实验二、Activity界面基本实验
实验二、Activity界面基本实验链接: 大佬原文:我很好请走开谢谢.【实验名称】实验二、Activity界面基本实验【实验目的】1、掌握Activity的基本功能;2、掌握preference的基本功能;3、掌握断点的设置,调试程序;【实验内容】任务1:通过intent实现跳转,完成Activity之间的跳转;任务2:intent数据的传递;任务3:采用用preference实现随数据的存储;
实验二、Activity界面基本实验
链接: 大佬原文:我很好请走开谢谢.
【实验名称】实验二、Activity界面基本实验
【实验目的】
1、掌握Activity的基本功能;
2、掌握preference的基本功能;
3、掌握断点的设置,调试程序;
【实验内容】
任务1:通过intent实现跳转,完成Activity之间的跳转;
任务2:intent数据的传递;
任务3:采用用preference实现随数据的存储;
任务4:掌握在虚拟机和真机环境下,对程序的调试;
【实验要求】
1、实现Android界面,并通过intent实现跳转,界面显示学生的姓名,学号,email.
2、要求intent的实现传递姓名,学号,email等数据,到第二个activity;
3、同时要求可以在虚拟机及手机上运行结果;
4、采用preference实现对如上数据的存储,存储及读取。
5、学会如何设置断点,并学会debug模式下,调试程序。
(请完成如下部分)
【实验设计】
1、程序主入口:
(1)MainActivity.java
该程序的默认页面,显示表单信息,通过用户填写后,将信息存至student对象中,由bundle类以键值对的方式通过Intent进行显示跳转至ResultActivity的页面,将对象信息反序列化之后使用SharedPreferences 以显示表单结果。
import android.content.Intent;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.RadioButton;
import android.widget.RadioGroup;
import android.widget.TextView;
import androidx.appcompat.app.AppCompatActivity;
import com.liu.myapplication01.R;
public class MainActivity extends AppCompatActivity {
private EditText name;
private EditText number;
private RadioButton female;
private RadioButton male;
private EditText email;
private Button btn;
private String sex;
private TextView textviewlast;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
name = findViewById(R.id.name);
number = findViewById(R.id.number);
female = findViewById(R.id.female);
male = findViewById(R.id.male);
// sex = female.isChecked()?"女":"男";
textviewlast = findViewById(R.id.textviewlast);
email = findViewById(R.id.email);
RadioGroup radioGroup = findViewById(R.id.radioGroup);
radioGroup.setOnCheckedChangeListener(new RadioGroup.OnCheckedChangeListener() {
@Override
public void onCheckedChanged(RadioGroup group, int checkedId) {
RadioButton rb = findViewById(checkedId);
sex = rb.getText().toString();
textviewlast.setText("当前选中性别:"+rb.getText());
}
});
btn = findViewById(R.id.register);
btn.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Student stu = new Student(
name.getText().toString(),
number.getText().toString(),
sex, email.getText().toString());
Bundle data = new Bundle();
data.putSerializable("student",stu);
Intent intent = new Intent(MainActivity.this,ResultActivity.class);
intent.putExtras(data);
//启动Activity
startActivity(intent);
}
});
}
}
(2)ResultActivity.java
import android.content.Intent;
import android.content.SharedPreferences;
import android.os.Bundle;
import android.widget.TextView;
import androidx.appcompat.app.AppCompatActivity;
import com.liu.myapplication01.R;
public class ResultActivity extends AppCompatActivity {
protected void onCreate(Bundle savedInstanceState){
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_result);
TextView name = findViewById(R.id.name);
TextView number = findViewById(R.id.number);
TextView sex = findViewById(R.id.sex);
TextView email = findViewById(R.id.email);
//获取本Activity的Intent
Intent intent = getIntent();
//获取传递的数据
Student stu = (Student)intent.getSerializableExtra("student");
name.setText("姓名:"+stu.getName());
number.setText("学号:"+stu.getNumber());
sex.setText("性别:"+stu.getSex());
email.setText("邮箱:"+stu.getEmail());
//拿到一个SharedPreference对象
SharedPreferences sp = getSharedPreferences("studentInfo", MODE_PRIVATE); //config为要生成的文件名
//拿到编辑器
SharedPreferences.Editor ed = sp.edit();
//写数据
ed.putString("name", name.getText().toString());
ed.putString("number",number.getText().toString());
ed.putString("sex",sex.getText().toString());
ed.putString("email",email.getText().toString());
//提交
ed.commit();
//显示
show();
}
public void show(){
//拿到一个SharedPreference对象
SharedPreferences sp = getSharedPreferences("studentInfo", MODE_PRIVATE);
//从SharedPreference里取数据
String stuName = sp.getString("name","");
String stuNumber = sp.getString("number","");
String stuSex = sp.getString("sex","");
String stuEmail = sp.getString("email","");
System.out.println("姓名:"+stuName);
System.out.println("学号:"+stuNumber);
System.out.println("性别:"+stuSex);
System.out.println("邮箱:"+stuEmail);
}
}
(3)activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<TableLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="horizontal"
tools:context="com.liu.shiyan02.MainActivity">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="请输入信息"
android:textSize="28sp"/>
<TableRow>
<TextView android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="姓名:"
android:textSize="20sp"/>
<EditText android:id="@+id/name"
android:layout_height="wrap_content"
android:layout_width="match_parent"
android:textSize="20sp"/>
</TableRow>
<TableRow>
<TextView android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="性别"
android:textSize="20sp"/>
<RadioGroup
android:id="@+id/radioGroup"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal">
<RadioButton
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/male"
android:text="男"
android:textSize="20sp"/>
<!-- android:checked="true"-->
<RadioButton
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/female"
android:text="女"
android:textSize="20sp"/>
</RadioGroup>
</TableRow>
<TableRow>
<TextView android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="学号:"
android:textSize="20sp"/>
<EditText android:id="@+id/number"
android:layout_height="wrap_content"
android:layout_width="match_parent"
android:textSize="20sp"/>
</TableRow>
<TableRow>
<TextView android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="邮箱:"
android:textSize="20sp"/>
<EditText android:id="@+id/email"
android:layout_height="wrap_content"
android:layout_width="match_parent"
android:textSize="20sp"/>
</TableRow>
<Button
android:id="@+id/register"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="注册"
android:textSize="20sp"/>
<TextView
android:id="@+id/textviewlast"
android:layout_height="wrap_content"
android:layout_width="wrap_content"
android:text=""
android:textSize="20sp"
android:gravity="center"
>
</TextView>
</TableLayout>
(4) activity_result.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="match_parent"
android:layout_height="match_parent"
>
<TextView
android:id="@+id/name"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:textSize="20sp"/>
<TextView
android:id="@+id/number"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:textSize="20sp"/>
<TextView
android:id="@+id/sex"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:textSize="20sp"/>
<TextView
android:id="@+id/email"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:textSize="20sp"/>
</LinearLayout>
(5)实体类:Student.java
package com.liu.shiyan02;
import java.io.Serializable;
public class Student implements Serializable {
private String name;
private String number;
private String sex;
private String email;
public Student(String name, String number, String sex, String email) {
this.name = name;
this.number = number;
this.sex = sex;
this.email = email;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getNumber() {
return number;
}
public void setNumber(String number) {
this.number = number;
}
public String getSex() {
return sex;
}
public void setSex(String sex) {
this.sex = sex;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
}
【实验结果】
虚拟机:
1、主界面:
2、填入信息:
3、输出结果:
手机端:
1、主页面:
2、结果:
【实验分析或心得】
通过此次实验,在性别的单选框中,想要获取不同的值(性别),还需要为其设置触发的事件:
radioGroup.setOnCheckedChangeListener(new RadioGroup.OnCheckedChangeListener() {
@Override
public void onCheckedChanged(RadioGroup group, int checkedId) {
RadioButton rb = findViewById(checkedId);
sex = rb.getText().toString();
textviewlast.setText("当前选中性别:"+rb.getText());
}
});
否则将一直是定值。
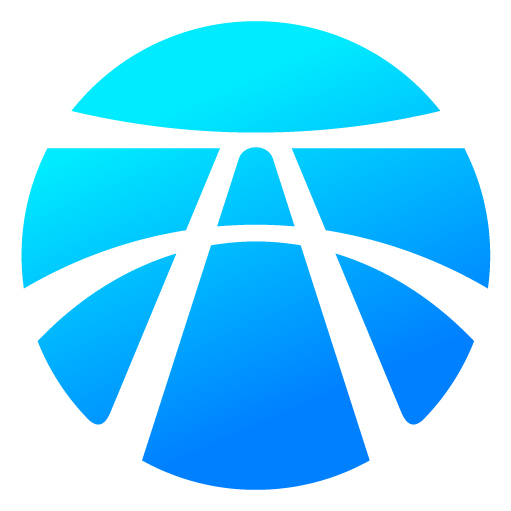
开放原子开发者工作坊旨在鼓励更多人参与开源活动,与志同道合的开发者们相互交流开发经验、分享开发心得、获取前沿技术趋势。工作坊有多种形式的开发者活动,如meetup、训练营等,主打技术交流,干货满满,真诚地邀请各位开发者共同参与!
更多推荐
所有评论(0)