复数的四则运算——C++实现
复数的四则运算法则:加法:(a+bi)+(c+di)=(a+c)+(b+d)i减法:(a+b)-(c+di)=(a-c)+(b-d)i乘法:(a+bi)(c+di)=(ac-bd)+(bc+a*d)i除法:(a+bi)/(c+di)=((ac+bd)+(bc-ad)i)/(cc+dd)**注意:**在复数除法运算中,若c、d均为0,则不可计算(除数不能为0)。C++代码:#def...
·
复数的四则运算法则:
- 加法:(a+bi)+(c+di)=(a+c)+(b+d)i
- 减法:(a+b)-(c+di)=(a-c)+(b-d)i
- 乘法:(a+bi)*(c+di)=(ac-bd)+(bc+ad)i
- 除法:(a+bi)/(c+di)=((ac+bd)+(bc-ad)i)/(c2+d2)
注意:在复数除法运算中,若c、d均为0,则不可计算(除数不能为0)。
1、代码中直接输入参数:
C++代码:
#define _CRT_SECURE_NO_WARNINGS
#include<iostream>
using namespace std;
class Complex {
public:
Complex(double real, double imag) {
this->real = real;
this->imag = imag;
}
friend Complex operator+(Complex c1, Complex c2);
friend Complex operator-(Complex c1, Complex c2);
friend Complex operator*(Complex c1, Complex c2);
friend Complex operator/(Complex c1, Complex c2);
void Print_Complex();
private:
double real;
double imag;
};
Complex operator+(Complex c1, Complex c2) {
return Complex(c1.real + c2.real, c1.imag + c2.imag);
}
Complex operator-(Complex c1, Complex c2) {
return Complex(c1.real - c2.real, c1.imag - c2.imag);
}
Complex operator*(Complex c1, Complex c2) {
return Complex(c1.real*c2.real - c1.imag*c2.imag, c1.real*c2.imag + c1.imag*c2.real);
}
Complex operator/(Complex c1, Complex c2) {
if (c2.real == 0 && c2.imag == 0) {
cout << "Error ";
return c2;
}
else {
return Complex((c1.real*c2.real + c1.imag*c2.imag) / (c2.real*c2.real + c2.imag*c2.imag), (c1.imag*c2.real - c1.real*c2.imag) / (c2.real*c2.real + c2.imag*c2.imag));
}
}
void Complex::Print_Complex() {
if (real == 0) {
cout << imag << "i" << endl;
}
else {
if (imag > 0) {
cout << real << "+" << imag << "i" << endl;
}
else {
cout << real << imag << "i" << endl;
}
}
}
int main() {
Complex c1(1, 3);
Complex c2(1, 1);
Complex c3 = c1 + c2;
cout << "Add:";
c3.Print_Complex();
Complex c4 = c1 - c2;
cout << "Minus:";
c4.Print_Complex();
Complex c5 = c1 * c2;
cout << "Mul:";
c5.Print_Complex();
Complex c6 = c1 / c2;
cout << "Div:";
c6.Print_Complex();
return 0;
}
C++运行结果:
说明:若c2的实部和虚部均为0,输出结果为:
2 、如果想自己输入参数,代码如下:
C++代码:
#define _CRT_SECURE_NO_WARNINGS
#include<iostream>
using namespace std;
class Complex {
public:
Complex() {
this->real = 0;
this->imag = 0;
}
Complex(double real, double imag) {
this->real = real;
this->imag = imag;
}
void setRI(double real, double imag) {
this->real = real;
this->imag = imag;
}
friend Complex operator+(Complex c1, Complex c2);
friend Complex operator-(Complex c1, Complex c2);
friend Complex operator*(Complex c1, Complex c2);
friend Complex operator/(Complex c1, Complex c2);
void Print_Complex();
private:
double real;
double imag;
};
Complex operator+(Complex c1, Complex c2) {
return Complex(c1.real + c2.real, c1.imag + c2.imag);
}
Complex operator-(Complex c1, Complex c2) {
return Complex(c1.real - c2.real, c1.imag - c2.imag);
}
Complex operator*(Complex c1, Complex c2) {
return Complex(c1.real*c2.real - c1.imag*c2.imag, c1.real*c2.imag + c1.imag*c2.real);
}
Complex operator/(Complex c1, Complex c2) {
if (c2.real == 0 && c2.imag == 0) {
cout << "c2 Error ";
return c2;
}
else {
return Complex((c1.real*c2.real + c1.imag*c2.imag) / (c2.real*c2.real + c2.imag*c2.imag), (c1.imag*c2.real - c1.real*c2.imag) / (c2.real*c2.real + c2.imag*c2.imag));
}
}
void Complex::Print_Complex() {
if (real == 0) {
cout << imag << "i" << endl;
}
else {
if (imag > 0) {
cout << real << "+" << imag << "i" << endl;
}
else {
cout << real << imag << "i" << endl;
}
}
}
int main() {
Complex c1, c2;
double real, imag;
cout << "Please input c1 real:";
cin >> real;
cout << "Please input c1 imag:";
cin >> imag;
c1.setRI(real, imag);
cout << "Please input c2 real:";
cin >> real;
cout << "Please input c2 imag:";
cin >> imag;
c2.setRI(real, imag);
Complex c3 = c1 + c2;
cout << "Add:";
c3.Print_Complex();
Complex c4 = c1 - c2;
cout << "Minus:";
c4.Print_Complex();
Complex c5 = c1 * c2;
cout << "Mul:";
c5.Print_Complex();
Complex c6 = c1 / c2;
cout << "Div:";
c6.Print_Complex();
return 0;
}
C++运行结果:
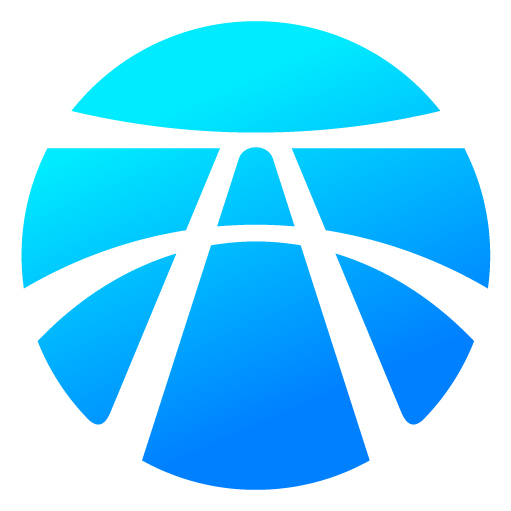
开放原子开发者工作坊旨在鼓励更多人参与开源活动,与志同道合的开发者们相互交流开发经验、分享开发心得、获取前沿技术趋势。工作坊有多种形式的开发者活动,如meetup、训练营等,主打技术交流,干货满满,真诚地邀请各位开发者共同参与!
更多推荐
所有评论(0)