双向链表源代码
list.c#include#include#include#include "list.h"LLIST *llist_create(int size){LLIST *newnode;newnode=malloc(sizeof(*newnode));if(newnode==NULL)ret
·
list.c
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
#include "list.h"
LLIST *llist_create(int size)
{
LLIST *newnode;
newnode=malloc(sizeof(*newnode));
if(newnode==NULL)
return NULL;
newnode->size=size;
newnode->head.data=NULL;
newnode->head.prev=&newnode->head;
newnode->head.next=&newnode->head;
return newnode;
}
int llist_insert(LLIST *ptr, void *data,int mode)
{
struct llist_node_st *newnode;
newnode=malloc(sizeof(*newnode));
if(newnode==NULL)
return -1;
newnode->data=malloc(ptr->size);//give list's data mem
if(newnode->data==NULL)
{
free(newnode);
return -1;
}
memcpy(newnode->data,data,ptr->size);
if(mode==LLIST_FORWARD)
{
newnode->prev=&ptr->head;
newnode->next=ptr->head.next;
}
else
if(mode==LLIST_BACKWARD)
{
newnode->prev=ptr->head.prev;
newnode->next=&ptr->head;
}
else
{
return -2;
}
newnode->prev->next=newnode;
newnode->next->prev=newnode;
return 0;
}
static struct llist_node_st *find_(LLIST *ptr,const void *key,llist_cmp *cmp)
{
struct llist_node_st *cur;
for(cur=ptr->head.next;cur!=&ptr->head;cur=cur->next)
{
if(cmp(key,cur->data)==0)
break;
}
return cur;
}
void *llist_find(LLIST *ptr,const void *key,llist_cmp *cmp)
{
return find_(ptr,key,cmp)->data;
}
void llist_travel(LLIST *ptr, llist_op *op)
{
struct llist_node_st *cur;
for(cur=ptr->head.next;cur!=&ptr->head;cur=cur->next)
{
op(cur->data);
}
}
int llist_delete(LLIST *ptr,const void *key ,llist_cmp *cmp)
{
struct llist_node_st *node;
node=find_(ptr,key,cmp);
if(node==&ptr->head)
return -1; //can not find
node->prev->next=node->next;
node->next->prev=node->prev;
free(node->data);
free(node);
return 0;
}
int llist_fetch(LLIST *ptr,const void *key,llist_cmp *cmp,void *data) //del the key and return the del's data
{
struct llist_node_st *node;
node=find_(ptr,key,cmp);
if(node==&ptr->head)
return -1; //can not find
memcpy(data,node->data,ptr->size);
node->prev->next=node->next;
node->next->prev=node->prev;
free(node->data);
free(node);
return 0;
}
void llist_destory(LLIST *ptr)
{
struct llist_node_st *cur;
void *next;
for(cur=ptr->head.next;cur!=&ptr->head;cur=next)
{
next=cur->next;
free(cur->data);
free(cur);
}
}
list.h
#ifndef MY_LIST_H_
#define MY_LIST_H_
#define LLIST_FORWARD 1
#define LLIST_BACKWARD 2
typedef void llist_op(const void *);
typedef int llist_cmp(const void *,const void *);
struct llist_node_st //link list node struct
{
void * data;
struct llist_node_st *prev,*next;
};
// header pointer
typedef struct
{
int size;
struct llist_node_st head;
}LLIST;
LLIST * llist_create(int size);
int llist_insert(LLIST *, void *,int);// shou bu cha ru, wei bu cha ru 2zhong fang shi
void *llist_find(LLIST *,const void *,llist_cmp *);//
int llist_delete(LLIST *,const void *,llist_cmp *);
int llist_fetch(LLIST *,const void *,llist_cmp *,void *); //del the key and return the del's data
void llist_travel(LLIST *, llist_op *);
void llist_destory(LLIST *);
#endif
main.c
#include <stdlib.h>
#include <stdio.h>
#include "list.h"
#define NAMESIZE 32
struct person
{
int id;
char name[NAMESIZE];
int age;
};
static void print_s(const void * data)
{
const struct person *d=(void *)data;
printf("%d %s %d\n",d->id,d->name,d->age);
}
static int id_cmp(const void * key,const void * data)
{
const int *k=key;
const struct person *d=data;
return (*k-d->id);
}
static int name_cmp(const void * key,const void * data)
{
const char *k=key;
const struct person *d=data;
return ( strcmp(k,d->name));
}
int main()
{
LLIST *handle;
char *name="person26";
int ret,i,id=3;
struct person tmp,*datap;
handle=llist_create(sizeof(struct person));
if(handle==NULL)
return -1;
for( i=0;i<6;i++)
{
tmp.id=i;
tmp.age=20+i;
snprintf(tmp.name,NAMESIZE,"person%d",i);
llist_insert(handle,&tmp,LLIST_BACKWARD);
//llist_insert(handle,&tmp,LLIST_BACKWARD);
}
llist_travel(handle,print_s);
printf("\n\n");
#if 0
datap=llist_find(handle,name,name_cmp);
//datap=llist_find(handle,&id,id_cmp);
if(datap!=NULL)
print_s(datap);
else
printf("can not find!\n");
llist_delete(handle,&id,id_cmp);
llist_travel(handle,print_s);
#endif
ret=llist_fetch(handle,&id,id_cmp,&tmp);
llist_travel(handle,print_s);
printf("\n\n");
if(ret==0)
{
print_s(&tmp);
}
llist_destory(handle);
return 0;
}
makefile
all:main
main:main.o list.o
clean:
rm -rf *.o main
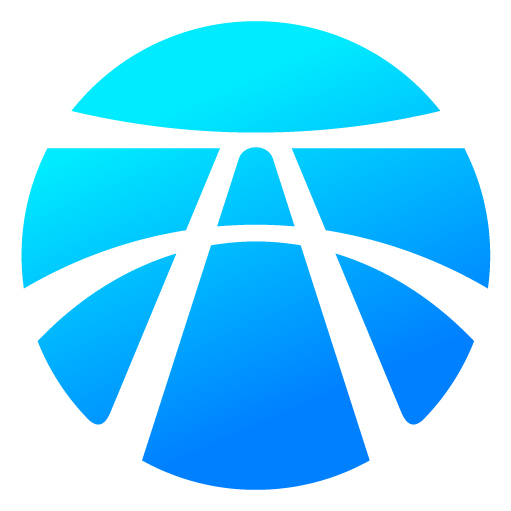
开放原子开发者工作坊旨在鼓励更多人参与开源活动,与志同道合的开发者们相互交流开发经验、分享开发心得、获取前沿技术趋势。工作坊有多种形式的开发者活动,如meetup、训练营等,主打技术交流,干货满满,真诚地邀请各位开发者共同参与!
更多推荐
所有评论(0)