nodejs 微信开发相关,抓取相关
nodejs开源项目: 1.nodejs中间件,https://github.com/node-webot/wechat 2.微信公众平台开发(76) 无高级接口账号获取用户基本信息 www.cnblogs.com/txw1958/p/weixin76-user-info.html 关于微信开放平台的申请过程,请查看官方地址:http://mp
真正项目中用到的模块:
1.https://github.com/scottkiss/nodegrass nodejs的抓取插件,解决了gbk编码问题
2.https://github.com/LearnBoost/mongoose nodejs的monggodb的orm框架。
3.http://olado.github.io/doT/ 项目中前端和后端都是用的一个前端数据绑定模板
4.我们参考的是 通过《nodeclub项目源码来讲解如何做一个nodejs + express + mongodb项目》
http://segmentfault.com/blog/6174/1190000000478457
.网络资源
Heroku 支持部署nodejs MongoHQ是一个托管MongoDB的第三方平台
nodejs开源项目:
1.nodejs中间件,https://github.com/node-webot/wechat
2.微信公众平台开发(76) 无高级接口账号获取用户基本信息 www.cnblogs.com/txw1958/p/weixin76-user-info.html
3.微信开发相关 http://gexia.com/
4.微信自定义菜单 http://www.cnblogs.com/yank/p/3418194.html
5.nodejs jquery库, https://github.com/cheeriojs/cheerio
6.nodejs web抓取,解决中文问题 https://github.com/scottkiss/nodegrass
7.nodejs 爬虫 http://blog.sina.com.cn/s/blog_816bcbe70101f9vw.html
8. 基于NodeJs和mongoose的CommonDao http://www.oschina.net/code/snippet_698737_17103
9.抓取 http://www.oschina.net/code/piece_full?code=26611&piece=44432#44432
关于微信开放平台的申请过程,请查看官方地址:http://mp.weixin.qq.com/cgi-bin/indexpage?t=wxm-callbackapi-doc&lang=zh_CN
现在贴出来如何处理消息的,XML的解析使用了node-xml这个模块 ,使用命令:npm install node-xml 安装.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
|
var
crypto=require(
"crypto"
);
var
xml=require(
"./node-xml/lib/node-xml.js"
);
var
messageSender=require(
"./messageSender.js"
);
function
isLegel(signature,timestamp,nonce,token){
var
array=
new
Array();
array[0]=timestamp;
array[1]=nonce;
array[2]=token;
array.sort();
var
hasher=crypto.createHash(
"sha1"
);
var
msg=array[0]+array[1]+array[2];
hasher.update(msg);
var
msg=hasher.digest(
'hex'
);
if
(msg==signature){
return
true
;
}
else
{
return
false
;
}
}
function
processMessage(data,response){
var
ToUserName=
""
;
var
FromUserName=
""
;
var
CreateTime=
""
;
var
MsgType=
""
;
var
Content=
""
;
var
Location_X=
""
;
var
Location_Y=
""
;
var
Scale=1;
var
Label=
""
;
var
PicUrl=
""
;
var
FuncFlag=
""
;
var
tempName=
""
;
var
parse=
new
xml.SaxParser(
function
(cb){
cb.onStartElementNS(
function
(elem,attra,prefix,uri,namespaces){
tempName=elem;
});
cb.onCharacters(
function
(chars){
chars=chars.replace(/(^\s*)|(\s*$)/g,
""
);
if
(tempName==
"CreateTime"
){
CreateTime=chars;
}
else
if
(tempName==
"Location_X"
){
Location_X=cdata;
}
else
if
(tempName==
"Location_Y"
){
Location_Y=cdata;
}
else
if
(tempName==
"Scale"
){
Scale=cdata;
}
});
cb.onCdata(
function
(cdata){
if
(tempName==
"ToUserName"
){
ToUserName=cdata;
}
else
if
(tempName==
"FromUserName"
){
FromUserName=cdata;
}
else
if
(tempName==
"MsgType"
){
MsgType=cdata;
}
else
if
(tempName==
"Content"
){
Content=cdata;
}
else
if
(tempName==
"PicUrl"
){
PicUrl=cdata;
}
else
if
(tempName==
"Label"
){
Label=cdata;
}
console.log(
"cdata:"
+cdata);
});
cb.onEndElementNS(
function
(elem,prefix,uri){
tempName=
""
;
});
cb.onEndDocument(
function
(){
console.log(
"onEndDocument"
);
tempName=
""
;
var
date=
new
Date();
var
yy=date.getYear();
var
MM=date.getMonth() + 1;
var
dd=date.getDay();
var
hh=date.getHours();
var
mm=date.getMinutes();
var
ss=date.getSeconds();
var
sss=date.getMilliseconds();
var
result=Date.UTC(yy,MM,dd,hh,mm,ss,sss);
var
msg=
""
;
if
(MsgType==
"text"
){
msg=
"谢谢关注,你说的是:"
+Content;
}
else
if
(MsgType=
"location"
){
msg=
"你所在的位置: 经度:"
+Location_X+
"纬度:"
+Location_Y;
}
else
if
(MsgType=
"image"
){
msg=
"你发的图片是:"
+PicUrl;
}
messageSender.sendTextMessage(FromUserName,ToUserName,CreateTime,msg,FuncFlag,response);
});
});
parse.parseString(data);
}
module.exports.isLegel=isLegel;
module.exports.processMessage=processMessage;
|
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
|
var
app=require(
"express"
).createServer();
var
wx=require(
'./lib/wx'
);
var
fs=require(
"fs"
);
app.get(
'/'
,
function
(req,response){
fs.readFile(
"./qrCode.jpg"
,
"binary"
,
function
(err,file){
if
(err){
response.writeHead(500,{
"Content-Type"
:
"text/plain"
});
response.write(err+
"\n"
);
}
else
{
response.writeHead(200,{
"Content-Type"
:
"image/jpg"
});
response.write(file,
"binary"
);
}
response.end();
});
});
app.get(
'/wx'
,
function
(req,res){
var
signature=req.query.signature;
var
timestamp=req.query.timestamp;
var
nonce=req.query.nonce;
var
echostr=req.query.echostr;
var
check=
false
;
check=wx.isLegel(signature,timestamp,nonce,token);
//替换成你的token
if
(check){
res.write(echostr);
}
else
{
res.write(
"error data"
);
}
res.end();
});
app.post(
'/wx'
,
function
(req,res){
var
response=res;
var
formData=
""
;
req.on(
"data"
,
function
(data){
formData+=data;
});
req.on(
"end"
,
function
(){
wx.processMessage(formData,response);
});
});
app.listen(3000);
<div>
</div>
|
要是想玩一下的话,可以打开关注,添加我这个账号:http://wxtest.cloudfoundry.com/
或者用微信扫描这个二维码:
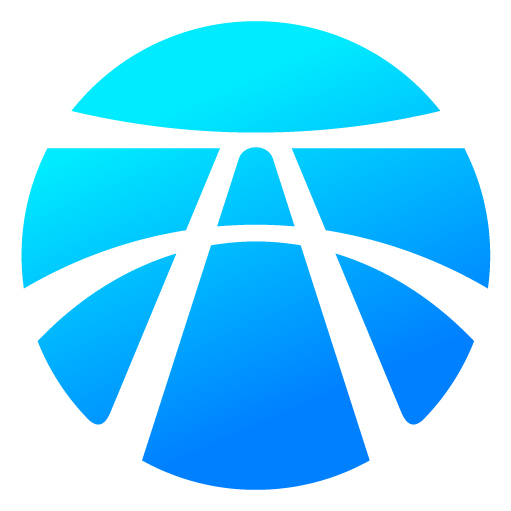
开放原子开发者工作坊旨在鼓励更多人参与开源活动,与志同道合的开发者们相互交流开发经验、分享开发心得、获取前沿技术趋势。工作坊有多种形式的开发者活动,如meetup、训练营等,主打技术交流,干货满满,真诚地邀请各位开发者共同参与!
更多推荐
所有评论(0)