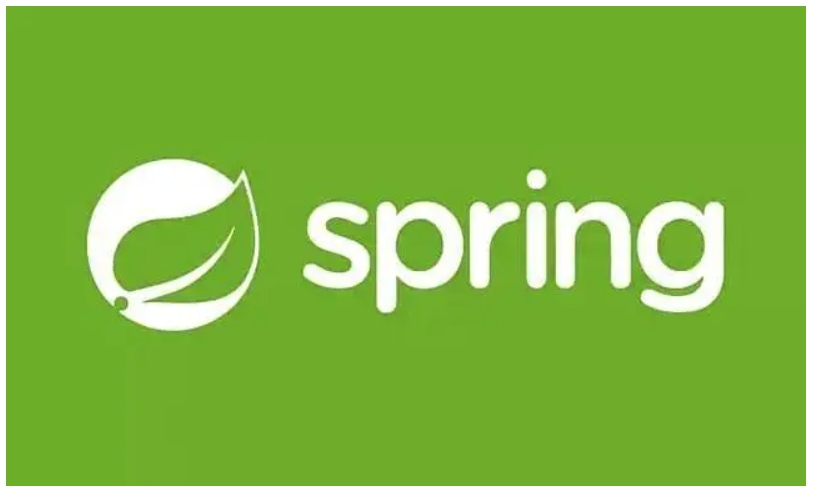
Spring:Spring WebFlux中使用WebClient远程接口调用
Spring WebFlux中使用WebClient远程接口调用
一、前言
WebClient是Spring 5中引入的一个新的非阻塞、响应式HTTP客户端,用于发送HTTP请求和接收响应。它基于Reactor和Netty,支持异步调用,并提供了简洁且易于使用的API。WebClient是Spring WebFlux模块的一部分,从Spring 5.0开始,它作为RestTemplate的替代品,具有更好的响应式能力和异步支持。
二、WebClient的主要特点
非阻塞:WebClient采用响应式编程模型,支持非阻塞IO操作,使得在高并发场景下性能更佳。
异步调用:支持异步发送HTTP请求,并通过回调函数处理响应结果,使得在等待响应时不会阻塞当前线程。
简洁易用:提供了简洁的API用于构建和发送HTTP请求,使得编写HTTP客户端代码更加容易。
灵活性:允许自定义请求的各个方面,包括请求头、请求体、URI变量等。
三、WebClient的常见方法
post():创建一个POST请求。
put():创建一个PUT请求。
get():创建一个GET请求。
delete():创建一个DELETE请求。
uri(String uri):设置请求的URI地址。
header(String headerName, String… headerValues):设置请求头。
headers(Consumer headersConsumer):使用Consumer对象来自定义请求头。
accept(MediaType… acceptableMediaTypes):设置期望的响应内容类型。
contentType(MediaType contentType):设置请求的内容类型。
body(BodyInserter<?, ? super ClientHttpRequest> bodyInserter):设置请求的内容体,使用BodyInserter对象。
retrieve():发送请求并获取响应。
四、WebClient方法代码示例
使用WebClient进行HTTP请求时,以下是GET请求(包括带参数的GET请求)和POST请求(发送JSON或表单数据)的代码示例。
4.1 GET 请求(不带参数的GET请求)
不带参数的GET请求
import org.springframework.web.reactive.function.client.WebClient;
public class WebClientExample {
public static void main(String[] args) {
WebClient webClient = WebClient.create("http://example.com");
webClient.get()
.uri("/api/resource")
.retrieve()
.bodyToMono(String.class)
.subscribe(
result -> System.out.println("Result: " + result),
error -> System.err.println("Error: " + error)
);
}
}
4.2 GET请求(带参数的使用URI模板变量)
import org.springframework.web.reactive.function.client.WebClient;
public class WebClientExample {
public static void main(String[] args) {
WebClient webClient = WebClient.create("http://example.com");
String id = "123";
webClient.get()
.uri("/api/resource/{id}", id) // 使用URI模板变量
.retrieve()
.bodyToMono(String.class)
.subscribe(
result -> System.out.println("Result: " + result),
error -> System.err.println("Error: " + error)
);
}
}
4.3 GET请求(带参数的使用URI查询参数)
import org.springframework.web.reactive.function.client.WebClient;
public class WebClientExample {
public static void main(String[] args) {
WebClient webClient = WebClient.create("http://example.com");
String id = "123";
webClient.get()
.uri(uriBuilder -> uriBuilder
.path("/api/resource")
.queryParam("id", id) // 添加查询参数
.build())
.retrieve()
.bodyToMono(String.class)
.subscribe(
result -> System.out.println("Result: " + result),
error -> System.err.println("Error: " + error)
);
}
}
4.4 POST 请求(发送JSON数据)
import org.springframework.http.MediaType;
import org.springframework.web.reactive.function.client.WebClient;
public class WebClientExample {
public static void main(String[] args) {
WebClient webClient = WebClient.create("http://example.com");
String jsonData = "{\"name\":\"John\", \"age\":30}";
webClient.post()
.uri("/api/resource")
.contentType(MediaType.APPLICATION_JSON)
.bodyValue(jsonData)
.retrieve()
.bodyToMono(String.class)
.subscribe(
result -> System.out.println("Result: " + result),
error -> System.err.println("Error: " + error)
);
}
}
4.5 POST 请求(发送表单数据,使用MultiValueMap)
import org.springframework.http.MediaType;
import org.springframework.util.LinkedMultiValueMap;
import org.springframework.util.MultiValueMap;
import org.springframework.web.reactive.function.client.WebClient;
public class WebClientExample {
public static void main(String[] args) {
WebClient webClient = WebClient.create("http://example.com");
MultiValueMap<String, String> formData = new LinkedMultiValueMap<>();
formData.add("name", "John");
formData.add("age", "30");
webClient.post()
.uri("/api/resource")
.contentType(MediaType.APPLICATION_FORM_URLENCODED)
.bodyValue(formData)
.retrieve()
.bodyToMono(String.class)
.subscribe(
result -> System.out.println("Result: " + result),
error -> System.err.println("Error: " + error)
);
}
}
请注意,以上代码示例中的subscribe方法用于触发请求
四、WebClient异步与同步
当使用WebClient时,通常推荐的做法是使用其异步API,因为它基于响应式编程模型,并且可以充分利用非阻塞IO来提高性能和吞吐量。但是,有时为了简化代码或满足特定需求,你可能想要以同步的方式使用WebClient。然而,请注意,将响应式编程转换为同步调用可能会牺牲性能和响应性。
异步代码示例
异步代码示例使用Mono或Flux类型来处理响应,并使用block()方法(尽管在生产代码中不推荐,因为它会阻塞当前线程)来等待结果。但通常,你会使用.subscribe()方法来异步处理响应。
import org.springframework.http.MediaType;
import org.springframework.web.reactive.function.client.WebClient;
import reactor.core.publisher.Mono;
public class WebClientAsyncExample {
public static void main(String[] args) {
WebClient webClient = WebClient.create("http://example.com");
Mono<String> responseMono = webClient.get()
.uri("/api/resource")
.accept(MediaType.APPLICATION_JSON)
.retrieve()
.bodyToMono(String.class);
// 使用subscribe异步处理响应
responseMono.subscribe(
result -> System.out.println("Result: " + result),
error -> System.err.println("Error: " + error)
);
// 注意:通常不推荐在main线程中调用block(),因为它会阻塞
// 以下代码仅用于演示目的
String result = responseMono.block(); // 阻塞当前线程直到结果可用
System.out.println("Blocked Result: " + result);
}
}
同步代码示例
由于WebClient本身并不直接支持同步调用,但你可以通过封装异步调用来模拟同步行为。这通常涉及到使用block()方法,但如前所述,这不应该在生产代码中使用,因为它会阻塞当前线程。
import org.springframework.http.MediaType;
import org.springframework.web.reactive.function.client.WebClient;
import reactor.core.publisher.Mono;
public class WebClientSyncExample {
public static void main(String[] args) {
WebClient webClient = WebClient.create("http://example.com");
// 使用block()方法阻塞当前线程以等待结果
String result = webClient.get()
.uri("/api/resource")
.accept(MediaType.APPLICATION_JSON)
.retrieve()
.bodyToMono(String.class)
.block(); // 注意:这会阻塞当前线程
System.out.println("Result: " + result);
}
}
在这个同步代码示例中,我们使用block()方法来等待异步操作完成并返回结果。但是,这会导致当前线程被阻塞,直到结果可用为止。这可能会降低应用程序的性能和响应性,因此应谨慎使用。在生产环境中,通常建议使用异步编程模型来处理HTTP请求。
以上是Spring 5.0 中引入的一个新的非阻塞、响应式HTTP客户端WebClient,用于发送HTTP请求和接收响应的一些介绍与示例。
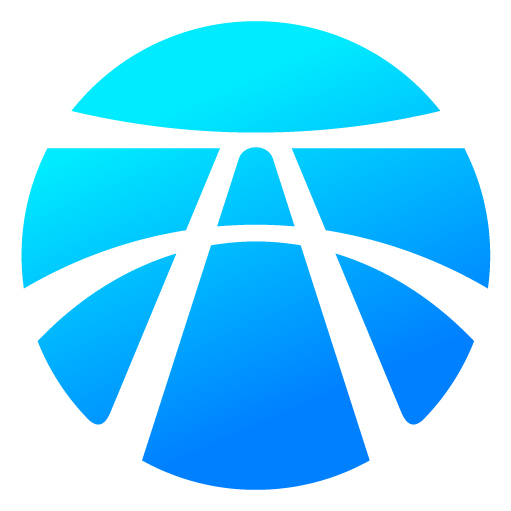
开放原子开发者工作坊旨在鼓励更多人参与开源活动,与志同道合的开发者们相互交流开发经验、分享开发心得、获取前沿技术趋势。工作坊有多种形式的开发者活动,如meetup、训练营等,主打技术交流,干货满满,真诚地邀请各位开发者共同参与!
更多推荐
所有评论(0)