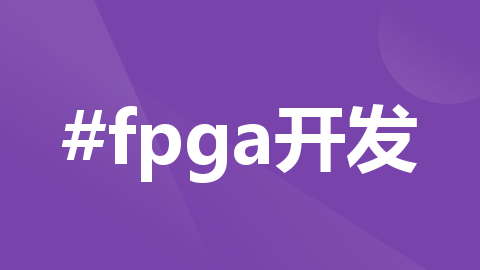
verilog常用技巧 (个人总结版)
Verilog 是一种硬件描述语言(HDL),广泛用于数字电路和系统设计。以下是一些常用的 Verilog 编程技巧以及具体操作,供参考和使用。
Verilog 是一种硬件描述语言(HDL),广泛用于数字电路和系统设计。以下是一些常用的 Verilog 编程技巧以及具体操作,供参考和使用。
1. 模块化设计
模块化设计可以提高代码的可读性和可维护性,将复杂电路划分为多个模块进行设计。
- 定义模块
- 方法:使用
module
和endmodule
关键字定义一个模块。 - 具体步骤:
- 定义模块名称和端口列表。
- 编写模块内部逻辑。
- 使用
endmodule
结束模块定义。
- 示例代码:
module adder ( input wire [3:0] a, input wire [3:0] b, output wire [3:0] sum ); assign sum = a + b; endmodule
- 方法:使用
2. 使用参数化模块
参数化模块可以提高模块的灵活性,允许在实例化时指定参数值。
- 定义参数化模块
- 方法:使用
parameter
关键字定义参数。 - 具体步骤:
- 在模块定义中加入参数。
- 在实例化时指定参数值。
- 示例代码:
module adder #(parameter WIDTH = 4) ( input wire [WIDTH-1:0] a, input wire [WIDTH-1:0] b, output wire [WIDTH-1:0] sum ); assign sum = a + b; endmodule // 实例化带参数的模块 module top; wire [7:0] sum; adder #(8) my_adder ( .a(8'b00001111), .b(8'b00000001), .sum(sum) ); endmodule
- 方法:使用
3. 使用 always
块
always
块用于描述时序逻辑和组合逻辑。
-
描述组合逻辑
- 方法:使用
always @(*)
块。 - 具体步骤:
- 定义
always @(*)
块。 - 在块内编写组合逻辑。
- 定义
- 示例代码:
module mux ( input wire a, input wire b, input wire sel, output reg y ); always @(*) begin if (sel) y = b; else y = a; end endmodule
- 方法:使用
-
描述时序逻辑
- 方法:使用
always @(posedge clk)
块。 - 具体步骤:
- 定义
always @(posedge clk)
块。 - 在块内编写时序逻辑。
- 定义
- 示例代码:
module dff ( input wire clk, input wire d, output reg q ); always @(posedge clk) begin q <= d; end endmodule
- 方法:使用
4. 使用 generate
语句
generate
语句用于生成重复的硬件结构,提高代码的可重用性。
- 定义
generate
块- 方法:使用
generate
和endgenerate
关键字。 - 具体步骤:
- 定义
generate
块。 - 在块内编写生成逻辑。
- 定义
- 示例代码:
module genvar_example; genvar i; generate for (i = 0; i < 8; i = i + 1) begin : gen_block wire a, b, sum; assign sum = a + b; end endgenerate endmodule
- 方法:使用
5. 使用 initial
块
initial
块用于描述仿真时的初始条件和一次性事件。
- 定义
initial
块- 方法:使用
initial
关键字。 - 具体步骤:
- 定义
initial
块。 - 在块内编写初始条件。
- 定义
- 示例代码:
module testbench; reg clk; reg reset; initial begin clk = 0; reset = 1; #5 reset = 0; end always #5 clk = ~clk; endmodule
- 方法:使用
6. 使用 case
语句
case
语句用于描述多路选择器等条件分支逻辑。
- 定义
case
语句- 方法:使用
case
和endcase
关键字。 - 具体步骤:
- 定义
case
语句。 - 在块内编写条件分支。
- 定义
- 示例代码:
module alu ( input wire [1:0] op, input wire [3:0] a, input wire [3:0] b, output reg [3:0] result ); always @(*) begin case (op) 2'b00: result = a + b; 2'b01: result = a - b; 2'b10: result = a & b; 2'b11: result = a | b; default: result = 4'b0000; endcase end endmodule
- 方法:使用
7. 使用 ifdef
语句
ifdef
语句用于条件编译,根据宏定义选择性地编译代码。
- 定义
ifdef
语句- 方法:使用
ifdef
和endif
关键字。 - 具体步骤:
- 定义宏和
ifdef
语句。 - 在块内编写条件编译代码。
- 定义宏和
- 示例代码:
`define DEBUG module debug_example ( input wire a, input wire b, output wire y ); assign y = a & b; `ifdef DEBUG initial begin $display("Debug: a = %b, b = %b, y = %b", a, b, y); end `endif endmodule
- 方法:使用
在 Verilog 编程中,除了基础的模块化设计、参数化模块、always
块等,还有一些进阶技巧可以帮助你更高效地进行数字电路设计。以下是一些进阶技巧及具体操作:
8. 多模块实例化
在大型设计中,将多个子模块实例化可以提高代码的可读性和模块的可复用性。
- 实例化多个模块
- 方法:在顶层模块中实例化多个子模块。
- 具体步骤:
- 定义各个子模块。
- 在顶层模块中实例化这些子模块。
- 示例代码:
module submodule1 ( input wire a, output wire y ); assign y = ~a; endmodule module submodule2 ( input wire b, output wire z ); assign z = b & 1'b1; endmodule module top ( input wire a, input wire b, output wire y, output wire z ); submodule1 u1 ( .a(a), .y(y) ); submodule2 u2 ( .b(b), .z(z) ); endmodule
9. 状态机设计
状态机是数字电路设计中常用的控制逻辑设计方法。
- 使用状态机设计
- 方法:定义状态变量,使用
always
块描述状态转移和输出逻辑。 - 具体步骤:
- 定义状态编码。
- 定义状态变量和下一个状态变量。
- 在
always
块中描述状态转移逻辑。 - 在另一个
always
块中描述输出逻辑。
- 示例代码:
module fsm ( input wire clk, input wire reset, input wire in, output reg out ); typedef enum reg [1:0] { S0 = 2'b00, S1 = 2'b01, S2 = 2'b10 } state_t; state_t current_state, next_state; // State transition always @(posedge clk or posedge reset) begin if (reset) current_state <= S0; else current_state <= next_state; end // Next state logic always @(*) begin case (current_state) S0: if (in) next_state = S1; else next_state = S0; S1: if (in) next_state = S2; else next_state = S0; S2: if (in) next_state = S0; else next_state = S1; default: next_state = S0; endcase end // Output logic always @(*) begin case (current_state) S0: out = 1'b0; S1: out = 1'b1; S2: out = 1'b0; default: out = 1'b0; endcase end endmodule
- 方法:定义状态变量,使用
10. 使用 task
和 function
task
和 function
可以提高代码的重用性和可读性,适用于重复逻辑和复杂计算。
-
定义和使用
task
- 方法:使用
task
关键字定义一个任务。 - 具体步骤:
- 定义
task
,编写任务逻辑。 - 在
always
块或初始块中调用task
。
- 定义
- 示例代码:
module task_example; task automatic my_task; input [3:0] a, b; output [3:0] result; begin result = a + b; end endtask reg [3:0] x, y, z; initial begin x = 4'b0011; y = 4'b0101; my_task(x, y, z); $display("Result: %b", z); end endmodule
- 方法:使用
-
定义和使用
function
- 方法:使用
function
关键字定义一个函数。 - 具体步骤:
- 定义
function
,编写函数逻辑。 - 在
always
块或初始块中调用function
。
- 定义
- 示例代码:
module function_example; function [3:0] add; input [3:0] a, b; begin add = a + b; end endfunction reg [3:0] x, y, result; initial begin x = 4'b0011; y = 4'b0101; result = add(x, y); $display("Result: %b", result); end endmodule
- 方法:使用
11. 使用 assert
进行验证
assert
语句用于在仿真时验证设计的正确性。
- 定义
assert
语句- 方法:使用系统任务
assert
进行断言。 - 具体步骤:
- 在需要验证的地方插入
assert
语句。 - 定义条件和错误处理。
- 在需要验证的地方插入
- 示例代码:
module assert_example; reg [3:0] a, b, sum; initial begin a = 4'b0011; b = 4'b0101; sum = a + b; assert (sum == 4'b1000) else $fatal("Sum is incorrect: %b", sum); end endmodule
- 方法:使用系统任务
12. 使用 generate
语句
generate
语句用于条件生成或循环生成硬件结构。
-
条件生成
- 方法:使用
if-generate
语句。 - 具体步骤:
- 定义条件生成语句。
- 编写条件生成的硬件逻辑。
- 示例代码:
module generate_if_example ( input wire enable, output wire [3:0] y ); generate if (enable) begin : gen_block assign y = 4'b1111; end else begin assign y = 4'b0000; end endgenerate endmodule
- 方法:使用
-
循环生成
- 方法:使用
for-generate
语句。 - 具体步骤:
- 定义循环生成语句。
- 编写循环生成的硬件逻辑。
- 示例代码:
module generate_for_example ( input wire [7:0] a, output wire [7:0] b ); genvar i; generate for (i = 0; i < 8; i = i + 1) begin : gen_block assign b[i] = a[i]; end endgenerate endmodule
- 方法:使用
13. 使用多维数组
多维数组用于存储和处理多维数据。
- 定义和使用多维数组
- 方法:定义多维数组并进行操作。
- 具体步骤:
- 定义多维数组。
- 对多维数组进行赋值和操作。
- 示例代码:
module multidim_array_example; reg [7:0] memory [0:15][0:15]; integer i, j; initial begin // Initialize the memory for (i = 0; i < 16; i = i + 1) begin for (j = 0; j < 16; j = j + 1) begin memory[i][j] = i * j; end end // Display the memory content for (i = 0; i < 16; i = i + 1) begin for (j = 0; j < 16; j = j + 1) begin $display("memory[%0d][%0d] = %0d", i, j, memory[i][j]); end end end endmodule
14. 使用 system
任务
系统任务用于仿真控制和调试,如 $display
、$monitor
、$stop
等。
- 常用系统任务
- 方法:在代码中插入系统任务。
- 具体步骤:
- 使用
$display
打印信息。 - 使用
$monitor
监控信号变化。 - 使用
$stop
或$finish
终止仿真。
- 使用
- 示例代码:
module system_task_example; reg [3:0] a, b, sum; initial begin a = 4'b0011; b = 4'b0101; sum = a + b; $display("Time: %0t | a = %b, b = %b, sum = %b", $time, a, b, sum); $monitor("Time: %0t | a = %b, b = %b, sum = %b", $time, a, b, sum); #10 $stop; end endmodule
这些进阶技巧和具体操作可以帮助你在 Verilog 编程中更高效地进行复杂数字电路设计和验证。如果需要进一步的详细说明或有任何疑问,欢迎随时联系我。
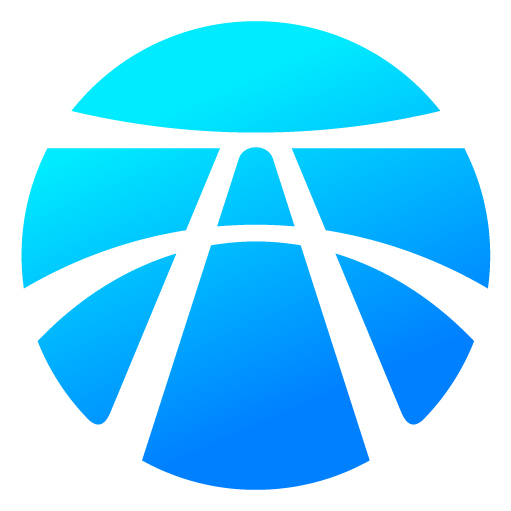
开放原子开发者工作坊旨在鼓励更多人参与开源活动,与志同道合的开发者们相互交流开发经验、分享开发心得、获取前沿技术趋势。工作坊有多种形式的开发者活动,如meetup、训练营等,主打技术交流,干货满满,真诚地邀请各位开发者共同参与!
更多推荐
所有评论(0)