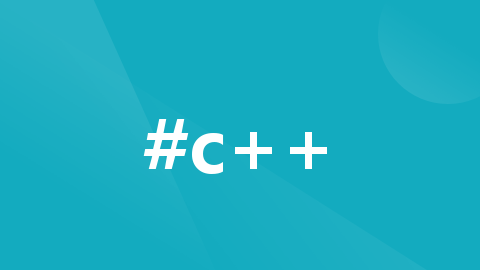
【C++】字符串(string)的使用
C++ 中,`std::string` 是用于处理字符串的标准库类。它提供了一系列成员函数和操作符,使得字符串的操作更加方便和灵活。
·
目录
一、string 简介
C++ 中,std::string
是用于处理字符串的标准库类。它提供了一系列成员函数和操作符,使得字符串的操作更加方便和灵活。
string与char*的区别:
char*
是一个指针string
本质上是一个类,类的内部封装了char*
,即string
是一个char*
型的容器string
管理char*
所分配的内存,不用担心复制越界和取值越界等
二、string 构造函数
构造函数原型 | 解释 | |
---|---|---|
1 | string() | 创建一个空的字符串 |
2 | string(const char* c) | 使用字符串c初始化 |
3 | string(const string& str) | 使用string对象初始化 |
4 | string(int n, char c) | 使用n个字符c初始化 |
示例:
#include <iostream>
#include <string>
using namespace std;
void test01()
{
string s1; //创建空字符串,调用无参构造函数
string s2("hello C++"); //把const char*转换成了string
string s3(s2); //调用拷贝构造函数,使用s2初始化s3
string s4(10, 'c');
cout << "s1 = " << s1 << endl;
cout << "s2 = " << s2 << endl;
cout << "s3 = " << s3 << endl;
cout << "s4 = " << s4 << endl;
}
int main()
{
test01();
system("pause");
return 0;
}
//result
s1 =
s2 = hello C++
s3 = hello C++
s4 = cccccccccc
三、string 字符串拼接
函数原型: += 、append | 解释 | |
---|---|---|
1 | string& operator+=(const char* str) | 重载+=操作符 |
2 | string& operator+=(const char c) | 重载+=操作符 |
3 | string& operator+=(const string& str) | 重载+=操作符 |
4 | string& append(const char *s) | 把字符串s连接到当前字符串结尾 |
5 | string& append(const char *s, int n) | 把字符串s的前n个字符连接到当前字符串结尾 |
6 | string& append(const string &s) | 同3 |
7 | string& append(const string &s, int p, int n) | 将字符串s下标p开始的n个字符连接到当前字符串结尾 |
示例:
#include <iostream>
#include <string>
using namespace std;
void test01()
{
string s1 = "AB";
s1 += "CD"; //第1种拼接方法:+=char*字符串
cout << "s1 = " << s1 << endl;
s1 += 'e'; //第2种拼接方法:+=字符
cout << "s1 = " << s1 << endl;
string s0 = "FG";
s1 += s0; //第3种拼接方法;+=string字符串
cout << "s1 = " << s1 << endl;
string s2 = "AB";
s2.append("CD"); //第4种拼接方法;append("");
cout << "s2 = " << s2 << endl;
s2.append("EFGH",3); //第5种拼接方法;append("",n);
cout << "s2 = " << s2 << endl;
string s3 = "HIJ";
s2.append(s3); //第6种拼接方法;append(string);
cout << "s2 = " << s2 << endl;
string s4 = "KLMNOPQ";
s2.append(s4,0,5); //第7种拼接方法;append(string,p,n);
cout << "s2 = " << s2 << endl;
}
int main()
{
test01();
system("pause");
return 0;
}
//result
s1 = ABCD
s1 = ABCDe
s1 = ABCDeFG
s2 = ABCD
s2 = ABCDEFG
s2 = ABCDEFGHIJ
s2 = ABCDEFGHIJKLMNO
四、string 查找与替换
函数原型:find、rfind、replace | 解释 | |
---|---|---|
1 | int find(const string& s, int p) | 从p开始查找s第一次出现位置 |
2 | int find(const char* s, int p) | 同1 |
3 | int find(const char* s, int p, int n) | 从p位置查找s的前n个字符第一次位置 |
4 | int find(const char c, int p) | 同1 |
5 | int rfind(const string& s, int p) | 从p开始查找s最后一次位置 |
6 | int rfind(const char* s, int p) | 同5 |
7 | int rfind(const char* s, int p, int n) | 从p查找s的前n个字符最后一次位置 |
8 | int rfind(const char c, int p) | 查找字符c最后一次出现位置 |
9 | string& replace(int p, int n, const string& s) | 替换p开始n个字符为字符串s |
10 | string& replace(int p, int n,const char* s) | 同9 |
注意:
find
查找是从左向后,rfind
从右向左find
与rfind
找到指定字符串时,返回查找的第一个字符的位置下标;未找到则返回-1replace
在替换时,要指定起始位置p,替换字符数量n,替换后的字符串s
示例:
#include <iostream>
#include <string>
using namespace std;
void test01()
{
//查找
string s1 = "ABCDEFGHIGK";
int p = s1.find("EF");
if (p == -1)
{
cout << "not find!" << endl;
}
else
{
cout << "find p = " << p << endl;
}
p = s1.rfind("EF");
if (p == -1)
{
cout << "not find!" << endl;
}
else
{
cout << "rfind p = " << p << endl;
}
}
void test02()
{
//替换
string s1 = "ABCDEF";
s1.replace(1, 3, "0000");
cout << "s1 = " << s1 << endl;
}
int main()
{
test01();
test02();
system("pause");
return 0;
}
//result
find p = 4
rfind p = 4
s1 = A0000EF
五、string 字符串比较
函数原型:compare | 解释 | |
---|---|---|
1 | int compare(const string &s) | 与字符串s比较 |
2 | int compare(const char *s) | 同1 |
注意:
- 字符串比较是按字符的ASCII码进行对比(= 返回 0) (> 返回 1) (< 返回 -1)
- 字符串对比主要是用于比较两个字符串是否相等,判断谁大谁小的意义不大
示例:
#include <iostream>
#include <string>
using namespace std;
void test01()
{
string s1 = "aaaaa";
string s2 = "AAAAA";
int ret = s1.compare(s2);
if (ret == 0)
{
cout << "s1 等于 s2" << endl;
}
else if (ret > 0)
{
cout << "s1 大于 s2" << endl;
}
else
{
cout << "s1 小于 s2" << endl;
}
}
int main()
{
test01();
system("pause");
return 0;
}
//result
s1 大于 s2
六、string 字符获取、插入、删除
函数原型:[]、at、insert、erase | 解释 | |
---|---|---|
1 | char& operator[](int n) | 重载[]操作符 |
2 | char& at(int n) | 通过.at方法获取字符 |
3 | string& insert(int p, const char* s) | 在下标p处插入字符串 |
4 | string& insert(int p, const string& str) | 同3 |
5 | string& insert(int p, int n, char c) | 在指定位置插入n个字符c |
6 | string& erase(int p, int n) | 删除从P开始的n个字符 |
示例:
#include <iostream>
#include <string>
using namespace std;
void test01()
{
string s = "ABCDEFG";
cout << "s[1] = " << s[1] << endl;
//修改s的第二个字符
s.at(1) = 'X';
cout << "s.at(1)" << s.at(1) << endl;
//插入字符串
s.insert(1, "555");
cout << "s.insert = " << s << endl;
//删除字符串
s.erase(1, 3);
cout << "s.erase = " << s << endl;
}
int main()
{
test01();
system("pause");
return 0;
}
//result
s[1] = B
s.at(1)X
s.insert = A555XCDEFG
s.erase = AXCDEFG
七、string 子串
函数原型:substr | 解释 | |
---|---|---|
1 | string substr(int p = 0, int n) | 返回由p开始的n个字符组成的字符串 |
示例:
#include <iostream>
#include <string>
using namespace std;
void test01()
{
string s1 = "ABCDEFG";
//截取子字符串
string s2 = s1.substr(1,4);
cout << "s2 = " << s2 << endl;
}
int main()
{
test01();
system("pause");
return 0;
}
//result
s2 = BCDE
如果这篇文章对你有所帮助,渴望获得你的一个点赞!
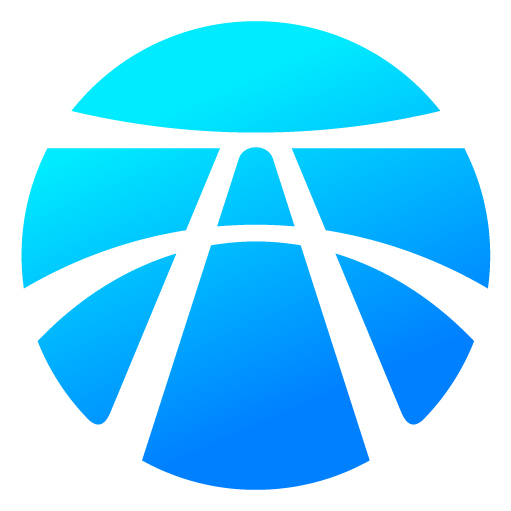
开放原子开发者工作坊旨在鼓励更多人参与开源活动,与志同道合的开发者们相互交流开发经验、分享开发心得、获取前沿技术趋势。工作坊有多种形式的开发者活动,如meetup、训练营等,主打技术交流,干货满满,真诚地邀请各位开发者共同参与!
更多推荐
所有评论(0)