ADO.NET作业
1).JOB表格2). 源代码如下: using System;using System.Drawing;using System.Collections;using System.ComponentModel;using System.Windows.Forms;using System.Data;using System.Data.SqlClient;namespace Windows
1).JOB表格
2). 源代码如下:
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
using System.Data.SqlClient;
namespace WindowsApplication2
{
/// <summary>
/// Form1 的摘要说明。
/// </summary>
public class Form1 : System.Windows.Forms.Form
{
private DataSet myDs;
private BindingManagerBase myBind;
private System.Windows.Forms.Label lname;
private System.Windows.Forms.Label Job_ID;
private System.Windows.Forms.Button lastrec;
private System.Windows.Forms.Button fristrec;
private System.Windows.Forms.Button addrec;
private System.Windows.Forms.Button nextrec;
private System.Windows.Forms.Button delrec;
private System.Windows.Forms.Button prevrec;
private System.Windows.Forms.TextBox jid;
private System.Windows.Forms.TextBox jname;
private System.Windows.Forms.TextBox jtitle;
private System.Windows.Forms.TextBox jtel;
private System.Windows.Forms.Label ltitle;
private System.Windows.Forms.Label ltel;
private System.Windows.Forms.Label tage;
private System.Windows.Forms.TextBox jage;
private System.Windows.Forms.Button cadd;
private System.Windows.Forms.Label MCAD;
private System.Windows.Forms.TextBox uid;
private System.Windows.Forms.Button modtrec;
private System.Windows.Forms.Button cno;
/// <summary>
/// 必需的设计器变量。
/// </summary>
private System.ComponentModel.Container components = null;
public Form1()
{
//
// Windows 窗体设计器支持所必需的
//
dataShow();
InitializeComponent();
dataBind();
//
// TODO: 在 InitializeComponent 调用后添加任何构造函数代码
//
}
/// <summary>
/// 清理所有正在使用的资源。
/// </summary>
protected override void Dispose( bool disposing )
{
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
private void dataShow()
{
string strConn = "Initial Catalog = NorthWind; Data Source = 127.0.0.1; UID=sa; PWD=";
SqlConnection conn = new SqlConnection();
conn.ConnectionString = strConn;
SqlCommand selcmd = new SqlCommand();
selcmd.CommandText = "select * from job";
selcmd.Connection = conn;
try
{
conn.Open();
SqlDataAdapter myda = new SqlDataAdapter();
myda.SelectCommand = selcmd;
myDs = new DataSet();
myda.Fill(myDs, "job");
conn.Close();
}
catch ( Exception e )
{
MessageBox.Show("连接错误!" + e.ToString());
}
}
#region Windows 窗体设计器生成的代码
/// <summary>
/// 设计器支持所需的方法 - 不要使用代码编辑器修改
/// 此方法的内容。
/// </summary>
private void InitializeComponent()
{
this.MCAD = new System.Windows.Forms.Label();
this.jid = new System.Windows.Forms.TextBox();
this.jname = new System.Windows.Forms.TextBox();
this.jtitle = new System.Windows.Forms.TextBox();
this.jtel = new System.Windows.Forms.TextBox();
this.Job_ID = new System.Windows.Forms.Label();
this.lname = new System.Windows.Forms.Label();
this.ltitle = new System.Windows.Forms.Label();
this.ltel = new System.Windows.Forms.Label();
this.lastrec = new System.Windows.Forms.Button();
this.fristrec = new System.Windows.Forms.Button();
this.modtrec = new System.Windows.Forms.Button();
this.addrec = new System.Windows.Forms.Button();
this.nextrec = new System.Windows.Forms.Button();
this.delrec = new System.Windows.Forms.Button();
this.prevrec = new System.Windows.Forms.Button();
this.jage = new System.Windows.Forms.TextBox();
this.tage = new System.Windows.Forms.Label();
this.cadd = new System.Windows.Forms.Button();
this.uid = new System.Windows.Forms.TextBox();
this.cno = new System.Windows.Forms.Button();
this.SuspendLayout();
//
// MCAD
//
this.MCAD.Font = new System.Drawing.Font("宋体", 12F, System.Drawing.FontStyle.Bold, System.Drawing.GraphicsUnit.Point, ((System.Byte)(134)));
this.MCAD.ForeColor = System.Drawing.Color.Green;
this.MCAD.Location = new System.Drawing.Point(80, 24);
this.MCAD.Name = "MCAD";
this.MCAD.Size = new System.Drawing.Size(264, 24);
this.MCAD.TabIndex = 0;
this.MCAD.Text = "MCAD课程ADO.NET课后作业";
this.MCAD.TextAlign = System.Drawing.ContentAlignment.MiddleCenter;
//
// jid
//
this.jid.Location = new System.Drawing.Point(216, 72);
this.jid.Name = "jid";
this.jid.TabIndex = 1;
this.jid.Text = "";
//
// jname
//
this.jname.Location = new System.Drawing.Point(216, 112);
this.jname.Name = "jname";
this.jname.TabIndex = 2;
this.jname.Text = "";
//
// jtitle
//
this.jtitle.Location = new System.Drawing.Point(216, 160);
this.jtitle.Name = "jtitle";
this.jtitle.TabIndex = 3;
this.jtitle.Text = "";
//
// jtel
//
this.jtel.Location = new System.Drawing.Point(216, 208);
this.jtel.Name = "jtel";
this.jtel.TabIndex = 4;
this.jtel.Text = "";
//
// Job_ID
//
this.Job_ID.Location = new System.Drawing.Point(80, 72);
this.Job_ID.Name = "Job_ID";
this.Job_ID.TabIndex = 5;
this.Job_ID.Text = "Job ID:";
this.Job_ID.TextAlign = System.Drawing.ContentAlignment.MiddleRight;
//
// lname
//
this.lname.Location = new System.Drawing.Point(80, 112);
this.lname.Name = "lname";
this.lname.TabIndex = 5;
this.lname.Text = "Job Name:";
this.lname.TextAlign = System.Drawing.ContentAlignment.MiddleRight;
//
// ltitle
//
this.ltitle.Location = new System.Drawing.Point(80, 160);
this.ltitle.Name = "ltitle";
this.ltitle.TabIndex = 5;
this.ltitle.Text = "Job Title;";
this.ltitle.TextAlign = System.Drawing.ContentAlignment.MiddleRight;
//
// ltel
//
this.ltel.Location = new System.Drawing.Point(80, 208);
this.ltel.Name = "ltel";
this.ltel.TabIndex = 5;
this.ltel.Text = "Job Telphone:";
this.ltel.TextAlign = System.Drawing.ContentAlignment.MiddleRight;
//
// lastrec
//
this.lastrec.ForeColor = System.Drawing.SystemColors.HotTrack;
this.lastrec.Location = new System.Drawing.Point(336, 288);
this.lastrec.Name = "lastrec";
this.lastrec.TabIndex = 7;
this.lastrec.Text = "Last";
this.lastrec.Click += new System.EventHandler(this.lastrec_Click);
//
// fristrec
//
this.fristrec.ForeColor = System.Drawing.SystemColors.HotTrack;
this.fristrec.Location = new System.Drawing.Point(24, 288);
this.fristrec.Name = "fristrec";
this.fristrec.TabIndex = 6;
this.fristrec.Text = "Frist";
this.fristrec.Click += new System.EventHandler(this.fristrec_Click);
//
// modtrec
//
this.modtrec.ForeColor = System.Drawing.SystemColors.HotTrack;
this.modtrec.Location = new System.Drawing.Point(176, 336);
this.modtrec.Name = "modtrec";
this.modtrec.TabIndex = 8;
this.modtrec.Text = "Modify";
this.modtrec.Click += new System.EventHandler(this.editrec_Click);
//
// addrec
//
this.addrec.ForeColor = System.Drawing.SystemColors.HotTrack;
this.addrec.Location = new System.Drawing.Point(56, 336);
this.addrec.Name = "addrec";
this.addrec.TabIndex = 9;
this.addrec.Text = "Add";
this.addrec.Click += new System.EventHandler(this.addrec_Click);
//
// nextrec
//
this.nextrec.ForeColor = System.Drawing.SystemColors.HotTrack;
this.nextrec.Location = new System.Drawing.Point(232, 288);
this.nextrec.Name = "nextrec";
this.nextrec.TabIndex = 7;
this.nextrec.Text = "Next";
this.nextrec.Click += new System.EventHandler(this.nextrec_Click);
//
// delrec
//
this.delrec.ForeColor = System.Drawing.SystemColors.HotTrack;
this.delrec.Location = new System.Drawing.Point(296, 336);
this.delrec.Name = "delrec";
this.delrec.TabIndex = 8;
this.delrec.Text = "Delete";
this.delrec.Click += new System.EventHandler(this.delrec_Click);
//
// prevrec
//
this.prevrec.ForeColor = System.Drawing.SystemColors.HotTrack;
this.prevrec.Location = new System.Drawing.Point(128, 288);
this.prevrec.Name = "prevrec";
this.prevrec.TabIndex = 7;
this.prevrec.Text = "Previous";
this.prevrec.Click += new System.EventHandler(this.prevrec_Click);
//
// jage
//
this.jage.Location = new System.Drawing.Point(216, 248);
this.jage.Name = "jage";
this.jage.TabIndex = 4;
this.jage.Text = "";
//
// tage
//
this.tage.Location = new System.Drawing.Point(80, 248);
this.tage.Name = "tage";
this.tage.TabIndex = 5;
this.tage.Text = "Job Age:";
this.tage.TextAlign = System.Drawing.ContentAlignment.MiddleRight;
//
// cadd
//
this.cadd.Location = new System.Drawing.Point(336, 248);
this.cadd.Name = "cadd";
this.cadd.Size = new System.Drawing.Size(32, 23);
this.cadd.TabIndex = 10;
this.cadd.Text = "Yes";
this.cadd.Visible = false;
this.cadd.Click += new System.EventHandler(this.cadd_Click);
//
// uid
//
this.uid.Location = new System.Drawing.Point(216, 48);
this.uid.Name = "uid";
this.uid.Size = new System.Drawing.Size(0, 21);
this.uid.TabIndex = 11;
this.uid.Text = "";
//
// cno
//
this.cno.Location = new System.Drawing.Point(376, 248);
this.cno.Name = "cno";
this.cno.Size = new System.Drawing.Size(32, 23);
this.cno.TabIndex = 10;
this.cno.Text = "No";
this.cno.Visible = false;
this.cno.Click += new System.EventHandler(this.cno_Click);
//
// Form1
//
this.AutoScaleBaseSize = new System.Drawing.Size(6, 14);
this.ClientSize = new System.Drawing.Size(452, 386);
this.Controls.Add(this.uid);
this.Controls.Add(this.cadd);
this.Controls.Add(this.addrec);
this.Controls.Add(this.modtrec);
this.Controls.Add(this.prevrec);
this.Controls.Add(this.fristrec);
this.Controls.Add(this.Job_ID);
this.Controls.Add(this.jtel);
this.Controls.Add(this.jtitle);
this.Controls.Add(this.jname);
this.Controls.Add(this.jid);
this.Controls.Add(this.MCAD);
this.Controls.Add(this.lname);
this.Controls.Add(this.ltitle);
this.Controls.Add(this.ltel);
this.Controls.Add(this.nextrec);
this.Controls.Add(this.lastrec);
this.Controls.Add(this.delrec);
this.Controls.Add(this.jage);
this.Controls.Add(this.tage);
this.Controls.Add(this.cno);
this.ForeColor = System.Drawing.SystemColors.HotTrack;
this.Name = "Form1";
this.Text = "我的第一个ADO.NET数据库应用程序";
this.ResumeLayout(false);
}
#endregion
private void dataBind()
{
uid.DataBindings.Add("Text", myDs,"job.uid");
jid.DataBindings.Add("Text", myDs,"job.id");
jname.DataBindings.Add("Text", myDs,"job.name");
jtitle.DataBindings.Add("Text", myDs,"job.title");
jtel.DataBindings.Add("Text", myDs,"job.tel");
jage.DataBindings.Add("Text", myDs,"job.age");
//把对象DataSet和"books"数据表绑定到此myBind对象
myBind= this.BindingContext [myDs, "job"];
}
/// <summary>
/// 应用程序的主入口点。
/// </summary>
[STAThread]
static void Main()
{
Application.Run(new Form1());
}
private void fristrec_Click(object sender, System.EventArgs e)
{
myBind.Position = 0;
}
private void prevrec_Click(object sender, System.EventArgs e)
{
if(myBind.Position == 0)
MessageBox.Show("已经是第一条记录!");
else
myBind.Position -=1;
}
private void nextrec_Click(object sender, System.EventArgs e)
{
if(myBind.Position == myBind.Count-1 )
MessageBox.Show("已经到了最后一条记录!");
else
myBind.Position +=1;
}
private void lastrec_Click(object sender, System.EventArgs e)
{
myBind.Position =myBind.Count-1;
}
public void addrec_Click(object sender, System.EventArgs e)
{
myBind.AddNew();
cadd.Visible =true;
cno.Visible = true;
fristrec.Enabled = false;
prevrec.Enabled = false;
nextrec.Enabled = false;
lastrec.Enabled = false;
modtrec.Enabled = false;
delrec.Enabled = false;
}
private void editrec_Click(object sender, System.EventArgs e)
{
int ie= myBind.Position;
try
{
string strconn = "Initial Catalog = NorthWind; Data Source= 127.0.0.1; Uid= sa; PWD=";
SqlConnection conn = new SqlConnection();
conn.ConnectionString = strconn;
conn.Open();
string strUpdt = "update job set id ='" + jid.Text +"',name ='" + jname.Text +"',title ='" + jtitle.Text +"',tel ='" + jtel.Text+"',age ='" + jage.Text +"' where uid='"+ uid.Text+"'";
SqlCommand updt = new SqlCommand();
updt.CommandText = strUpdt;
updt.Connection = conn;
updt.ExecuteNonQuery();
myDs.AcceptChanges();
updt.Dispose();
conn.Close();
}
catch(Exception eu)
{
MessageBox.Show(eu.Message);
}
myBind.Position = ie;
}
private void delrec_Click(object sender, System.EventArgs e)
{
try
{
string strconn = "Initial Catalog = NorthWind; Data Source= 127.0.0.1; Uid= sa; PWD=";
SqlConnection conn = new SqlConnection();
conn.ConnectionString = strconn;
conn.Open();
string strDel = "delete from job where id="+ jid.Text;
SqlCommand del = new SqlCommand();
del.CommandText = strDel;
del.Connection = conn;
del.ExecuteNonQuery();
myDs.Tables["job"].Rows[myBind.Position].Delete();
myDs.AcceptChanges();
del.Dispose();
conn.Close();
}
catch (Exception ed)
{
MessageBox.Show(ed.Message);
}
}
private void cadd_Click(object sender, System.EventArgs e)
{
fristrec.Enabled = true;
prevrec.Enabled = true;
nextrec.Enabled = true;
lastrec.Enabled = true;
modtrec.Enabled = true;
delrec.Enabled = true;
int i= myBind.Position;
try
{
DataRow r=myDs.Tables[0].NewRow();
r["id"]=jid.Text;
r["name"]=jname.Text;
r["title"]=jtitle.Text;
r["tel"]=jtel.Text;
r["age"]=jage.Text;
string strconn = "Initial Catalog = NorthWind; Data Source= 127.0.0.1; Uid= sa; PWD=";
SqlConnection conn = new SqlConnection();
conn.ConnectionString = strconn;
conn.Open();
string strAdd = "insert into job(id,name,title,tel,age) values('"+r["id"]+"','"+r["name"]+"','"+r["title"]+"','"+r["tel"]+"','"+r["age"]+"')";
SqlCommand add = new SqlCommand();
add.CommandText = strAdd;
add.Connection = conn;
add.ExecuteNonQuery();
myDs.AcceptChanges();
add.Dispose();
conn.Close();
}
catch (Exception) //详细信息可用到e
{
MessageBox.Show("没有新增成功");
myBind.RemoveAt(myBind.Count-1);
}
finally
{
myBind.Position = i;
cadd.Visible=false;
cno.Visible = false;
}
}
private void cno_Click(object sender, System.EventArgs e)
{
fristrec.Enabled = true;
prevrec.Enabled = true;
nextrec.Enabled = true;
lastrec.Enabled = true;
modtrec.Enabled = true;
delrec.Enabled = true;
cadd.Visible=false;
cno.Visible=false;
myBind.RemoveAt(myBind.Count-1);
myBind.Position =myBind.Count-1;
}
}
}
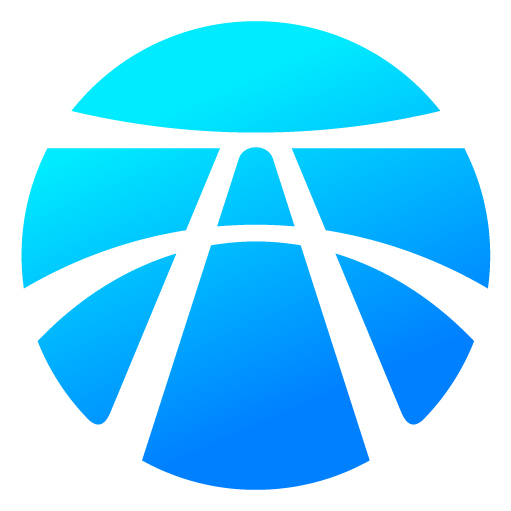
开放原子开发者工作坊旨在鼓励更多人参与开源活动,与志同道合的开发者们相互交流开发经验、分享开发心得、获取前沿技术趋势。工作坊有多种形式的开发者活动,如meetup、训练营等,主打技术交流,干货满满,真诚地邀请各位开发者共同参与!
更多推荐
所有评论(0)