Node.js:node项目中连接postgresql以及基础使用
node项目中连接postgresql以及基础使用
·
1. 使用node-postgres模块连接postgresql
- github地址
node-postgres - 官方文档
node-postgres
2. 基础使用
-
项目中安装node-postgress
npm install pg
-
在项目中连接数据库
最后一句export default client不是必须的,我是为了将数据库连接抽出来全局使用将服务器连接抽成了单独的组件。const {Client} = require('pg') const client = new Client({ user: 'postgres', host: '218.241.129.181', database: 'geo', password: 'p@ssw0rd', port: 5433, }) client.connect(); export default client;
-
基础查询
client.query("select id,node_name,node_type,data_type from department_info where pid = '管理员'", (err, res) => { console.log(err, res) if (err) throw err; client.end(); result.json({ status: 200, message: "数据查询成功!", data: res.rows, dataCount: res.rowCount }) })
其中,res的格式如下:
之中的rows为我们需要的查询结果,rowCount为查询结果数量
3. 基础增删改查操作练习
练习表的字段格式
其中,geo为geometry类型的数据
因为node-postgress中回调函数、promise、async/await都支持,所以都练习了一下
这里的增删改查,都是使用的query方法,即query可以直接执行一条SQL语句,并返回查询结果放在rows数组中。
① 增
insertByCallBack(request, result) {
let sql = `INSERT INTO huankaitest ( NAME, age, geo, date, date1 )
VALUES
\t(
\t'test',
\t1,
\t( SELECT ST_GeomFromText ( 'point(0 0)', 4326 ) ),
\t( SELECT now( ) :: TIMESTAMP ( 0 ) ),
\t( SELECT to_timestamp ( '2020-03-21 18:13:59', 'yyyy-MM-dd hh24:mi:ss' ) )
\t)`;
client.query(sql, (err, res) => {
if (err) {
console.log(err);
} else {
result.json({
status: 200,
message: "数据插入成功!"
})
}
})
}
insertByPromise(request, result) {
let sql = `INSERT INTO huankaitest ( NAME, age, geo, date, date1 )
VALUES
\t(
\t'test',
\t1,
\t( SELECT ST_GeomFromText ( 'point(0 0)', 4326 ) ),
\t( SELECT now( ) :: TIMESTAMP ( 0 ) ),
\t( SELECT to_timestamp ( '2020-03-21 18:13:59', 'yyyy-MM-dd hh24:mi:ss' ) )
\t)`;
client
.query(sql)
.then(res => {
result.json({
status: 200,
message: "数据插入成功!"
})
})
.catch(e => {
console.log(e);
})
}
② 删
deleteByCallBack(request, result) {
let sql = `delete from huankaitest where age = 1`;
client.query(sql, (err, res) => {
if (err) {
console.log(err);
} else {
result.json({
statue: 200,
message: "数据删除成功!",
});
}
})
}
deleteByPromise(request, result) {
let sql = `delete from huankaitest where age = 1`;
client
.query(sql)
.then(res => {
result.json({
statue: 200,
message: "数据删除成功!",
});
})
.catch(e => {
console.log(e);
})
}
③ 改
updateByCallBack(request, result) {
let sql = `update huankaitest set name = 'kaisar' where age = 1`;
client.query(sql, (err, res) => {
if (err) {
console.log(err);
} else {
result.json({
statue: 200,
message: "数据更新成功!",
});
}
})
}
updateByPromise(request, result) {
let sql = `update huankaitest set name = 'huankai' where age = 1`;
client
.query(sql)
.then(res => {
result.json({
statue: 200,
message: "数据更新成功!",
});
})
.catch(e => {
console.log(e);
})
}
④ 查
searchByCallBack(request, result) {
let sql = `select * from huankaitest`;
client.query(sql, (err, res) => {
if (err) {
console.log(err);
} else {
result.json({
statue: 200,
count: res.rowCount,
message: "查询成功!",
date: res.rows
});
}
})
}
searchByPromise(request, result) {
let sql = `select * from huankaitest`;
client
.query(sql)
.then(res => {
result.json({
statue: 200,
count: res.rowCount,
message: "查询成功!",
date: res.rows
});
})
.catch(e => {
console.log(e);
})
}
4. 参数化查询
在书写SQL的时候,有可能会产生SQL注入漏洞。
node-postgresql给出的解决方案是参数化查询,即不将查询条件字符串直接放到查询文本中,而是以数组的形式传递到PostgreSQL服务器,参数在服务器上安全的替换到查询中
使用方法1:
- 定义查询sql字符串语句,将查询条件的位置用$n表示
- 将查询条件,放到另外一个数组中,并按照顺序存储。在查询字符串中,以
$1、$2···$n
的形式依次获取第1、2、n个参数。
searchByParams(request, result) {
const sql = "select * from huankaitest where name = $1 and age = $2"
const values = ['test', 1]
client.query(sql, values, (err, res) => {
if (!err) {
result.json({
status: 200,
count: res.rowCount,
message: "查询成功!",
date: res.rows
})
}
})
}
使用方法2:
除了定义两个数组,也可以直接将参数定义为一个对象
注意:如果使用这种方法传递参数与SQL语句,则sql语句必须定义为text!
searchByParams1(request,result){
const query = {
text: "select * from huankaitest where name = $1 and age = $2",
values: ['test', 1]
}
client.query(query, (err, res) => {
if (!err) {
result.json({
status: 200,
count: res.rowCount,
message: "查询成功!",
date: res.rows
})
}
})
}
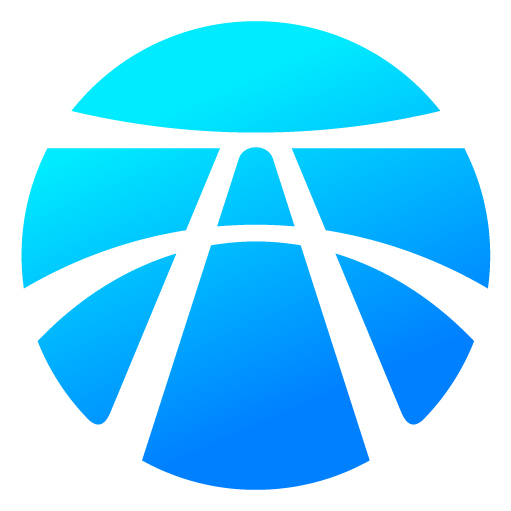
开放原子开发者工作坊旨在鼓励更多人参与开源活动,与志同道合的开发者们相互交流开发经验、分享开发心得、获取前沿技术趋势。工作坊有多种形式的开发者活动,如meetup、训练营等,主打技术交流,干货满满,真诚地邀请各位开发者共同参与!
更多推荐
所有评论(0)