Spring(一):Spring基本介绍
Spring 是一个开源框架.Spring 为简化企业级应用开发而生. 使用 Spring 可以使简单的 JavaBean 实现以前只有 EJB 才能实现的功能.Spring 是一个 IOC(DI) 和 AOP 容器框架.具体描述 Spring:轻量级:Spring 是非侵入性的 - 基于 Spring 开发的应用中的对象可以不依赖于 Spring 的 API依赖注入(DI
·
Spring 是一个开源框架.
Spring 为简化企业级应用开发而生. 使用 Spring 可以使简单的 JavaBean 实现以前只有 EJB 才能实现的功能.
Spring 是一个 IOC(DI) 和 AOP 容器框架.
具体描述 Spring:
轻量级:Spring 是非侵入性的 - 基于 Spring 开发的应用中的对象可以不依赖于 Spring 的 API
依赖注入(DI --- dependency injection、IOC)
面向切面编程(AOP --- aspect oriented programming)
容器: Spring 是一个容器, 因为它包含并且管理应用对象的生命周期
框架: Spring 实现了使用简单的组件配置组合成一个复杂的应用. 在 Spring 中可以使用 XML 和 Java 注解组合这些对象
Spring 为简化企业级应用开发而生. 使用 Spring 可以使简单的 JavaBean 实现以前只有 EJB 才能实现的功能.
Spring 是一个 IOC(DI) 和 AOP 容器框架.
具体描述 Spring:
轻量级:Spring 是非侵入性的 - 基于 Spring 开发的应用中的对象可以不依赖于 Spring 的 API
依赖注入(DI --- dependency injection、IOC)
面向切面编程(AOP --- aspect oriented programming)
容器: Spring 是一个容器, 因为它包含并且管理应用对象的生命周期
框架: Spring 实现了使用简单的组件配置组合成一个复杂的应用. 在 Spring 中可以使用 XML 和 Java 注解组合这些对象
一站式:在 IOC 和 AOP 的基础上可以整合各种企业应用的开源框架和优秀的第三方类库 (实际上 Spring 自身也提供了展现层的 SpringMVC 和 持久层的 Spring JDBC)
package com.jiangtao.spring.helloworld;
public class HelloWorld {
private String name;
public void setName(String name) {
System.out.println("setName:"+ name);
this.name = name;
}
public void hello() {
System.out.println("hello:"+name);
}
public HelloWorld() {
System.out.println("helloworld constructs...");
}
public HelloWorld(String name){
this.name = name;
}
}
<!-- 配置bean -->
<!-- 通过属性注值 通过setter方法 -->
<bean id="helloworld" class="com.jiangtao.spring.helloworld.HelloWorld">
<property name="name" value="豆豆"></property>
</bean>
package com.jiangtao.spring.helloworld;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
import com.jiangtao.spring.collection.Student;
public class Main {
public static void main(String[] args) {
//创建spring的IOC容器
ApplicationContext applicationContext =
new ClassPathXmlApplicationContext("ApplicationContext.xml");
//从容器中获取bean
HelloWorld hw = applicationContext.getBean(HelloWorld.class);
/**
* 或者这样写,helloworld为在配置文件的bean id
* HelloWorld hw = (HelloWorld)applicationContext.getBean("helloworld");
*
*/
System.out.println(hw);
//调用方法
hw.hello();
}
}
输出结果:
helloworld constructs...
setName:豆豆
com.jiangtao.spring.helloworld.HelloWorld@595660c3
hello:豆豆
这里我们可以发现,如果通过set属性赋值,是利用反射,调用无参数的构造器创建实例,然后在调用setName赋值,这个过程是在第一步就完成了,就是创建spring的IOC容器那一步
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
import com.jiangtao.spring.collection.Student;
public class Main {
public static void main(String[] args) {
//创建spring的IOC容器
ApplicationContext applicationContext =
new ClassPathXmlApplicationContext("ApplicationContext.xml");
}
}
输出结果为:
<pre name="code" class="java">helloworld constructs...
setName:豆豆
上面是通过set属性赋值,下面我们通过构造器赋值
配置文件中:
<bean id="helloworld" class="com.jiangtao.spring.helloworld.HelloWorld">
<constructor-arg name="name" value="豆豆" />
</bean>-->
<pre name="code" class="java">package com.jiangtao.spring.helloworld;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
import com.jiangtao.spring.collection.Student;
public class Main {
public static void main(String[] args) {
//创建spring的IOC容器
ApplicationContext applicationContext =
new ClassPathXmlApplicationContext("ApplicationContext.xml");
//从容器中获取bean
HelloWorld hw = applicationContext.getBean(HelloWorld.class);
/**
* 或者这样写,helloworld为在配置文件的bean id
* HelloWorld hw = (HelloWorld)applicationContext.getBean("helloworld");
*
*/
System.out.println(hw);
//调用方法
hw.hello();
}
}
输出结果:
com.jiangtao.spring.helloworld.HelloWorld@595660c3
hello:豆豆
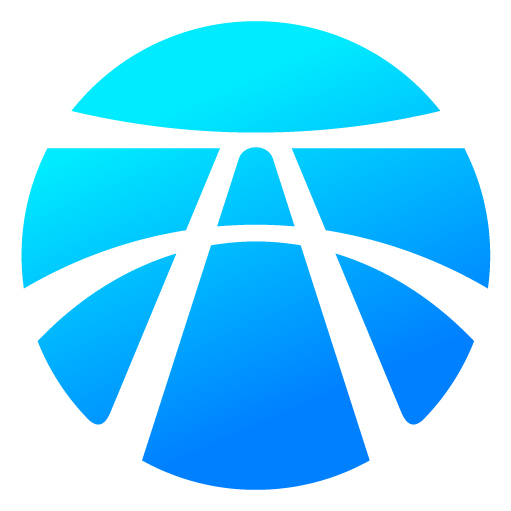
开放原子开发者工作坊旨在鼓励更多人参与开源活动,与志同道合的开发者们相互交流开发经验、分享开发心得、获取前沿技术趋势。工作坊有多种形式的开发者活动,如meetup、训练营等,主打技术交流,干货满满,真诚地邀请各位开发者共同参与!
更多推荐
所有评论(0)