leetcode 2 -- Add Two Numbers
题目You are given two linked lists representing two non-negative numbers. The digits are stored in reverse order and each of their nodes contain a single digit. Add the two numbers and return it as a
题目
You are given two linked lists representing two non-negative numbers. The digits are stored in reverse order and each of their nodes contain a single digit. Add the two numbers and return it as a linked list.
Input: (2 -> 4 -> 3) + (5 -> 6 -> 4)
Output: 7 -> 0 -> 8
题意
两链表内各逆序保存一整数,将两整数相加之和逆序存于链表,返回该链表头指针
代码
struct ListNode* addTwoNumbers(struct ListNode* l1, struct ListNode* l2) {
struct ListNode *p,*q,*head;
int num=0,c=0,i;
p=l1;
q=l2;
while(1)
{
num++;//保存较短链表的长度
p = p->next;
q = q->next;
if(q==NULL)//q指向长链表 p指向短链表
{
p=l2;
q=l1;
break;
}
if(p==NULL)
{
p=l1;
q=l2;
break;
}
}
head=q;
while(num--)//按位相加并进位
{
q->val += c + p->val;
c = q->val /10;
q->val %= 10;
p=p->next;
q=q->next;
}
while(c)//若还有位未进
{
if(q==NULL)//q为NULL说明是最后一位 直接添加节点 赋值为1并break
{
p=(struct ListNode*)malloc(sizeof(struct ListNode));
p->val=1;
p->next=NULL;
q=head;
while(q->next!=NULL)
{
q=q->next;
}
q->next=p;
break;
}
else
{
q->val += c;
c = q->val /10;
q->val %= 10;
if(q->next == NULL && c)//为链表添加节点
{
p=(struct ListNode*)malloc(sizeof(struct ListNode));
p->val=0;
p->next=NULL;
q->next=p;
q=p;
}
else
{
q=q->next;
}
}
}
return head;
}
收获
添加节点时容易犯的逻辑错误
例:q为链表指针 此时 q->next = NULL;
错 : q=q->next; q=()malloc();
对 :p=()malloc(); q->next = p;
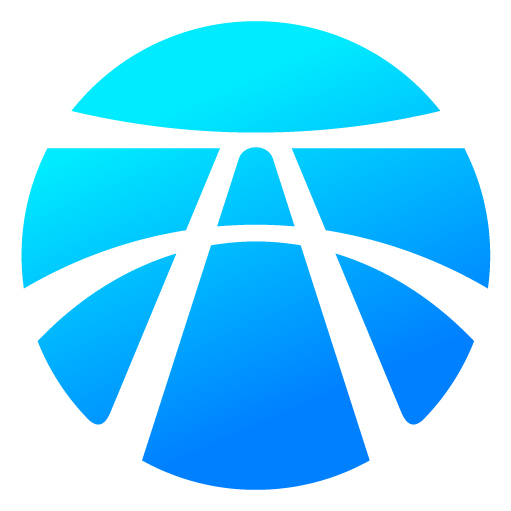
开放原子开发者工作坊旨在鼓励更多人参与开源活动,与志同道合的开发者们相互交流开发经验、分享开发心得、获取前沿技术趋势。工作坊有多种形式的开发者活动,如meetup、训练营等,主打技术交流,干货满满,真诚地邀请各位开发者共同参与!
更多推荐
所有评论(0)