cocotb使用指南(一)
关于对于cocotb这个芯片测试框架的理解。
·
前序知识
- 阅读这篇文章,需要对python,verilog有基础的知识。
- 需要对芯片验证有一定的了解。
1. cocotb安装
- 经过测试,
pip install cocotb
可以直接成功安装cocotb。 - 如果说要使用example,请把github上的cocotb下载到你的工作目录。
2. 案例一
- 我们要测试硬件设计,那必须得先有一个硬件设计,这里演示一个最简单的D flip-flop。
- D触发器有一个输入、一个输出和一个时脉输入,当时脉由0转为1时,输出的值会和输入的值相等。此类触发器可用于防止因为噪声所带来的错误,以及通过管线增加处理资料的数量。
- 在你的工作文件夹创建文件
dff.sv
。
// dff.sv
`timescale 1us/1ns
module dff (
output logic q,
input logic clk, d
);
always @(posedge clk) begin
q <= d;
end
endmodule
- 创建
test_dff.py
,一个简单的随机测试如下:
# test_dff.py
import random
import cocotb
from cocotb.clock import Clock
from cocotb.triggers import FallingEdge
@cocotb.test()
async def test_dff_simple(dut):
""" Test that d propagates to q """
clock = Clock(dut.clk, 10, units="us") # 在clk上创建一个10ns的时序
cocotb.start_soon(clock.start()) # 开始这个时序
for i in range(10):
val = random.randint(0, 1)
dut.d.value = val # 在这个时序里反复
await FallingEdge(dut.clk)
assert dut.q.value == val, "output q was incorrect on the {}th cycle".format(i)
- 上面这个测试的specification是
dut.q.value
是否恒等于val
- 然后需要编写Makefile文件
# Makefile
TOPLEVEL_LANG = verilog
VERILOG_SOURCES = $(shell pwd)/dff.sv
TOPLEVEL = dff
MODULE = test_dff
include $(shell cocotb-config --makefiles)/Makefile.sim
cocotb-config
是cocotb
的一个命令。cocotb-config --makefiles
打印的是一个存有makefiles
信息的文件夹。/home/ziyue/.local/lib/python3.8/site-packages/cocotb/share/makefiles
文件夹。/home/ziyue/.local/lib/python3.8/site-packages/cocotb/share/makefiles/Makefile.sim
应该是一个库文件。
- 最后通过
make SIM=icarus
运行程序。
make -f Makefile results.xml
make[1]: Entering directory '/home/ziyue/researchlib/Micro_Eletronic/cocotb_test'
MODULE=test_dff TESTCASE= TOPLEVEL=dff TOPLEVEL_LANG=verilog \
/usr/local/bin/vvp -M /home/ziyue/.local/lib/python3.8/site-packages/cocotb/libs -m libcocotbvpi_icarus sim_build/sim.vvp
-.--ns INFO cocotb.gpi ..mbed/gpi_embed.cpp:76 in set_program_name_in_venv Did not detect Python virtual environment. Using system-wide Python interpreter
-.--ns INFO cocotb.gpi ../gpi/GpiCommon.cpp:99 in gpi_print_registered_impl VPI registered
0.00ns INFO Running on Icarus Verilog version 11.0 (stable)
0.00ns INFO Running tests with cocotb v1.6.2 from /home/ziyue/.local/lib/python3.8/site-packages/cocotb
0.00ns INFO Seeding Python random module with 1655349072
0.00ns WARNING Pytest not found, assertion rewriting will not occur
0.00ns INFO Found test test_dff.test_dff_simple
0.00ns INFO running test_dff_simple (1/1)
95000.00ns INFO test_dff_simple passed
95000.00ns INFO **************************************************************************************
** TEST STATUS SIM TIME (ns) REAL TIME (s) RATIO (ns/s) **
**************************************************************************************
** test_dff.test_dff_simple PASS 95000.00 0.00 73259585.25 **
**************************************************************************************
** TESTS=1 PASS=1 FAIL=0 SKIP=0 95000.00 0.00 19889132.68 **
**************************************************************************************
make[1]: Leaving directory '/home/ziyue/researchlib/Micro_Eletronic/cocotb_test'
- 这边它说我们没装
pytest
,我们装一下:pip install pytest
。
make -f Makefile results.xml
make[1]: Entering directory '/home/ziyue/researchlib/Micro_Eletronic/cocotb_test'
MODULE=test_dff TESTCASE= TOPLEVEL=dff TOPLEVEL_LANG=verilog \
/usr/local/bin/vvp -M /home/ziyue/.local/lib/python3.8/site-packages/cocotb/libs -m libcocotbvpi_icarus sim_build/sim.vvp
-.--ns INFO cocotb.gpi ..mbed/gpi_embed.cpp:76 in set_program_name_in_venv Did not detect Python virtual environment. Using system-wide Python interpreter
-.--ns INFO cocotb.gpi ../gpi/GpiCommon.cpp:99 in gpi_print_registered_impl VPI registered
0.00ns INFO Running on Icarus Verilog version 11.0 (stable)
0.00ns INFO Running tests with cocotb v1.6.2 from /home/ziyue/.local/lib/python3.8/site-packages/cocotb
0.00ns INFO Seeding Python random module with 1655349984
0.00ns INFO Found test test_dff.test_dff_simple
0.00ns INFO running test_dff_simple (1/1)
95000.00ns INFO test_dff_simple passed
95000.00ns INFO **************************************************************************************
** TEST STATUS SIM TIME (ns) REAL TIME (s) RATIO (ns/s) **
**************************************************************************************
** test_dff.test_dff_simple PASS 95000.00 0.00 62473954.88 **
**************************************************************************************
** TESTS=1 PASS=1 FAIL=0 SKIP=0 95000.00 0.01 13723398.80 **
**************************************************************************************
make[1]: Leaving directory '/home/ziyue/researchlib/Micro_Eletronic/cocotb_test'
问题:@cocotb.test()
是什么?
@
在python中指的是装饰器,装饰器就是在不修改被装饰器对象源代码以及调用方式的前提下为被装饰对象添加新功能。- 有一篇非常好的博客介绍了装饰器这个性质,python装饰器详解。
- 我们继续往下:
问题:python的内的协程是什么,await的用处又是什么?
- 首先我们得理解cocotb的工作原理:
- cocotb测试集不需要额外的RTL代码,这和其他的测试工具不同。(其他的方案往往需要对RTL代码进行手动,或者是自动的插桩、修改,进行了这些操作的代码统称为DUT(Design Under Test))
- cocotb驱动激发输入到DUT,并从python直接检测输出结果。
- 一个测试往往是Python函数。
- 在任何时间,要么是模拟器在推进时间,要么是Python代码在执行。
await
的关键字是用于指出何时将执行控制传回simulator。一个测试可以产生多个协程,允许独立的执行流(每个协程可以单独执行自己的测试方案)。
- 而像
cocotb.test()
这种类型的,我们来看看它的注释:
Decorator to mark a Callable which returns a Coroutine as a test.
The test decorator provides a test timeout, and allows us to mark tests as skipped or expecting errors or failures.
Tests are evaluated in the order they are defined in a test module.
- 其实对于
test
这样的函装饰器,需求是在基本不改变源码(或者对源码改动比较小的)的情况下,添加协程
、规范(Sepcification)
等功能。 await
代表触发的时机,在这里FallingEdge(下降沿)
的时候,关于FallingEdge
可以看这里Simulator Triggers。
问题:Makefile怎么书写,要注意什么?
# Makefile
# defaults
SIM ?= icarus
TOPLEVEL_LANG ?= verilog
VERILOG_SOURCES += $(PWD)/my_design.sv
# use VHDL_SOURCES for VHDL files
# TOPLEVEL is the name of the toplevel module in your Verilog or VHDL file
TOPLEVEL = my_design
# MODULE is the basename of the Python test file
MODULE = test_my_design
# include cocotb's make rules to take care of the simulator setup
include $(shell cocotb-config --makefiles)/Makefile.sim
- 默认的仿真器是
SIM
。 - 默认的语言是
verilog
。 - 要实例化的顶层模块是
toplevel
,my_design
。
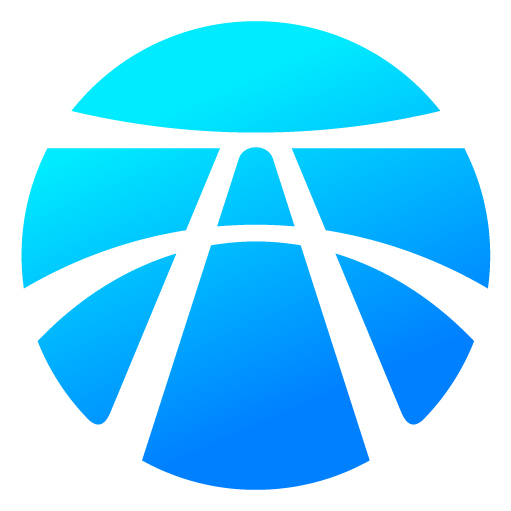
开放原子开发者工作坊旨在鼓励更多人参与开源活动,与志同道合的开发者们相互交流开发经验、分享开发心得、获取前沿技术趋势。工作坊有多种形式的开发者活动,如meetup、训练营等,主打技术交流,干货满满,真诚地邀请各位开发者共同参与!
更多推荐
所有评论(0)