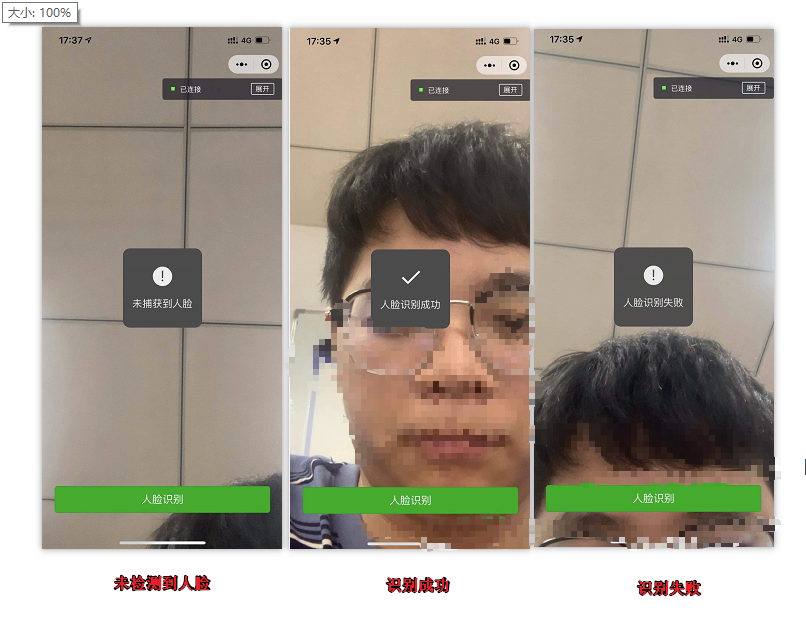
uni-app 微信小程序之实现使用百度云 人脸录入人脸识别功能
文章目录1. 实现效果2. 准备工作(开始撸)2.1 访问百度智能云注册3. 小程序代码3.1 camera.wxml3.2 camera.js3.3 camera.wxss1. 实现效果1.1 小程序进行人脸拍照上传(上传成功状态)录入成功后,可以在后台查看到自己的人脸数据1.2 小程序进行人脸拍照上传(上传失败状态)2. 准备工作(开始撸)就是需要在百度智能云注册,并创建人脸识别的应用。(完全
·
文章目录
1. 实现效果
1.1 人脸录入效果
- 未检测到人脸
- 人脸录入成功
录入成功后,可以在后台查看到自己的人脸数据
1.2 小程序进行人脸拍照上传(上传失败状态)
1.2 人脸识别效果
- 未检测到人脸
- 人脸识别成功
- 人脸识别失败
2. 准备工作(开始撸)
就是需要在百度智能云注册,并创建人脸识别的应用。(完全免费)
2.1 访问百度智能云注册
完成后进入管理控制台->产品服务->人工智能->人脸识别->创建应用->填写必要信息->立即创建
至此,我们已经完成了在云上的所有必要操作。下面,我们在小程序中,拍照上传即可。
3. 实现人脸录入代码
新建页面命名为: faceEntry
3.1 faceEntry.wxml
<view class="camera-box">
<camera device-position="front" flash="off" binderror="error" class="camera"></camera>
<view class="face-box">
<button class="face" type="primary" bindtap="takePhoto"> 人脸录入 </button>
</view>
</view>
3.2 faceEntry.js
const app = getApp()
Page({
data: {
canIUse: wx.canIUse('button.open-type.getUserInfo'),
src: "", //图片的链接
baidutoken: "",
base64: "",
msg: ""
},
//拍照
takePhoto() {
var that = this;
//拍照
const ctx = wx.createCameraContext()
ctx.takePhoto({
quality: 'high',
success: (res) => {
this.setData({
src: res.tempImagePath //获取图片
})
//图片base64编码
wx.getFileSystemManager().readFile({
filePath: this.data.src, //选择图片返回的相对路径
encoding: 'base64', //编码格式
success: res => { //成功的回调
this.setData({
base64: res.data
})
}
})
this.getBaiduToken();
} //拍照成功结束
}) //调用相机结束
//失败尝试
wx.showToast({
title: '请重试',
icon: 'loading',
duration: 500
})
},
error(e) {
console.log(e.detail)
},
getBaiduToken() {
var that = this;
//acess_token获取,qs:需要多次尝试
wx.request({
url: 'https://aip.baidubce.com/oauth/2.0/token', //是真实的接口地址
data: {
grant_type: 'client_credentials',
client_id: 'xxxxxxxxxxxxxxxxxxx', //用你创建的应用的API Key
client_secret: 'xxxxxxxxxxxxxxxxxxx' //用你创建的应用的Secret Key
},
header: {
'Content-Type': 'application/json' // 默认值
},
success(res) {
that.setData({
baidutoken: res.data.access_token //获取到token
})
that.uploadPhoto();
}
})
},
uploadPhoto() {
var that = this;
//上传人脸进行注册-----test
wx.request({
url: 'https://aip.baidubce.com/rest/2.0/face/v3/faceset/user/add?access_token=' + this.data.baidutoken,
method: 'POST',
data: {
image: this.data.base64,
image_type: 'BASE64',
group_id: 'xh_0713', //自己建的用户组id
user_id: 'xiehao' //这里获取用户昵称
},
header: {
'Content-Type': 'application/json' // 默认值
},
success(res) {
that.setData({
msg: res.data.error_msg
})
//做成功判断
if (that.data.msg == "pic not has face") {
wx.showToast({
title: '未捕获到人脸',
icon: 'error',
})
}
if (that.data.msg == 'SUCCESS') {
wx.showToast({
title: '人脸录入成功',
icon: 'success',
})
}
}
})
},
})
3.3 faceEntry.wxss
.camera-box {
width: 100%;
height: 100vh;
position: relative;
}
.camera-box .camera {
width: 100%;
height: 100%;
position: absolute;
top: 0;
}
.camera-box .face-box {
position: absolute;
width: 100%;
bottom: 40px;
padding: 40rpx;
box-sizing: border-box;
}
.camera-box .face-box .face {
width: 100%;
}
4. 实现人脸识别代码
新建页面命名为: faceRecognition
4.1 faceRecognition.wxml
<view class="camera-box">
<camera device-position="front" flash="off" binderror="error" class="camera"></camera>
<view class="face-box">
<button class="face" type="primary" bindtap="takePhoto"> 人脸识别 </button>
</view>
</view>
4.2 faceRecognition.js
Page({
data: {
src: '',
base64: "",
baidutoken: "",
msg: null
},
//拍照并编码
takePhoto() {
var that = this;
//拍照
const ctx = wx.createCameraContext()
ctx.takePhoto({
quality: 'high',
success: (res) => {
that.setData({
src: res.tempImagePath
})
//图片base64编码
wx.getFileSystemManager().readFile({
filePath: that.data.src, //选择图片返回的相对路径
encoding: 'base64', //编码格式
success: res => { //成功的回调
that.setData({
base64: res.data
})
that.checkPhoto();
}
})
}
})
wx.showToast({
title: '请重试',
icon: 'loading',
duration: 500
})
},
error(e) {
console.log(e.detail)
},
checkPhoto() {
var that = this;
//acess_token获取
wx.request({
url: 'https://aip.baidubce.com/oauth/2.0/token', //真实的接口地址
data: {
grant_type: 'client_credentials',
client_id: 'xxxxxxxxxxxxxxxxxxxxxxxxxx', //用你创建的应用的API Key
client_secret: 'xxxxxxxxxxxxxxxxxxxxxxxxxx' //用你创建的应用的Secret Key
},
header: {
'Content-Type': 'application/json' // 默认值
},
success(res) {
that.setData({
baidutoken: res.data.access_token //获取到token
})
that.validPhoto();
}
})
},
validPhoto() {
var that = this;
//上传人脸进行 比对
wx.request({
url: 'https://aip.baidubce.com/rest/2.0/face/v3/search?access_token=' + that.data.baidutoken,
method: 'POST',
data: {
image: this.data.base64,
image_type: 'BASE64',
group_id_list: 'xh_0713', //自己建的用户组id
},
header: {
'Content-Type': 'application/json' // 默认值
},
success(res) {
that.setData({
// msg: res.data.result.user_list[0].score
msg: res.data.error_msg
})
//做成功判断
if (that.data.msg == "pic not has face") {
wx.showToast({
title: '未捕获到人脸',
icon: 'error',
})
}
if (that.data.msg == 'SUCCESS') {
if(res.data.result.user_list[0].score>80){
wx.showToast({
title: '人脸识别成功',
icon: 'success',
})
}else{
wx.showToast({
title: '人脸识别失败',
icon: 'error',
})
}
}
}
});
}
})
4.3 faceRecognition.wxss
.camera-box {
width: 100%;
height: 100vh;
position: relative;
}
.camera-box .camera {
width: 100%;
height: 100%;
position: absolute;
top: 0;
}
.camera-box .face-box {
position: absolute;
width: 100%;
bottom: 40px;
padding: 40rpx;
box-sizing: border-box;
}
.camera-box .face-box .face {
width: 100%;
}
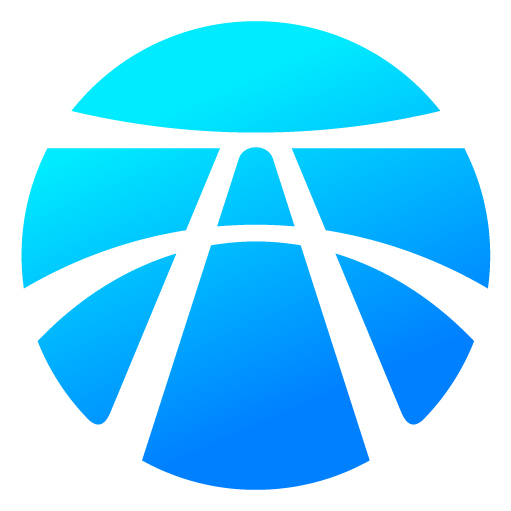
开放原子开发者工作坊旨在鼓励更多人参与开源活动,与志同道合的开发者们相互交流开发经验、分享开发心得、获取前沿技术趋势。工作坊有多种形式的开发者活动,如meetup、训练营等,主打技术交流,干货满满,真诚地邀请各位开发者共同参与!
更多推荐
所有评论(0)