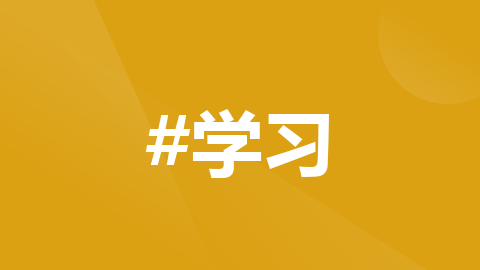
学习Math.random()的应用
在 JavaScript 中,Math是一个内置对象,提供了许多用于数学计算的函数和常量。它不需要实例化,因为所有的属性和方法都是静态的。 toString()方法用法。padStart()方法的用法。时间戳格式化。
在 JavaScript 中,Math是一个内置对象,提供了许多用于数学计算的函数和常量。它不需要实例化,因为所有的属性和方法都是静态的。比如下面这些类型的方法:
基本数学函数
Math.abs(x) | 返回 x 的绝对值。
|
Math.ceil(x) | 向上取整,返回大于或等于 x 的最小整数。Math.ceil(4.2); // 5 |
Math.floor(x) | 向下取整,返回小于或等于 x 的最大整数。Math.floor(4.7); // 4 |
Math.round(x) | 四舍五入,返回最接近 x 的整数。
|
Math.trunc(x) | 去掉小数部分,只保留整数部分。
|
指数和对数函数
Math.exp(x) | 返回 e 的 x 次方。
|
Math.log(x) | 返回 x 的自然对数 (以 e 为底)。
|
Math.log10(x) | 返回 x 的以 10 为底的对数。Math.log10(100); // 2 |
Math.log2(x) | 返回 x 的以 2 为底的对数。
|
Math.pow(base, exponent) | 返回 base 的 exponent 次方
|
Math.sqrt(x) | 返回 x 的平方根。
|
三角函数
Math.sin(x) | 返回 x 弧度的正弦值。Math.sin(Math.PI / 2); // 1 |
Math.cos(x) | 返回 x 弧度的余弦值。Math.cos(Math.PI); // -1 |
Math.tan(x) | 返回 x 弧度的正切值。Math.tan(Math.PI / 4); // 1 |
其他函数
Math.max(...values) | 返回一组值中的最大值。
|
Math.min(...values) | 返回一组值中的最小值。
|
Math.random() | 返回一个介于 0(包含)和 1(不包含)之间的随机数。 |
Math.sign(x) | 返回 x 的符号,-1 表示负数,1 表示正数,0 表示零。
|
Math.radmon() 学习
JavaScript 的内置函数,属于 Math
对象,用于生成伪随机数。
基本用法
Math.random()返回一个介于 0(包含)和 1(不包含)之间的伪随机浮点数。每次调用 Math.random()时,它会生成一个新的随机值。
console.log(Math.random()); // 例如 0.345678123456789
应用场景
生成指定范围的随机整数
虽然 Math.random()只生成 0 到 1 之间的浮点数,但可以通过一些数学运算将其转换为其他范围的随机数。
-
生成 0 到
max
之间的随机整数
要生成一个范围在 0
到 max
(包含)之间的随机整数,可以使用以下方法:
const max = 10;
const randomInt = Math.floor(Math.random() * (max + 1));
console.log(randomInt); // 例如 7
这里,Math.random() * (max + 1)
生成一个介于 0 和 max + 1
之间的浮点数,Math.floor()
将其向下取整,得到一个从 0 到 max
(包含)的整数。
-
生成指定范围的随机整数
要生成一个范围在min 包含)到 max (包含)之间的随机整数,可以使用:
const min = 5;
const max = 15;
const randomIntInRange = Math.floor(Math.random() * (max - min + 1)) + min;
console.log(randomIntInRange); // 例如 12
这里,Math.random() * (max - min + 1)
生成一个介于 0 和max -
min + 1
之间的浮点数,加上 min之后,Math.floor()
使其成为范围在min到 max 之间的整数。
随机选择数组元素
const items = ['apple', 'banana', 'cherry'];
const randomIndex = Math.floor(Math.random() * items.length);
const randomItem = items[randomIndex];
console.log(randomItem); // 例如 'banana'
生成随机颜色
- 生成随机的 RGB 颜色
function getRandomColor() {
const r = Math.floor(Math.random() * 256);
const g = Math.floor(Math.random() * 256);
const b = Math.floor(Math.random() * 256);
return `rgb(${r},${g},${b})`;
}
console.log(getRandomColor()); // 例如 'rgb(123,45,67)'
- 生成随机的十六进制颜色
function getRandomHexColor() {
const color = Math.floor(Math.random() * 0xFFFFFF).toString(16);
return `#${color.padStart(6, '0')}`;
}
console.log(getRandomHexColor()); // 例如 '#a3c2f5'
或者
const randomColor = '#' + Math.floor(Math.random() * 16777215).toString(16);
console.log(randomColor); // 例如 '#a3c2f5'
生成随机日期
function getRandomDate(start, end) {
return new Date(start.getTime() + Math.random() * (end.getTime() - start.getTime()));
}
const startDate = new Date(2020, 0, 1);
const endDate = new Date(2024, 0, 1);
const randomDate = getRandomDate(startDate, endDate);
console.log(randomDate); // 例如 Fri Nov 10 2023 15:43:55 GMT+0000 (Coordinated Universal Time)
生成随机布尔值
如果你需要生成随机的布尔值(true
或 false
),可以这样做:
const randomBoolean = Math.random() < 0.5;
console.log(randomBoolean); // true 或 false
模拟掷骰子
生成 1 到 6 的随机整数,模拟掷骰子:
const diceRoll = Math.floor(Math.random() * 6) + 1;
console.log(diceRoll); // 1 到 6 之间的整数
生成随机字符或字符串
- 生成一个随机字符(例如,字母或数字)
const characters = 'ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789';
const randomChar = characters.charAt(Math.floor(Math.random() * characters.length));
console.log(randomChar); // 例如 'A'
- 生成指定长度的随机字符串
function generateRandomString(length) {
const characters = 'ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789';
let result = ''; for (let i = 0; i < length; i++) {
result += characters.charAt(Math.floor(Math.random() * characters.length));
}
return result;
}
const randomString = generateRandomString(10);
console.log(randomString); // 例如 'a1B2c3D4e5'
随机打乱数组
将数组中的元素随机打乱(类似洗牌):
const items = ['apple', 'banana', 'cherry', 'date'];
const randomItem = items[Math.floor(Math.random() * items.length)];
console.log(randomItem); // 例如 'cherry'
生成随机坐标
生成指定范围内的随机经纬度坐标:
function getRandomCoordinates(latRange, lonRange) {
const latitude = latRange[0] + Math.random() * (latRange[1] - latRange[0]);
const longitude = lonRange[0] + Math.random() * (lonRange[1] - lonRange[0]);
return { latitude, longitude };
}
const latRange = [34.0, 37.0]; // 纬度范围
const lonRange = [-118.5, -117.5]; // 经度范围
const randomCoordinates = getRandomCoordinates(latRange, lonRange);
console.log(randomCoordinates); // 例如 { latitude: 35.6789, longitude: -118.2345 }
模拟随机事件
在模拟事件(例如,用户点击、天气变化)时,随机事件的概率可以这样计算:
const eventOccurs = Math.random() < 0.1; // 10% 的概率发生事件
console.log(eventOccurs); // true 或 false
拓展:一些例子中的方法解析
Math.floor()和Math.trunc()有什么区别?
Math.floor(x)
:返回小于或等于 x
的最大整数,即向下舍入到最接近的整数。它总是将数字向负无穷方向舍入。
Math.floor(4.7); // 4
Math.floor(-4.7); // -5
Math.trunc(x)
:返回数字 x
的整数部分,即去掉数字的小数部分。它不会考虑数字的符号,只是简单地去掉小数部分。
Math.trunc(4.9); // 4
Math.trunc(-4.9); // -4
toString()方法用法?
toString()
是 JavaScript 中的一个方法,用于将对象转换为字符串。这个方法在各种数据类型和对象中都可以使用,但具体的行为取决于对象的类型。但值得注意的是,toString()
方法可以接受一个可选的参数 radix
,这是一个整数值,指定了用于表示数字的基数(进制)。这个参数通常用于数字类型,但在字符串和其他对象的 toString()
方法中通常不使用。
toString(radix)
详解
toString(radix)
方法用于将数字对象转换为字符串,并使用指定的基数(进制)来表示该数字。基数的值可以是 2 到 36 之间的整数,表示不同的进制系统:
- 基数 2:二进制(例如,
1010
) - 基数 8:八进制(例如,
12
) - 基数 10:十进制(例如,
10
) - 基数 16:十六进制(例如,
A
)
十进制转十六进制
const num = 255;
console.log(num.toString(16)); // "ff"
使用不合法基数
如果 radix
不是有效的基数(即不在 2 到 36 之间),则 toString(radix)
方法会抛出 RangeError
异常。
const num = 10;
console.log(num.toString(1)); // 抛出 RangeError
padStart()方法的用法?
padStart()
是 JavaScript 字符串对象的一个方法,用于在当前字符串的开始位置填充指定的字符,直到字符串的长度达到指定的长度。这个方法在处理字符串格式化时非常有用,比如在生成固定宽度的输出或者格式化数字时。
基本用法:
targetLength
:目标字符串的长度。如果原始字符串的长度大于或等于这个值,则不会进行填充。padString
(可选):用于填充的字符串。如果指定的填充字符串长度超过了targetLength
与原始字符串长度的差值,则仅使用填充字符串的前面部分。-
返回值 :
padStart()
返回一个新的字符串,该字符串的长度为targetLength
,如果原始字符串长度不足,则在字符串前面填充padString
。
string.padStart(targetLength [, padString])
应用场景:
使用长填充字符:
这里,填充字符串是 abc
,它会被循环使用直到填满所需长度,因此结果是 'abcabcabc1
'。
const str = '123';
console.log(str.padStart(10, 'abc')); // "abcabcabc1"
使用不同的填充字符:
const str = 'abc';
console.log(str.padStart(10, '*')); // "*******abc"
格式化数字:
这个示例将数字 7 格式化为 3 位数的字符串,前面用 '0'
填充。
const num = 7;
const formattedNum = num.toString().padStart(3, '0');
console.log(formattedNum); // "007"
时间戳格式化:
在这个例子中,分钟和秒数都被填充到两位数,以确保时间格式的一致性。
const minutes = 5;
const seconds = 9;
const formattedTime = `${minutes.toString().padStart(2, '0')}:${seconds.toString().padStart(2, '0')}`;
console.log(formattedTime); // "05:09"
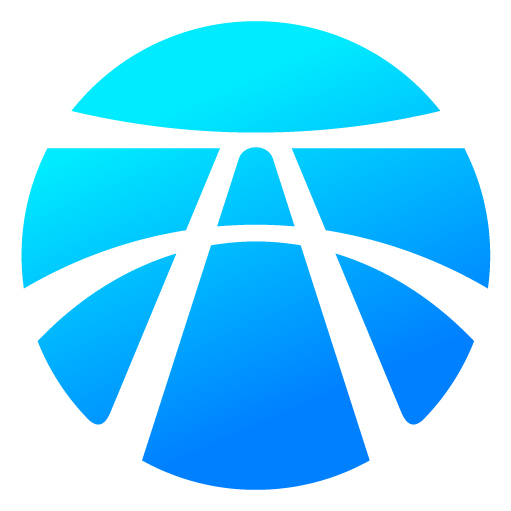
开放原子开发者工作坊旨在鼓励更多人参与开源活动,与志同道合的开发者们相互交流开发经验、分享开发心得、获取前沿技术趋势。工作坊有多种形式的开发者活动,如meetup、训练营等,主打技术交流,干货满满,真诚地邀请各位开发者共同参与!
更多推荐
所有评论(0)