numpy保存和读取dictionary字典 python
It is very important for training to record the parameters or data!Codeimport numpy as np# definedict = {'a' : {1,2,3}, 'b': {4,5,6}}# savenp.save('dict.npy',dict)# loaddict_load=np.loa...
·
It is very important for training to record the parameters or data!
Code
import numpy as np
# define
dict = {'a' : {1,2,3}, 'b': {4,5,6}}
# save
np.save('dict.npy', dict)
# load
dict_load=np.load('dict.npy', allow_pickle=True)
print("dict =", dict_load.item())
print("dict['a'] =", dict_load.item()['a'])
output:
dict = {'a': {1, 2, 3}, 'b': {4, 5, 6}}
dict['a'] = {1, 2, 3}
References
Note
numpy.savez
is also a interesting method, you could use it to record the specific array with keywords like dict
. The type of file is .npz
- Example
>>> from tempfile import TemporaryFile
>>> outfile = TemporaryFile()
>>> x = np.arange(10)
>>> y = np.sin(x)
>>> np.savez(outfile, x=x, y=y)
>>> npzfile = np.load(outfile)
>>> npzfile.files
['x', 'y']
>>> npzfile['x']
array([0, 1, 2, 3, 4, 5, 6, 7, 8, 9])
from numpy.savez
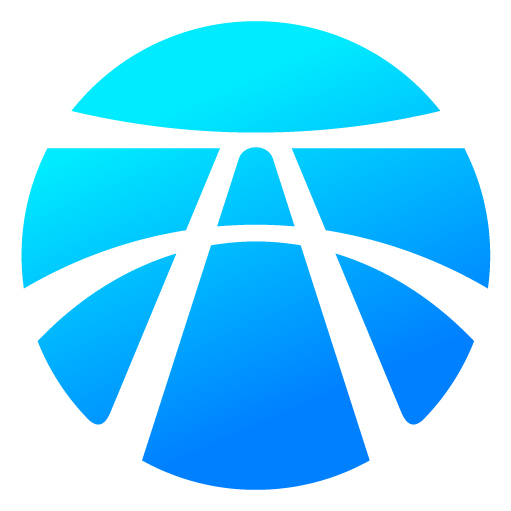
开放原子开发者工作坊旨在鼓励更多人参与开源活动,与志同道合的开发者们相互交流开发经验、分享开发心得、获取前沿技术趋势。工作坊有多种形式的开发者活动,如meetup、训练营等,主打技术交流,干货满满,真诚地邀请各位开发者共同参与!
更多推荐
所有评论(0)