基于QT开发的音乐播放器(附源码)
基于QT开发的音乐播放器(附源码)一、简介1、介绍2、功能描述3、系统功能层次模块图4、各模块功能描述(1)播放界面(2)歌词(3)歌曲信息(4)歌曲列表5、文件格式6、运行环境7、软件总体模型二、源代码LyricWidget.cppwidget.cppLyricWidget.uiwidget.ui一、简介1、介绍基于C++语言的QT开发,主要的知识点有:QT控件的使用、信号与槽函数和子窗口的设置
基于QT开发的音乐播放器(附源码)
一、简介
1、介绍
基于C++语言的QT开发,主要的知识点有:QT控件的使用、信号与槽函数和子窗口的设置。
2、功能描述
音乐播放器实现的功能有:暂停和播放音乐、切换歌曲、调节音量、显示歌曲的图片、改变进度条、显示歌曲的相关信息、与歌曲同步显示歌词、显示歌曲列表、刷新歌曲列表、点击歌曲便可切换歌曲的列表。
3、系统功能层次模块图
4、各模块功能描述
(1)播放界面
点击播放按键可以播放音乐,点击暂停按键可以暂停音乐,点击上一曲按键切换歌曲到上一曲,点击下一曲按键切换歌曲到下一曲。
点击加大音量和减小音量的按键来调节音量,也可通过移动音量滑动条、在框中输入数字和点击框中的上下键来调节。
歌曲的图片会随着歌曲的变化而变化,如果没有歌曲,则会显示默认图片。
改变进度条,歌曲及其播放时间也会随着改变。
鼠标停留在按键上时,会显示该按键的功能。
(2)歌词
歌曲从歌曲对应的txt文件中调用,通过QMap键值对来存储每一句歌词,再通过键将每一句歌词调用并显示到界面中,能够与歌曲同步显示歌词。
(3)歌曲信息
歌曲信息从歌曲对应的txt文件中调用,通过QMap键值对来存储开头几行的歌曲信息,歌曲名字的键是0、歌手名字的键是1000、专辑名字的键是2000、提供者名字的键是3000,再通过键分别将其显示到界面中。
(4)歌曲列表
歌曲都存放该项目文件的music文件夹中,歌曲列表能够显示在music文件下所有的mp3歌曲,点击列表中的歌曲,便可以播放该歌曲。如果在music文件夹中添加歌曲,点击刷新便可在列表中显示该歌曲。
5、文件格式
1、歌曲文件为mp3格式。
2、歌词文件为txt格式。
6、运行环境
名称 | 版本 | 语种 | |
---|---|---|---|
操作系统 | Qt Creator | 4.3.0 | UTF-8 |
7、软件总体模型
二、源代码
在本账号的资源中有分享出源文件
LyricWidget.cpp
#include "LyricWidget.h"
#include "ui_LyricWidget.h"
LyricWidget::LyricWidget(QWidget *parent) :
QWidget(parent),
ui(new Ui::LyricWidget)
{
ui->setupUi(this);
}
LyricWidget::~LyricWidget()
{
delete ui;
}
void LyricWidget::slotShowCurrentLrc(){
initlrc(musicName);
}
void LyricWidget::initlrc(const QString& fileName)
{
QString l;
QFile file(LrcPath+fileName);
if(file.exists()){
// qDebug("文件存在");
}
else{
// qDebug("文件不存在");
}
if (!file.open(QIODevice::ReadOnly | QIODevice::Text)){
return;
}
QTextCodec *codec = QTextCodec::codecForName("UTF-8");
while(!file.atEnd())
{
QString line = codec->toUnicode(file.readLine());
QString s(line);
if(s.length()<4)
continue;
if(s.startsWith("[ti:"))
{
getHeader("[ti:", s);
map.insert(0, s);
ui->topmusicname->setText("歌曲:"+s);
continue;
}
else if(s.startsWith("[ar:"))
{
getHeader("[ar:", s);
map.insert(1000, s);
ui->singer->setText("歌手:"+s);
continue;
}
else if(s.startsWith("[al:"))
{
getHeader("[al:", s);
map.insert(2000, s);
ui->album->setText("专辑:"+s);
continue;
}
else if(s.startsWith("[by:"))
{
getHeader("[by:", s);
ui->fromlab->setText("提供者:"+s);
map.insert(3000, s);
continue;
}
else
{
getTime(s);
}
}
file.close();
}
// 获取lrc文件的每句的时间
void LyricWidget::getTime(QString line)
{
if(line.startsWith("["))
{
int index = line.indexOf("]");
QString t = line.mid(1, index - 1);
QString mm = t.mid(0,2);
QString ss = t.mid(3, 2);
int m = mm.toInt();
int s = ss.toInt();
int time = m*60*1000 + s*1000;
t = line.mid(index + 1);
index = t.lastIndexOf("]");
if(index < 0)
{
map.insert(time, t);
}
else
{
t = t.mid(index + 1);
map.insert(time, t);
getTime(t);
}
}
}
void LyricWidget::getHeader(const char *str, QString &des)
{
des = des.remove(str);
des = des.remove("]");
}
void LyricWidget::showWord(int time)
{
if(map.contains(time))
{
ui->label->setText(map.value(time));
}
}
void LyricWidget::cleanmap(){
map.clear();
}
widget.cpp
#include "widget.h"
#include "ui_widget.h"
Widget::Widget(QWidget *parent) :
QWidget(parent),
ui(new Ui::Widget)
{
ui->setupUi(this);
isPlay=true;
isSilence=false;
lrc=new LyricWidget(this);
lrc->setWindowFlags(Qt::CustomizeWindowHint|Qt::FramelessWindowHint);
lrc->move(210, 20);
lrc->show();
ui->startBtn->setIcon(QIcon(":/image/PlayMusic.png"));// 暂停时候显示播放图标
ui->startBtn->setIconSize(QSize(50,50));
ui->startBtn->setFlat(true);
ui->startBtn->setStyleSheet("QToolTip{border:1px solid rgb(0, 0, 0); background-color: #ffffff; color:#484848; font-size:12px;}");
ui->startBtn->setToolTip(tr("播放"));// 鼠标停留时显示播放文字
ui->lastBtn->setIcon(QIcon(":/image/circle_previous.png"));// 显示上一曲图标
ui->lastBtn->setIconSize(QSize(50,50));
ui->lastBtn->setFlat(true);
ui->lastBtn->setStyleSheet("QToolTip{border:1px solid rgb(0, 0, 0); background-color: #ffffff; color:#484848; font-size:12px;}");
ui->lastBtn->setToolTip(tr("上一曲"));// 鼠标停留时显示上一曲文字
ui->nextBtn->setIcon(QIcon(":/image/circle_next.png"));// 显示下一曲图标
ui->nextBtn->setIconSize(QSize(50,50));
ui->nextBtn->setFlat(true);
ui->nextBtn->setStyleSheet("QToolTip{border:1px solid rgb(0, 0, 0); background-color: #ffffff; color:#484848; font-size:12px;}");
ui->nextBtn->setToolTip(tr("下一曲"));// 鼠标停留时显示下一曲文字
ui->addBtn->setIcon(QIcon(":/image/addIcon.png"));// 显示加大音量图标
ui->addBtn->setIconSize(QSize(30,30));
ui->addBtn->setFlat(true);
ui->addBtn->setStyleSheet("QToolTip{border:1px solid rgb(0, 0, 0); background-color: #ffffff; color:#484848; font-size:12px;}");
ui->addBtn->setToolTip(tr("加大音量"));// 鼠标停留时显示加大音量文字
ui->loseBtn->setIcon(QIcon(":/image/loseIcon.png"));// 显示减小音量图标
ui->loseBtn->setIconSize(QSize(30,30));
ui->loseBtn->setFlat(true);
ui->loseBtn->setStyleSheet("QToolTip{border:1px solid rgb(0, 0, 0); background-color: #ffffff; color:#484848; font-size:12px;}");
ui->loseBtn->setToolTip(tr("减小音量"));// 鼠标停留时显示减小音量文字
fileList = getFileNames(this->MusicPath);
QImage *img=new QImage;
img->load(":/image/soundOn.png");
*img=img->scaled(20,20,Qt::KeepAspectRatio);
ui->label->setPixmap(QPixmap::fromImage(*img));
player = new QMediaPlayer;
playerlist = new QMediaPlaylist(player);
ui->strTime->setText("00:00");
ui->volumeSlider->setRange(0,100);
ui->volumeSpinBox->setRange(0,100);
ui->volumeSlider->setValue(5);
ui->volumeSpinBox->setValue(5);
player->setVolume(5);
Vposition=5;
for(int i=0;i<fileList.size();i++)
{
QString fileName=fileList.at(i);
(fiaddItemleName);
playerlist->addMedia(QUrl::fromLocalFile(MusicPath+"\\"+fileName));
}
playerlist->setCurrentIndex(0);
player->setPlaylist(playerlist);
// 切换歌曲的同时改变歌曲名和歌曲图片
connect(player, SIGNAL(metaDataChanged()), this, SLOT(musicInfo()));
connect(ui->volumeSlider, SIGNAL(valueChanged(int)), ui->volumeSpinBox, SLOT(setValue(int)));
connect(ui->volumeSpinBox, SIGNAL(valueChanged(int)), ui->volumeSlider, SLOT(setValue(int)));
connect(ui->volumeSlider, SIGNAL(valueChanged(int)), this, SLOT(volumChange(int)));
connect(ui->volumeSpinBox, SIGNAL(valueChanged(int)), this, SLOT(volumChange(int)));
connect(ui->startBtn,SIGNAL(clicked(bool)),this,SLOT(play_pause_music()));
connect(ui->listWidget,SIGNAL(currentRowChanged(int)),this,SLOT(play_list_music(int)));
//进度条改变时播放位置随着改变
connect(ui->progressBar,SIGNAL(sliderMoved(int)),this,SLOT(setPlayerPosition(int)));
//当播放歌曲位置改变时progressBar设置对应的位置 对应的播放时间与总时间的显示
connect(player,SIGNAL(positionChanged(qint64)),this,SLOT(positionChanged(qint64)));
// 当progressBar改变时 构造歌曲总时间
connect(player, SIGNAL(durationChanged(qint64)), this, SLOT(durationChanged(qint64)));
connect(player,&QMediaPlayer::currentMediaChanged,lrc,&LyricWidget::slotShowCurrentLrc);
connect(player,&QMediaPlayer::currentMediaChanged,lrc,&LyricWidget::cleanmap);
connect(player, SIGNAL(stateChanged(QMediaPlayer::State)), this, SLOT(skipFirst(QMediaPlayer::State)));
}
Widget::~Widget()
{
delete ui;
}
void Widget::skipFirst(QMediaPlayer::State MusicState)
{
//当歌曲播放到最后一首结束时,跳到第一首
if(MusicState == QMediaPlayer::StoppedState)
{
MusicCurrent=0;
//设置当前的currentIndex
playerlist->setCurrentIndex(MusicCurrent);
isPlay=true;
play_pause_music();
return;
}
}
void Widget::on_lastBtn_clicked()
{
//播放列表为空,按钮不可用,直接返回
if(playerlist->isEmpty())
return;
int MusicCount=playerlist->mediaCount();
int MusicCurrent=playerlist->currentIndex();
if(MusicCurrent==0){
playerlist->setCurrentIndex(MusicCount-1);
isPlay=true;
play_pause_music();
return;
}
playerlist->previous();
isPlay=true;
play_pause_music();
}
void Widget::play_pause_music()
{
//如果播放列表为空,按钮不可用,直接返回
if(playerlist->isEmpty())
return;
if(isPlay)
{
player->play();
ui->startBtn->setIcon(QIcon(":/image/pause.png"));// 播放时候显示暂停图标
ui->startBtn->setIconSize(QSize(50,50));
ui->startBtn->setFlat(true);
ui->startBtn->setStyleSheet("QToolTip{border:1px solid rgb(0, 0, 0); background-color: #ffffff; color:#484848; font-size:12px;}");
ui->startBtn->setToolTip(tr("暂停"));
}
else
{
player->pause();
ui->startBtn->setIcon(QIcon(":/image/PlayMusic.png"));// 暂停时候显示播放图标
ui->startBtn->setIconSize(QSize(50,50));
ui->startBtn->setFlat(true);
ui->startBtn->setStyleSheet("QToolTip{border:1px solid rgb(0, 0, 0); background-color: #ffffff; color:#484848; font-size:12px;}");
ui->startBtn->setToolTip(tr("播放"));
}
isPlay=!isPlay;
}
void Widget::on_nextBtn_clicked()
{
//如果播放列表为空,按钮不可用,直接返回
if(playerlist->isEmpty())
return;
//如果列表到尽头,则回到列表开头
int MusicCurrent=playerlist->currentIndex();
if(++MusicCurrent==playerlist->mediaCount())
{
MusicCurrent=0;
//设置当前的currentIndex
playerlist->setCurrentIndex(MusicCurrent);
isPlay=true;
play_pause_music();
return;
}
playerlist->next();
isPlay=true;
play_pause_music();
}
void Widget::musicInfo()
{
QString musicName=player->metaData("Title").toString().toUtf8().data();
QString authorName=player->metaData("Author").toString().toUtf8().data();
// 设置歌曲名
ui->nameLab->setText("歌曲名: "+musicName+" - "+authorName);
if(!(musicName=="" || authorName==""))
{
musicLrcName=musicName;
authorLrcName=authorName;
}
// 设置lrc子窗口 歌词文件的路径
lrc->musicName=authorLrcName+" - "+musicLrcName+".txt";
lrc->slotShowCurrentLrc();
// 显示歌曲的图片
QImage picImage= player->metaData(QStringLiteral("ThumbnailImage")).value<QImage>();
if(picImage.isNull())
{
ui->nameLab->setText("歌曲名: ");
picImage=QImage(":/image/non-music.png");
}
ui->imageLab->setPixmap(QPixmap::fromImage(picImage));
ui->imageLab->setScaledContents(true);
}
void Widget::volumChange(int position)
{
Vposition=position;
player->setVolume(position);
if(position == 0)
{
QImage *img=new QImage;
img->load(":/image/volumeMuted.png");
*img=img->scaled(20,20,Qt::KeepAspectRatio);
ui->label->setPixmap(QPixmap::fromImage(*img));
}
else
{
QImage *img=new QImage;
img->load(":/image/soundOn.png");
*img=img->scaled(20,20,Qt::KeepAspectRatio);
ui->label->setPixmap(QPixmap::fromImage(*img));
}
}
// 当进度条改变时,设置歌曲对应的播放位置
void Widget::setPlayerPosition(int position)
{
player->setPosition(position);
}
// 当播放歌曲位置改变时,progressBar设置对应的位置、对应的播放时间与总时间的显示
void Widget::positionChanged(qint64 position)//
{
ui->progressBar->setValue(position);//position 单位:毫秒 1000ms=1s
currentTime=new QTime(0,(position/60000)%60,(position/1000)%60);//构造当前时间(小时,分钟,秒和毫秒)
QString t=currentTime->toString("mm:ss");
ui->strTime->setText(currentTime->toString("mm:ss"));//播放时间 输出时间格式09:02(都是两位)
QString mm = t.mid(0,2);
QString ss = t.mid(3, 2);
int m = mm.toInt();
int s = ss.toInt();
time = m*60*1000 + s*1000;
lrc->showWord(time);
}
// 当progressBar改变时,构造歌曲总时间
void Widget::durationChanged(qint64 duration)
{
ui->progressBar->setRange(0,duration);// progressBar的范围从0到duration(总时间)
totalTime=new QTime(0,(duration/60000)%60,(duration/1000)%60);
ui->endTime->setText(totalTime->toString("mm:ss"));//歌曲总时间
}
QStringList Widget::getFileNames(const QString &path)
{
QDir dir(path);
QStringList nameFilters;
nameFilters << "*.mp3";
QStringList files = dir.entryList(nameFilters, QDir::Files|QDir::Readable, QDir::Name);
return files;
}
void Widget::on_addBtn_clicked()
{
Vposition+=1;
if(Vposition > 100)
{
Vposition=100;
}
volumChange(Vposition);
ui->volumeSpinBox->setValue(Vposition);
ui->volumeSlider->setValue(Vposition);
}
void Widget::on_loseBtn_clicked()
{
Vposition-=1;
if(Vposition < 0)
{
Vposition=0;
}
volumChange(Vposition);
ui->volumeSpinBox->setValue(Vposition);
ui->volumeSlider->setValue(Vposition);
}
void Widget::addItem(QString name)
{
QListWidgetItem *item = new QListWidgetItem;
item->setText(name);
if (name != NULL) {
ui->listWidget->addItem(item);
}
}
void Widget::play_list_music(int index)
{
playerlist->setCurrentIndex(index);
isPlay=true;
play_pause_music();
}
void Widget::on_pushButton_clicked()
{
playerlist->clear();
ui->listWidget->clear();
fileList = getFileNames(this->MusicPath);
for(int i=0;i<fileList.size();i++)
{
QString fileName=fileList.at(i);
addItem(fileName);
playerlist->addMedia(QUrl::fromLocalFile(MusicPath+"\\"+fileName));
}
playerlist->setCurrentIndex(0);
isPlay=false;
play_pause_music();
}
void Widget::paintEvent( QPaintEvent* )
{
QPainter myPainter(this);
myPainter.setOpacity(0.9);
myPainter.drawPixmap(0,0,this->width(),this->height(),QPixmap(":/image/background.jpg"));
}
LyricWidget.ui
<?xml version="1.0" encoding="UTF-8"?>
<ui version="4.0">
<class>LyricWidget</class>
<widget class="QWidget" name="LyricWidget">
<property name="geometry">
<rect>
<x>0</x>
<y>0</y>
<width>565</width>
<height>389</height>
</rect>
</property>
<property name="windowTitle">
<string>Form</string>
</property>
<property name="styleSheet">
<string notr="true"/>
</property>
<widget class="QGroupBox" name="groupBox">
<property name="geometry">
<rect>
<x>10</x>
<y>260</y>
<width>551</width>
<height>121</height>
</rect>
</property>
<property name="styleSheet">
<string notr="true">color: rgb(0, 0, 0);
font: 15pt "黑体";
border:1px solid black;
</string>
</property>
<property name="title">
<string>歌词</string>
</property>
<widget class="QLabel" name="label">
<property name="geometry">
<rect>
<x>10</x>
<y>40</y>
<width>521</width>
<height>71</height>
</rect>
</property>
<property name="styleSheet">
<string notr="true">color: rgb(0, 0, 127);
font: 14pt "黑体";
border:0px solid white;</string>
</property>
<property name="text">
<string/>
</property>
</widget>
</widget>
<widget class="QGroupBox" name="groupBox_2">
<property name="geometry">
<rect>
<x>10</x>
<y>0</y>
<width>551</width>
<height>241</height>
</rect>
</property>
<property name="styleSheet">
<string notr="true">color: rgb(0, 0, 0);
border:1px solid black;
font: 15pt "黑体";</string>
</property>
<property name="title">
<string>歌曲信息</string>
</property>
<widget class="QLabel" name="topmusicname">
<property name="geometry">
<rect>
<x>10</x>
<y>30</y>
<width>491</width>
<height>41</height>
</rect>
</property>
<property name="styleSheet">
<string notr="true">color: rgb(0, 0, 0);
font: 13pt "黑体";
border:0px solid white;</string>
</property>
<property name="text">
<string/>
</property>
</widget>
<widget class="QLabel" name="singer">
<property name="geometry">
<rect>
<x>10</x>
<y>80</y>
<width>491</width>
<height>41</height>
</rect>
</property>
<property name="styleSheet">
<string notr="true">color: rgb(0, 0, 0);
font: 13pt "黑体";
border:0px solid white;
</string>
</property>
<property name="text">
<string/>
</property>
</widget>
<widget class="QLabel" name="album">
<property name="geometry">
<rect>
<x>10</x>
<y>130</y>
<width>491</width>
<height>41</height>
</rect>
</property>
<property name="styleSheet">
<string notr="true">color: rgb(0, 0, 0);
font: 13pt "黑体";
border:0px solid white;</string>
</property>
<property name="text">
<string/>
</property>
</widget>
<widget class="QLabel" name="fromlab">
<property name="geometry">
<rect>
<x>10</x>
<y>180</y>
<width>491</width>
<height>41</height>
</rect>
</property>
<property name="styleSheet">
<string notr="true">color: rgb(0, 0, 0);
font: 13pt "黑体";
border:0px solid white;</string>
</property>
<property name="text">
<string/>
</property>
</widget>
</widget>
<zorder>groupBox_2</zorder>
<zorder>groupBox</zorder>
</widget>
<resources/>
<connections/>
</ui>
widget.ui
<?xml version="1.0" encoding="UTF-8"?>
<ui version="4.0">
<class>Widget</class>
<widget class="QWidget" name="Widget">
<property name="geometry">
<rect>
<x>0</x>
<y>0</y>
<width>782</width>
<height>603</height>
</rect>
</property>
<property name="maximumSize">
<size>
<width>782</width>
<height>603</height>
</size>
</property>
<property name="windowTitle">
<string>Widget</string>
</property>
<property name="styleSheet">
<string notr="true"/>
</property>
<widget class="QGroupBox" name="groupBox1">
<property name="geometry">
<rect>
<x>10</x>
<y>420</y>
<width>761</width>
<height>171</height>
</rect>
</property>
<property name="styleSheet">
<string notr="true">background-color: rgb(225, 225, 225);
border-radius:15px
</string>
</property>
<property name="title">
<string/>
</property>
<widget class="QPushButton" name="startBtn">
<property name="geometry">
<rect>
<x>350</x>
<y>60</y>
<width>61</width>
<height>51</height>
</rect>
</property>
<property name="styleSheet">
<string notr="true"/>
</property>
<property name="text">
<string/>
</property>
</widget>
<widget class="QPushButton" name="nextBtn">
<property name="geometry">
<rect>
<x>430</x>
<y>60</y>
<width>61</width>
<height>51</height>
</rect>
</property>
<property name="styleSheet">
<string notr="true"/>
</property>
<property name="text">
<string/>
</property>
</widget>
<widget class="QSpinBox" name="volumeSpinBox">
<property name="geometry">
<rect>
<x>700</x>
<y>90</y>
<width>51</width>
<height>22</height>
</rect>
</property>
<property name="styleSheet">
<string notr="true">QSpinBox {
border:1px solid rgb(123, 169, 255);
background:white
}
QSpinBox::up-button {
border-image:url(:/image/up_arrow.png)
}
QSpinBox::down-button {
border-image:url(:/image/down_arrow.png)
}
QSpinBox::up-button:pressed {
margin-top:3px;
}
QSpinBox::down-button:pressed {
margin-bottom:3px;
}</string>
</property>
</widget>
<widget class="QLabel" name="nameLab">
<property name="geometry">
<rect>
<x>20</x>
<y>10</y>
<width>451</width>
<height>41</height>
</rect>
</property>
<property name="styleSheet">
<string notr="true">font: 12pt "黑体";
color: rgb(0, 0, 0);</string>
</property>
<property name="text">
<string>歌曲名</string>
</property>
</widget>
<widget class="QLabel" name="label_2">
<property name="geometry">
<rect>
<x>180</x>
<y>10</y>
<width>54</width>
<height>12</height>
</rect>
</property>
<property name="text">
<string/>
</property>
</widget>
<widget class="QLabel" name="strTime">
<property name="geometry">
<rect>
<x>160</x>
<y>140</y>
<width>41</width>
<height>16</height>
</rect>
</property>
<property name="text">
<string>Time</string>
</property>
</widget>
<widget class="QPushButton" name="lastBtn">
<property name="geometry">
<rect>
<x>270</x>
<y>60</y>
<width>61</width>
<height>51</height>
</rect>
</property>
<property name="styleSheet">
<string notr="true"/>
</property>
<property name="text">
<string/>
</property>
</widget>
<widget class="QLabel" name="label">
<property name="geometry">
<rect>
<x>620</x>
<y>60</y>
<width>31</width>
<height>41</height>
</rect>
</property>
<property name="text">
<string/>
</property>
</widget>
<widget class="QLabel" name="imageLab">
<property name="geometry">
<rect>
<x>20</x>
<y>50</y>
<width>121</width>
<height>111</height>
</rect>
</property>
<property name="styleSheet">
<string notr="true">border:1px solid white;
border-radius:0px</string>
</property>
<property name="text">
<string/>
</property>
</widget>
<widget class="QSlider" name="volumeSlider">
<property name="geometry">
<rect>
<x>660</x>
<y>50</y>
<width>21</width>
<height>71</height>
</rect>
</property>
<property name="styleSheet">
<string notr="true">QSlider
{
border-color: #cbcbcb;
}
QSlider::groove:vertical
{
background: #cbcbcb;
width: 6px;
border-radius: 1px;
padding-left:-1px;
padding-right:-1px;
padding-top:-1px;
padding-bottom:-1px;
}
QSlider::sub-page:vertical
{
background: #cbcbcb;
border-radius: 2px;
}
QSlider::add-page:vertical
{
background: qlineargradient(x1:0, y1:0, x2:0, y2:1, stop:0 #439cf4, stop:1 #439cf4);
background: qlineargradient(x1: 0, y1: 0.2, x2: 1, y2: 1, stop: 0 #439cf4, stop: 1 #439cf4);
width: 10px;
border-radius: 2px;
}
QSlider::handle:vertical
{
border-image: url(:/image/volumeHandle.png);
margin: -2px -7px -2px -7px;
height: 17px;
} </string>
</property>
<property name="orientation">
<enum>Qt::Vertical</enum>
</property>
</widget>
<widget class="QLabel" name="endTime">
<property name="geometry">
<rect>
<x>570</x>
<y>140</y>
<width>54</width>
<height>12</height>
</rect>
</property>
<property name="text">
<string>endTime</string>
</property>
</widget>
<widget class="QSlider" name="progressBar">
<property name="geometry">
<rect>
<x>200</x>
<y>140</y>
<width>361</width>
<height>22</height>
</rect>
</property>
<property name="styleSheet">
<string notr="true">QSlider{
border-color: #bcbcbc;
}
QSlider::groove:horizontal {
border: 1px solid #999999;
height: 1px;
margin: 0px 0;
left: 5px; right: 5px;
}
QSlider::handle:horizontal {
border: 0px ;
border-image: url(:/image/blur.png);
width: 15px;
margin: -7px -7px -7px -7px;
}
QSlider::add-page:horizontal{
background: qlineargradient(spread:pad, x1:0, y1:1, x2:0, y2:0, stop:0 #bcbcbc, stop:0.25 #bcbcbc, stop:0.5 #bcbcbc, stop:1 #bcbcbc);
}
QSlider::sub-page:horizontal{
background: qlineargradient(spread:pad, x1:0, y1:1, x2:0, y2:0, stop:0 #439cf3, stop:0.25 #439cf3, stop:0.5 #439cf3, stop:1 #439cf3);
}</string>
</property>
<property name="orientation">
<enum>Qt::Horizontal</enum>
</property>
</widget>
<widget class="QPushButton" name="addBtn">
<property name="geometry">
<rect>
<x>650</x>
<y>10</y>
<width>41</width>
<height>31</height>
</rect>
</property>
<property name="styleSheet">
<string notr="true"/>
</property>
<property name="text">
<string/>
</property>
</widget>
<widget class="QPushButton" name="loseBtn">
<property name="geometry">
<rect>
<x>650</x>
<y>130</y>
<width>41</width>
<height>31</height>
</rect>
</property>
<property name="styleSheet">
<string notr="true"/>
</property>
<property name="text">
<string/>
</property>
</widget>
<widget class="QLabel" name="label_3">
<property name="geometry">
<rect>
<x>710</x>
<y>60</y>
<width>31</width>
<height>16</height>
</rect>
</property>
<property name="text">
<string>音量</string>
</property>
</widget>
</widget>
<widget class="QGroupBox" name="groupBox">
<property name="geometry">
<rect>
<x>10</x>
<y>20</y>
<width>201</width>
<height>381</height>
</rect>
</property>
<property name="styleSheet">
<string notr="true">font: 15pt "黑体";
color: rgb(0, 0, 0);
border:1px solid black;
</string>
</property>
<property name="title">
<string>歌曲列表</string>
</property>
<widget class="QListWidget" name="listWidget">
<property name="geometry">
<rect>
<x>10</x>
<y>50</y>
<width>181</width>
<height>311</height>
</rect>
</property>
<property name="styleSheet">
<string notr="true">font: 12pt "黑体";
color: rgb(0, 0, 0);
border:1px solid white;
</string>
</property>
</widget>
<widget class="QPushButton" name="pushButton">
<property name="geometry">
<rect>
<x>144</x>
<y>12</y>
<width>41</width>
<height>31</height>
</rect>
</property>
<property name="styleSheet">
<string notr="true">font: 10pt "黑体";
background-color: rgb(225, 225, 225);
color: rgb(0, 0, 0);
border:1px solid rgb(126, 126, 126);
border-radius:5px</string>
</property>
<property name="text">
<string>刷新</string>
</property>
</widget>
</widget>
<zorder>groupBox</zorder>
<zorder>groupBox1</zorder>
</widget>
<layoutdefault spacing="6" margin="11"/>
<resources/>
<connections/>
</ui>
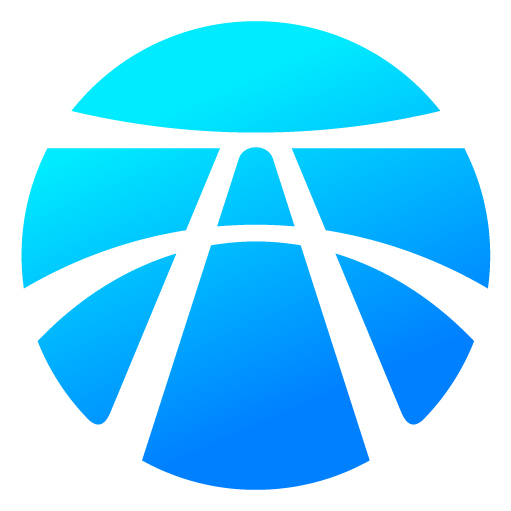
开放原子开发者工作坊旨在鼓励更多人参与开源活动,与志同道合的开发者们相互交流开发经验、分享开发心得、获取前沿技术趋势。工作坊有多种形式的开发者活动,如meetup、训练营等,主打技术交流,干货满满,真诚地邀请各位开发者共同参与!
更多推荐
所有评论(0)