Python字典(dict)与列表(list)与数组(nbarray)详解
一、字典#声明字典#一、空字典声明方式dict = {}#二、普通声明方式(key:value = 1:1)dict = {key1 : value1, key2 : value2 }#三、#字典格式:#键必须是唯一的,但值则不必。#值可以取任何数据类型,但键必须是不可变的...
·
目录
以横向方式遍历每个key的value, 每完成一行得到一个最大值(一维)
以纵向方式遍历每一个key的value,每完成一列得到一个最大值(一维)
数组先转化成列表,然后按列表的方式进行剪切拼接,然后转化回数组。
一、字典
-
声明字典
-
空字典声明方式
dict = {}
-
普通声明方式(key:value = 1:1)
#一维 dict = {key1 : value1, key2 : value2 } #多维 dict_2d = {'a': {'a': 1, 'b': 3}, 'b': {'a': 6}}
-
在字典里加入新的键值对
#一维 dict.setdefault(key,value) #二维 def addtodict2(thedict, key_a, key_b, val): if key_a in thedict: thedict[key_a].update({key_b: val}) else: thedict.update({key_a:{key_b: val}}) #多维 def addtodict3(thedict,key_a,key_b,key_c,val): if key_a in thedict: if key_b in thedict[key_a]: thedict[key_a][key_b].update({key_c:val}) else: thedict[key_a].update({key_b:{key_c:val}}) else: thedict.update({key_a:{key_b:{key_c:val}}})
(如果想一个值对应多个value,可以把value设置成一个列表或者数组)
-
字典格式:
键必须是唯一的,但值则不必。
值可以取任何数据类型,但键必须是不可变的
-
字典的遍历
-
普通循环遍历与输出对应key的value
#一维 #普通循环遍历 for key, value in dict.items(): print(key+':'+str(value)) #输出关键字对应的value print(dict[key]) #多维 for key1 in thedict: for key2 in thedict[key1]: for key3 in thedict[key1][key2]: print (key3+":"+str(thedict[key1][key2][key3]))
-
以横向方式遍历每个key的value, 每完成一行得到一个最大值(一维)
maxvaluelist=[] maxkeylist=[] valuelength=0 for k,v in data.item():获取一次data的value的长度 valuelength = len(v) break for i in range(0,valuelength):#遍历起止是0-valuelength的长度 maxvalue=0 maxkey='' for k,v in data.item():#遍历每一个key if v[i]>maxvalue : maxvalue = v[i] maxkey = k else continue maxvaluelist.append(maxvalue) maxkeylist.append(maxkey)
-
以纵向方式遍历每一个key的value,每完成一列得到一个最大值(一维)
maxvaluelist={}
for k,v in data.item():#遍历每一个key
maxvalue = 0
for item in v:
if item>maxvalue :
maxvalue = item
else: continue
maxvaluelist.setdefault(k,maxvalue)
-
字典的操作:排序与更新
-
按key排序
#正向排序 dict1={'a':2,'e':3,'f':8,'d':4} dict2 = sorted(dict1) print(dict2) #输出 >>['a', 'd', 'e', 'f'] #反向排序 dict1={'a':2,'e':3,'f':8,'d':4} dict2 = sorted(dict1,reverse=True) print(dict2) #输出 >>['f', 'e', 'd', 'a']
-
按值排序
dict1={'a':2,'e':3,'f':8,'d':4} list1= sorted(dict1.values()) print(list1) #输出 >>[2, 3, 4, 8] #设值reverse=True 可进行反向排序
-
以元组的方式分别对值和键进行排序
#用dict1.items(),得到包含键,值的元组 #由于迭代对象是元组,返回值自然是元组组成的列表 #这里对排序的规则进行了定义,x指元组,x[1]是值,x[0]是键) dict1={'a':2,'e':3,'f':8,'d':4} list1= sorted(dict1.items(),key=lambda x:x[1]) print(list1) #输出 >>[('a', 2), ('e', 3), ('d', 4), ('f', 8)] #同理对键排序有: dict1={'a':2,'e':3,'f':8,'d':4} list1= sorted(dict1.items(),key=lambda x:x[0]) print(list1) #输出 >>[('a', 2), ('d', 4), ('e', 3), ('f', 8)]
-
引用itemgetter包进行更简洁的排序
from operator import itemgetter d = {"a":8,"b":4,"c":12} print(sorted(d.items(),key=itemgetter(0),reverse=True)) print(sorted(d.items(),key=itemgetter(1),reverse=True)) #itemgetter(0),获取key #itemgetter(1),获取value #输出 >>[('c', 12), ('b', 4), ('a', 8)] >>[('c', 12), ('a', 8), ('b', 4)]
-
用updata函数进行更新
# 传入一个字典,改变原来的值 d = {'one':1,'two':2} d.update({'one':3,'two':4}) print(d) >>{'one': 3, 'two': 4} # 传入一个字典,添加新的键值对 d = {'one':1,'two':2} d.update({'three':3,'four':4}) #或者可以用它的等价方式 #d.update(three=3,four=4) #d.update([('three',3),('four',4)]) print(d) {'four': 4, 'one': 1, 'three': 3, 'two': 2}
二、列表
-
声明列表
-
空列表声明方式
list = []
-
普通列表声明方式
list1 = ['Beijing', 'Shenzhen', 1998, 2020]; list2 = [1, 2, 3, 4, 5 ]; list3 = ["a", "b", "c", "d"];
-
在列表中里加入新的值
list.append(value)
-
append函数与extend函数的区别
#使用append a = [1, 2, 3] b = [4, 5, 6] a.append(b) print(a) #输出 >>[1, 2, 3, [4, 5, 6]] #使用extend a = [1, 2, 3] b = [4, 5, 6] a.extend(b) print(a) 输出 >>[1, 2, 3, 4, 5, 6]
-
列表格式:
列表的数据项不需要有相同的类型
-
列表的遍历
-
直接按范围遍历
#一维 for i in range(0,len(list)): print(list(i)) #二维 for i in range(0,len(list)): for j in range(0,len(list[i]): print(list[i][j])
-
按元素遍历
#一维 for item in list: print(item) #二维 for x in list: for y in x: print(y)
-
列表操作:剪切,拼接
-
列表剪切
list=['123','abc',0,True] x=list[1:] y=list[:3] z=list[2:3] print(x) print(y) #输出 >>['abc', 0, True] >>['123', 'abc', 0] >>[0] #加入步长 #格式:变量[头下标:尾下标:步长] list=['123','abc',0,True,"12345"] x=list[1:4:2] print(x) #输出 >>['abc', True]
-
列表拼接
list1 = [1,2,3] list2 = [4,5,6] #使用+号拼接 newlist = list1+list2 print(newList) #输出 >> [1,2,3,4,5,6] #----------------------------- #切片拼接 newList=list1 newList[len(list1),len(list2)] = list2 #输出 >> [1,2,3,4,5,6] #----------------------------- #使用extend 上面已经提及
三、数组
-
声明数组方式
-
普通数组声明方式
#一维 np.array([2,3,4]) #多维 np.array([2,3,4],[5,6,7])
-
声明全0数组zeros
np.zeros( (3,4) ) #输出 >>>array([[ 0., 0., 0., 0.], [ 0., 0., 0., 0.], [ 0., 0., 0., 0.]])
-
声明全1数组ones
np.ones( (2,3,4), dtype=np.int16 ) # dtype can also be specified >>array([[[ 1, 1, 1, 1], [ 1, 1, 1, 1], [ 1, 1, 1, 1]], [[ 1, 1, 1, 1], [ 1, 1, 1, 1], [ 1, 1, 1, 1]]], dtype=int16)
-
声明随机数数组
np.empty( (2,3) ) # uninitialized, output may vary >>array([[ 3.73603959e-262, 6.02658058e-154, 6.55490914e-260], [ 5.30498948e-313, 3.14673309e-307, 1.00000000e+000]])
-
生成均匀分布的数组:
arange(最小值,最大值,步长)(左闭右开)
linspace(最小值,最大值,元素数量)
np.arange( 10, 30, 5 )
#输出
>>array([10, 15, 20, 25])
np.arange( 0, 2, 0.3 ) # it accepts float arguments
#输出
>>array([ 0. , 0.3, 0.6, 0.9, 1.2, 1.5, 1.8])
np.linspace( 0, 2, 9 ) # 9 numbers from 0 to 2
#输出
>>array([ 0. , 0.25, 0.5 , 0.75, 1. , 1.25, 1.5 , 1.75, 2. ])
-
数组的格式:
ndarray.ndim:数组的维数
ndarray.shape:数组每一维的大小
ndarray.size:数组中全部元素的数量
ndarray.dtype:数组中元素的类型(numpy.int32, numpy.int16, and numpy.float64等)
ndarray.itemsize:每个元素占几个字节
-
数组的遍历与列表的遍历一样
-
直接按范围遍历
#一维 for i in range(0,len(list)): print(list(i)) #二维 for i in range(0,len(list)): for j in range(0,len(list[i]): print(list[i][j])
-
按元素遍历
#一维 for item in list: print(item) #二维 for x in list: for y in x: print(y)
-
数组操作:剪切,拼接
-
数组先转化成列表,然后按列表的方式进行剪切拼接,然后转化回数组。
#列表&矩阵转数组 array = np.array(list) array = np.array(matrix) # 列表&数组转矩阵 matrix = np.mat(list) matrix = np.mat(array) # 数组&矩阵转列表 list = array.tolist() list = matrix.tolist()
-
数组的shape和reshape
import numpy as np
#shape的用法
a = np.array([1,2,3,4,5,6,7,8]) #一维数组
print(a.shape[0]) #值为8,因为有8个数据
print(a.shape[1]) #IndexError: tuple index out of range
a = np.array([[1,2,3,4],[5,6,7,8]]) #二维数组
print(a.shape[0]) #值为2,最外层矩阵有2个元素,2个元素还是矩阵。
print(a.shape[1]) #值为4,内层矩阵有4个元素。
print(a.shape[2]) #IndexError: tuple index out of range
#reshape的用法
a = np.array([1,2,3,4,5,6,7,8]) #一维数组
a_2d = a.reshape(2,4)
#输出
>>[[1,2,3,4],
[5,6,7,8]]
a_3d = a.reshape(2,2,2)
#输出
>>[[[1,2],[3,4]],[[5,6],[7,8]]]
以上为所有博主关于字典、数组、列表积累的基础知识。希望对大家有帮助!
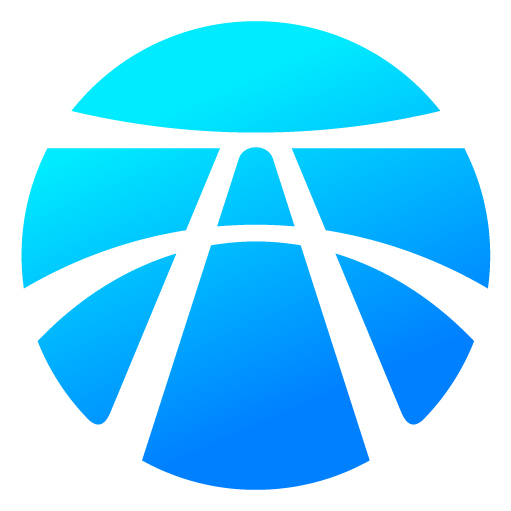
开放原子开发者工作坊旨在鼓励更多人参与开源活动,与志同道合的开发者们相互交流开发经验、分享开发心得、获取前沿技术趋势。工作坊有多种形式的开发者活动,如meetup、训练营等,主打技术交流,干货满满,真诚地邀请各位开发者共同参与!
更多推荐
所有评论(0)