WPF编程,Live Charts使用说明(10)——自定义工具提示和图例
默认情况下,每个需要工具提示或图例的图表都将初始化DefaultLegend 或 DefaultTooltip的新实例 。自定义默认值可以自定义这些类,例如背景色,项目符号大小或方向:<lvc:CartesianChart Series="{Binding SeriesCollection}"><lvc:CartesianChart.ChartLegend...
·
默认情况下,每个需要工具提示或图例的图表都将初始化DefaultLegend 或 DefaultTooltip的新实例 。
自定义默认值
- 可以自定义这些类,例如背景色,项目符号大小或方向:
<lvc:CartesianChart Series="{Binding SeriesCollection}">
<lvc:CartesianChart.ChartLegend>
<lvc:DefaultLegend BulletSize="20" Background="Red"/>
</lvc:CartesianChart.ChartLegend>
<lvc:CartesianChart.DataTooltip>
<lvc:DefaultTooltip BulletSize="20" Background="Gray"/>
</lvc:CartesianChart.DataTooltip>
</lvc:CartesianChart>
- 还可以设置工具提示的选择模式,例如,使用下一个代码,我们强制工具提示仅显示悬停的点。
<lvc:CartesianChart Series="{Binding SeriesCollection}">
<lvc:CartesianChart.DataTooltip>
<lvc:DefaultTooltip SelectionMode="OnlySender" />
</lvc:CartesianChart.DataTooltip>
</lvc:CartesianChart>
- 事例
<lvc:CartesianChart>
<lvc:CartesianChart.Resources>
<Style TargetType="lvc:DefaultTooltip">
<Setter Property="Background" Value="DarkOrange"></Setter>
<Setter Property="Foreground" Value="White"></Setter>
<Setter Property="ShowTitle" Value="False"></Setter><!--new property-->
<Setter Property="ShowSeries" Value="False"></Setter><!--new property-->
<Setter Property="FontSize" Value="16"></Setter>
<Setter Property="FontWeight" Value="Bold"></Setter>
<Setter Property="CornerRadius" Value="20"></Setter>
<Setter Property="Width" Value="40"></Setter>
<Setter Property="Height" Value="40"></Setter>
<Setter Property="BorderThickness" Value="0"></Setter>
</Style>
</lvc:CartesianChart.Resources>
<lvc:CartesianChart.Series>
<lvc:LineSeries Values="4,2,6,4"></lvc:LineSeries>
</lvc:CartesianChart.Series>
</lvc:CartesianChart>
自定义提示
- 在解决方案资源管理器中右键单击,然后单击添加->新建项->浏览类,将文件命名为CustomerVm.cs并将生成的文件内容替换为:
namespace Wpf.CartesianChart.CustomTooltipAndLegend
{
public class CustomerVm
{
public string Name { get; set; }
public string LastName { get; set; }
public int Phone { get; set; }
public int PurchasedItems { get; set; }
}
}
- 在解决方案资源管理器中右键单击Add- > New Item-> Browse for User Control(WPF),将其命名为UserControl CustomersTooltip。
将CustomersTooltip.xaml替换为:
<UserControl x:Class="Wpf.CartesianChart.CustomTooltipAndLegend.CustomersTooltip"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:wpf="clr-namespace:LiveCharts.Wpf;assembly=LiveCharts.Wpf"
xmlns:local="clr-namespace:Wpf.CartesianChart.CustomTooltipAndLegend"
mc:Ignorable="d"
d:DesignHeight="300" d:DesignWidth="300"
d:DataContext="{d:DesignInstance local:CustomersTooltip}"
Background="#E4555555" Padding="20 10" BorderThickness="2" BorderBrush="#555555">
<ItemsControl ItemsSource="{Binding Data.Points}" Grid.IsSharedSizeScope="True">
<ItemsControl.ItemTemplate>
<DataTemplate DataType="{x:Type wpf:DataPointViewModel}">
<Grid Margin="2">
<Grid.ColumnDefinitions>
<ColumnDefinition Width="Auto"/>
<ColumnDefinition Width="Auto" SharedSizeGroup="Title"/>
<ColumnDefinition Width="Auto" SharedSizeGroup="LastName"/>
<ColumnDefinition Width="Auto" SharedSizeGroup="Phone"/>
<ColumnDefinition Width="Auto" SharedSizeGroup="PurchasedItems"/>
</Grid.ColumnDefinitions>
<Rectangle Grid.Column="0" Stroke="{Binding Series.Stroke}" Fill="{Binding Series.Fill}"
Height="15" Width="15"></Rectangle>
<TextBlock Grid.Column="1" Text="{Binding ChartPoint.Instance.(local:CustomerVm.Name)}"
Margin="5 0 0 0" VerticalAlignment="Center" Foreground="White"/>
<TextBlock Grid.Column="2" Text="{Binding ChartPoint.Instance.(local:CustomerVm.LastName)}"
Margin="5 0 0 0" VerticalAlignment="Center" Foreground="White"/>
<TextBlock Grid.Column="3" Text="{Binding ChartPoint.Instance.(local:CustomerVm.Phone),
StringFormat=Phone: {0}}"
Margin="5 0 0 0" VerticalAlignment="Center" Foreground="White"/>
<TextBlock Grid.Column="4" Text="{Binding ChartPoint.Instance.(local:CustomerVm.PurchasedItems),
StringFormat=Purchased Items: {0:N}}"
Margin="5 0 0 0" VerticalAlignment="Center" Foreground="White"/>
</Grid>
</DataTemplate>
</ItemsControl.ItemTemplate>
</ItemsControl>
</UserControl>
- 以及用户控件后面的代码(CustomersTooltip.xaml.cs)
using System.ComponentModel;
using LiveCharts;
using LiveCharts.Wpf;
namespace Wpf.CartesianChart.CustomTooltipAndLegend
{
public partial class CustomersTooltip : IChartTooltip
{
private TooltipData _data;
public CustomersTooltip()
{
InitializeComponent();
//LiveCharts will inject the tooltip data in the Data property
//your job is only to display this data as required
DataContext = this;
}
public event PropertyChangedEventHandler PropertyChanged;
public TooltipData Data
{
get { return _data; }
set
{
_data = value;
OnPropertyChanged("Data");
}
}
public TooltipSelectionMode? SelectionMode { get; set; }
protected virtual void OnPropertyChanged(string propertyName = null)
{
if (PropertyChanged != null)
PropertyChanged.Invoke(this, new PropertyChangedEventArgs(propertyName));
}
}
}
CustomersTooltip实现了IChartTooltip, 此接口需要我们的用户控件实现INotifyPropertyChanged 和一个类型为TooltipData的新属性Data,LiveCharts将注入所有有关当前点的信息以显示在工具提示中,您的工作是显示此数据根据您的要求。
注意,我们使用了用户控件的DataContext属性,并将Data.Points属性绑定到我们的ItemsControl, 以根据需要显示当前点。
我们还创建一个具有自定义样式的简单自定义图例,添加另一个User控件并将其命名为 CustomersLegend,其逻辑与自定义工具提示相同,实现IChartLegend, 然后通过LiveCharts处理注入的数据
<UserControl x:Class="Wpf.CartesianChart.CustomTooltipAndLegend.CustomersLegend"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:local="clr-namespace:Wpf.CartesianChart.CustomTooltipAndLegend"
xmlns:lvc="clr-namespace:LiveCharts.Wpf;assembly=LiveCharts.Wpf"
mc:Ignorable="d"
Background="#555555" BorderThickness="2" Padding="20 10" BorderBrush="AntiqueWhite"
d:DataContext="{d:DesignInstance local:CustomersLegend}">
<ItemsControl ItemsSource="{Binding Series}" Grid.IsSharedSizeScope="True">
<ItemsControl.ItemTemplate>
<DataTemplate DataType="{x:Type lvc:SeriesViewModel}">
<Grid Margin="2">
<Grid.ColumnDefinitions>
<ColumnDefinition Width="Auto"/>
<ColumnDefinition Width="Auto" SharedSizeGroup="Title"/>
</Grid.ColumnDefinitions>
<Rectangle Grid.Column="0" Stroke="{Binding Stroke}" Fill="{Binding Fill}"
Width="15" Height="15"/>
<TextBlock Grid.Column="1" Margin="4 0" Text="{Binding Title}" Foreground="White" VerticalAlignment="Center" />
</Grid>
</DataTemplate>
</ItemsControl.ItemTemplate>
</ItemsControl>
</UserControl>
using System.Collections.Generic;
using System.ComponentModel;
using System.Windows.Controls;
using LiveCharts.Wpf;
namespace Wpf.CartesianChart.CustomTooltipAndLegend
{
public partial class CustomersLegend : UserControl, IChartLegend
{
private List<SeriesViewModel> _series;
public CustomersLegend()
{
InitializeComponent();
DataContext = this;
}
public List<SeriesViewModel> Series
{
get { return _series; }
set
{
_series = value;
OnPropertyChanged("Series");
}
}
public event PropertyChangedEventHandler PropertyChanged;
protected virtual void OnPropertyChanged(string propertyName = null)
{
if (PropertyChanged != null)
PropertyChanged.Invoke(this, new PropertyChangedEventArgs(propertyName));
}
}
}
最后使用:
<UserControl x:Class="Wpf.CartesianChart.CustomTooltipAndLegend.CustomTooltipAndLegendExample"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:lvc="clr-namespace:LiveCharts.Wpf;assembly=LiveCharts.Wpf"
xmlns:local="clr-namespace:Wpf.CartesianChart.CustomTooltipAndLegend"
mc:Ignorable="d"
d:DesignHeight="300" d:DesignWidth="300" d:DataContext="{d:DesignInstance local:CustomTooltipAndLegendExample}">
<Grid>
<lvc:CartesianChart LegendLocation="Right">
<lvc:CartesianChart.Series>
<lvc:ColumnSeries Title="2016 Customers" Values="{Binding Customers}"></lvc:ColumnSeries>
</lvc:CartesianChart.Series>
<lvc:CartesianChart.AxisX >
<lvc:Axis Labels="{Binding Labels}" LabelsRotation="-15">
<lvc:Axis.Separator>
<lvc:Separator Step="1"></lvc:Separator>
</lvc:Axis.Separator>
</lvc:Axis>
</lvc:CartesianChart.AxisX>
<lvc:CartesianChart.DataTooltip>
<local:CustomersTooltip/>
</lvc:CartesianChart.DataTooltip>
<lvc:CartesianChart.ChartLegend>
<local:CustomersLegend></local:CustomersLegend>
</lvc:CartesianChart.ChartLegend>
</lvc:CartesianChart>
</Grid>
</UserControl>
后台:
using System.Windows.Controls;
using LiveCharts;
using LiveCharts.Configurations;
namespace Wpf.CartesianChart.CustomTooltipAndLegend
{
public partial class CustomTooltipAndLegendExample : UserControl
{
public CustomTooltipAndLegendExample()
{
InitializeComponent();
Customers = new ChartValues<CustomerVm>
{
new CustomerVm
{
Name = "Irvin",
LastName = "Hale",
Phone = 123456789,
PurchasedItems = 8
},
new CustomerVm
{
Name = "Malcolm",
LastName = "Revees",
Phone = 098765432,
PurchasedItems = 3
},
new CustomerVm
{
Name = "Anne",
LastName = "Rios",
Phone = 758294026,
PurchasedItems = 6
},
new CustomerVm
{
Name = "Vivian",
LastName = "Howell",
Phone = 309382739,
PurchasedItems = 3
},
new CustomerVm
{
Name = "Caleb",
LastName = "Roy",
Phone = 682902826,
PurchasedItems = 2
}
};
Labels = new[] { "Irvin", "Malcolm", "Anne", "Vivian", "Caleb" };
//let create a mapper so LiveCharts know how to plot our CustomerViewModel class
var customerVmMapper = Mappers.Xy<CustomerVm>()
.X((value, index) => index) // lets use the position of the item as X
.Y(value => value.PurchasedItems); //and PurchasedItems property as Y
//lets save the mapper globally
Charting.For<CustomerVm>(customerVmMapper);
DataContext = this;
}
public ChartValues<CustomerVm> Customers { get; set; }
public string[] Labels { get; set; }
}
}
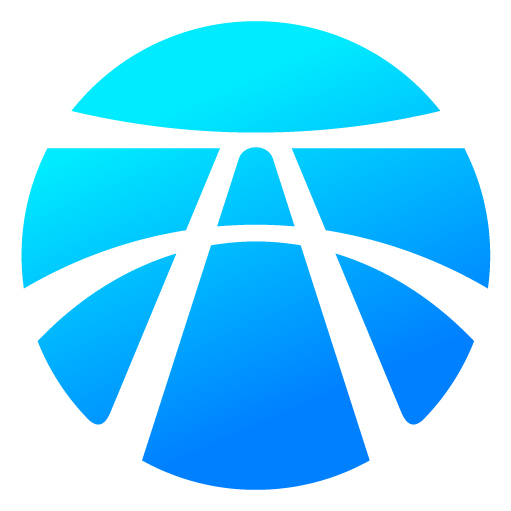
开放原子开发者工作坊旨在鼓励更多人参与开源活动,与志同道合的开发者们相互交流开发经验、分享开发心得、获取前沿技术趋势。工作坊有多种形式的开发者活动,如meetup、训练营等,主打技术交流,干货满满,真诚地邀请各位开发者共同参与!
更多推荐
所有评论(0)