python-gitlab API基本操作以及(下载指定文件或文件夹)
一、使用python对gitlab进行自动化操作1.python-gitlab模块官网文档添加链接描述2.gitlab官网文档添加链接描述二、常用使用功能//1.登录gitlabdef login():gl = gitlab.Gitlab('https://git.4399.com', private_token='dfmkbarHTTSLSGDsdqwt4')r...
·
一、使用python对gitlab进行自动化操作
二、常用使用功能
//1.登录gitlab
def login():
gl = gitlab.Gitlab('https://git.4399.com', private_token='dfmkbarHTTSLSGDsdqwt4')
return gl
gl = login()
//2.获取指定项目(项目ID)
project = gl.projects.get(4920)
//3.查看此项目所有分支
branches = project.branches.list()
print branches
//4.查找分支,存在则删除
try:
branch = project.branches.get('ReplaceWeb')
project.branches.delete('ReplaceWeb')
print u"删除旧分支成功"
except gitlab.exceptions.GitlabGetError as error:
print(error)
print u"旧分支不存在"
//5.创建分支
try:
branch = project.branches.create({'branch': 'ReplaceWeb', 'ref': 'develop'})
print u"创建新分支ReplaceWeb成功"
except gitlab.exceptions.GitlabCreateError as error:
print(error)
//6.上传多文件,可以多次执行create、update、delete、move、chmod等操作
//需要注意的是,对于二进制文件,压缩包文件需要使用base64进行编码
data = {
'branch': 'ReplaceWeb',
'commit_message': 'update web file',
'actions': [
{
'action': 'update',
'file_path': '/assist/src/main/AppConfig.h',
'content': open('D:/WebContent/AppConfig.h').read(),
},
{
'action': 'update',
'file_path': '/bin/web_content/assist_web.zip',
'encoding': 'base64',
'content': base64.b64encode(open('D:/WebContent/assist_web.zip', "rb").read()),
}
]
}
project.commits.create(data)
//7.请求合并操作
mr = project.mergerequests.create({'source_branch': 'ReplaceWeb',
'target_branch': 'develop',
'title': 'update oa_web pack'})
三、python-gitlab:下载文件或者指定文件夹
1.下载文件
//需要先创建本地目录,先删除再创建
def emptyFolder(pathName):
if 1 == os.path.exists(pathName):
shutil.rmtree(pathName)
allPath = 'D:/WebContent/'
emptyFolder(allPath)
os.makedirs(allPath)
//下载gitlab文件到本地,先打开本地文件,file_path是远程Git路径,ref是分支
with open('D:/WebContent/AppConfig.h', 'wb') as f:
project.files.raw(file_path='/src/main/AppConfig.h', ref='ReplaceWeb', streamed=True, action=f.write)
print u"下载AppConfig.h成功"
2.下载文件夹
//需要先创建本地目录,先删除再创建
def emptyFolder(pathName):
if 1 == os.path.exists(pathName):
shutil.rmtree(pathName)
allPath = 'D:/WebContent/'
emptyFolder(allPath)
os.makedirs(allPath)
//获取远程文件树(path是远程Git文件夹目录,ref是分支名,递归获取)
fileList = project.repository_tree(path='bin/tools/', ref='master', recursive=True, all=True)
//本地创建文件树中的目录
for i in fileList:
if (i['type'] == "tree"):
dirs = 'D:/WebContent/%s' % i['path']
if not os.path.exists(dirs):
os.makedirs(dirs)
print u"...下载文件中..."
//下载tools文件夹
for i in fileList:
if (i['type'] == "blob"):
with open('D:/WebContent/%s' % i['path'], 'wb') as f:
project.files.raw(file_path=i['path'], ref='master', streamed=True, action=f.write)
print u"%s" %i['path']
print u"...文件下载完毕..."
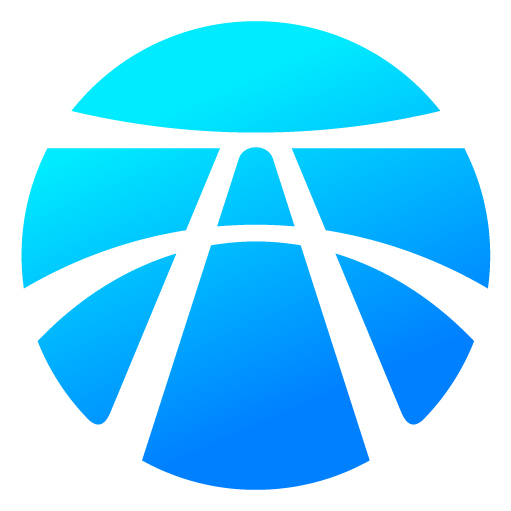
开放原子开发者工作坊旨在鼓励更多人参与开源活动,与志同道合的开发者们相互交流开发经验、分享开发心得、获取前沿技术趋势。工作坊有多种形式的开发者活动,如meetup、训练营等,主打技术交流,干货满满,真诚地邀请各位开发者共同参与!
更多推荐
所有评论(0)