依赖注入的方式
依赖注入的基本原则是:应用组件不应该负责查找资源或者其他依赖的协作对象。配置对象的工作应该由IoC容器负责,“查找资源”的逻辑应该从应用组件的代码中抽取出来,交给IoC容器负责。下面分别演示3中注入机制。代码2 待注入的业务对象Content.javapackage com.zj.ioc.di; public class Content { pu
package
com.zj.ioc.di;
public
class
Content {
public
void
BusniessContent(){
System.
out
.println(
"do business"
);
}
public
void
AnotherBusniessContent(){
System.
out
.println(
"do another business"
);
}
}
|
package
com.zj.ioc.di.ctor;
import
com.zj.ioc.di.Content;
public
class
MyBusiness {
private
Content
myContent
;
public
MyBusiness(Content content) {
myContent
= content;
}
public
void
doBusiness(){
myContent
.BusniessContent();
}
public
void
doAnotherBusiness(){
myContent
.AnotherBusniessContent();
}
}
|
package
com.zj.ioc.di.set;
import
com.zj.ioc.di.Content;
public
class
MyBusiness {
private
Content
myContent
;
public
void
setContent(Content content) {
myContent
= content;
}
public
void
doBusiness(){
myContent
.BusniessContent();
}
public
void
doAnotherBusiness(){
myContent
.AnotherBusniessContent();
}
}
|
package
com.zj.ioc.di.iface;
import
com.zj.ioc.di.Content;
public
interface
InContent {
void
createContent(Content content);
}
|
package
com.zj.ioc.di.iface;
import
com.zj.ioc.di.Content;
public
class
MyBusiness
implements
InContent{
private
Content
myContent
;
public
void
createContent(Content content) {
myContent
= content;
}
public
void
doBusniess(){
myContent
.BusniessContent();
}
public
void
doAnotherBusniess(){
myContent
.AnotherBusniessContent();
}
}
|
public static void main(String[] args) throws Exception{
//get the bean factory
BeanFactory factory = getBeanFactory();
MessageRender mr = (MessageRender) factory.getBean(“renderer”);
mr.render();
}
|
Spring依赖注入(DI)的三种方式,分别为:
1. Setter方法注入
2. 构造方法注入
3. 接口注入
下面介绍一下这三种依赖注入在Spring中是怎么样实现的。
Setter方法注入
首先我们需要以下几个类:
接口 Logic.java
接口实现类 LogicImpl.java
一个处理类 LoginAction.java
还有一个测试类 TestMain.java
Logic.java如下:
- <span style="font-size:18px;"><span style="font-size:18px;">package DI;
- //定义接口
- public interface Logic {
- public String getName();
- }
- </span></span>
LogicImpl.java如下:
- <span style="font-size:18px;"><span style="font-size:18px;">package DI;
- public class LogicImpl implements Logic {
- //实现类
- public String getName() {
- return "lishehe";
- }
- }
- </span></span>
LoginAction.java 会根据使用不同的注入方法而稍有不同
Setter方法注入:
- <span style="font-size:18px;"><span style="font-size:18px;">package DI;
- public class LoginAction {
- private Logic logic;
- public void execute() {
- String name = logic.getName();
- System.out.print("My Name Is " + name);
- }
- /**
- * @return the logic
- */
- public Logic getLogic() {
- return logic;
- }
- /**
- * @param logic
- * the logic to set
- */
- public void setLogic(Logic logic) {
- this.logic = logic;
- }
- }
- </span></span>
客户端测试类
TestMain.java
- <span style="font-size:18px;">package DI;
- import org.springframework.context.ApplicationContext;
- import org.springframework.context.support.FileSystemXmlApplicationContext;
- public class TestMain {
- /**
- * @param args
- */
- public static void main(String[] args) {
- // 得到ApplicationContext对象
- ApplicationContext ctx = new FileSystemXmlApplicationContext(
- "applicationContext.xml");
- // 得到Bean
- LoginAction loginAction = (LoginAction) ctx.getBean("loginAction");
- loginAction.execute();
- }
- }
- </span>
定义了一个Logic 类型的变量 logic, 在LoginAction并没有对logic 进行实例化,而只有他对应的setter/getter方法,因为我们这里使用的是Spring的依赖注入的方式
applicationContext.xml配置文件如下:
- <span style="font-size:18px;"><?xml version="1.0" encoding="UTF-8"?>
- <!--
- - Application context definition for JPetStore's business layer.
- - Contains bean references to the transaction manager and to the DAOs in
- - dataAccessContext-local/jta.xml (see web.xml's "contextConfigLocation").
- -->
- <beans xmlns="http://www.springframework.org/schema/beans"
- xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
- xmlns:aop="http://www.springframework.org/schema/aop"
- xmlns:tx="http://www.springframework.org/schema/tx"
- xsi:schemaLocation="
- http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-2.5.xsd
- http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-2.5.xsd
- http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx-2.5.xsd">
- <bean id="logic" class="DI.LogicImpl"/>
- <bean id="loginAction" class="DI.LoginAction">
- <property name="logic" ref="logic"></property>
- </bean>
- </beans>
- </span>
运行效果:
构造器注入
顾名思义,构造方法注入,就是我们依靠LoginAction的构造方法来达到DI的目的,如下所示:
- <span style="font-size:18px;">package DI;
- public class LoginAction {
- private Logic logic;
- public LoginAction(Logic logic) {
- this.logic = logic;
- }
- public void execute() {
- String name = logic.getName();
- System.out.print("My Name Is " + name);
- }
- }
- </span>
里我们添加了一个LoginAction的构造方法
applicationContext.xml配置文件如下:
- <span style="font-size:18px;"> <bean id="logic" class="DI.LogicImpl"/>
- <bean id="loginAction" class="DI.LoginAction">
- <constructor-arg index="0" ref="logic"></constructor-arg>
- </bean></span>
我们使用constructor-arg来进行配置, index属性是用来表示构造方法中参数的顺序的,如果有多个参数,则按照顺序,从 0,1...来配置
我们现在可以运行testMain.java了,结果跟使用Setter方法注入完全一样.
效果图
其中需要注意一点有:构造函数有多个参数的话,如:参数1,参数2,而参数2依赖于参数1,这中情况则要注意构造函数的顺序,必须将参数1放在参数2之前。
接口注入
下面继续说说我们不常用到的接口注入,还是以LogicAction为例,我们对他进行了修改,如下所示:
LogicAction.java
- <span style="font-size:18px;">package DI;
- public class LoginAction {
- private Logic logic;
- public void execute() {
- try {
- Object obj = Class.forName("DI.LogicImpl")
- .newInstance();
- logic = (Logic) obj;
- String name = logic.getName();
- System.out.print("My Name Is " + name);
- } catch (Exception e) {
- e.printStackTrace();
- }
- }
- }
- </span>
配置文件:
- <span style="font-size:18px;"><bean id="logic" class="DI.LogicImpl"/>
- <bean id="loginAction" class="DI.LoginAction"></span>
总结
对于Spring的依赖注入,最重要的就是理解他的,一旦理解了,将会觉得非常的简单。无非就是让容器来给我们实例化那些类,我们要做的就是给容器提供这个接口,这个接口就我们的set方法或者构造函数了。
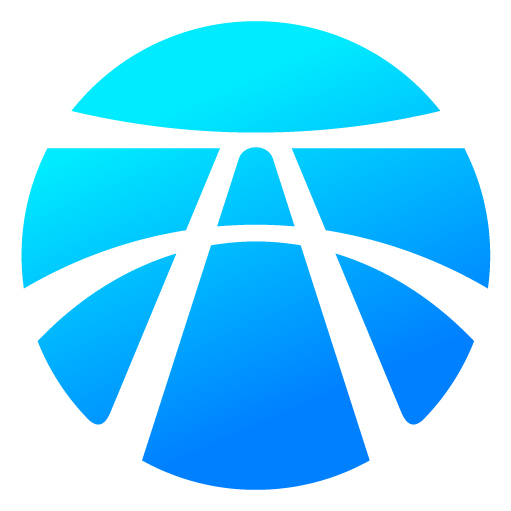
开放原子开发者工作坊旨在鼓励更多人参与开源活动,与志同道合的开发者们相互交流开发经验、分享开发心得、获取前沿技术趋势。工作坊有多种形式的开发者活动,如meetup、训练营等,主打技术交流,干货满满,真诚地邀请各位开发者共同参与!
更多推荐
所有评论(0)