RT-Threaad的。drive.c
/** File: device.c* This file is part of RT-Thread RTOS* COPYRIGHT (C) 2006 - 2012, RT-Thread Development Team** The license and distribution terms for this file may be* found in the
·
/*
* File : device.c
* This file is part of RT-Thread RTOS
* COPYRIGHT (C) 2006 - 2012, RT-Thread Development Team
*
* The license and distribution terms for this file may be
* found in the file LICENSE in this distribution or at
* http://www.rt-thread.org/license/LICENSE
*
* Change Logs:
* Date Author Notes
* 2007-01-21 Bernard the first version
* 2010-05-04 Bernard add rt_device_init implementation
* 2012-10-20 Bernard add device check in register function,
* provided by Rob <rdent@iinet.net.au>
* 2012-12-25 Bernard return RT_EOK if the device interface not exist.
*/
#include <rtthread.h>
#ifdef RT_USING_DEVICE
/*
*********************************************************************************************************
* 函 数 名: rt_device_register
* 功能说明: 注册设备
* 形 参: dev-设备类型 name-设备名称 flag-设备标识
* 返 回 值: RT_ERROR-加入失败 RT_EOK-加入成功
*********************************************************************************************************
*/
rt_err_t rt_device_register(rt_device_t dev,const char *name,rt_uint16_t flags)
{
if (dev == RT_NULL)
{
return -RT_ERROR;
}
//查找设备是否存在
if (rt_device_find(name) != RT_NULL)
{
return -RT_ERROR;
}
//初始化对象结构体
rt_object_init(&(dev->parent), RT_Object_Class_Device, name);
dev->flag = flags;
return RT_EOK;
}
RTM_EXPORT(rt_device_register);
/*
*********************************************************************************************************
* 函 数 名: rt_device_unregister
* 功能说明: 取消设备
* 形 参: dev-设备类型
* 返 回 值: RT_ERROR-加入失败 RT_EOK-加入成功
*********************************************************************************************************
*/
rt_err_t rt_device_unregister(rt_device_t dev)
{
RT_ASSERT(dev != RT_NULL);
rt_object_detach(&(dev->parent));
return RT_EOK;
}
RTM_EXPORT(rt_device_unregister);
/*
*********************************************************************************************************
* 函 数 名: rt_device_init_all
* 功能说明: 初始化所有设备
* 形 参: 无
* 返 回 值: RT_EOK-初始化完成
*********************************************************************************************************
*/
rt_err_t rt_device_init_all(void)
{
struct rt_device *device;
struct rt_list_node *node;
struct rt_object_information *information;
register rt_err_t result;
extern struct rt_object_information rt_object_container[];
information = &rt_object_container[RT_Object_Class_Device];
//遍历每一个已注册的设备
for (node = information->object_list.next; node != &(information->object_list); node = node->next)
{
rt_err_t (*init)(rt_device_t dev);
device = (struct rt_device *)rt_list_entry(node,struct rt_object,list);
//获取设备初始化句柄
init = device->init;
if (init != RT_NULL && !(device->flag & RT_DEVICE_FLAG_ACTIVATED))
{
//初始化设备
result = init(device);
if (result != RT_EOK)
{
rt_kprintf("To initialize device:%s failed. The error code is %d\n",device->parent.name, result);
}
else
{
//设备已激活
device->flag |= RT_DEVICE_FLAG_ACTIVATED;
}
}
}
return RT_EOK;
}
/*
*********************************************************************************************************
* 函 数 名: rt_device_find
* 功能说明: 通过设备名称,查找设备
* 形 参: name-设备名称
* 返 回 值: 设备类型结构体 RT_NULL-查找失败
*********************************************************************************************************
*/
rt_device_t rt_device_find(const char *name)
{
struct rt_object *object;
struct rt_list_node *node;
struct rt_object_information *information;
extern struct rt_object_information rt_object_container[];
//进入临界区
if (rt_thread_self() != RT_NULL)
{
rt_enter_critical();
}
//查找设备类型结构体
information = &rt_object_container[RT_Object_Class_Device];
for (node = information->object_list.next; node != &(information->object_list); node = node->next)
{
object = rt_list_entry(node, struct rt_object, list);
if (rt_strncmp(object->name, name, RT_NAME_MAX) == 0)
{
//退出临界区
if (rt_thread_self() != RT_NULL)
{
rt_exit_critical();
}
return (rt_device_t)object;
}
}
//退出临界区
if (rt_thread_self() != RT_NULL)
{
rt_exit_critical();
}
//未查找到设备
return RT_NULL;
}
RTM_EXPORT(rt_device_find);
/*
*********************************************************************************************************
* 函 数 名: rt_device_init
* 功能说明: 初始化设备
* 形 参: dev-设备
* 返 回 值: RT_EOK-初始化完成
*********************************************************************************************************
*/
rt_err_t rt_device_init(rt_device_t dev)
{
rt_err_t result = RT_EOK;
RT_ASSERT(dev != RT_NULL);
//获取设备初始化句柄
if (dev->init != RT_NULL)
{
if (!(dev->flag & RT_DEVICE_FLAG_ACTIVATED))
{
result = dev->init(dev);
if (result != RT_EOK)
{
rt_kprintf("To initialize device:%s failed. The error code is %d\n",dev->parent.name, result);
}
else
{
dev->flag |= RT_DEVICE_FLAG_ACTIVATED;
}
}
}
return result;
}
/*
*********************************************************************************************************
* 函 数 名: rt_device_open
* 功能说明: 打开设备
* 形 参: dev-设备 oflag-开启标识
* 返 回 值: 打开状态
*********************************************************************************************************
*/
rt_err_t rt_device_open(rt_device_t dev, rt_uint16_t oflag)
{
rt_err_t result = RT_EOK;
RT_ASSERT(dev != RT_NULL);
//若设备未初始化,则先初始化设备
if (!(dev->flag & RT_DEVICE_FLAG_ACTIVATED))
{
if (dev->init != RT_NULL)
{
result = dev->init(dev);
if (result != RT_EOK)
{
rt_kprintf("To initialize device:%s failed. The error code is %d\n",dev->parent.name, result);
return result;
}
}
dev->flag |= RT_DEVICE_FLAG_ACTIVATED;
}
//若设备是独一无二的,且已经打开了,则返回忙标识
if ((dev->flag & RT_DEVICE_FLAG_STANDALONE) && (dev->open_flag & RT_DEVICE_OFLAG_OPEN))
{
return -RT_EBUSY;
}
//调用设备打开接口
if (dev->open != RT_NULL)
{
result = dev->open(dev, oflag);
}
//设备打开成功标识
if (result == RT_EOK || result == -RT_ENOSYS)
{
dev->open_flag = oflag | RT_DEVICE_OFLAG_OPEN;
}
return result;
}
RTM_EXPORT(rt_device_open);
/*
*********************************************************************************************************
* 函 数 名: rt_device_close
* 功能说明: 关闭设备
* 形 参: dev-设备
* 返 回 值: 关闭状态
*********************************************************************************************************
*/
rt_err_t rt_device_close(rt_device_t dev)
{
rt_err_t result = RT_EOK;
RT_ASSERT(dev != RT_NULL);
//调用设备关闭接口
if (dev->close != RT_NULL)
{
result = dev->close(dev);
}
//设备打开标识为关闭
if (result == RT_EOK || result == -RT_ENOSYS)
{
dev->open_flag = RT_DEVICE_OFLAG_CLOSE;
}
return result;
}
RTM_EXPORT(rt_device_close);
/*
*********************************************************************************************************
* 函 数 名: rt_device_read
* 功能说明: 读取设备数据
* 形 参: dev-设备 pos-读取位置 buffer-数据缓存 size-数据大小
* 返 回 值: 读取状态
*********************************************************************************************************
*/
rt_size_t rt_device_read(rt_device_t dev, rt_off_t pos, void *buffer, rt_size_t size)
{
RT_ASSERT(dev != RT_NULL);
//调用设备读取接口
if (dev->read != RT_NULL)
{
return dev->read(dev, pos, buffer, size);
}
//读取失败
rt_set_errno(-RT_ENOSYS);
return 0;
}
RTM_EXPORT(rt_device_read);
/*
*********************************************************************************************************
* 函 数 名: rt_device_write
* 功能说明: 写入设备数据
* 形 参: dev-设备 pos-写入位置 buffer-数据缓存 size-数据大小
* 返 回 值: 写状态
*********************************************************************************************************
*/
rt_size_t rt_device_write(rt_device_t dev, rt_off_t pos, const void *buffer, rt_size_t size)
{
RT_ASSERT(dev != RT_NULL);
//调用设备写接口
if (dev->write != RT_NULL)
{
return dev->write(dev, pos, buffer, size);
}
//写入失败
rt_set_errno(-RT_ENOSYS);
return 0;
}
RTM_EXPORT(rt_device_write);
/*
*********************************************************************************************************
* 函 数 名: rt_device_control
* 功能说明: 执行设备控制功能
* 形 参: dev-设备 cmd-控制命令 arg-控制参数
* 返 回 值: 执行状态
*********************************************************************************************************
*/
rt_err_t rt_device_control(rt_device_t dev, rt_uint8_t cmd, void *arg)
{
RT_ASSERT(dev != RT_NULL);
//调用设备控制执行接口
if (dev->control != RT_NULL)
{
return dev->control(dev, cmd, arg);
}
return RT_EOK;
}
RTM_EXPORT(rt_device_control);
/*
*********************************************************************************************************
* 函 数 名: rt_device_set_rx_indicate
* 功能说明: 设备接收数据回调函数
* 形 参: dev-设备 rx_ind-回调函数
* 返 回 值: 执行状态
*********************************************************************************************************
*/
rt_err_t rt_device_set_rx_indicate(rt_device_t dev, rt_err_t (*rx_ind)(rt_device_t dev, rt_size_t size))
{
RT_ASSERT(dev != RT_NULL);
//执行回调函数
dev->rx_indicate = rx_ind;
return RT_EOK;
}
RTM_EXPORT(rt_device_set_rx_indicate);
/*
*********************************************************************************************************
* 函 数 名: rt_device_set_rx_indicate
* 功能说明: 设备写入数据回调函数
* 形 参: dev-设备 tx_done-回调函数
* 返 回 值: 执行状态
*********************************************************************************************************
*/
rt_err_t rt_device_set_tx_complete(rt_device_t dev, rt_err_t (*tx_done)(rt_device_t dev, void *buffer))
{
RT_ASSERT(dev != RT_NULL);
//执行回调函数
dev->tx_complete = tx_done;
return RT_EOK;
}
RTM_EXPORT(rt_device_set_tx_complete);
#endif
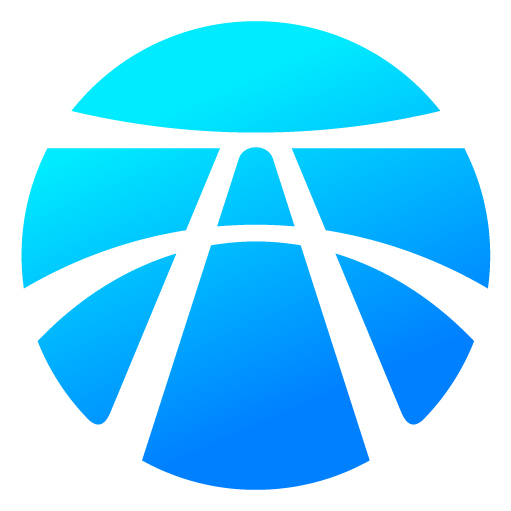
开放原子开发者工作坊旨在鼓励更多人参与开源活动,与志同道合的开发者们相互交流开发经验、分享开发心得、获取前沿技术趋势。工作坊有多种形式的开发者活动,如meetup、训练营等,主打技术交流,干货满满,真诚地邀请各位开发者共同参与!
更多推荐
所有评论(0)