dva的使用,附带一个Demo
加入QQ群:864680898,一起学习进步!点击群名可查看本人网站,有最新文章!dva的使用官方文档GIT仓库demo地址一、安装 dva-clinpm install dva-cli -g二、创建新应用dva new examplecd examplenpm start三、文件目录结构\mockmock的数据\pulic存放index.h...
·
加入QQ群:864680898,一起学习进步!点击群名可查看本人网站,有最新文章!
dva的使用官方文档
一、安装 dva-cli
npm install dva-cli -g
二、创建新应用
dva new example
cd example
npm start
三、文件目录结构
\mock mock的数据
\pulic 存放index.html
\src
\assets 存放一些静态文件,会被webpack打包
\components 存放一些公用的组件
\models 存放公用状态
\routes 页面
\services 存放复用性高的接口获取
\utils 存放一些模块
index.css
index.js 页面入口
router.js 配置路由
.roadhogrc.mock.js 配置接口数据,来模拟
.webpackrc 配置webpack
四、配置alias来使用路径
- 在根目录新建 webpack.config.js,dva项目会自动识别该文件
// webpack.config.js
module.exports = (webpackConfig, env) => {
// 别名配置
webpackConfig.resolve.alias = {
'@': `${__dirname}/src`,
}
return webpackConfig
}
五、路由和model的懒加载
- 不使用懒加载,首页加载速度会非常慢,懒加载会将路由拆成多个文件打包,使用dva的dynamic函数
import React from 'react';
import { Router, Route, Switch } from 'dva/router';
import dynamic from 'dva/dynamic';
function RouterConfig({ history, app }) {
const routes = [
{ path: '/', name: 'home', component: ()=>import('./routes/home/IndexPage') },
{ path: '/detail', name: 'detail', component: () => import('./routes/detail/Detail') },
]
return (
<Router history={history}>
<Switch>
{
routes.map(({path, name, component}) => {
return(
<Route path={path} key={name} exact component={dynamic({ app, models:[], component })} />
)
})
}
</Switch>
</Router>
);
}
export default RouterConfig;
六、model的使用
- 1、编写一个计数器的model
// /models/counter.js
export default {
namespace: 'counter',
state: 5, // 状态,和vuex中state一样
subscriptions: { // 监听,当发生变化时能dispach相应操作
setup({ dispatch, history }) { // eslint-disable-line
},
},
effects: { // 异步action,与vuex中的actions一样
*fetch({ payload }, { call, put }) { // eslint-disable-line
yield put({ type: 'save' });
},
},
reducers: { // 同步action,与vuex中的mutations一样
add(state) {
return state + 1
},
reduce(state) {
return state - 1
}
},
};
- 2、引入到index.js中
app.model(require('./models/counter').default);
- 3、到页面中使用,使用connect(类似react-redux)后,直接使用this.props.dispatch()来分发就是了
import React from 'react';
import style from './Counter.css'
import { connect } from 'dva'
class Counter extends React.Component{
constructor(props){
super(props);
this.state = {
}
}
add(){
this.props.dispatch({ type: 'counter/add' })
}
reduce(){
if (this.props.counter <= 0){
alert('我也是有底线的!')
return;
}
this.props.dispatch({ type: 'counter/reduce' })
}
render(){
return (
<div className={style.counter}>
<div className={style.reduce} onClick={()=>this.reduce()}>-</div>
<div className={style.num}>{this.props.counter}</div>
<div className={style.add} onClick={()=>this.add()}>+</div>
</div>
);
}
};
export default connect(({counter})=>({
counter
}))(Counter);
七、按需加载使用antd
- 1、安装
npm install antd babel-plugin-import --save
如果安装不上使用国内的镜像
npm install cnpm -g
cnmp install antd babel-plugin-import --save
- 2、按需引入,更改.webpackrc
{
"extraBabelPlugins": [
["import", { "libraryName": "antd", "libraryDirectory": "es", "style": "css" }]
]
}
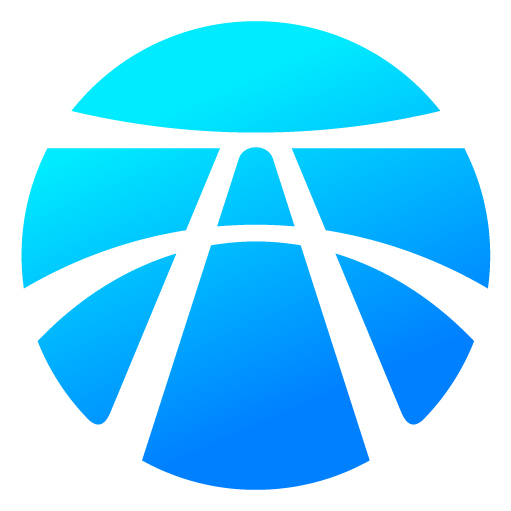
开放原子开发者工作坊旨在鼓励更多人参与开源活动,与志同道合的开发者们相互交流开发经验、分享开发心得、获取前沿技术趋势。工作坊有多种形式的开发者活动,如meetup、训练营等,主打技术交流,干货满满,真诚地邀请各位开发者共同参与!
更多推荐
所有评论(0)