Spring基本操作
一,概述1.1 Spring介绍Spring框架是目前最流行的javaEE开源框架之一。它的核心功能是IOC(Inverse Of Control:控制反转)和AOP(Aspect Oriented Programming:面向切面编程)。除此以外,Spring还提供了Spring MVC,Spring JDBC,以及业务层事务管理等众多出色的企业级应用技术,它还能整合开源世界众多著名的第三方..
一,概述
1.1 Spring介绍
Spring框架是目前最流行的javaEE开源框架之一。它的核心功能是IOC(Inverse Of Control:控制反转)和AOP(Aspect Oriented Programming:面向切面编程)。除此以外,Spring还提供了Spring MVC,Spring JDBC,以及业务层事务管理等众多出色的企业级应用技术,它还能整合开源世界众多著名的第三方框架和类库。
- 面向接口编程:一个类依赖另外一个类的时候,并不是直接依赖它,而是依赖它的接口。
- 面向接口编程的优点:降低类与类之间的耦合度。
- Spring框架可以帮我们解决类与类之间的耦合问题,降低耦合度,提高程序的维护性。
1.2 搭建环境
1.2.1导入核心Spring的jar包
commons-logging-1.2.jar
spring-instrument-tomcat-4.0.5.RELEASE.jar
spring-orm-4.0.5.RELEASE.jar
spring-core-4.0.5.RELEASE.jar
spring-tx-4.0.5.RELEASE.jar
spring-jdbc-4.0.5.RELEASE.jar
spring-framework-bom-4.0.5.RELEASE.jar
spring-oxm-4.0.5.RELEASE.jar
spring-messaging-4.0.5.RELEASE.jar
spring-context-4.0.5.RELEASE.jar
spring-websocket-4.0.5.RELEASE.jar
spring-beans-4.0.5.RELEASE.jar
spring-jms-4.0.5.RELEASE.jar
spring-context-support-4.0.5.RELEASE.jar
spring-aop-4.0.5.RELEASE.jar
spring-webmvc-portlet-4.0.5.RELEASE.jar
spring-web-4.0.5.RELEASE.jar
spring-aspects-4.0.5.RELEASE.jar
spring-webmvc-4.0.5.RELEASE.jar
spring-test-4.0.5.RELEASE.jar
spring-instrument-4.0.5.RELEASE.jar
spring-expression-4.0.5.RELEASE.jar
1.2.2 在项目下创建bean类
package lmc.bean;
public class Hello {
private String name;
public void setName(String name) {
this.name = name;
}
public void show(){
System.out.println("hello, "+name);
}
}
1.2.3 在src目录下创建beans.xml文件
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd">
<!--bean就是Java对象,由spring来创建和管理-->
<bean name="hello" class="lmc.bean.Hello"/>
</beans>
1.2.4 测试
package lmc.test;
import lmc.bean.Hello;
import org.omg.CORBA.portable.ApplicationException;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class Test {
public static void main(String[] args){
//解析beans.xml文件,生成管理相应的bean对象
ApplicationContext context = new ClassPathXmlApplicationContext("beans.xml");
Hello hello = (Hello) context.getBean("hello");
hello.show();
}
}
- 控制的内容:指谁来控制对象的创建,传统的应用程序中对象的创建是由程序本身控制的,使用Spring后是由Spring来创建对象的。
- 反转:正转指程序来创建对象;反转指程序本身不去创建对象,而变为被动接收的对象。
- 总结:以前对象是由程序本身来创建,使用Spring后,程序变为被动接收Spring创建好的对象(控制反转-----依赖注入)。
1.3 Bean的配置
如果需要把一个对象交给Spring容器来管理,那么就需要在Spring配置文件的Bean节点对该对象进行配置。
配置Bean的语法格式:
<bean id=”” class=”” scope=”” init-method="" destroy-method=""/>
参数说明:
-
id:对象的唯一标识。Spring用户会根据id的值创建对象。
-
class:对象所在类的完整类名。
-
scope:对象的作用范围。该属性的取值为:
1)singleton:默认值,服务器启动的时候,Spring容器中就会创建一个对象。如果指定了singleton,那么在Spring容器中只有一个Bean对象。
2)prototype:每次调用获取Bean对象的时候,Spring容器都会创建一个Bean对象。
3)request:在web项目中,该对象只在Request中有效。
4)session:在web项目中只在session中有效。
5)globalSession:在web项目中,该对象只在Portlet环境中有效。如果没有Portlet环境,那么该作用域就相当于session。
-
init-method:指定类中初始化方法的名称;
-
destory-method:指定类中销毁方法的名称
二,控制反转(IOC)
所谓依赖注入,就是spring容器负责创建bean对象,并把对象传入到一个类里面,而不是我们手动把对象传入到其他类中。
2.1 构造注入
通过构造函数向一个bean注入数据。
定义格式:
<bean ...>
<constructor-arg name="" index="" value="" type="" ref=""/>
<constructor-arg name="" index="" value="" type="" ref=""/>
<constructor-arg name="" index="" value="" type="" ref=""/>
...
</bean>
参数说明:
- name:参数名
- value:参数值
- index:参数的下标
- type:参数类型
- ref:引用其他bean对象
例如:
<bean id="userBean" class="com.lmc.demo02spring入门示例.UserBean" scope="prototype" init-method="init" destroy-method="destroy">
<!-- 构造注入 -->
<!-- <constructor-arg name="name" value="mickey" />
<constructor-arg name="age" value="18" /> -->
<constructor-arg index="0" value="jacky" type="String"/>
<constructor-arg index="1" value="20" type="int"/>
<constructor-arg index="2" ref="petBean" />
</bean>
<bean id="petBean" class="com.lmc.demo02spring入门示例.PetBean">
<constructor-arg index="0" value="小黑" type="String"/>
<constructor-arg index="1" value="黑色" type="String"/>
</bean>
注意:使用构造注入的bean需要提供相应的构造函数
2.2 属性注入
通过setter方法把数据传入到bean对象中。
定义格式:
<bean ...>
<property name="" value= ref=""/>
</bean>
参数说明:
- name:属性值。注意:spring是根据setter方法来判断属性的。
- value:属性值。
- ref:引用其他bean对象。
例如:
<bean id="userService" class="com.lmc.demo03依赖注入.UserService">
<!-- 属性注入
属性名:setter方法,去掉set后,首字母小写
-->
<property name="userDao" ref="userDao"/>
</bean>
<bean id="userDao" class="com.lmc.demo03依赖注入.MyBatisUserDao"/>
注意:
1)使用属性注入的bean需要提供相应的setter方法。setter方法的名字就是name属性的首字母大写,然后在前面加上set。比如上面的userDao属性,在UserService中就应该提供一个名为setUserDao的方法。
2)因为执行属性注入前,spring容器会调用bean类的无参构造对象创建对象,然后再把属性注入到该对象中。所以bean类必须要提供一个无参构造函数。
三,注解使用
3.1 基本注解
- @Controller:用在控制器类上,例如springMVC
- @Service:用在业务类上
- @Repository:用在Dao类上,例如mapper接口
- @Component:可以用在任何类上
3.2 使用注解
第一步:创建一个类,使用@Component注解标注。
@Component("ub")
class UserBean {
public void code() {
System.out.println("写代码...");
}
}
第二步:在配置文件上配置包扫描功能。
<context:component-scan base-package="com.lmc"/>
第三步:测试
public static void main(String[] args) {
// 创建Spring容器
ClassPathXmlApplicationContext ac = new ClassPathXmlApplicationContext("beans-annotation.xml");
// 获取Bean
UserBean ub = (UserBean) ac.getBean("ub");
ub.code();
}
3.3 其他注解
3.3.1 @Autowired注解
如果在成员属性上使用该注解,那么服务器启动的时候,spring容器就会自动查找该类型以及他的子类型。如果找到就会创建该类对象,并且注入到该成员属性中。
@Autowired
DeviceBean deviceBean;
Spring容器会< context:component-scan base-package=“com.lmc.bean”/>指定包以及子包下查找所有DeviceBean类型或他的子类和实现类的对象,如果找到就注入到该属性中。
3.3.2 @Qualifier注解
指定名字的bean对象。它不能独立使用,必须和@Autowire一起使用。
@Autowired
@Qualifier("tvBean")
DeviceBean deviceBean;
3.3.3 @Resource注解
@Autowired和 @Qualifier的结合。
//@Autowired
//@Qualifier("tvBean")
@Resource(name="tvBean")
DeviceBean deviceBean;
3.3.4 @Scope注解
定义bean的作用范围。一般在类中使用。例如:
@Component
@Scope("singleton")
class UserBean {
}
3.3.5 @PostConstruct和@PreDestroy注解
类似于bean节点的init-method和destroy-method属性。
<bean id="userBean" class="com.lmc.demo02spring入门示例.UserBean" scope="prototype" init-method="init" destroy-method="destroy">
四,spring零配置
spring支持使用配置类来代替配置文件。零配置使用到的注解有:
4.1 @Configuration
作用:指定spring容器从当前类中加载读取配置信息。在类上使用。
@Configuration
class SpringConfig {
}
4.2 @ComponentScan
作用:指定spring容器初始化时候要扫描的包。
@Configuration
@ComponentScan(baskPackages={"com.lmc"})
class SpringConfig {
}
测试:
public static void main(String[] args) {
// 创建Spring容器
AnnotationConfigApplicationContext ac = new AnnotationConfigApplicationContext(SpringConfig.class);
// 调用getBean方法
UserBean userBean = (UserBean) ac.getBean("userBean");
userBean.eat();
}
4.3 @PropertySource
作用:加载properties文件中的配置。在类中使用。
@Configuration
@PropertySource(value={"classpath:jdbc.properties"})
class SpringConfig2 {
}
4.4 @Value
作用:给属性注入数据。在属性上使用。一般与@PropertySource一起使用。
@Value("${driverClassName}")
private String driverClassName;
@Value("${jdbcUrl}")
private String jdbcUrl;
@Value("${user}")
private String user;
@Value("${password}")
private String password;
@Value("${xxx}")的作用是读取属性文件XXX属性值,然后注入到该属性中。
4.5 @Bean
作用:该注解只能写在方法上,表明使用此方法创建一个对象,并且交给spring管理。
@Bean(name="ds")
public DataSource createDataSource() {
ComboPooledDataSource dataSource = new ComboPooledDataSource();
dataSource.setDriverClassName(driverClassName);
dataSource.setJdbcUrl(jdbcUrl);
dataSource.setUser(user);
dataSource.setPassword(password);
return dataSource;
}
五,使用spring进行单元测试
SpringTest是Spring框架用来做单元测试的工具。使用SpringTest需要结合Junit一起使用。
第一步:导入Junit的jar包;
第二步:新建一个测试类。一般来说,每一个业务类都应该对应一个测试类。
第三步:在测试类上使用SpringTest相关注解。
@RunWith:指定Junit运行器,它负责执行相关注解;
@ContextConfiguration:指定spring配置文件的位置。
@RunWith(SpringJUnit4ClassRunner.class)
@ContextConfiguration(classes={UserServiceTest.class})
@Configuration
@ComponentScan(basePackages={"com.lmc.demo06整合junit"})
public class UserServiceTest {
// 注入UserService的Bean
@Autowired
IUserService userService;
@Test
public void testAddUser() {
userService.addUser();
}
}
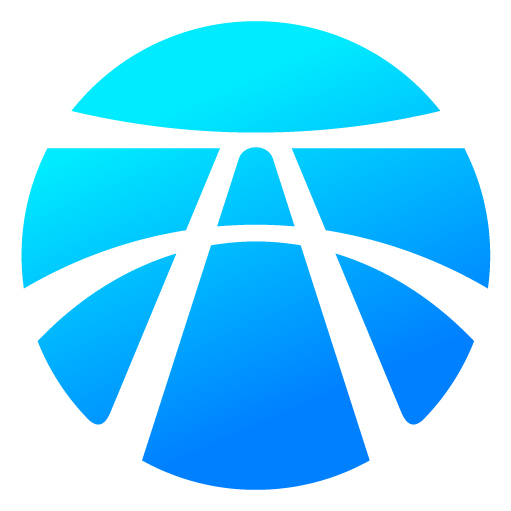
开放原子开发者工作坊旨在鼓励更多人参与开源活动,与志同道合的开发者们相互交流开发经验、分享开发心得、获取前沿技术趋势。工作坊有多种形式的开发者活动,如meetup、训练营等,主打技术交流,干货满满,真诚地邀请各位开发者共同参与!
更多推荐
所有评论(0)