学习笔记08从零开始学java-第九章课后习题
学习笔记08-第九章 图形用户界面设计用书参考:孙连英,刘畅,彭涛所著的Java面向对象程序设计。我的所有代码你都可以通过GitHub获取,以下为我的GitHub地址:[[[https://github.com/MrNeoJeep/java-code.git]]]本章习题对于新手来说比较繁杂,博主略懂皮毛,GUI学习仍需深入,本文谨提供给初学Java GUI设计的一个简单参考,代码中的一些注释阐明
·
学习笔记08-第九章 图形用户界面设计
用书参考:孙连英,刘畅,彭涛所著的Java面向对象程序设计。
我的所有代码你都可以通过GitHub获取,
以下为我的GitHub地址:[[[https://github.com/MrNeoJeep/java-code.git]]]
本章习题对于新手来说比较繁杂,博主略懂皮毛,GUI学习仍需深入,本文谨提供给初学Java GUI设计的一个简单参考,代码中的一些注释阐明实现的功能,仅供参考。
文章目录
- 学习笔记08-第九章 图形用户界面设计
(1)创建一个Frame,有两个Button按钮和一个TextField,单击按钮,在TextField上显示Button信息
代码
import java.awt.FlowLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JTextField;
public class Text9_1 {
public static void main(String[] args) {
//创建一个Frame,有两个Button按钮和一个TextField,单击按钮,在TextField上显示Button信息
testButton t1 = new testButton();
//一定要设置为可见。否则无法显示按钮和文本框
t1.setVisible(true);
}
}
class testButton extends JFrame implements ActionListener
{
JButton button1;
JButton button2;
JTextField text;
public testButton()
{
super("testButton");
this.setSize(300,300);
this.setVisible(true);
//设置默认关闭方式
this.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
//设置顺序布局(居中对齐),间距为水平10,垂直10;
this.setLayout(new FlowLayout(FlowLayout.CENTER,10,10));
text = new JTextField(30);
button1 = new JButton("按钮1");
button2 = new JButton("按钮2");
//做标记,方便下面的事件监听机制捕获这个标记,然后进行相应的处理
button1.setActionCommand("button1");
button2.setActionCommand("button2");
//将这些全部添加到frame的框架里
add(button1);
add(button2);
add(text);
button1.addActionListener(this);
button2.addActionListener(this);
}
//事件监听机制
@Override
public void actionPerformed(ActionEvent e) {
if(e.getActionCommand().equals("button1"))
{
text.setText("按钮1在这里呀!");
}else if(e.getActionCommand().equals("button2")) {
text.setText("按钮2在这里呀!");
}
}
}
运行结果
(2)做一个简易的“加减乘除”计算器
做一个简易的“加减乘除”计算器。JFrame中加入一个显示结果的标签,两个输入文本框
4个单选框(标题分别为+、-、*、/),一个按钮。分别输入两个整数,选择相应的运算符,单击后显示运算结果。
代码
import java.awt.FlowLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.ButtonGroup;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JPanel;
import javax.swing.JRadioButtonMenuItem;
import javax.swing.JTextField;
public class Text9_2 {
public static void main(String[] args) {
/*做一个简易的“加减乘除”计算器。JFrame中加入一个显示结果的标签,两个输入文本框
* 4个单选框(标题分别为+、-、*、/),一个按钮。分别输入两个整数,选择相应的运算符,单击后显示运算结果。
*
*/
Compute compute = new Compute();
compute.setVisible(true);
}
}
class Compute extends JFrame implements ActionListener
{
JLabel result;
JTextField input1;
JTextField input2;
JButton button;
JRadioButtonMenuItem plus;
JRadioButtonMenuItem subtract;
JRadioButtonMenuItem multiply;
JRadioButtonMenuItem division;
public Compute() {
super("极简计算器");
input1 = new JTextField(10);
input2 = new JTextField(10);
button = new JButton("=");
this.setSize(500,500);
this.setVisible(true);
this.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
this.setLayout(new FlowLayout(FlowLayout.CENTER,10,10));
//自定义布局
// input1.setBounds(100,150,75,75);
// input2.setBounds(300,150,75,75);
// button.setBounds(50,300,75,50);
this.add(input1);
plus = new JRadioButtonMenuItem("+");
subtract = new JRadioButtonMenuItem("-");
multiply = new JRadioButtonMenuItem("*");
division = new JRadioButtonMenuItem("/");
ButtonGroup signGroup = new ButtonGroup();
signGroup.add(plus);
signGroup.add(subtract);
signGroup.add(multiply);
signGroup.add(division);
JPanel jp = new JPanel();
jp.add(plus);
jp.add(subtract);
jp.add(multiply);
jp.add(division);
getContentPane().add(jp);
plus.addActionListener(this);
subtract.addActionListener(this);
multiply.addActionListener(this);
division.addActionListener(this);
button.setActionCommand("getres");
this.add(input2);
this.add(button);
button.addActionListener(this);
input1.addActionListener(this);
input2.addActionListener(this);
}
@Override
public void actionPerformed(ActionEvent e ){
String Text1 = input1.getText();
String Text2 = input2.getText();
try {
int num1 = Integer.parseInt(Text1);
int num2 = Integer.parseInt(Text2);
//如果加法被选择的话
if(plus.isSelected())
{
int res = num1 + num2;
//整型转字符串
//方法1
String reString = String.valueOf(res);
//方法2
//String reString = Integer.toString(res);
//方法3 自动类型转换
//String reString = ""+res;
result = new JLabel(reString);
}
//如果减法被选择的话
if(subtract.isSelected())
{
int res = num1 - num2;
//整型转字符串
//方法1
String reString = String.valueOf(res);
//方法2
//String reString = Integer.toString(res);
//方法3 自动类型转换
//String reString = ""+res;
result = new JLabel(reString);
}
//如果乘法被选择的话
if(multiply.isSelected())
{
int res = num1 * num2;
//整型转字符串
//方法1
String reString = String.valueOf(res);
//方法2
//String reString = Integer.toString(res);
//方法3 自动类型转换
//String reString = ""+res;
result = new JLabel(reString);
}
//如果除法被选择的话
if(division.isSelected())
{
double res = (double)num1 / num2;
//整型转字符串
//方法1
String reString = String.valueOf(res);
//方法2
//String reString = Integer.toString(res);
//方法3 自动类型转换
//String reString = ""+res;
result = new JLabel(reString);
}
if(e.getActionCommand().equals("getres"))
{
this.add(result);
result.setBounds(225, 100, 50, 50);
}
} catch(Exception e3) {
System.out.println("你的输入或输入顺序错误");
}
}
}
运行结果
(3)将JFrame区域分成大小相等的2*2块,分别装入4幅图片,鼠标进入哪个区域,就在该区域显示一幅图片,移出后则不显示图片
博主放弃了
(4)在JFrame中加入两个复选框,显示标题为学习和玩耍,根据选择的情况分别显示“玩耍”“学习”“劳逸结合”(两者都选)
代码
import java.awt.FlowLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.JRadioButtonMenuItem;
import javax.swing.JTextField;
public class Text9_4 {
public static void main(String[] args) {
// 在JFrame中加入两个复选框,显示标题为学习和玩耍,根据选择的情况分别显示“玩耍”“学习”“劳逸结合”(两者都选)
life l1 = new life();
l1.setVisible(true);
}
}
class life extends JFrame implements ActionListener
{
JTextField text1;
JRadioButtonMenuItem play;
JRadioButtonMenuItem study;
public life() {
super("学习玩耍");
this.setSize(300,300);
this.setVisible(true);
this.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
this.setLayout(new FlowLayout(FlowLayout.CENTER,10,10));
play = new JRadioButtonMenuItem("玩耍");
study = new JRadioButtonMenuItem("学习");
JPanel jp = new JPanel();
jp.add(play);
jp.add(study);
getContentPane().add(jp);
play.addActionListener(this);
study.addActionListener(this);
text1 = new JTextField(10);
this.add(text1);
}
@Override
public void actionPerformed(ActionEvent e) {
if(play.isSelected())
{
text1.setText("玩耍!");
}
if(study.isSelected())
{
text1.setText("学习!");
}
if(play.isSelected() && study.isSelected())
{
text1.setText("劳逸结合!");
}
}
}
运行结果
(5)编写一个可以接收字符输入的GUI程序,接收用户输入的10个整数,并输出这是个整数的最大值和最小值
代码
import java.awt.FlowLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JTextField;
public class Text9_5 {
public static void main(String[] args) {
// 编写一个可以接收字符输入的GUI程序,接收用户输入的10个整数,并输出这是个整数的最大值和最小值
AnalysisNum test1 = new AnalysisNum();
test1.setVisible(true);
}
}
class AnalysisNum extends JFrame implements ActionListener
{
JLabel text;
JLabel in;
JLabel out;
JLabel maxJLabel;
JLabel minJLabel;
JButton cal;
JTextField inArea;
JTextField maxNum;
JTextField minNum;
public AnalysisNum() {
super("输出最值");
in = new JLabel("输入");
out = new JLabel("输出");
cal = new JButton("计算");
text = new JLabel("请输入10个整数,用逗号分隔,程序将输出最大值和最小值");
inArea = new JTextField(20);
maxNum = new JTextField(10);
minNum = new JTextField(10);
maxJLabel = new JLabel("最大值");
minJLabel = new JLabel("最小值");
//给计算按钮添加事件监听
cal.addActionListener(this);
//对界面的设置
this.setSize(650,200);
this.setVisible(true);
this.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
this.setLayout(new FlowLayout(FlowLayout.CENTER,10,10));
//添加各个组件到框架中
this.add(text);
this.add(in);
this.add(inArea);
this.add(cal);
this.add(out);
this.add(maxJLabel);
this.add(maxNum);
this.add(minJLabel);
this.add(minNum);
}
@Override
public void actionPerformed(ActionEvent e) {
if(e.getSource() == cal)
{
String string = inArea.getText();
String []arr = string.split(",");
int []arrInt = new int[arr.length];
for(int i = 0; i < arr.length;i++)
{
arrInt[i] = Integer.parseInt(arr[i]);
}
//写一个简单的方法得到最大值和最小值
int maxNum1 = arrInt[0];
int minNum1 = arrInt[0];
for(int i = 0;i < arr.length;i++)
{
if(arrInt[i] >= maxNum1)
{
maxNum1 = arrInt[i];
}
if(arrInt[i] <= minNum1)
{
minNum1 = arrInt[i];
}
}
String temp1 = "" + maxNum1;
String temp2 = "" + minNum1;
maxNum.setText(temp1);
minNum.setText(temp2);
}
}
}
运行结果
(6)编写一个支持中文文本编辑程序TextEdit.java
编写一个支持中文文本编辑程序TextEdit.java,要求如下
- ①、用户界面大小为400*200像素
- ②、程序启动后,多行文本输入框TextArea中显示当前目录下myText.txt文件中原有的内容
如果文件不存在,则新建该文件- ③、“保存”按钮功能:将多行文本输入框TextArea中的内容写入myText.txt文件中保存
- ④、“取消”按钮功能;将多行文本输入框TextArea中的内容清空。
- ⑤、“退出”按钮功能:退出程序
- ⑥、窗口时间不处理
代码
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.io.BufferedReader;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.FileReader;
import java.io.IOException;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JOptionPane;
import javax.swing.JScrollPane;
import javax.swing.JTextArea;
public class Text9_6 {
public static void main(String[] args) {
/*编写一个支持中文文本编辑程序TextEdit.java,要求如下
* ①、用户界面大小为400*200像素
* ②、程序启动后,多行文本输入框TextArea中显示当前目录下myText.txt文件中原有的内容
* 如果文件不存在,则新建该文件
* ③、“保存”按钮功能:将多行文本输入框TextArea中的内容写入myText.txt文件中保存
* ④、“取消”按钮功能;将多行文本输入框TextArea中的内容清空。
* ⑤、“退出”按钮功能:退出程序
* ⑥、窗口时间不处理
*
*/
TextEdit test1 = new TextEdit();
test1.setVisible(true);
}
}
class TextEdit extends JFrame implements ActionListener
{
//定义一些组件
JButton save;
JButton cancel;
JButton exit;
JTextArea text;
//构造方法
public TextEdit() {
super("文本编辑器");
save = new JButton("保存");
cancel = new JButton("取消");
exit = new JButton("退出");
save.addActionListener(this);
cancel.addActionListener(this);
exit.addActionListener(this);
//打开即加载myText.txt文件,读取该文件,设置到文本区
File file = new File("C:\\Users\\风\\Desktop\\test\\myText.txt");
if(!file.exists())
{
try {
file.createNewFile();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
BufferedReader reader = null;
StringBuffer sbf = new StringBuffer();
try {
reader = new BufferedReader(new FileReader(file));
String tempStr;
while ((tempStr = reader.readLine()) != null) {
sbf.append(tempStr);
}
reader.close();
}
catch (IOException e) {
// TODO: handle exception
e.printStackTrace();
}
finally {
if (reader != null) {
try {
reader.close();
} catch (IOException e1) {
e1.printStackTrace();
}
}
}
//实例化文本区域
text = new JTextArea(sbf.toString(),350,200);
//设置界面
this.setSize(400,200);
this.setVisible(true);
this.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
this.setLayout(null);
save.setBounds(110,120,60,30);
cancel.setBounds(180,120,60,30);
exit.setBounds(250,120,60,30);
text.setBounds(0, 0, 400, 100);
this.add(text);
JScrollPane scrollpane = new JScrollPane(text);
scrollpane.setHorizontalScrollBarPolicy(JScrollPane.HORIZONTAL_SCROLLBAR_ALWAYS);
scrollpane.setVerticalScrollBarPolicy(JScrollPane.VERTICAL_SCROLLBAR_ALWAYS);
scrollpane.setBounds(0, 0, 400, 100);
//滚动条的大小要与文本输入区域一样大!!!!!
scrollpane.setViewportView(text);
this.add(scrollpane);
//自动换行
text.setLineWrap(true);
validate();
this.add(save);
this.add(cancel);
this.add(exit);
}
@Override
public void actionPerformed(ActionEvent e) {
if(e.getSource()==cancel)
{
//清空文本区
text.setText("");
//加入弹窗
JOptionPane.showMessageDialog(this, "已清空区域");
}
if(e.getSource() == exit)
{
//设置退出
System.exit(0);
}
if(e.getSource() == save)
{
try {
//文件流
FileOutputStream fout = new FileOutputStream("C:\\Users\\风\\Desktop\\test\\myText.txt");
try {
fout.write(text.getText().getBytes());
JOptionPane.showMessageDialog(this, "保存成功");
} catch (IOException e1) {
// TODO Auto-generated catch block
e1.printStackTrace();
}
} catch (FileNotFoundException e1) {
// TODO Auto-generated catch block
e1.printStackTrace();
}
}
}
}
运行结果
再次打开会加载之前保存的文件
初学java,代码多有不足,如有错误,非常感谢你的指正。以上代码仅供参考。
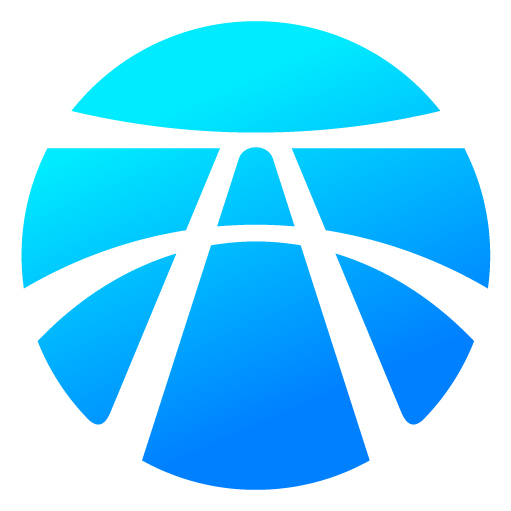
开放原子开发者工作坊旨在鼓励更多人参与开源活动,与志同道合的开发者们相互交流开发经验、分享开发心得、获取前沿技术趋势。工作坊有多种形式的开发者活动,如meetup、训练营等,主打技术交流,干货满满,真诚地邀请各位开发者共同参与!
更多推荐
所有评论(0)