Android 系统 CPU 基本信息
1.源码下载https://github.com/sufadi/AndroidCpuTools2.基本信息string name="cpu_core_number">CPU 核数: string>string name="cpu_bits">CPU 位数: string>string name="cpu_bits_64">64 位 string>
·
1.源码下载
https://github.com/sufadi/AndroidCpuTools
2.基本信息
<string name="cpu_core_number">CPU 核数: </string>
<string name="cpu_bits">CPU 位数: </string>
<string name="cpu_bits_64">64 位 </string>
<string name="cpu_bits_32">32 位 </string>
<string name="cpu_abi">CPU 指令集: </string>
<string name="cpu_plateform">CPU 平台信息: </string>
<string name="cpu_processor">CPU 架构: </string>
<string name="cpu_max_freq">CPU 最大频率: </string>
<string name="cpu_min_freq">CPU 最小频率: </string>
<string name="cpu_hz"> Hz </string>
<string name="cpu_available_frequencies">CPU 频率档位: </string>
<string name="cpu_cur_governor">CPU 当前调频策略: </string>
<string name="cpu_available_governors">CPU 支持的调频策略: </string>
3.UI 效果
4.具体源码
package com.fadisu.cpurun.util;
import android.util.Log;
import java.io.BufferedReader;
import java.io.File;
import java.io.FileFilter;
import java.io.FileNotFoundException;
import java.io.FileReader;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public class CpuUtils {
private static final String TAG = CpuUtils.class.getSimpleName();
/**
* Gets the number of cores available in this device, across all processors.
* Requires: Ability to peruse the filesystem at "/sys/devices/system/cpu"
* <p/>
* Source: http://stackoverflow.com/questions/7962155/
*
* @return The number of cores, or 1 if failed to get result
*/
public static int getNumCpuCores() {
try {
// Get directory containing CPU info
File dir = new File("/sys/devices/system/cpu/");
// Filter to only list the devices we care about
File[] files = dir.listFiles(new FileFilter() {
@Override
public boolean accept(File file) {
// Check if filename is "cpu", followed by a single digit number
if (Pattern.matches("cpu[0-9]+", file.getName())) {
return true;
}
return false;
}
});
// Return the number of cores (virtual CPU devices)
return files.length;
} catch (Exception e) {
// Default to return 1 core
Log.e(TAG, "Failed to count number of cores, defaulting to 1", e);
return 1;
}
}
/**
* 64 系统判断
*
* @return
*/
public static boolean isCpu64() {
boolean result = false;
if (BuildHelper.isCpu64() || ProcCpuInfo.isCpu64()) {
result = true;
}
return result;
}
/**
* CPU 最大频率
*
* @return
*/
public static long getCpuMaxFreq() {
long result = 0L;
try {
String line;
BufferedReader br = new BufferedReader(new FileReader("/sys/devices/system/cpu/cpu0/cpufreq/cpuinfo_max_freq"));
if ((line = br.readLine()) != null) {
result = Long.parseLong(line);
}
br.close();
} catch (IOException e) {
e.printStackTrace();
}
return result;
}
/**
* CPU 最小频率
*
* @return
*/
public static long getCpuMinFreq() {
long result = 0L;
try {
String line;
BufferedReader br = new BufferedReader(new FileReader("/sys/devices/system/cpu/cpu0/cpufreq/cpuinfo_min_freq"));
if ((line = br.readLine()) != null) {
result = Long.parseLong(line);
}
br.close();
} catch (IOException e) {
e.printStackTrace();
}
return result;
}
/**
* 可调节 CPU 频率档位
*
* @return
*/
public static String getCpuAvailableFrequenciesSimple() {
String result = null;
try {
String line;
BufferedReader br = new BufferedReader(new FileReader("/sys/devices/system/cpu/cpu0/cpufreq/scaling_available_frequencies"));
if( (line = br.readLine()) != null) {
result = line;
}
br.close();
} catch (IOException e) {
e.printStackTrace();
}
return result;
}
/**
* 可调节 CPU 频率档位
*
* @return
*/
public static List<Long> getCpuAvailableFrequencies() {
List<Long> result = new ArrayList<>();
try {
String line;
BufferedReader br = new BufferedReader(new FileReader("/sys/devices/system/cpu/cpu0/cpufreq/scaling_available_frequencies"));
if( (line = br.readLine()) != null) {
String[] list = line.split("\\s+");
for (String value : list) {
long freq = Long.parseLong(value);
result.add(freq);
}
}
br.close();
} catch (IOException e) {
e.printStackTrace();
}
return result;
}
/**
* CPU 调频策略
*
* @return
*/
public static String getCpuGovernor() {
String result = null;
try {
String line;
BufferedReader br = new BufferedReader(new FileReader("/sys/devices/system/cpu/cpu0/cpufreq/scaling_governor"));
if ((line = br.readLine()) != null) {
result = line;
}
br.close();
} catch (IOException e) {
e.printStackTrace();
}
return result;
}
/**
* CPU 支持的调频策略
*
* @return
*/
public static String getCpuAvailableGovernorsSimple() {
String result = null;
try {
String line;
BufferedReader br = new BufferedReader(new FileReader("/sys/devices/system/cpu/cpu0/cpufreq/scaling_available_governors"));
if( (line = br.readLine()) != null) {
result = line;
}
br.close();
} catch (IOException e) {
e.printStackTrace();
}
return result;
}
/**
* CPU 支持的调频策略
*
* @return
*/
public static List<String> getCpuAvailableGovernors() {
List<String> result = new ArrayList<>();
try {
String line;
BufferedReader br = new BufferedReader(new FileReader("/sys/devices/system/cpu/cpu0/cpufreq/scaling_available_governors"));
if( (line = br.readLine()) != null) {
String[] list = line.split("\\s+");
for (String value : list) {
result.add(value);
}
}
br.close();
} catch (IOException e) {
e.printStackTrace();
}
return result;
}
/**
* Get cpu's current frequency
* unit:KHZ
* 获取cpu当前频率,单位KHZ
*
* @return
*/
public static List<Integer> getCpuCurFreq() {
List<Integer> results = new ArrayList<Integer>();
String freq = "";
FileReader fr = null;
try {
int cpuIndex = 0;
Integer lastFreq = 0;
while (true) {
File file = new File("/sys/devices/system/cpu/cpu" + cpuIndex + "/");
if (!file.exists()) {
break;
}
file = new File("/sys/devices/system/cpu/cpu" + cpuIndex + "/cpufreq/");
if (!file.exists()) {
lastFreq = 0;
results.add(0);
cpuIndex++;
continue;
}
file = new File("/sys/devices/system/cpu/cpu" + cpuIndex + "/cpufreq/scaling_cur_freq");
if (!file.exists()) {
results.add(lastFreq);
cpuIndex++;
continue;
}
fr = new FileReader(
"/sys/devices/system/cpu/cpu" + cpuIndex + "/cpufreq/scaling_cur_freq");
BufferedReader br = new BufferedReader(fr);
String text = br.readLine();
freq = text.trim();
lastFreq = Integer.valueOf(freq);
results.add(lastFreq);
fr.close();
cpuIndex++;
}
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
} finally {
if (fr != null) {
try {
fr.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
return results;
}
}
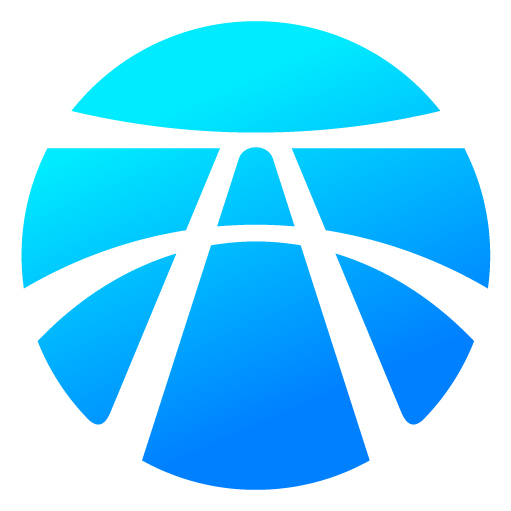
开放原子开发者工作坊旨在鼓励更多人参与开源活动,与志同道合的开发者们相互交流开发经验、分享开发心得、获取前沿技术趋势。工作坊有多种形式的开发者活动,如meetup、训练营等,主打技术交流,干货满满,真诚地邀请各位开发者共同参与!
更多推荐
所有评论(0)