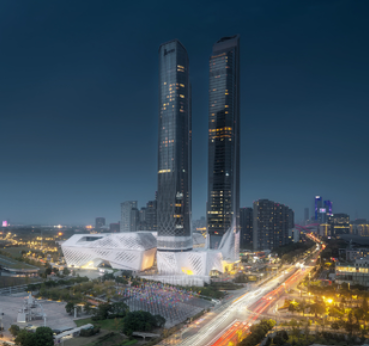
Android开发 GradientDrawable详解
Android开发 GradientDrawable详解
前言
GradientDrawable 支持渐变色的Drawable,与shapeDrawable在画型上是类似的,多了支持渐变色。代码上的GradientDrawable比在xml里的shape下gradient属性强大的多,因为shape下gradient属性只支持三色阶渐变,而GradientDrawable可以有更多的色阶渐变。
GradientDrawable在Android中便是shape标签的代码实现,利用GradientDrawable也可以创建出各种形状。
GradientDrawable 支持渐变色的Drawable,与shapeDrawable在画型上是类似的,多了支持渐变色。代码上的GradientDrawable比在xml里的shape下gradient属性强大的多,因为shape下gradient属性只支持三色阶渐变,而GradientDrawable可以有更多的色阶渐变。
GradientDrawable使用方法
1. 获取控件的shape并进行动态修改:
既然GradientDrawable是shape的动态实现,那么他就可以通过动态的获取控件的shape获取实例并进行修改,
比如我的上一篇文章
android 动态生成shape以及动态的改变shape颜色
2. 通过代码动态创建:
//什么都不指定默认为矩形
GradientDrawable background = new GradientDrawable();
background.setColor(Color.GREEN);
view.setBackgroundDrawable(background);
如果想要设置形状的话可以通过setShape(int shape) 方法来进行设置,这里一共可以设置四种形状:
GradientDrawable.RECTANGLE:矩形
GradientDrawable.OVAL:椭圆形
GradientDrawable.LINE:一条线
GradientDrawable.RING:环形(环形试了好久不知为何画不出来)
这里用GradientDrawable.OVAL来实验一下:
GradientDrawable background = new GradientDrawable();
background.setColor(Color.GREEN);
background.setShape(GradientDrawable.OVAL);
view.setBackgroundDrawable(background);
如果想让效果更加丰富一些添加描边或者颜色渐变:
GradientDrawable background = new GradientDrawable();
background.setShape(GradientDrawable.OVAL);
background.setStroke(10,Color.RED);//设置宽度为10px的红色描边
background.setGradientType(GradientDrawable.LINEAR\_GRADIENT);//设置线性渐变,除此之外还有:GradientDrawable.SWEEP\_GRADIENT(扫描式渐变),GradientDrawable.RADIAL\_GRADIENT(圆形渐变)
background.setColors(new int\[\]{Color.RED,Color.BLUE});//增加渐变效果需要使用setColors方法来设置颜色(中间可以增加多个颜色值)
view.setBackgroundDrawable(background);
画线
GradientDrawable gradientDrawable = new GradientDrawable();
gradientDrawable.setShape(GradientDrawable.LINE);
gradientDrawable.setStroke(5, Color.YELLOW);//线的宽度 与 线的颜色
mTextView.setBackground(gradientDrawable);
效果图:
画虚线
mTextView.setLayerType(View.LAYER\_TYPE\_SOFTWARE,null); //要显示虚线一定要关闭硬件加速
GradientDrawable gradientDrawable = new GradientDrawable();
gradientDrawable.setShape(GradientDrawable.LINE);
gradientDrawable.setStroke(1, Color.BLACK, 10, 10);//第一个参数为线的宽度 第二个参数是线的颜色 第三个参数是虚线段的长度 第四个参数是虚线段之间的间距长度
mTextView.setBackground(gradientDrawable);
也可以在布局里关闭指定view的硬件加速
android:layerType="software"
效果图:
画圆
GradientDrawable gradientDrawable = new GradientDrawable();
gradientDrawable.setShape(GradientDrawable.OVAL);
gradientDrawable.setColor(Color.BLUE);
gradientDrawable.setSize(50,50);
mTextView.setBackground(gradientDrawable);
效果图:
画圆环
GradientDrawable gradientDrawable = new GradientDrawable();
gradientDrawable.setShape(GradientDrawable.OVAL);
gradientDrawable.setColor(Color.BLUE);
gradientDrawable.setStroke(10,Color.YELLOW);
gradientDrawable.setSize(50,50);
mTextView.setBackground(gradientDrawable);
效果图:
圆角矩形
GradientDrawable gradientDrawable = new GradientDrawable();
gradientDrawable.setShape(GradientDrawable.RECTANGLE);
gradientDrawable.setColor(Color.RED);
gradientDrawable.setStroke(10,Color.BLUE);
gradientDrawable.setCornerRadius(10);
gradientDrawable.setSize(50,50);
mTextView.setBackground(gradientDrawable);
效果图:
虚线矩形
GradientDrawable gradientDrawable = new GradientDrawable();
gradientDrawable.setShape(GradientDrawable.LINEAR\_GRADIENT);
gradientDrawable.setStroke(1, Color.GREEN,30, 30);
mTextView.setBackground(gradientDrawable);
效果图:
颜色渐变
线性渐变
int\[\] colors = {Color.YELLOW, Color.GREEN, Color.BLUE};
GradientDrawable gradientDrawable = new GradientDrawable();
gradientDrawable.setShape(GradientDrawable.RECTANGLE);
gradientDrawable.setColors(colors); //添加颜色组
gradientDrawable.setGradientType(GradientDrawable.LINEAR\_GRADIENT);//设置线性渐变
gradientDrawable.setSize(50,50);
mTextView.setBackground(gradientDrawable);
效果图:
改变线性渐变方向
int\[\] colors = {Color.YELLOW, Color.GREEN, Color.BLUE};
GradientDrawable gradientDrawable = new GradientDrawable();
gradientDrawable.setShape(GradientDrawable.RECTANGLE);
gradientDrawable.setColors(colors); //添加颜色组
gradientDrawable.setGradientType(GradientDrawable.LINEAR\_GRADIENT);//设置线性渐变
gradientDrawable.setOrientation(GradientDrawable.Orientation.RIGHT\_LEFT);//设置渐变方向
gradientDrawable.setSize(50,50);
mTextView.setBackground(gradientDrawable);
效果图:
半径渐变
int\[\] colors = {Color.YELLOW, Color.GREEN, Color.BLUE};
GradientDrawable gradientDrawable = new GradientDrawable();
gradientDrawable.setShape(GradientDrawable.RECTANGLE);
gradientDrawable.setColors(colors); //添加颜色组
gradientDrawable.setGradientType(GradientDrawable.RADIAL\_GRADIENT);//设置半径渐变
gradientDrawable.setGradientRadius(50);//渐变的半径值
gradientDrawable.setSize(50,50);
mTextView.setBackground(gradientDrawable);
效果图:
扫描渐变
int\[\] colors = {Color.YELLOW, Color.GREEN, Color.BLUE};
GradientDrawable gradientDrawable = new GradientDrawable();
gradientDrawable.setShape(GradientDrawable.RECTANGLE);
gradientDrawable.setColors(colors); //添加颜色组
gradientDrawable.setGradientType(GradientDrawable.SWEEP\_GRADIENT);//设置扫描渐变
gradientDrawable.setGradientCenter(0.5f,0.5f);//渐变中心点
gradientDrawable.setSize(50,50);
mTextView.setBackground(gradientDrawable);
防抖
gradientDrawable.setDither(true);
可以让渐变的时候颜色阶梯降低,变得更柔和
透明度
GradientDrawable gradientDrawable = new GradientDrawable();
gradientDrawable.setShape(GradientDrawable.RECTANGLE);
gradientDrawable.setColor(Color.YELLOW);
gradientDrawable.setAlpha(70);//设置透明度
mTextView.setBackground(gradientDrawable);
最后
Android开发 GradientDrawable详解到这了,需要更多Android开发的学习资料可以扫码免费领取!《架构师筑基必备技能》、《Android百大框架源码解析》、《Android性能优化实战解析》、《高级kotlin强化实战》、《Android高级UI开源框架进阶解密》、《NDK模块开发》、《Flutter技术进阶》、《微信小程序开发》。附全套视频资料,包含面试合集、源码合集、开源框架合集。
完整文档资料扫码领取
目录
一、架构师筑基必备技能
1.深入理解Java泛型 2.注解深入浅出 3.并发编程 4.数据传输与序列化 5.Java虚拟机原理 6.高效IO ……
二、Android百大框架源码解析
1.Retrofit 2.0源码解析 2.Okhttp3源码解析 3.ButterKnife源码解析 4.MPAndroidChart 源码解析 5.Glide源码解析 6.Leakcanary 源码解析 7.Universal-lmage-Loader源码解析 8.EventBus 3.0源码解析 9.zxing源码分析 10.Picasso源码解析 11.LottieAndroid使用详解及源码解析 12.Fresco 源码分析——图片加载流程
三、Android性能优化实战解析
1.腾讯Bugly:对字符串匹配算法的一点理解
2.爱奇艺:安卓APP崩溃捕获方案——xCrash
3.字节跳动:深入理解Gradle框架之一:Plugin, Extension, buildSrc
4.百度APP技术:Android H5首屏优化实践
5.支付宝客户端架构解析:Android 客户端启动速度优化之「垃圾回收」
6.携程:从智行 Android 项目看组件化架构实践
7.网易新闻构建优化:如何让你的构建速度“势如闪电”?
…
四、高级kotlin强化实战
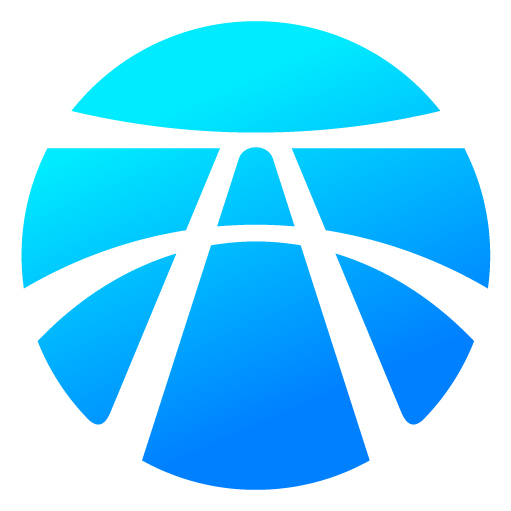
开放原子开发者工作坊旨在鼓励更多人参与开源活动,与志同道合的开发者们相互交流开发经验、分享开发心得、获取前沿技术趋势。工作坊有多种形式的开发者活动,如meetup、训练营等,主打技术交流,干货满满,真诚地邀请各位开发者共同参与!
更多推荐
所有评论(0)