生成二维码,批量导出带格式的execl
1.导入pom<dependency><groupId>com.gitee.lwpwork</groupId><artifactId>excel</artifactId><version>0.0.2-RELEASE</version></dependency>2.对象实体类pac...
·
1.导入pom
<dependency>
<groupId>com.gitee.lwpwork</groupId>
<artifactId>excel</artifactId>
<version>0.0.2-RELEASE</version>
</dependency>
2.对象实体类
package execl;
import com.lwp.excel.annotation.Cell;
import com.lwp.excel.annotation.Sheet;
import com.lwp.excel.annotation.Style;
import com.lwp.excel.annotation.Title;
import com.lwp.excel.resolver.ExcelAble;
import lombok.Data;
@Data
@Sheet(name = "二维码" )
@Title(value = "生产车间二维码",heightInPoints = 30)
public class QrCode implements ExcelAble {
@Cell(value = "编号")
private String number;
@Cell(value = "规格")
private String code;
@Cell(value = "状态")
private String status;
@Cell(value = "日期")
private String date;
@Cell(value = "创建人")
private String createBy;
@Cell(value = "二维码")
private String image;
}
3.实现类
package execl;
import com.lwp.excel.util.ExcelUtil;
import org.apache.poi.hssf.usermodel.*;
import javax.imageio.ImageIO;
import javax.swing.*;
import java.awt.*;
import java.awt.image.BufferedImage;
import java.io.ByteArrayOutputStream;
import java.util.ArrayList;
import java.util.List;
public class ExprExeclMain {
static String filePath = "C:\\Users\\pax\\Desktop\\js\\" ;
public static void main(String[] args) throws Exception {
String fileName = "qrcode.xls";
String path = filePath + fileName;
Long start = System.currentTimeMillis(); // 开始时间
List<QrCode> qrCodes = qrCodeInit();//初始化数据列表。
HSSFWorkbook wb = ExcelUtil.exportExcel(qrCodes);//生成Excel的HSSFWorkbook 对象。
auotCreateQrCode(wb,qrCodes); //设置生产二维码
ExcelUtil.createExcelFile(wb,path);//生成文件
System.out.println(System.currentTimeMillis()-start); //结束时间
}
/**
* 设置单元格样式,插入二维码
* @param wb
* @param qrCodes
* @throws Exception
*/
public static void auotCreateQrCode(HSSFWorkbook wb ,List<QrCode> qrCodes) throws Exception {
//读一张图片
String imag = "qrCodeUrl_taima_0001000001.png";
String imagePath = filePath + imag;
ByteArrayOutputStream byteArrayOut = new ByteArrayOutputStream();
Image src = Toolkit.getDefaultToolkit().getImage(imagePath);
BufferedImage bufferImg = toBufferedImage(src);
ImageIO.write(bufferImg, "jpg", byteArrayOut);
//画图的顶级管理器,一个sheet只能获取一个(一定要注意这点)
HSSFSheet sheet1 = wb.getSheetAt(0);
sheet1.setColumnWidth(5,1000 *5); // 二维码那列的宽度
HSSFPatriarch patriarch = sheet1.createDrawingPatriarch();
//生产二维码数据
for(int i = 0 ; i< qrCodes.size() ; i++){
System.out.println("数据 :"+i);
sheet1.getRow(2+i).setHeight((short) 2000); //每行数据高度
//anchor主要用于设置图片的属性
HSSFClientAnchor anchor = new HSSFClientAnchor(0, 0, 1023, 255,
(short) 5, 2 +i, (short) 5, 2+i);
anchor.setAnchorType(3);
//插入图片
patriarch.createPicture(anchor, wb.addPicture(byteArrayOut.toByteArray(), HSSFWorkbook.PICTURE_TYPE_PNG));
}
}
public static List<QrCode> qrCodeInit(){
List<QrCode> qrCodes = new ArrayList<QrCode>();
for(int i=0 ; i<10; i++){
QrCode qrCode = new QrCode();
qrCode.setNumber("No."+i);
qrCode.setCode("YX-123000"+i);
qrCode.setCreateBy("管理员");
qrCode.setDate("2021-01-06");
qrCode.setStatus("正常");
qrCodes.add(qrCode);
}
return qrCodes;
}
/**
* 图片转换
* @param image
* @return
*/
public static BufferedImage toBufferedImage(Image image) {
if (image instanceof BufferedImage) {
return (BufferedImage)image;
}
image = new ImageIcon(image).getImage();
BufferedImage bimage = null;
GraphicsEnvironment ge = GraphicsEnvironment.getLocalGraphicsEnvironment();
try {
int transparency = Transparency.OPAQUE;
GraphicsDevice gs = ge.getDefaultScreenDevice();
GraphicsConfiguration gc = gs.getDefaultConfiguration();
bimage = gc.createCompatibleImage(
image.getWidth(null), image.getHeight(null), transparency);
} catch (HeadlessException e) {
}
if (bimage == null) {
int type = BufferedImage.TYPE_INT_RGB;
bimage = new BufferedImage(image.getWidth(null), image.getHeight(null), type);
}
Graphics g = bimage.createGraphics();
g.drawImage(image, 0, 0, null);
g.dispose();
return bimage;
}
}
参考链接:https://blog.csdn.net/qq_36622496/article/details/100152838
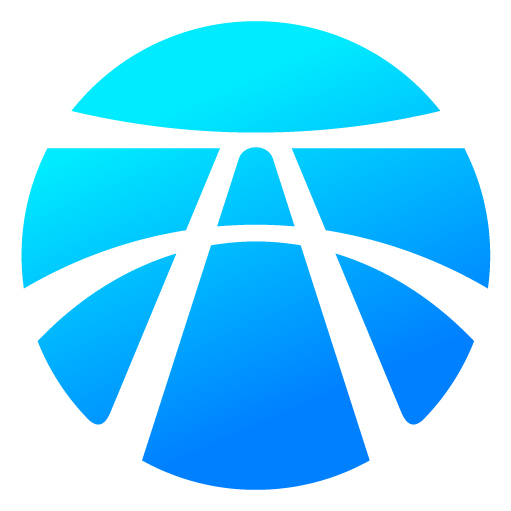
开放原子开发者工作坊旨在鼓励更多人参与开源活动,与志同道合的开发者们相互交流开发经验、分享开发心得、获取前沿技术趋势。工作坊有多种形式的开发者活动,如meetup、训练营等,主打技术交流,干货满满,真诚地邀请各位开发者共同参与!
更多推荐
所有评论(0)