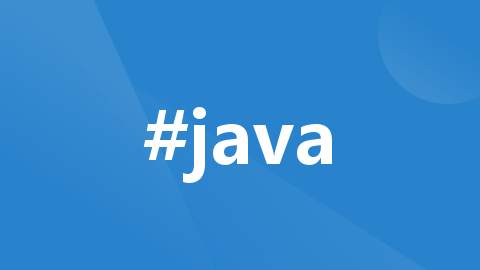
NumberFormatException(数字格式异常)可能原因和解决方法
确保在进行字符串到数字的转换时,根据情况选择适当的方法,并进行适当的验证和异常处理。考虑到国际化和本地化因素,使用与用户本地设置兼容的转换方法是一种良好的实践。(数字格式异常)通常在尝试将字符串转换为数字类型时发生,但字符串的格式不符合数字的规范时抛出。确保在将字符串转换为数字时,进行适当的验证和异常处理,以避免。在处理用户输入等不确定情况时,使用。块能够更好地应对潜在的异常。
NumberFormatException
(数字格式异常)通常在尝试将字符串转换为数字类型时发生,但字符串的格式不符合数字的规范时抛出。以下是可能导致NumberFormatException
的一些常见原因和解决方法:
-
字符串包含非数字字符:
- 可能原因: 字符串中包含不能被解释为数字的字符,如字母、特殊字符或空格。
- 解决方法: 在进行转换之前,确保字符串中只包含数字字符。可以使用正则表达式或其他验证方法来检查字符串的格式。
javaCopy code
String numberString = "123a"; // Incorrect: string contains non-numeric characters int number = Integer.parseInt(numberString); // This will throw NumberFormatException
javaCopy code
// Correct: check for valid numeric format before conversion if (numberString.matches("\\d+")) { int numberCorrect = Integer.parseInt(numberString); // Continue with operations using numberCorrect } else { // Handle the case when the string is not in a valid numeric format }
-
字符串为空或为null:
- 可能原因: 尝试将空字符串或
null
转换为数字。 - 解决方法: 在进行转换之前,检查字符串是否为非空且非
null
,以避免NumberFormatException
。
javaCopy code
String emptyString = ""; // Incorrect: attempting to convert an empty string int number = Integer.parseInt(emptyString); // This will throw NumberFormatException
javaCopy code
// Correct: check for non-empty and non-null string before conversion if (emptyString != null && !emptyString.isEmpty()) { int numberCorrect = Integer.parseInt(emptyString); // Continue with operations using numberCorrect } else { // Handle the case when the string is empty or null }
- 可能原因: 尝试将空字符串或
-
溢出:
- 可能原因: 尝试将一个超出目标数据类型表示范围的数值字符串转换为数字。
- 解决方法: 在进行转换之前,确保数值在目标数据类型的有效范围内。
javaCopy code
String largeNumberString = "2147483648"; // Incorrect: integer overflow int number = Integer.parseInt(largeNumberString); // This will throw NumberFormatException
javaCopy code
// Correct: check for valid range before conversion long numberCorrect = Long.parseLong(largeNumberString); if (numberCorrect >= Integer.MIN_VALUE && numberCorrect <= Integer.MAX_VALUE) { int result = (int) numberCorrect; // Continue with operations using result } else { // Handle the case when the string value is out of the valid range for an integer }
-
使用
try-catch
进行异常处理:- 可能原因: 在某些情况下,可能难以事先验证字符串是否可以成功转换为数字。例如,用户输入的情况。
- 解决方法: 使用
try-catch
块捕获NumberFormatException
,并在捕获到异常时采取适当的措施。
javaCopy code
String userInput = "abc"; // Incorrect: attempting to convert user input without handling exceptions int number = Integer.parseInt(userInput); // This will throw NumberFormatException
javaCopy code
// Correct: use try-catch block to handle NumberFormatException try { int numberCorrect = Integer.parseInt(userInput); // Continue with operations using numberCorrect } catch (NumberFormatException e) { // Handle the case when the conversion fails e.printStackTrace(); }
确保在将字符串转换为数字时,进行适当的验证和异常处理,以避免NumberFormatException
的发生。在处理用户输入等不确定情况时,使用try-catch
块能够更好地应对潜在的异常。
-
使用
Scanner
或NumberFormat
进行安全转换:- 可能原因: 直接使用
parseInt
等方法进行转换时,容易出现异常。更安全的方式是使用Scanner
或NumberFormat
等工具类。 - 解决方法: 使用
Scanner
或NumberFormat
进行转换,并在转换之前进行适当的检查。
javaCopy code
import java.text.NumberFormat; import java.text.ParseException; import java.util.Scanner; String userInput = "123"; // Incorrect: using parseInt directly int number = Integer.parseInt(userInput); // This may throw NumberFormatException
javaCopy code
// Correct: using Scanner for safe conversion try (Scanner scanner = new Scanner(userInput)) { if (scanner.hasNextInt()) { int numberCorrect = scanner.nextInt(); // Continue with operations using numberCorrect } else { // Handle the case when the conversion fails } }
javaCopy code
// Correct: using NumberFormat for safe conversion try { NumberFormat format = NumberFormat.getInstance(); Number numberObj = format.parse(userInput); int numberCorrect = numberObj.intValue(); // Continue with operations using numberCorrect } catch (ParseException | ClassCastException e) { // Handle the case when the conversion fails e.printStackTrace(); }
- 可能原因: 直接使用
-
处理小数和浮点数:
- 可能原因: 在将字符串转换为小数或浮点数时,可能存在格式问题。
- 解决方法: 使用适当的方法,如
Double.parseDouble
,并在转换之前进行适当的检查。
javaCopy code
String decimalString = "12.34"; // Incorrect: using parseInt for decimal string int number = Integer.parseInt(decimalString); // This will throw NumberFormatException
javaCopy code
// Correct: using Double.parseDouble for decimal string try { double numberCorrect = Double.parseDouble(decimalString); // Continue with operations using numberCorrect } catch (NumberFormatException e) { // Handle the case when the conversion fails e.printStackTrace(); }
-
国际化和本地化:
- 可能原因: 在处理数字时,考虑到国际化和本地化因素是重要的。
- 解决方法: 使用与用户本地设置兼容的
NumberFormat
进行转换。
javaCopy code
String localizedNumberString = "1.234,56"; // Example for German locale // Incorrect: using parseInt without considering locale int number = Integer.parseInt(localizedNumberString); // This will throw NumberFormatException
javaCopy code
// Correct: using NumberFormat with locale for safe conversion try { NumberFormat format = NumberFormat.getInstance(Locale.GERMANY); Number numberObj = format.parse(localizedNumberString); int numberCorrect = numberObj.intValue(); // Continue with operations using numberCorrect } catch (ParseException | ClassCastException e) { // Handle the case when the conversion fails e.printStackTrace(); }
确保在进行字符串到数字的转换时,根据情况选择适当的方法,并进行适当的验证和异常处理。考虑到国际化和本地化因素,使用与用户本地设置兼容的转换方法是一种良好的实践。
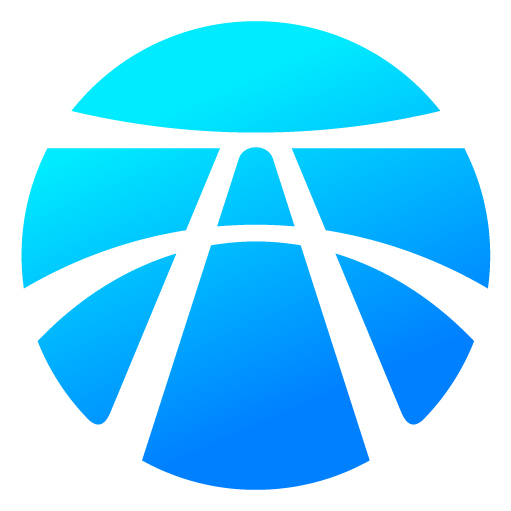
开放原子开发者工作坊旨在鼓励更多人参与开源活动,与志同道合的开发者们相互交流开发经验、分享开发心得、获取前沿技术趋势。工作坊有多种形式的开发者活动,如meetup、训练营等,主打技术交流,干货满满,真诚地邀请各位开发者共同参与!
更多推荐
所有评论(0)