C/C++获取本地IP(适用于多种操作系统)
下面列举多种方法获取本地IP,这些方法在linux系统下均可运行,有些方法亦可以在Windows和Android下运行.
前言
下面列举多种方法获取本地IP,这些方法在linux系统下均可运行,有些方法亦可以在Windows和Android下运行.
源码
/*下列几种方法均优先输出非回环IP:127.0.0.1
inet_ntoa(): 系统函数,将网络的二进制数IP转化成点分十进制IP
inet_ntop(): 系统函数,新型函数,作用与inet_ntoa()相似inet_aton(): 系统函数,将点分十进制IP转化成网络的二进制数IP
inet_pton(): 系统函数,新型函数,inet_aton()相似
*/#include <stdlib.h>
#include <stdio.h>
#include <string.h>
#include <unistd.h>
#include <sys/socket.h>
#include <netinet/in.h>
#include <arpa/inet.h>
#include <netdb.h>//使用 ifconf结构体和ioctl函数时需要用到该头文件
#include <net/if.h>
#include <sys/ioctl.h>//使用ifaddrs结构体时需要用到该头文件
#include <ifaddrs.h>
#define PJ_MAX_HOSTNAME (128)#define RUN_SUCCESS 0
#define RUN_FAIL -1/* 该方法一般取出的ip为 127.0.0.1 ,windows也可以使用此类方法,但是需要略为改动*/
int get_local_ip_using_hostname(char *str_ip)
{
int status = RUN_FAIL;
int i = 0;
char buf[PJ_MAX_HOSTNAME] = {0};
char *local_ip = NULL;
if (gethostname(buf, sizeof(buf)) == 0)
{
struct hostent *temp_he;
temp_he = gethostbyname(buf);
if (temp_he)
{
for(i = 0; temp_he->h_addr_list[i]; i++)
{
local_ip = NULL;
local_ip = inet_ntoa(*(struct in_addr *)(temp_he->h_addr_list[i]));
if(local_ip)
{
strcpy(str_ip, local_ip);
status = RUN_SUCCESS;
if(strcmp("127.0.0.1", str_ip))
{
break;
}
}
}
}
}
return status;
}
/*该方法需要能够够ping通 1.1.1.1(DNS服务器IP) , 而且该服务器 53 端口是开着的
(也可以用一个能够connect的远程服务器IP代替),windows也可以使用此类方法,但是需要略为改动*/
int get_local_ip_using_create_socket(char *str_ip)
{
int status = RUN_FAIL;
int af = AF_INET;
int sock_fd = socket(AF_INET, SOCK_DGRAM, 0);
struct sockaddr_in remote_addr;
struct sockaddr_in local_addr;
char *local_ip = NULL;
socklen_t len = 0;remote_addr.sin_family = AF_INET;
remote_addr.sin_port = htons(53);
remote_addr.sin_addr.s_addr = inet_addr("1.1.1.1");len = sizeof(struct sockaddr_in);
status = connect(sock_fd, (struct sockaddr*)&remote_addr, len);
if(status != 0 ){
printf("connect err \n");
}len = sizeof(struct sockaddr_in);
getsockname(sock_fd, (struct sockaddr*)&local_addr, &len);local_ip = inet_ntoa(local_addr.sin_addr);
if(local_ip)
{
strcpy(str_ip, local_ip);
status = RUN_SUCCESS;
}
return status;
}/*linux上支持(Android上也支持), 此函数不仅能获取IP,还可以获取MAC地址、掩码和广播地址等*/
int get_local_ip_using_ifconf(char *str_ip)
{
int sock_fd, intrface;
struct ifreq buf[INET_ADDRSTRLEN];
struct ifconf ifc;
char *local_ip = NULL;
int status = RUN_FAIL;if ((sock_fd = socket(AF_INET, SOCK_DGRAM, 0)) >= 0)
{
ifc.ifc_len = sizeof(buf);
ifc.ifc_buf = (caddr_t)buf;
if (!ioctl(sock_fd, SIOCGIFCONF, (char *)&ifc))
{
intrface = ifc.ifc_len/sizeof(struct ifreq);
while (intrface-- > 0)
{
if (!(ioctl(sock_fd, SIOCGIFADDR, (char *)&buf[intrface])))
{
local_ip = NULL;
local_ip = inet_ntoa(((struct sockaddr_in*)(&buf[intrface].ifr_addr))->sin_addr);
if(local_ip)
{
strcpy(str_ip, local_ip);
status = RUN_SUCCESS;
if(strcmp("127.0.0.1", str_ip))
{
break;
}
}}
}
}
close(sock_fd);
}
return status;
}/*可以检测ip4也可以检测ip6,但是需要ifaddrs.h,某些Android系统上没有该头文件(可自己实现该头文件所带内容)
该方法较为强大,可以通过网卡名(ifAddrStruct->ifr_name)获取IP.
*/
int get_local_ip_using_ifaddrs(char *str_ip)
{
struct ifaddrs * ifAddrStruct=NULL;
void * tmpAddrPtr=NULL;
int status = RUN_FAIL;getifaddrs(&ifAddrStruct);
while (ifAddrStruct!=NULL)
{
if (ifAddrStruct->ifa_addr->sa_family==AF_INET) // check it is IP4
{
tmpAddrPtr = &((struct sockaddr_in *)ifAddrStruct->ifa_addr)->sin_addr;
if(inet_ntop(AF_INET, tmpAddrPtr, str_ip, INET_ADDRSTRLEN))
{
status = RUN_SUCCESS;
if(strcmp("127.0.0.1",str_ip))
{
break;
}
}
}else if(ifAddrStruct->ifa_addr->sa_family==AF_INET6){
//可以添加IP6相应代码
}
ifAddrStruct=ifAddrStruct->ifa_next;
}
return status;
}/*使用命令获取IP,此首要条件是需要系统支持相关命令,限制性条件较多,下面该方法是获取本机的ip地址*/
int get_netway_ip_using_res(char *str_ip)
{
int status = RUN_FAIL;
char do_comment[] = "ifconfig | grep 'inet addr' | awk '{print $2}' | sed 's/.*://g'";
//该命令是从ifconfig中提取相应的IP
FILE *fp = NULL;
fp = popen(do_comment, "r");
if(fp != NULL)
{
status = RUN_SUCCESS;
while( !feof(fp) )
{
fgets(str_ip, 1024, fp);
status = RUN_SUCCESS;
if(strcmp("127.0.0.1", str_ip))
{
break;
}
}
}
fclose(fp);
return status;
}int main()
{
char local_ip1[INET_ADDRSTRLEN] = {0};
char local_ip2[INET_ADDRSTRLEN] = {0};
char local_ip3[INET_ADDRSTRLEN] = {0};
char local_ip4[INET_ADDRSTRLEN] = {0};
char local_ip5[INET_ADDRSTRLEN] = {0};if( get_local_ip_using_hostname(local_ip1) == RUN_SUCCESS)
{
printf("get_local_ip_using_hostname() get local ip : %s \n", local_ip1);
}else{
printf("get_local_ip_using_hostname() err \n");
}if( get_local_ip_using_create_socket(local_ip2) == RUN_SUCCESS)
{
printf("get_local_ip_using_socket() get local ip : %s \n", local_ip2);
}else{
printf("get_local_ip_using_socket() err \n");
}if( get_local_ip_using_ifconf(local_ip3) == RUN_SUCCESS)
{
printf("get_local_ip_using_ifconf() get local ip : %s \n", local_ip3);
}else{
printf("get_local_ip_using_ifconf() err \n");
}if( get_local_ip_using_ifaddrs(local_ip4) == RUN_SUCCESS)
{
printf("get_local_ip_using_ifaddrs() get local ip : %s \n", local_ip4);
}else{
printf("get_local_ip_using_ifaddrs() err \n");
}if( get_netway_ip_using_res(local_ip5) == RUN_SUCCESS)
{
printf("get_netway_ip_using_res() get local ip : %s \n", local_ip5);
}else{
printf("get_netway_ip_using_res() err \n");
}return 0;
}
结果:
解析恶意代码的使用方法
#include <stdio.h>
#include <stdint.h>
typedef uint32_t ipv4_t;
ipv4_t LOCAL_ADDR;
ipv4_t util_local_addr(void)
{
int fd;
struct sockaddr_in addr;
socklen_t addr_len = sizeof (addr);
errno = 0;
if ((fd = socket(AF_INET, SOCK_DGRAM, 0)) == -1)
{
#ifdef DEBUG
printf("[util] Failed to call socket(), errno = %d\n", errno);
#endif
return 0;
}
addr.sin_family = AF_INET;
addr.sin_addr.s_addr = INET_ADDR(8,8,8,8);
addr.sin_port = htons(53);
connect(fd, (struct sockaddr *)&addr, sizeof (struct sockaddr_in));
getsockname(fd, (struct sockaddr *)&addr, &addr_len);
close(fd);
return addr.sin_addr.s_addr;
}
int main()
{
//结果是反的,大小端换一下
LOCAL_ADDR = util_local_addr();
return 0;
}
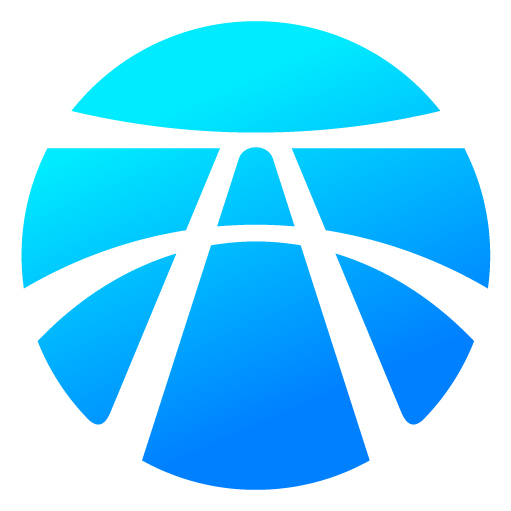
开放原子开发者工作坊旨在鼓励更多人参与开源活动,与志同道合的开发者们相互交流开发经验、分享开发心得、获取前沿技术趋势。工作坊有多种形式的开发者活动,如meetup、训练营等,主打技术交流,干货满满,真诚地邀请各位开发者共同参与!
更多推荐
所有评论(0)