化测试功能,使你可以使用不同的参数多次运行同一个测试方法。
import org.junit.jupiter.params.ParameterizedTest;
import org.junit.jupiter.params.provider.ValueSource;
import static org.junit.jupiter.api.Assertions.assertTrue;
public class ParameterizedTestExample {
@ParameterizedTest
@ValueSource(strings = {"racecar", "radar", "level"})
public void testPalindrome(String candidate) {
assertTrue(isPalindrome(candidate));
}
public boolean isPalindrome(String str) {
int n = str.length();
for (int i = 0; i < (n / 2); i++) {
if (str.charAt(i) != str.charAt(n - i - 1)) {
return false;
}
}
return true;
}
}
6. 条件测试
JUnit 提供了条件测试功能,使你可以根据条件执行或跳过测试方法。
import org.junit.jupiter.api.Test;
import org.junit.jupiter.api.condition.EnabledOnOs;
import org.junit.jupiter.api.condition.OS;
public class ConditionalTest {
@Test
@EnabledOnOs(OS.WINDOWS)
public void testOnlyOnWindows() {
System.out.println("This test runs only on Windows");
}
@Test
@EnabledOnOs(OS.LINUX)
public void testOnlyOnLinux() {
System.out.println("This test runs only on Linux");
}
}
四、JUnit 的高级功能
1. 扩展模型
JUnit 5 提供了一个强大的扩展模型,使你可以创建自定义的扩展来增强测试功能。
import org.junit.jupiter.api.extension.BeforeAllCallback;
import org.junit.jupiter.api.extension.ExtensionContext;
import org.junit.jupiter.api.extension.RegisterExtension;
public class CustomExtensionTest {
static class CustomExtension implements BeforeAllCallback {
@Override
public void beforeAll(ExtensionContext context) {
System.out.println("Custom extension before all tests");
}
}
@RegisterExtension
static CustomExtension customExtension = new CustomExtension();
@Test
void test() {
System.out.println("Test method");
}
}
2. 嵌套测试
JUnit 5 支持嵌套测试类,使你可以更好地组织和分组测试。
import org.junit.jupiter.api.Nested;
import org.junit.jupiter.api.Test;
public class NestedTest {
@Nested
class InnerTest {
@Test
void innerTest() {
System.out.println("Inner test");
}
}
@Test
void outerTest() {
System.out.println("Outer test");
}
}
五、总结
JUnit 是一个强大的单元测试框架,通过提供丰富的注解和断言方法,极大地简化了 Java 应用程序的测试工作。在本篇文章中,我们介绍了 JUnit 的核心功能和使用方法。在接下来的文章中,我们将继续探讨更多的 Java 工具库,帮助你在开发过程中事半功倍。
如果你有任何问题或建议,欢迎在评论区留言,我们将会一一解答。祝大家编码愉快!
敬请期待下一篇文章,我们将详细介绍另一个强大的 Java 工具库。
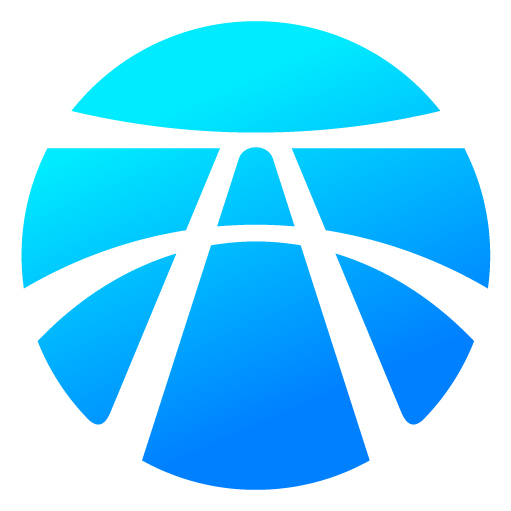
开放原子开发者工作坊旨在鼓励更多人参与开源活动,与志同道合的开发者们相互交流开发经验、分享开发心得、获取前沿技术趋势。工作坊有多种形式的开发者活动,如meetup、训练营等,主打技术交流,干货满满,真诚地邀请各位开发者共同参与!
更多推荐
所有评论(0)