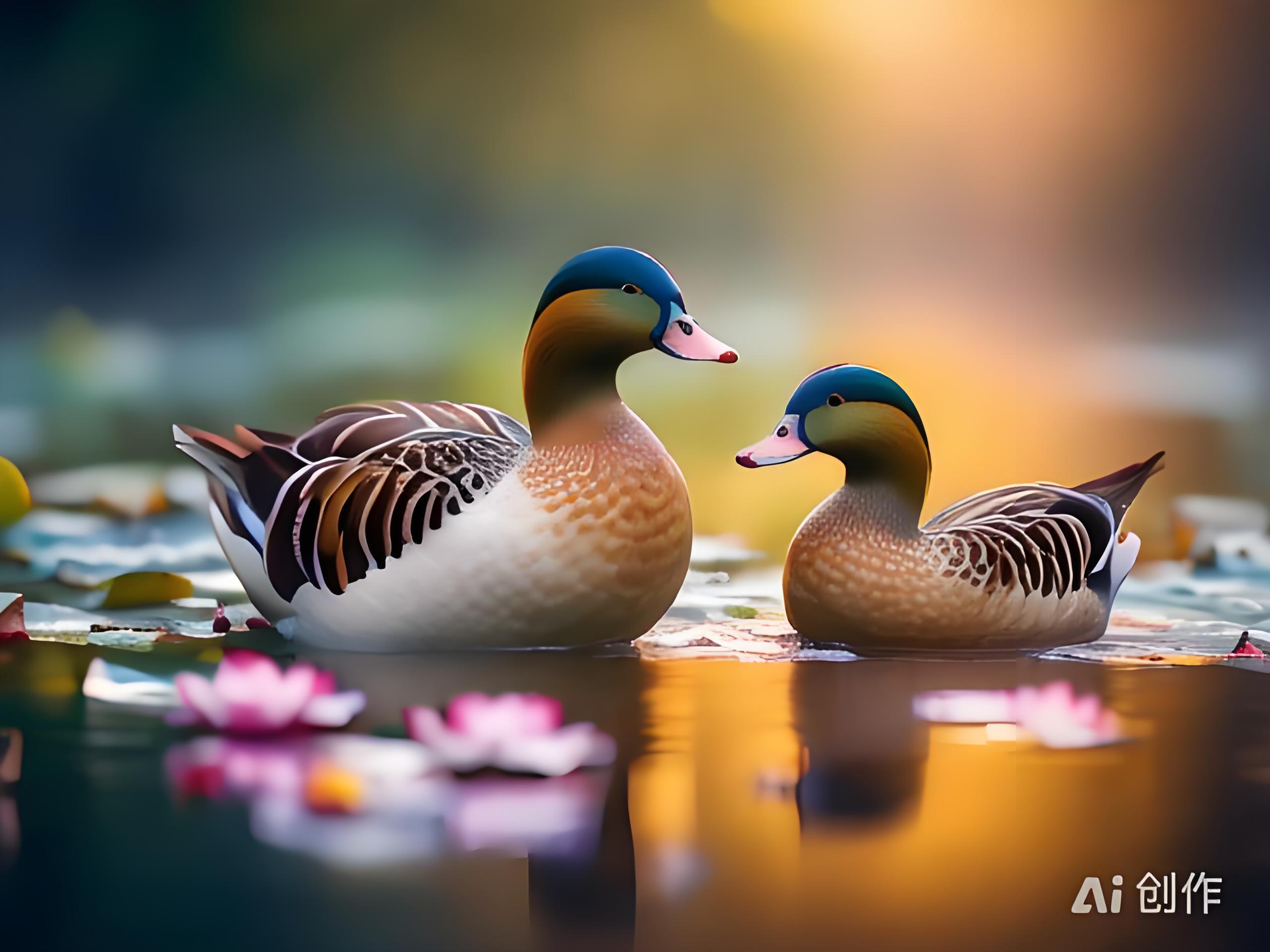
Spring Boot 集成Spring Cloud Gateway:实现API网关
Spring Cloud Gateway是Spring Cloud提供的一个API网关解决方案,基于Spring 5、Spring Boot 2和Project Reactor构建。它支持路由、过滤器、断路器、限流等功能,是替代Zuul的现代化网关解决方案。
Spring Boot 集成Spring Cloud Gateway:实现API网关
引言
在微服务架构中,API网关是一个非常重要的组件。它作为客户端请求的入口,提供路由、负载均衡、限流、认证和监控等功能。Spring Cloud Gateway是Spring生态系统中的一个API网关解决方案,它为微服务架构提供了强大的网关功能。本文将介绍如何在Spring Boot中集成Spring Cloud Gateway,实现API网关功能。
什么是Spring Cloud Gateway
Spring Cloud Gateway是Spring Cloud提供的一个API网关解决方案,基于Spring 5、Spring Boot 2和Project Reactor构建。它支持路由、过滤器、断路器、限流等功能,是替代Zuul的现代化网关解决方案。
添加依赖
在Spring Boot项目中添加Spring Cloud Gateway的依赖。在pom.xml
文件中添加以下依赖项:
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-gateway</artifactId>
</dependency>
配置Spring Cloud Gateway
在application.yml
文件中配置Spring Cloud Gateway:
spring:
cloud:
gateway:
routes:
- id: user-service
uri: http://localhost:8081
predicates:
- Path=/users/**
filters:
- StripPrefix=1
- id: order-service
uri: http://localhost:8082
predicates:
- Path=/orders/**
filters:
- StripPrefix=1
创建用户服务
创建一个简单的用户服务,作为API网关的路由目标。在Spring Initializr中生成一个新的Spring Boot项目,添加Spring Web依赖。创建一个名为UserController.java
的控制器类:
package com.example.userservice;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class UserController {
@GetMapping("/users/{id}")
public String getUser(@PathVariable String id) {
return "User: " + id;
}
}
在application.yml
文件中配置服务端口:
server:
port: 8081
创建订单服务
创建一个简单的订单服务,作为API网关的路由目标。在Spring Initializr中生成一个新的Spring Boot项目,添加Spring Web依赖。创建一个名为OrderController.java
的控制器类:
package com.example.orderservice;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class OrderController {
@GetMapping("/orders/{id}")
public String getOrder(@PathVariable String id) {
return "Order: " + id;
}
}
在application.yml
文件中配置服务端口:
server:
port: 8082
运行和测试
- 启动用户服务和订单服务。
- 启动Spring Cloud Gateway。
打开浏览器访问以下URL测试路由功能:
- 用户服务:
http://localhost:8080/users/1
,你将会看到响应User: 1
。 - 订单服务:
http://localhost:8080/orders/1
,你将会看到响应Order: 1
。
配置限流
为了保护后端服务,Spring Cloud Gateway支持基于令牌桶算法的限流。我们可以在配置文件中添加限流过滤器:
spring:
cloud:
gateway:
routes:
- id: user-service
uri: http://localhost:8081
predicates:
- Path=/users/**
filters:
- StripPrefix=1
- name: RequestRateLimiter
args:
redis-rate-limiter.replenishRate: 10
redis-rate-limiter.burstCapacity: 20
配置断路器
为了提高系统的稳定性,Spring Cloud Gateway还支持断路器模式。我们可以在配置文件中添加断路器过滤器:
spring:
cloud:
gateway:
routes:
- id: user-service
uri: http://localhost:8081
predicates:
- Path=/users/**
filters:
- StripPrefix=1
- name: CircuitBreaker
args:
name: userServiceCircuitBreaker
fallbackUri: forward:/fallback
创建Fallback控制器
为了处理断路器的回退逻辑,我们需要创建一个Fallback控制器:
package com.example.gateway;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class FallbackController {
@GetMapping("/fallback")
public String fallback() {
return "Service is unavailable. Please try again later.";
}
}
结论
通过本文的学习,你已经掌握了如何在Spring Boot中集成Spring Cloud Gateway,实现API网关功能。Spring Cloud Gateway作为一个强大的网关解决方案,能够帮助你构建高性能、可扩展的微服务架构。在下一篇文章中,我们将继续探索Spring Boot的更多高级特性,帮助你进一步提升开发技能。
这篇文章是我们Spring系列的第十九篇,旨在帮助你掌握Spring Boot与Spring Cloud Gateway的集成使用。如果你喜欢这篇文章,请关注我的CSDN博客,后续将有更多Spring相关的深入讲解和实战案例,带你一步步成为Spring专家!
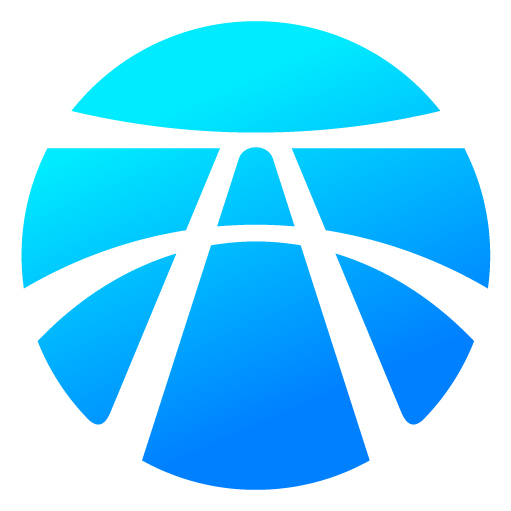
开放原子开发者工作坊旨在鼓励更多人参与开源活动,与志同道合的开发者们相互交流开发经验、分享开发心得、获取前沿技术趋势。工作坊有多种形式的开发者活动,如meetup、训练营等,主打技术交流,干货满满,真诚地邀请各位开发者共同参与!
更多推荐
所有评论(0)