MD5算法
MD5的全称是Message-Digest Algorithm 5(信息-摘要算法),是基于消息摘要原理的,消息摘要的基本特征就是很难根据摘要推算出消息报文。消息摘要(Message Digest)又称为数字摘要(Digital Digest)。它是一个唯一对应一个消息或文本的固定长度的值,它由一个单向Hash加密函数对消息进行作用而产生。如果消息在途中改变了,则接收者通过对收到消息的新产
MD5的全称是Message-Digest Algorithm 5(信息-摘要算法),是基于消息摘要原理的,消息摘要的基本特征就是很难根据摘要推算出消息报文。
消息摘要(Message Digest)又称为数字摘要(Digital Digest)。它是一个唯一对应一个消息或文本的固定长度的值,它由一个单向Hash加密函数对消息进行作用而产生。如果消息在途中改变了,则接收者通过对收到消息的新产生的摘要与原摘要比较,就可知道消息是否被改变了。因此消息摘要保证了消息的完整性。 消息摘要采用单向Hash 函数将需加密的明文"摘要"成一串128bit的密文,这一串密文亦称为数字指纹(Finger Print),它有固定的长度,且不同的明文摘要成密文,其结果总是不同的,而同样的明文其摘要必定一致。这样这串摘要便可成为验证明文是否是"真身"的"指纹"了。
MD5算法加密~16位、32位、64位。
MD5加密后所得到的通常是32位的编码,而在不少地方会用到16位的编码
它们有什么区别呢?
16位加密就是从32位MD5散列中把中间16位提取出来!
其实破解16位MD5散列要比破解32位MD5散列还慢
因为他多了一个步骤,就是使用32位加密后再把中间16位提取出来, 然后再进行对比
而破解32位的则不需要,加密后直接对比就可以了
admin 的加密代码:
16位加密:7a57a5a743894a0e
32位加密:21232f297a57a5a743894a0e4a801fc3
package net.test.util;
import java.io.UnsupportedEncodingException;
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
import sun.misc.BASE64Encoder;
public class MD5 {
/**Determine encrypt algorithm MD5*/
private static final String ALGORITHM_MD5 = "MD5";
/**UTF-8 Encoding*/
private static final String UTF_8 = "UTF-8";
/**
* MD5 16bit Encrypt Methods.
* @param readyEncryptStr ready encrypt string
* @return String encrypt result string
* @throws NoSuchAlgorithmException
* */
public static final String MD5_16bit(String readyEncryptStr) throws NoSuchAlgorithmException{
if(readyEncryptStr != null){
return MD5.MD5_32bit(readyEncryptStr).substring(8, 24);
}else{
return null;
}
}
/**
* MD5 32bit Encrypt Methods.
* @param readyEncryptStr ready encrypt string
* @return String encrypt result string
* @throws NoSuchAlgorithmException
* */
public static final String MD5_32bit(String readyEncryptStr) throws NoSuchAlgorithmException{
if(readyEncryptStr != null){
//Get MD5 digest algorithm's MessageDigest's instance.
MessageDigest md = MessageDigest.getInstance(ALGORITHM_MD5);
//Use specified byte update digest.
md.update(readyEncryptStr.getBytes());
//Get cipher text
byte [] b = md.digest();
//The cipher text converted to hexadecimal string
StringBuilder su = new StringBuilder();
//byte array switch hexadecimal number.
for(int offset = 0,bLen = b.length; offset < bLen; offset++){
String haxHex = Integer.toHexString(b[offset] & 0xFF);
if(haxHex.length() < 2){
su.append("0");
}
su.append(haxHex);
}
return su.toString();
}else{
return null;
}
}
/**
* MD5 32bit Encrypt Methods.
* @param readyEncryptStr ready encrypt string
* @return String encrypt result string
* @throws NoSuchAlgorithmException
* */
public static final String MD5_32bit1(String readyEncryptStr) throws NoSuchAlgorithmException{
if(readyEncryptStr != null){
//The cipher text converted to hexadecimal string
StringBuilder su = new StringBuilder();
//Get MD5 digest algorithm's MessageDigest's instance.
MessageDigest md = MessageDigest.getInstance(ALGORITHM_MD5);
byte [] b = md.digest(readyEncryptStr.getBytes());
int temp = 0;
//byte array switch hexadecimal number.
for(int offset = 0,bLen = b.length; offset < bLen; offset++){
temp = b[offset];
if(temp < 0){
temp += 256;
}
int d1 = temp / 16;
int d2 = temp % 16;
su.append(Integer.toHexString(d1) + Integer.toHexString(d2)) ;
}
return su.toString();
}else{
return null;
}
}
/**
* MD5 32bit Encrypt Methods.
* @param readyEncryptStr ready encrypt string
* @return String encrypt result string
* @throws NoSuchAlgorithmException
* */
public static final String MD5_32bit2(String readyEncryptStr) throws NoSuchAlgorithmException{
if(readyEncryptStr != null){
//The cipher text converted to hexadecimal string
StringBuilder su = new StringBuilder();
//Get MD5 digest algorithm's MessageDigest's instance.
MessageDigest md = MessageDigest.getInstance(ALGORITHM_MD5);
//Use specified byte update digest.
md.update(readyEncryptStr.getBytes());
byte [] b = md.digest();
int temp = 0;
//byte array switch hexadecimal number.
for(int offset = 0,bLen = b.length; offset < bLen; offset++){
temp = b[offset];
if(temp < 0){
temp += 256;
}
if(temp < 16){
su.append("0");
}
su.append(Integer.toHexString(temp));
}
return su.toString();
}else{
return null;
}
}
/**
* MD5 16bit Encrypt Methods.
* @param readyEncryptStr ready encrypt string
* @return String encrypt result string
* @throws NoSuchAlgorithmException
* @throws UnsupportedEncodingException
* */
public static final String MD5_64bit(String readyEncryptStr) throws NoSuchAlgorithmException, UnsupportedEncodingException{
MessageDigest md = MessageDigest.getInstance(ALGORITHM_MD5);
BASE64Encoder base64Encoder = new BASE64Encoder();
return base64Encoder.encode(md.digest(readyEncryptStr.getBytes(UTF_8)));
}
public static void main(String[] args) {
try {
String s = "admin";
String md516 = MD5.MD5_16bit(s);
System.out.println("16bit-md5:\n" + md516); //
// String md532 = MD5.MD5_32bit(s);
// String md5321 = MD5.MD5_32bit1(s);
// String md5322 = MD5.MD5_32bit2(s);
// System.out.println("32bit-md5:"); //5d052f1e32af4e4ac2544a5fc2a9b992
// System.out.println("1: " + md532);
// System.out.println("2: " + md5321);
// System.out.println("3: " + md5322);
// String md564 = MD5.MD5_64bit(s);
// System.out.println("64bit-md5:\n" + md564); //
} catch (Exception e) {
e.printStackTrace();
}
}
}
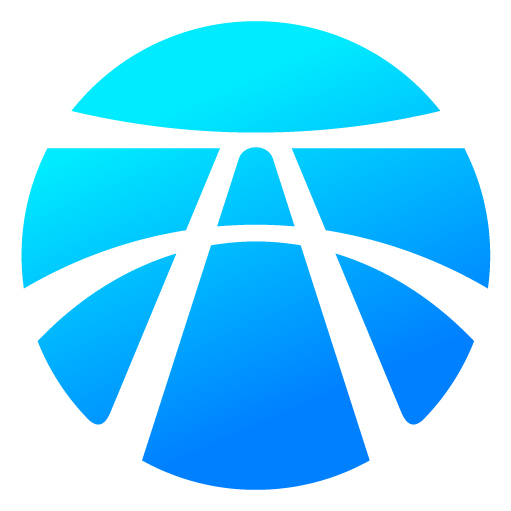
开放原子开发者工作坊旨在鼓励更多人参与开源活动,与志同道合的开发者们相互交流开发经验、分享开发心得、获取前沿技术趋势。工作坊有多种形式的开发者活动,如meetup、训练营等,主打技术交流,干货满满,真诚地邀请各位开发者共同参与!
更多推荐
所有评论(0)