C++ 基础之职工管理系统
本文代码仓库地址: gitee码云CSDN笔记仓库地址目录前言一、自己写de职工管理系统_xy1、(.h)头文件1.1、staffManagement_xy.h【职工管理类】1.2、staff_xy.h【职工类 -- 父类】1.3、ordinaryStaff_xy.h【普通职工类 -- 子类】1.4、managerStaff_xy.h【经理类 -- 子类】1.5、bossStaff_xy.h【老板
·
本文代码仓库地址: gitee码云CSDN笔记仓库地址
前言
—— 自己写的代码很丑,但是对于小白比较友好
—— 完全是凭借前面的基础部分进行的
—— 我是先过一遍视频,了解其中的需求,有了大概的思路,然后关上视频,纯凭感觉写一步看一步,零零碎碎的拼凑起来了,当然这期间也收获了不少东西,特别是采坑,再自己爬起来会印象比较深刻。
—— 就算是按照视频里面的思路来的,也会举一反三的想,这个东西用别的方式或者换个思路去完成相同的目的是否可行,这而期间就会发现有些东西并不是我们想的那样,这就需要去爬坑,这种成长的过程非常棒。
—— 更重要的就是多动手进行敲代码,正因为前面基础部分的代码全部是我手敲出来,所以我在写这个小小小系统的时候非常的有感觉,也相对比较容易理解,想要什么东西,马上直接可以用,或者忘记了,之前做了笔记的地方都会有印象去查找一下就知道了。
一、自己写de职工管理系统_xy
1、(.h)头文件
1.1、staffManagement_xy.h【职工管理类】
// 确保头文件命名不重复
#pragma once
#include <iostream>
#include "staff_xy.h"
#include "ordinaryStaff_xy.h"
#include "managerStaff_xy.h"
#include "bossStaff_xy.h"
#include <fstream>
// 定义宏 -- 文件名
#define FILEADDRESS "StaffInformationDocument_fxy.txt"
using namespace std;
// 职工管理类
class staffManagement_xy {
public:
// 判断文件是否存在【存在为true,不存在为false】
bool mFileIsExist; // 待完成
staffManagement_xy();
// 展示菜单
void mShowMenu();
// 退出系统
void mExitSystem();
// 添加职工
void mAddStaff();
// 员工信息追加到文件中
void mAppendInformation(int id, string name, int age, int title);
// 显示所有职工信息
void mShowStaffInformation();
// 职工总人数(多少行数据)
int mRedRow();
// 删除职工(通过职工编号)
void mDelStaff(int id);
// 清空文件
void mEmptyFile();
// 判断职工是否存在(通过编号)
bool mIsExist(int id);
// 修改职工信息
void mEditStaffInformation(int id);
// 查找(筛选)职工
void mFindStaff(char ch0);
// 按照职工的编号进行排序
void mSortStaff(char ch0);
};
1.2、staff_xy.h【职工类 – 父类】
#pragma once
#include <iostream>
#define FILEADDRESS "StaffInformationDocument_fxy.txt"
using namespace std;
// 职工类 -- 父类
class staff_xy {
public:
// 职工编号
int mId;
// 职工姓名
string mName;
// 职工年龄
int mAge;
// 职工职称
int mTitle;
staff_xy() {
mId = 0;
mAge = 0;
mTitle = 0;
}
// 设置职工信息
virtual void mSetInformation(int id, string name, int age, int title) = 0;
// 显示个人信息
virtual void mShowInformation(int id, string name, int age) = 0;
};
1.3、ordinaryStaff_xy.h【普通职工类 – 子类】
#pragma once
#include "staff_xy.h"
// 普通职工类 -- 子类
class ordinaryStaff_xy : public staff_xy {
public:
ordinaryStaff_xy();
// 重写父类中的设置职工信息函数
virtual void mSetInformation(int id, string name, int age, int title);
// 重写显示个人信息
virtual void mShowInformation(int id, string name, int age);
};
1.4、managerStaff_xy.h【经理类 – 子类】
#pragma once
#include "staff_xy.h"
// 经理类 -- 子类
class managerStaff_xy :public staff_xy {
public:
managerStaff_xy();
// 重写父类中的设置职工信息函数
virtual void mSetInformation(int id, string name, int age, int title);
// 重写显示个人信息
virtual void mShowInformation(int id, string name, int age);
};
1.5、bossStaff_xy.h【老板类 – 子类】
#pragma once
#include "staff_xy.h"
// 老板类 -- 子类
class bossStaff_xy :public staff_xy {
public:
bossStaff_xy();
// 重写父类中的设置职工信息函数
virtual void mSetInformation(int id, string name, int age, int title);
// 重写显示个人信息
virtual void mShowInformation(int id, string name, int age);
};
2、(.cpp)源文件
2.1、Main.cpp
#include <iostream>
#include "staffManagement_xy.h"
using namespace std;
/*
* 循环+判断让用户进行选择功能
*/
int main() {
// 实例化职工管理
staffManagement_xy sm;
char ch01 = 0;
// 循环用户输入选择操作功能
while (true)
{
// 展示菜单
sm.mShowMenu();
cin >> ch01;
switch (ch01)
{
case '0': // 退出管理系统
sm.mExitSystem();
break;
case '1': // 增加职工信息
{
sm.mAddStaff();
system("pause");
system("cls");
}
break;
case '2': // 显示职工信息
{
sm.mShowStaffInformation();
system("pause");
system("cls");
}
break;
case '3': // 删除职工信息
{
int tempId;
cout << "请输入需要删除职工的编号:" << endl;
cin >> tempId;
sm.mDelStaff(tempId);
system("pause");
system("cls");
}
break;
case '4': // 修改职工信息
{
int tempId = 0;
cout << "请输入需要修改的职工编号:" << endl;
cin >> tempId;
sm.mEditStaffInformation(tempId);
system("pause");
system("cls");
}
break;
case '5': // 查找职工信息
{
char temp0;
cout << "请选择查找方式:" << endl;
cout << "1:按照职工编号查找" << endl;
cout << "2:按照职工姓名查找" << endl;
cin >> temp0;
sm.mFindStaff(temp0);
system("pause");
system("cls");
}
break;
case '6': // 按照编号排序
{
char temp0;
cout << "请选择排序方式:" << endl;
cout << "1:按照职工编号升序排列" << endl;
cout << "2:按照职工编号降序排列" << endl;
cout << "其他:无排序" << endl;
cin >> temp0;
sm.mSortStaff(temp0);
system("pause");
system("cls");
}
break;
case '7': // 清空所有数据
{
char ch7;
cout << "是否清空所有职工信息?" << endl;
cout << "1:确认并返回" << endl;
cout << "2:取消并返回" << endl;
cin >> ch7;
if (ch7 == '1')
{
sm.mEmptyFile();
}
system("cls");
}
break;
default:
{
cout << "搞错了,请输入正确的选择" << endl;
system("pause");
system("cls");
}
break;
}
}
cout << endl;
system("pause");
return 0;
}
2.2、staffManagement_xy.cpp
#include "staffManagement_xy.h"
staffManagement_xy::staffManagement_xy() {
mFileIsExist = false;
}
// 展示菜单
void staffManagement_xy::mShowMenu() {
cout << "************************************************" << endl;
cout << "*********** 欢迎使用职工管理系统 ***********" << endl;
cout << "************* 0:退出管理系统 *************" << endl;
cout << "************* 1:增加职工信息 *************" << endl;
cout << "************* 2:显示职工信息 *************" << endl;
cout << "************* 3:删除职工信息 *************" << endl;
cout << "************* 4:修改职工信息 *************" << endl;
cout << "************* 5:查找职工信息 *************" << endl;
cout << "************* 6:按照编号排序 *************" << endl;
cout << "************* 7:清空所有数据 *************" << endl;
cout << "************************************************" << endl;
cout << " 请选择使用功能:" << endl;
}
// 退出系统
void staffManagement_xy::mExitSystem() {
cout << "退出成功啦!!!" << endl;
system("pause");
// 退出系统
exit(0);
}
// 添加职工
void staffManagement_xy::mAddStaff() {
// 职工类 -- 父类先指向空
staff_xy* s01 = NULL;
int sId = 0;
cout << "请输入职工编号:" << endl;
cin >> sId;
string sName;
cout << "请输入职工姓名:" << endl;
cin >> sName;
int sAge = 0;
cout << "请输入职工年龄:" << endl;
cin >> sAge;
int sTitle = 0;
cout << "请选择添加的职工的职称:" << endl;
cout << "1:老板" << endl;
cout << "2:经理:" << endl;
cout << "3:普通职工:" << endl;
cin >> sTitle;
switch (sTitle)
{
case 1: // 老板
{
s01 = new bossStaff_xy;
s01->mSetInformation(sId, sName, sAge, sTitle);
mAppendInformation(sId, sName, sAge, sTitle);
if (s01 != NULL)
{
delete s01;
s01 = NULL;
}
cout << "添加成功!" << endl;
}
break;
case 2: // 经理
{
s01 = new managerStaff_xy;
s01->mSetInformation(sId, sName, sAge, sTitle);
mAppendInformation(sId, sName, sAge, sTitle);
if (s01 != NULL)
{
delete s01;
s01 = NULL;
}
cout << "添加成功!" << endl;
}
break;
case 3: // 普通职员
{
s01 = new ordinaryStaff_xy;
s01->mSetInformation(sId, sName, sAge, sTitle);
mAppendInformation(sId, sName, sAge, sTitle);
if (s01 != NULL)
{
delete s01;
s01 = NULL;
}
cout << "添加成功!" << endl;
}
break;
default:
cout << "输入错误!!!" << endl;
break;
}
}
// 员工信息追加到文件中
void staffManagement_xy::mAppendInformation(int id, string name, int age, int title) {
// 创建对象流
ofstream ofs;
// 打开文件
ofs.open(FILEADDRESS, ios::app);
// 添加追加信息
ofs << id << " " << name << " " << age << " " << title << endl;
// 关闭文件
ofs.close();
}
// 显示所有职工信息
void staffManagement_xy::mShowStaffInformation() {
char ch[1024] = { 0 };
ifstream ifs;
ifs.open(FILEADDRESS, ios::in);
// 判断是否成功打开文件
if (!ifs.is_open())
{
cout << "文件打开失败!" << endl;
return;
}
int sId = 0;
string sName;
int sAge = 0;
char sTitle = NULL;
staff_xy* ms0 = NULL;
// 直接从文件流中取出数据,每次循环都是一行数据,>> 右移
while (ifs >> sId && ifs >> sName && ifs >> sAge && ifs >> sTitle)
{
switch (sTitle)
{
case '1': // 老板
{
ms0 = new bossStaff_xy;
ms0->mShowInformation(sId, sName, sAge);
if (ms0 != NULL)
{
delete ms0;
ms0 = NULL;
}
}
break;
case '2': // 经理
{
ms0 = new managerStaff_xy;
ms0->mShowInformation(sId, sName, sAge);
if (ms0 != NULL)
{
delete ms0;
ms0 = NULL;
}
}
break;
case '3': // 普通职工
{
ms0 = new ordinaryStaff_xy;
ms0->mShowInformation(sId, sName, sAge);
if (ms0 != NULL)
{
delete ms0;
ms0 = NULL;
}
}
break;
default:
cout << "啥也不是" << endl;
break;
}
}
ifs.close();
}
// 职工总人数(返回值为有多少行数据)
int staffManagement_xy::mRedRow() {
char ch[1024] = { 0 };
int i = 0;
ifstream ifs;
ifs.open(FILEADDRESS, ios::in);
if (!ifs.is_open())
{
cout << "文件打开失败!" << endl;
return 0; // 这里需要处理一下
}
while (ifs.getline(ch, sizeof(ifs)))
{
i++;
}
return i;
}
// 删除职工(编号唯一)
void staffManagement_xy::mDelStaff(int id) {
// 判断职工是否存在
if (!mIsExist(id))
{
cout << "查无此人!!!" << endl;
return;
}
// 获取数据行数
int mNum = mRedRow();
ifstream ifs;
ifs.open(FILEADDRESS, ios::in);
if (!ifs.is_open())
{
cout << "文件打开失败!" << endl;
return;
}
// 接受文件流的数据
int sId = 0;
string sName;
int sAge = 0;
int sTitle = 0;
// 判断是否有删除和删除条数
int sNum = 0;
// 作为临时存储数据
int arr01[100] = { 0 };
string arr02[100] = { "" };
int arr03[100] = { 0 };
int arr04[100] = { 0 };
// 用来进行判断覆盖操作在哪里开始执行
int j = -1;
for (int i = 0; i < mNum; i++)
{
// 从文件流中取出当前行所有数据
ifs >> sId && ifs >> sName && ifs >> sAge && ifs >> sTitle;
// 判断该条数据是否是需要删除的职工信息
if (sId == id)
{
// 进行确认是否删除
char ch7;
cout << "是否确认删除?" << endl;
cout << "1:确认" << endl;
cout << "2:取消" << endl;
cin >> ch7;
if (ch7 == '1')
{
// 确定删除 sNum++
sNum++;
j = i;
cout << "已成功删除" << sNum << "条信息" << endl;
}
else
{
// 取消,保存该条信息至临时数组
// cout << "保存" << endl;
arr01[i] = sId;
arr02[i] = sName;
arr03[i] = sAge;
arr04[i] = sTitle;
}
}
else
{
// 不是要删除的职工信息直接保存至临时数组
// cout << "保存" << endl;
arr01[i] = sId;
arr02[i] = sName;
arr03[i] = sAge;
arr04[i] = sTitle;
}
}
// 清空文件
mEmptyFile();
// 判断是否有删除的职工信息
if (sNum > 0)
{
// 有删除就把数据条数减一
mNum--;
// 遍历数组,进行数据左移,覆盖删除数据
for (int i = j; i < mNum; i++)
{
// 删除 -- 进行左移覆盖操作
arr01[i] = arr01[i + 1];
arr02[i] = arr02[i + 1];
arr03[i] = arr03[i + 1];
arr04[i] = arr04[i + 1];
}
// 左移后重置删除人数
sNum = 0;
}
// 遍历添加数据至空文件中,进而实现删除的效果
for (int i = 0; i < mNum; i++)
{
// cout << arr01[i] << arr02[i] << arr03[i] << arr04[i] << endl;
// 追加保存到文件中
mAppendInformation(arr01[i], arr02[i], arr03[i], arr04[i]);
}
ifs.close();
}
// 清空文件
void staffManagement_xy::mEmptyFile() {
ofstream ofs;
ofs.open(FILEADDRESS, ios::trunc);
ofs.close();
}
// 判断职工是否存在(通过编号)
bool staffManagement_xy::mIsExist(int id) {
int mNum = mRedRow();
char ch[1024] = { 0 };
ifstream ifs;
ifs.open(FILEADDRESS, ios::in);
if (!ifs.is_open())
{
cout << "文件打开失败!" << endl;
return false;
}
int sId = 0;
string sName;
int sAge = 0;
char sTitle = NULL;
for (int i = 0; i < mNum; i++)
{
ifs >> sId && ifs >> sName && ifs >> sAge && ifs >> sTitle;
if (sId == id)
{
return true;
}
}
ifs.close();
return false;
}
// 修改职工信息
void staffManagement_xy::mEditStaffInformation(int id) {
// 判断职工是否存在
if (!mIsExist(id))
{
cout << "查无此人!!!" << endl;
return;
}
// 获取数据行数
int mNum = mRedRow();
ifstream ifs;
ifs.open(FILEADDRESS, ios::in);
if (!ifs.is_open())
{
cout << "文件打开失败!" << endl;
return;
}
// 接受文件流的数据
int sId = 0;
string sName;
int sAge = 0;
int sTitle = 0;
int sNum = 0;
// 作为临时存储数据
int arr01[100] = { 0 };
string arr02[100] = { "" };
int arr03[100] = { 0 };
int arr04[100] = { 0 };
for (int i = 0; i < mNum; i++)
{
// 从文件流中取出当前行所有数据
ifs >> sId && ifs >> sName && ifs >> sAge && ifs >> sTitle;
// 判断该条数据是否是需要修改的职工信息
if (sId == id)
{
cout << "请输入修改后的姓名:" << endl;
cin >> arr02[i];
cout << "请输入修改后的年龄:" << endl;
cin >> arr03[i];
cout << "请输入修改后的职称:" << endl;
cin >> arr04[i];
// 进行确认是否修改
char ch7;
cout << "是否确认修改?" << endl;
cout << "1:确认" << endl;
cout << "2:取消" << endl;
cin >> ch7;
if (ch7 == '1')
{
// 确定修改
sNum++;
arr01[i] = sId;
cout << "已成功修改" << sNum << "条信息" << endl;
}
else
{
// 取消,保存该条信息至临时数组
// cout << "保存" << endl;
arr01[i] = sId;
arr02[i] = sName;
arr03[i] = sAge;
arr04[i] = sTitle;
}
}
else
{
// 不需要修改的职工信息直接保存至临时数组
// cout << "保存" << endl;
arr01[i] = sId;
arr02[i] = sName;
arr03[i] = sAge;
arr04[i] = sTitle;
}
}
// 清空文件
mEmptyFile();
// 遍历添加数据至空文件中,进而实现修改的效果
for (int i = 0; i < mNum; i++)
{
// cout << arr01[i] << arr02[i] << arr03[i] << arr04[i] << endl;
// 追加保存到文件中
mAppendInformation(arr01[i], arr02[i], arr03[i], arr04[i]);
}
ifs.close();
}
// 查找(筛选)职工
void staffManagement_xy::mFindStaff(char ch0) {
switch (ch0)
{
case '1': // 按照编号进行查找
{
int id = 0;
cout << "请输入查找的职工编号:" << endl;
cin >> id;
// 判断职工是否存在
if (!mIsExist(id))
{
cout << "查无此人!!!" << endl;
return;
}
// 获取数据行数
int mNum = mRedRow();
ifstream ifs;
ifs.open(FILEADDRESS, ios::in);
if (!ifs.is_open())
{
cout << "文件打开失败!" << endl;
return;
}
// 接受文件流的数据
int sId = 0;
string sName;
int sAge = 0;
int sTitle = 0;
int sNum = 0;
for (int i = 0; i < mNum; i++)
{
// 从文件流中取出当前行所有数据
ifs >> sId && ifs >> sName && ifs >> sAge && ifs >> sTitle;
if (sId == id)
{
switch (sTitle)
{
case 1: // 老板
{
cout << "职工编号:" << sId << "\t职工姓名:" << sName << "\t职工年龄:" << sAge << "\t职工职称:老板\t工作内容:需要做好公司的规划!" << endl;
}
break;
case 2: // 经理
{
cout << "职工编号:" << sId << "\t职工姓名:" << sName << "\t职工年龄:" << sAge << "\t职工职称:经理\t工作内容:做好老板交代的事情,并且给普通职工发放任务!" << endl;
}
break;
case 3: // 普通职工
{
cout << "职工编号:" << sId << "\t职工姓名:" << sName << "\t职工年龄:" << sAge << "\t职工职称:员工\t工作内容:做好经理下发的任务!" << endl;
}
break;
default:
cout << "职工编号:" << sId << "\t职工姓名:" << sName << "\t职工年龄:" << sAge << "\t职工职称:啥也不是" << endl;
break;
}
}
}
ifs.close();
}
break;
case '2': // 按照姓名进行查找
{
string name;
cout << "请输入查找的职工姓名:" << endl;
cin >> name;
// 获取数据行数
int mNum = mRedRow();
ifstream ifs;
ifs.open(FILEADDRESS, ios::in);
if (!ifs.is_open())
{
cout << "文件打开失败!" << endl;
return;
}
// 接受文件流的数据
int sId = 0;
string sName;
int sAge = 0;
int sTitle = 0;
int sNum = 0;
int j = 0;
for (int i = 0; i < mNum; i++)
{
// 从文件流中取出当前行所有数据
ifs >> sId && ifs >> sName && ifs >> sAge && ifs >> sTitle;
if (sName == name)
{
switch (sTitle)
{
case 1: // 老板
{
cout << "职工编号:" << sId << "\t职工姓名:" << sName << "\t职工年龄:" << sAge << "\t职工职称:老板\t工作内容:需要做好公司的规划!" << endl;
}
break;
case 2: // 经理
{
cout << "职工编号:" << sId << "\t职工姓名:" << sName << "\t职工年龄:" << sAge << "\t职工职称:经理\t工作内容:做好老板交代的事情,并且给普通职工发放任务!" << endl;
}
break;
case 3: // 普通职工
{
cout << "职工编号:" << sId << "\t职工姓名:" << sName << "\t职工年龄:" << sAge << "\t职工职称:员工\t工作内容:做好经理下发的任务!" << endl;
}
break;
default:
cout << "职工编号:" << sId << "\t职工姓名:" << sName << "\t职工年龄:" << sAge << "\t职工职称:啥也不是" << endl;
break;
}
j++;
}
}
ifs.close();
if (j == 0)
{
cout << "查找信息为空!!!" << endl;
}
}
break;
default:
cout << "啥也不是" << endl;
break;
}
}
// 按照职工的编号进行排序
void staffManagement_xy::mSortStaff(char ch0) {
// 获取数据行数
int mNum = mRedRow();
ifstream ifs;
ifs.open(FILEADDRESS, ios::in);
if (!ifs.is_open())
{
cout << "文件打开失败!" << endl;
return;
}
// 接受文件流的数据
int sId = 0;
string sName;
int sAge = 0;
int sTitle = 0;
int sNum = 0;
int j = 0;
// 作为临时存储数据
int arr01[100] = { 0 };
string arr02[100] = { "" };
int arr03[100] = { 0 };
int arr04[100] = { 0 };
for (int i = 0; i < mNum; i++)
{
ifs >> sId && ifs >> sName && ifs >> sAge && ifs >> sTitle;
arr01[i] = sId;
arr02[i] = sName;
arr03[i] = sAge;
arr04[i] = sTitle;
}
ifs.close();
switch (ch0)
{
case '1': // 升序
{
for (int i = 0; i < mNum - 1; i++)
{
for (int j = 0; j < mNum - i - 1; j++)
{
if (arr01[j] > arr01[j + 1])
{
arr01[mNum + 1] = arr01[j];
arr02[mNum + 1] = arr02[j];
arr03[mNum + 1] = arr03[j];
arr04[mNum + 1] = arr04[j];
arr01[j] = arr01[j + 1];
arr02[j] = arr02[j + 1];
arr03[j] = arr03[j + 1];
arr04[j] = arr04[j + 1];
arr01[j + 1] = arr01[mNum + 1];
arr02[j + 1] = arr02[mNum + 1];
arr03[j + 1] = arr03[mNum + 1];
arr04[j + 1] = arr04[mNum + 1];
}
}
}
for (int i = 0; i < mNum; i++)
{
switch (arr04[i])
{
case 1: // 老板
{
cout << "职工编号:" << arr01[i] << "\t职工姓名:" << arr02[i] << "\t职工年龄:" << arr03[i] << "\t职工职称:老板\t工作内容:需要做好公司的规划!" << endl;
}
break;
case 2: // 经理
{
cout << "职工编号:" << arr01[i] << "\t职工姓名:" << arr02[i] << "\t职工年龄:" << arr03[i] << "\t职工职称:经理\t工作内容:做好老板交代的事情,并且给普通职工发放任务!" << endl;
}
break;
case 3: // 普通职工
{
cout << "职工编号:" << arr01[i] << "\t职工姓名:" << arr02[i] << "\t职工年龄:" << arr03[i] << "\t职工职称:员工\t工作内容:做好经理下发的任务!" << endl;
}
break;
default:
cout << "职工编号:" << arr01[i] << "\t职工姓名:" << arr02[i] << "\t职工年龄:" << arr03[i] << "\t职工职称:啥也不是" << endl;
break;
}
}
}
break;
case '2': // 降序
{
for (int i = 0; i < mNum - 1; i++)
{
for (int j = 0; j < mNum - i - 1; j++)
{
if (arr01[j] < arr01[j + 1])
{
arr01[mNum + 1] = arr01[j];
arr02[mNum + 1] = arr02[j];
arr03[mNum + 1] = arr03[j];
arr04[mNum + 1] = arr04[j];
arr01[j] = arr01[j + 1];
arr02[j] = arr02[j + 1];
arr03[j] = arr03[j + 1];
arr04[j] = arr04[j + 1];
arr01[j + 1] = arr01[mNum + 1];
arr02[j + 1] = arr02[mNum + 1];
arr03[j + 1] = arr03[mNum + 1];
arr04[j + 1] = arr04[mNum + 1];
}
}
}
for (int i = 0; i < mNum; i++)
{
switch (arr04[i])
{
case 1: // 老板
{
cout << "职工编号:" << arr01[i] << "\t职工姓名:" << arr02[i] << "\t职工年龄:" << arr03[i] << "\t职工职称:老板\t工作内容:需要做好公司的规划!" << endl;
}
break;
case 2: // 经理
{
cout << "职工编号:" << arr01[i] << "\t职工姓名:" << arr02[i] << "\t职工年龄:" << arr03[i] << "\t职工职称:经理\t工作内容:做好老板交代的事情,并且给普通职工发放任务!" << endl;
}
break;
case 3: // 普通职工
{
cout << "职工编号:" << arr01[i] << "\t职工姓名:" << arr02[i] << "\t职工年龄:" << arr03[i] << "\t职工职称:员工\t工作内容:做好经理下发的任务!" << endl;
}
break;
default:
cout << "职工编号:" << arr01[i] << "\t职工姓名:" << arr02[i] << "\t职工年龄:" << arr03[i] << "\t职工职称:啥也不是" << endl;
break;
}
}
}
break;
default: // 默认排序
mShowStaffInformation();
break;
}
}
2.3、ordinaryStaff_xy.cpp
#include "ordinaryStaff_xy.h"
ordinaryStaff_xy::ordinaryStaff_xy() {
}
// 设置普通职工信息
void ordinaryStaff_xy::mSetInformation(int id, string name, int age, int title) {
this->mId = id;
this->mName = name;
this->mAge = age;
this->mTitle = title;
}
// 重写显示个人信息
void ordinaryStaff_xy::mShowInformation(int id, string name, int age) {
cout << "职工编号:" << id << "\t职工姓名:" << name << "\t职工年龄:" << age << "\t职工职称:员工\t工作内容:做好经理下发的任务!" << endl;
}
2.4、managerStaff_xy.cpp
#include "managerStaff_xy.h"
managerStaff_xy::managerStaff_xy() {
}
// 重写父类中的设置职工信息函数
void managerStaff_xy::mSetInformation(int id, string name, int age, int title) {
this->mId = id;
this->mName = name;
this->mAge = age;
this->mTitle = title;
}
// 重写显示个人信息
void managerStaff_xy::mShowInformation(int id, string name, int age) {
cout << "职工编号:" << id << "\t职工姓名:" << name << "\t职工年龄:" << age << "\t职工职称:经理\t工作内容:做好老板交代的事情,并且给普通职工发放任务!" << endl;
}
2.5、bossStaff_xy.cpp
#include "bossStaff_xy.h"
bossStaff_xy::bossStaff_xy() {
}
// 重写父类中的设置职工信息函数
void bossStaff_xy::mSetInformation(int id, string name, int age, int title) {
this->mId = id;
this->mName = name;
this->mAge = age;
this->mTitle = title;
}
// 重写显示个人信息
void bossStaff_xy::mShowInformation(int id, string name, int age) {
cout << "职工编号:" << id << "\t职工姓名:" << name << "\t职工年龄:" << age << "\t职工职称:老板\t工作内容:需要做好公司的规划!" << endl;
}
二、视频中de职工管理系统_xy
1、(.h)头文件
1.1、workerManager_xy.h【职工管理类】
// 防止头文件重复
#pragma once
// 添加标准流
#include <iostream>
#include "worker_xy.h"
#include "employee_xy.h"
#include "manager_xy.h"
#include "boss_xy.h"
#include <fstream>
#define FILEADDRESS "StaffInformationDocument_fxy.txt"
using namespace std;
// 管理类
class workerManager_xy {
public:
// 记录文件中当前人数
int mEmpNum;
// 职工数组的指针
worker_xy** mEmpArray;
// 判断文件是否为空 标志
bool mFileIsEmpty;
// ------------------------------
// 管理类的构造函数
workerManager_xy();
// ------------------------------
// 展示菜单
void mShowMenu();
// 退出程序
void mExitSystem();
// 添加职工
void mAddEmp();
// 保存至文件
void mSave();
// 获取文件中当前职工人数(数据行数)
int mGetRow();
// 初始化员工
void mInitEmp();
// 创建职工
void mNewEmp(int id, string name, int tId, int index);
// 显示职工信息
void mShowEmp();
// 删除职工信息
void mDelEmp();
// 判断职工是否存在,如果存在返回职工所在位置,不存在返回-1
int mIsExist(int id);
// 修改职工信息
void mEditEmp();
// 查找职工信息
void mFindEmp();
// 排序职工【选择排序】
void mSortEmp();
// 清空数据
void mCleanFile();
// ------------------------------
// 管理类的析构函数
~workerManager_xy();
};
1.2、worker_xy.h【职工类 – 父类】
#pragma once
#include <iostream>
using namespace std;
// 职工类 -- 父类
class worker_xy {
public:
// 职工编号
int mId;
// 职工姓名
string mName;
// 职工职称
int mTitle;
// 显示个人信息
virtual void mShowInfo() = 0;
// 获取岗位名称
virtual string mGetTitleName() = 0;
};
1.3、employee_xy.h【普通职工类 – 子类】
#pragma once
#include "worker_xy.h"
#include <iostream>
using namespace std;
// 普通职工类 -- 子类
class employee_xy :public worker_xy {
public:
// 普通职工类的构造函数
employee_xy(int id, string name, int tId);
// 显示个人信息
virtual void mShowInfo();
// 获取岗位名称
virtual string mGetTitleName();
};
1.4、manager_xy.h【经理类 – 子类】
#pragma once
#include "worker_xy.h"
#include <iostream>
using namespace std;
// 经理类 -- 子类
class manager_xy :public worker_xy {
public:
// 普通职工类的构造函数
manager_xy(int id, string name, int tId);
// 显示个人信息
virtual void mShowInfo();
// 获取岗位名称
virtual string mGetTitleName();
};
1.5、boss_xy.h【老板类 – 子类】
#pragma once
#include "worker_xy.h"
#include <iostream>
using namespace std;
// 老板类 -- 子类
class boss_xy :public worker_xy {
public:
// 老板类的构造函数
boss_xy(int id, string name, int tId);
// 显示个人信息
virtual void mShowInfo();
// 获取岗位名称
virtual string mGetTitleName();
};
2、(.cpp)源文件
2.1、Main.cpp
#include <iostream>
#include "workerManager_xy.h"
#include "worker_xy.h"
#include "employee_xy.h"
#include "manager_xy.h"
#include "boss_xy.h"
using namespace std;
int main() {
workerManager_xy wm;
int choos = '-';
while (true)
{
wm.mShowMenu();
cin >> choos;
switch (choos)
{
case 0: // 退出管理系统
{
wm.mExitSystem();
}
break;
case 1: // 增加职工信息
{
wm.mAddEmp();
}
break;
case 2: // 显示职工信息
{
wm.mShowEmp();
}
break;
case 3: // 删除职工信息
{
wm.mDelEmp();
}
break;
case 4: // 修改职工信息
{
wm.mEditEmp();
}
break;
case 5: // 查找职工信息
{
wm.mFindEmp();
}
break;
case 6: // 按照编号排序
{
wm.mSortEmp();
}
break;
case 7: // 清空所有数据
{
wm.mCleanFile();
}
break;
default:
system("cls");
break;
}
}
/*
system("pause");
system("cls");
*/
cout << endl;
system("pause");
return 0;
}
2.2、workerManager_xy.cpp
#include "workerManager_xy.h"
// 管理类的构造函数
workerManager_xy::workerManager_xy() {
ifstream ifs;
// 读文件
ifs.open(FILEADDRESS, ios::in);
if (!ifs.is_open())
{
// 1、文件不存在
// cout << "文件不存在" << endl;
// 初始化记录人数
this->mEmpNum = 0;
// 初始化数组指针
this->mEmpArray = NULL;
// 初始化文件是否为空
this->mFileIsEmpty = true;
ifs.close();
return;
}
char ch;
ifs >> ch;
if (ifs.eof())
{
// 2、文件存在 数据为空
// cout << "文件存在 数据为空" << endl;
// 初始化记录人数
this->mEmpNum = 0;
// 初始化数组指针
this->mEmpArray = NULL;
// 初始化文件是否为空
this->mFileIsEmpty = true;
ifs.close();
return;
}
// 3、文件存在,并且有数据
int mNum = this->mGetRow();
// cout << "职工人数为:" << mNum << endl;
this->mEmpNum = mNum;
// 在堆区开辟控件
this->mEmpArray = new worker_xy * [this->mEmpNum];
// 将文件中的数据,存到数组中
this->mInitEmp();
}
// 展示菜单
void workerManager_xy::mShowMenu() {
cout << "************************************************" << endl;
cout << "*********** 欢迎使用职工管理系统 ***********" << endl;
cout << "************* 0:退出管理系统 *************" << endl;
cout << "************* 1:增加职工信息 *************" << endl;
cout << "************* 2:显示职工信息 *************" << endl;
cout << "************* 3:删除职工信息 *************" << endl;
cout << "************* 4:修改职工信息 *************" << endl;
cout << "************* 5:查找职工信息 *************" << endl;
cout << "************* 6:按照编号排序 *************" << endl;
cout << "************* 7:清空所有数据 *************" << endl;
cout << "************************************************" << endl;
cout << endl;
cout << "请输入您的选择:";
}
// 退出程序
void workerManager_xy::mExitSystem() {
cout << "欢迎下次使用" << endl;
system("pause");
exit(0);
}
// 添加职工
void workerManager_xy::mAddEmp() {
cout << "请输入添加职工数量:";
int addNum = 0;
cin >> addNum;
if (addNum > 0)
{
// 添加
// 计算添加新空间大小【新空间的人数 = 文件中的人数 + 新增的人数】
int newSize = this->mEmpNum + addNum;
cout << "newSize:" << newSize << endl;
cout << "mEmpNum:" << mEmpNum << endl;
// 开辟新空间来存放添加的信息【感觉是这里出了问题】
worker_xy** newSpace = new worker_xy * [newSize];
if (this->mEmpNum != NULL)
{
for (int i = 0; i < this->mEmpNum; i++)
{
// 新开辟的数组接受原来数组中(文件中已有)的数据
newSpace[i] = this->mEmpArray[i];
}
}
// 添加新数据
for (int i = 0; i < addNum; i++)
{
// 职工编号
int id;
// 职工姓名
string name;
// 职工职称ID
int tId;
cout << "请输入第" << i + 1 << "个新职工的编号:";
cin >> id;
cout << "请输入第" << i + 1 << "个新职工的姓名:";
cin >> name;
cout << "请选择第" << i + 1 << "个新职工的职称:" << endl;
cout << "1:员工" << endl;
cout << "2:经理" << endl;
cout << "3:老板" << endl;
cin >> tId;
// 创建职工
// mNewEmp(id, name, tId, (this->mEmpNum + i));
worker_xy* worker = NULL;
// 创建职工
switch (tId)
{
case 1:
worker = new employee_xy(id, name, tId);
break;
case 2:
worker = new manager_xy(id, name, tId);
break;
case 3:
worker = new boss_xy(id, name, tId);
break;
default:
cout << "进来会报错" << endl;
break;
}
// 将创建职工指针,保存到数组中【这样做的目的是为了预留文件中信息的存放】
newSpace[(this->mEmpNum + i)] = worker;
}
// 释放原有空间【释放数组的时候需要加[]】
delete[] this->mEmpArray;
// 更改新空间的指向
this->mEmpArray = newSpace;
// 更新新的职工人数
this->mEmpNum = newSize;
// 添加成功后保存至文件中
this->mSave();
// 提示添加成功
cout << "成功添加" << addNum << "名职工信息" << endl;
// 更新职工文件不为空的情况
this->mFileIsEmpty = false;
}
else
{
cout << "提示:添加人数不能小于0" << endl;
}
// 按任意键后 清楚屏幕信息
system("pause");
system("cls");
}
// 保存至文件
void workerManager_xy::mSave() {
ofstream ofs;
// 将每个人的信息保存至文件中
ofs.open(FILEADDRESS, ios::out);
for (int i = 0; i < this->mEmpNum; i++)
{
ofs << this->mEmpArray[i]->mId << " "
<< this->mEmpArray[i]->mName << " "
<< this->mEmpArray[i]->mTitle << endl;
}
ofs.close();
}
// 获取文件中当前职工人数(数据行数)
int workerManager_xy::mGetRow() {
ifstream ifs;
ifs.open(FILEADDRESS, ios::in);
if (!ifs.is_open())
{
cout << "文件打开失败!" << endl;
return 0;
}
int i = 0;
char ch[1024] = { 0 };
while (ifs.getline(ch, sizeof(ifs)))
{
i++;
}
ifs.close();
return i;
}
// 初始化员工
void workerManager_xy::mInitEmp() {
ifstream ifs;
ifs.open(FILEADDRESS, ios::in);
int id;
string name;
int tId;
int index = 0;
// 读取文件中的所有信息
while (ifs >> id && ifs >> name && ifs >> tId)
{
// 创建职工【讲读取的数据存在数组中】
mNewEmp(id, name, tId, index);
// 存在下标为多少的数组中的标志
index++;
}
// 关闭文件
ifs.close();
}
// 创建职工
void workerManager_xy::mNewEmp(int id, string name, int tId, int index) {
// 声明父类指针
worker_xy* worker = NULL;
// 判断这条信息职工的职称
switch (tId)
{
case 1: // 员工
worker = new employee_xy(id, name, tId);
break;
case 2: // 经理
worker = new manager_xy(id, name, tId);
break;
case 3: // 老板
worker = new boss_xy(id, name, tId);
break;
default: // 啥也不是
cout << "啥也不是" << endl;
break;
}
// 信息存入类型为worker_xy的数组中
this->mEmpArray[index] = worker;
}
// 显示职工信息
void workerManager_xy::mShowEmp() {
// 判断文件是否为空
if (this->mFileIsEmpty)
{
cout << "文件不存在或数据为空" << endl;
}
else
{
for (int i = 0; i < mEmpNum; i++)
{
// 利用多态调用程序接口【子类中打印职工信息】
this->mEmpArray[i]->mShowInfo();
// 显示的时候会有点问题,不是实时文件的内容
}
}
// 任意键后清屏
system("pause");
system("cls");
}
// 删除职工信息
void workerManager_xy::mDelEmp() {
// 判断文件是否存在或文件是否为空
if (this->mFileIsEmpty)
{
cout << "文件不存在或数据为空" << endl;
}
else
{
// 按照职工编号删除职工
cout << "请输入需要删除的职工编号:" << endl;
int id = 0;
cin >> id;
// 判断职是否存在
int index = this->mIsExist(id);
if (index != -1)
{
// 遍历数组长度 - 1
for (int i = index; i < this->mEmpNum - 1; i++)
{
// 数据迁移,覆盖需要删除的信息
this->mEmpArray[i] = this->mEmpArray[i + 1];
}
// 更新数组中的人数
this->mEmpNum--;
// 数据同步保存至文件中
this->mSave();
cout << "删除成功!" << endl;
}
else
{
cout << "删除失败,职工不存在!" << endl;
}
}
system("pause");
system("cls");
}
// 判断职工是否存在,如果存在返回职工所在位置,不存在返回-1
int workerManager_xy::mIsExist(int id) {
int index = -1;
for (int i = 0; i < this->mEmpNum; i++)
{
if (this->mEmpArray[i]->mId == id)
{
index = i;
break;
}
}
return index;
}
// 修改职工信息
void workerManager_xy::mEditEmp() {
// 判断文件是否为空
if (this->mFileIsEmpty)
{
cout << "文件不存在或数据为空!" << endl;
}
else
{
cout << "请输入需要修改的职工编号:" << endl;
int id;
cin >> id;
// 判断职工是否存在
int ret = this->mIsExist(id);
if (ret != -1)
{
// 先清空该条职工信息
delete this->mEmpArray[ret];
int sNewId = 0;
string sNewName;
int sNewTId = 0;
cout << "查到:" << id << "号职工,请输入新的职工号" << endl;
cin >> sNewId;
cout << "请输入新的职工姓名" << endl;
cin >> sNewName;
cout << "请选择新的职工职称" << endl;
cout << "1:员工" << endl;
cout << "2:经理" << endl;
cout << "3:老板" << endl;
cin >> sNewTId;
// 修改职工信息
this->mNewEmp(sNewId, sNewName, sNewTId, ret);
cout << "修改成功" << endl;
// 同步保存至文件中
this->mSave();
}
else
{
cout << "该编号职工信息不存在!" << endl;
}
}
system("pause");
system("cls");
}
// 查找职工信息
void workerManager_xy::mFindEmp() {
// 判断文件是否为空
if (this->mFileIsEmpty)
{
cout << "文件不存在或数据为空!" << endl;
}
else
{
cout << "请选择查找方式:" << endl;
cout << "1:按照职工编号查找" << endl;
cout << "2:按照职姓名号查找" << endl;
int sSelect = 0;
cin >> sSelect;
switch (sSelect)
{
case 1: // 按照职工编号查找
{
cout << "请输入查找的编号:" << endl;
int id = 0;
cin >> id;
// 判断这个人是否存在
int ret = this->mIsExist(id);
if (ret != -1)
{
cout << "查找成功!该职工信息如下:" << endl;
for (int i = 0; i < this->mEmpNum; i++)
{
// 判断查找的和需要是是否一致
if (this->mEmpArray[i]->mId == id)
{
// 利用多态打印职工信息
this->mEmpArray[ret]->mShowInfo();
}
}
}
else
{
cout << "查找用户不存在!" << endl;
}
}
break;
case 2: // 按照职姓名号查找
{
cout << "请输入查找的姓名:" << endl;
string name;
cin >> name;
int sNum = 0;
for (int i = 0; i < this->mEmpNum; i++)
{
// 判断查找的和需要是是否一致
if (this->mEmpArray[i]->mName == name)
{
// 利用多态打印职工信息
this->mEmpArray[i]->mShowInfo();
sNum++;
}
}
if (sNum != 0)
{
cout << "成功查找到" << sNum << "条职工信息!" << endl;
}
else
{
cout << "查找职工不存在!" << endl;
}
}
break;
default:
cout << "啥也不是!" << endl;
break;
}
}
system("pause");
system("cls");
}
// 排序职工【选择排序】
void workerManager_xy::mSortEmp() {
// 判断文件是否存在和是否为空
if (this->mFileIsEmpty)
{
cout << "文件不存在或数据为空!" << endl;
system("pause");
system("cls");
}
else
{
cout << "请选择排序的方式:" << endl;
cout << "1:按照职工号升序排列" << endl;
cout << "2:按照职工号降序排列" << endl;
int sSelect = 0;
cin >> sSelect;
int sMinOrMax = 0;
worker_xy* temp = NULL;
switch (sSelect)
{
case 1: // 升序排序
{
for (int i = 0; i < this->mEmpNum; i++)
{
// 假设 最小值在数组中的下标为 i
sMinOrMax = i;
// 遍历未确认的数据
for (int j = i; j < this->mEmpNum; j++)
{
// 判断假设的 id 是不是最小值
if (this->mEmpArray[sMinOrMax]->mId > this->mEmpArray[j]->mId)
{
// 如果不是最小值,就更新最小值的下标
sMinOrMax = j;
}
}
// 遍历比较完成后,选出当轮最小值 与 假设最小值进行交换
temp = this->mEmpArray[sMinOrMax];
this->mEmpArray[sMinOrMax] = this->mEmpArray[i];
this->mEmpArray[i] = temp;
}
// 然后打印打印职工信息
cout << "排序成功,升序排序信息如下:" << endl;
this->mShowEmp();
}
break;
case 2: // 降序排序
{
for (int i = 0; i < this->mEmpNum; i++)
{
// 假设 最大值在数组中的下标为 i
sMinOrMax = i;
// 遍历未确认的数据
for (int j = i; j < this->mEmpNum; j++)
{
// 判断假设的 id 是不是最大值
if (this->mEmpArray[sMinOrMax]->mId < this->mEmpArray[j]->mId)
{
// 如果不是最小值,就更新最小值的下标
sMinOrMax = j;
}
}
// 遍历比较完成后,选出当轮最小值 与 假设最小值进行交换
temp = this->mEmpArray[sMinOrMax];
this->mEmpArray[sMinOrMax] = this->mEmpArray[i];
this->mEmpArray[i] = temp;
}
// 然后打印打印职工信息
cout << "排序成功,降序排序信息如下:" << endl;
this->mShowEmp();
}
break;
default: // 默认排序
{
cout << "选择错误,默认排序信息如下:" << endl;
this->mShowEmp();
}
break;
}
}
}
// 清空数据
void workerManager_xy::mCleanFile() {
// 判断文件是否为空或数据为空
if (this->mFileIsEmpty)
{
cout << "文件不存在或数据为空!" << endl;
}
else
{
cout << "确定清空文件" << endl;
cout << "1:确定" << endl;
cout << "2:取消" << endl;
int sSelect = 0;
cin >> sSelect;
switch (sSelect)
{
case 1:
{
ofstream ofs;
// 这种写入文件的方式是先删除文件再创建
ofs.open(FILEADDRESS, ios::trunc);
// 不做写入的操作直接关闭文件,这样新的文件里面的内容就是空的
ofs.close();
// 然后清空一下储存职工信息的数组
if (this->mEmpArray != NULL)
{
// 遍历我们清空的指针所指向的数组
for (int i = 0; i < this->mEmpNum; i++)
{
// 判断该数组是否为空
if (this->mEmpArray[i] != NULL)
{
// 如果不为空就先释放数组
delete this->mEmpArray[i];
this->mEmpArray[i] = NULL;
}
}
// 然后释放这个数组
delete[] this->mEmpArray;
// 再把指针指向空
this->mEmpArray = NULL;
// 更新职工数量
this->mEmpNum = 0;
// 更新文件是否存在标准
this->mFileIsEmpty = true;
cout << "文件清空成功!" << endl;
}
}
break;
case 2:
{
cout << "取消清空文件!" << endl;
}
break;
default:
cout << "咋就不能按照提示进行选择呢!" << endl;
break;
}
}
system("pause");
system("cls");
}
// 管理类的析构函数
workerManager_xy::~workerManager_xy() {
//
if (this->mEmpArray != NULL)
{
// 遍历我们清空的指针所指向的数组
for (int i = 0; i < this->mEmpNum; i++)
{
// 判断该数组是否为空
if (this->mEmpArray[i] != NULL)
{
// 如果不为空就先释放数组
delete this->mEmpArray[i];
this->mEmpArray[i] = NULL;
}
}
// 然后释放这个数组
delete[] this->mEmpArray;
// 最后再把指针指向空
this->mEmpArray = NULL;
}
}
2.3、employee_xy.cpp
#include "employee_xy.h"
// 普通职工类的构造函数
employee_xy::employee_xy(int id, string name, int tId) {
// 初始化
this->mId = id;
this->mName = name;
this->mTitle = tId;
}
// 显示个人信息
void employee_xy::mShowInfo() {
cout << "职工编号:" << this->mId << " "
<< "职工姓名:" << this->mName << " "
<< "职工职称:" << this->mGetTitleName() << " "
<< "工作内容:完成经理指派的任务!" << endl;
}
// 获取岗位名称
string employee_xy::mGetTitleName() {
return string("员工");
}
2.4、manager_xy.cpp
#include "manager_xy.h"
// 经理类类的构造函数
manager_xy::manager_xy(int id, string name, int tId) {
// 初始化
this->mId = id;
this->mName = name;
this->mTitle = tId;
}
// 显示个人信息
void manager_xy::mShowInfo() {
cout << "职工编号:" << this->mId << " "
<< "职工姓名:" << this->mName << " "
<< "职工职称:" << this->mGetTitleName() << " "
<< "工作内容:完成老板分配的任务,并给员工分配任务" << endl;
}
// 获取岗位名称
string manager_xy::mGetTitleName() {
return string("经理");
}
2.5、boss_xy.cpp
#include "boss_xy.h"
// 老板类的构造函数
boss_xy::boss_xy(int id, string name, int tId) {
// 初始化
this->mId = id;
this->mName = name;
this->mTitle = tId;
}
// 显示个人信息
void boss_xy::mShowInfo() {
cout << "职工编号:" << this->mId << " "
<< "职工姓名:" << this->mName << " "
<< "职工职称:" << this->mGetTitleName() << " "
<< "工作内容:做好公司规划,给经理分配任务" << endl;
}
// 获取岗位名称
string boss_xy::mGetTitleName() {
return string("老板");
}
一点点笔记,以便以后翻阅。
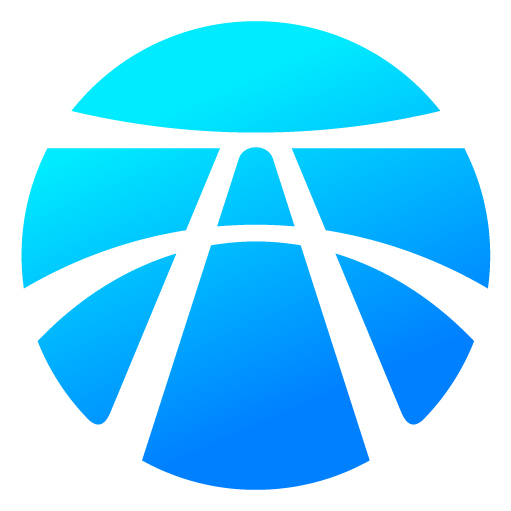
开放原子开发者工作坊旨在鼓励更多人参与开源活动,与志同道合的开发者们相互交流开发经验、分享开发心得、获取前沿技术趋势。工作坊有多种形式的开发者活动,如meetup、训练营等,主打技术交流,干货满满,真诚地邀请各位开发者共同参与!
更多推荐
所有评论(0)