mall整合Elasticsearch实现商品搜索
mall整合Elasticsearch实现商品搜索1、依赖1.1、启动ES服务下载ES:https://www.elastic.co/cn/downloads/past-releases/elasticsearch-6-2-2中文分词器(bin下cmd):elasticsearch-plugin install https://github.com/medcl/elasticsearch-anal
·
mall整合Elasticsearch实现商品搜索
1、依赖
1.1、启动ES服务
下载ES:https://www.elastic.co/cn/downloads/past-releases/elasticsearch-6-2-2
中文分词器(bin下cmd):elasticsearch-plugin install https://github.com/medcl/elasticsearch-analysis-ik/releases/download/v6.2.2/elasticsearch-analysis-ik-6.2.2.zip
ES客户端:ch-plugin install https://github.com/medcl/elasticsearch-analysis-ik/releases/download/v6.2.2/elasticsearch-analysis-ik-6.2.2.zip
1.2、pom依赖
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.1.3.RELEASE</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<groupId>com.wnx.mall.tiny</groupId>
<artifactId>mall-tiny-06</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>mall-tiny-06</name>
<description>Demo project for Spring Boot</description>
<properties>
<java.version>1.8</java.version>
</properties>
<dependencies>
<!--SpringBoot通用依赖模块-->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-aop</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-configuration-processor</artifactId>
</dependency>
<!--MyBatis分页插件-->
<dependency>
<groupId>com.github.pagehelper</groupId>
<artifactId>pagehelper-spring-boot-starter</artifactId>
<version>1.2.10</version>
</dependency>
<!--集成druid连接池-->
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>druid-spring-boot-starter</artifactId>
<version>1.1.10</version>
</dependency>
<!-- MyBatis 生成器 -->
<dependency>
<groupId>org.mybatis.generator</groupId>
<artifactId>mybatis-generator-core</artifactId>
<version>1.3.3</version>
</dependency>
<!--Mysql数据库驱动-->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.15</version>
</dependency>
<!--Swagger-UI API文档生产工具-->
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger2</artifactId>
<version>2.7.0</version>
</dependency>
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger-ui</artifactId>
<version>2.7.0</version>
</dependency>
<!--redis依赖配置-->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
<!--SpringSecurity依赖配置-->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
<!--Hutool Java工具包-->
<dependency>
<groupId>cn.hutool</groupId>
<artifactId>hutool-all</artifactId>
<version>4.5.7</version>
</dependency>
<!--JWT(Json Web Token)登录支持-->
<dependency>
<groupId>io.jsonwebtoken</groupId>
<artifactId>jjwt</artifactId>
<version>0.9.0</version>
</dependency>
<!--Elasticsearch相关依赖-->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-elasticsearch</artifactId>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
1.3、application.yaml配置
server:
port: 8080
spring:
datasource:
url: jdbc:mysql://localhost:3306/mall?useUnicode=true&characterEncoding=utf-8&serverTimezone=Asia/Shanghai
username: root
password: root
redis:
host: localhost # Redis服务器地址
database: 0 # Redis数据库索引(默认为0)
port: 6379 # Redis服务器连接端口
password: # Redis服务器连接密码(默认为空)
jedis:
pool:
max-active: 8 # 连接池最大连接数(使用负值表示没有限制)
max-wait: -1ms # 连接池最大阻塞等待时间(使用负值表示没有限制)
max-idle: 8 # 连接池中的最大空闲连接
min-idle: 0 # 连接池中的最小空闲连接
timeout: 3000ms # 连接超时时间(毫秒)
data:
elasticsearch:
repositories:
enabled: true
cluster-nodes: 127.0.0.1:9300 # es的连接地址及端口号
cluster-name: elasticsearch # es集群的名称
mybatis:
mapper-locations:
- classpath:mapper/*.xml
- classpath*:com/**/mapper/*.xml
# 自定义redis key
redis:
key:
prefix:
authCode: "portal:authCode:"
expire:
authCode: 120 # 验证码超期时间
# 自定义jwt key
jwt:
tokenHeader: Authorization #JWT存储的请求头
secret: mySecret #JWT加解密使用的密钥
expiration: 604800 #JWT的超期限时间(60*60*24)
tokenHead: Bearer #JWT负载中拿到开头
2、定义模型和接口
2.1、模型
- 搜索中的商品信息
package com.wnx.mall.tiny.nosql.document;
import com.wnx.mall.tiny.nosql.document.EsProductAttributeValue;
import org.springframework.data.annotation.Id;
import org.springframework.data.elasticsearch.annotations.Document;
import org.springframework.data.elasticsearch.annotations.Field;
import org.springframework.data.elasticsearch.annotations.FieldType;
import java.io.Serializable;
import java.math.BigDecimal;
import java.util.List;
/**
* 搜索中的商品信息
* Created by macro on 2018/6/19.
*/
@Document(indexName = "pms", type = "product",shards = 1,replicas = 0)
public class EsProduct implements Serializable {
private static final long serialVersionUID = -1L;
@Id
private Long id;
@Field(type = FieldType.Keyword)
private String productSn;
private Long brandId;
@Field(type = FieldType.Keyword)
private String brandName;
private Long productCategoryId;
@Field(type = FieldType.Keyword)
private String productCategoryName;
private String pic;
@Field(analyzer = "ik_max_word",type = FieldType.Text)
private String name;
@Field(analyzer = "ik_max_word",type = FieldType.Text)
private String subTitle;
@Field(analyzer = "ik_max_word",type = FieldType.Text)
private String keywords;
private BigDecimal price;
private Integer sale;
private Integer newStatus;
private Integer recommandStatus;
private Integer stock;
private Integer promotionType;
private Integer sort;
@Field(type =FieldType.Nested)
private List<EsProductAttributeValue> attrValueList;
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public String getProductSn() {
return productSn;
}
public void setProductSn(String productSn) {
this.productSn = productSn;
}
public Long getBrandId() {
return brandId;
}
public void setBrandId(Long brandId) {
this.brandId = brandId;
}
public String getBrandName() {
return brandName;
}
public void setBrandName(String brandName) {
this.brandName = brandName;
}
public Long getProductCategoryId() {
return productCategoryId;
}
public void setProductCategoryId(Long productCategoryId) {
this.productCategoryId = productCategoryId;
}
public String getProductCategoryName() {
return productCategoryName;
}
public void setProductCategoryName(String productCategoryName) {
this.productCategoryName = productCategoryName;
}
public String getPic() {
return pic;
}
public void setPic(String pic) {
this.pic = pic;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getSubTitle() {
return subTitle;
}
public void setSubTitle(String subTitle) {
this.subTitle = subTitle;
}
public BigDecimal getPrice() {
return price;
}
public void setPrice(BigDecimal price) {
this.price = price;
}
public Integer getSale() {
return sale;
}
public void setSale(Integer sale) {
this.sale = sale;
}
public Integer getNewStatus() {
return newStatus;
}
public void setNewStatus(Integer newStatus) {
this.newStatus = newStatus;
}
public Integer getRecommandStatus() {
return recommandStatus;
}
public void setRecommandStatus(Integer recommandStatus) {
this.recommandStatus = recommandStatus;
}
public Integer getStock() {
return stock;
}
public void setStock(Integer stock) {
this.stock = stock;
}
public Integer getPromotionType() {
return promotionType;
}
public void setPromotionType(Integer promotionType) {
this.promotionType = promotionType;
}
public Integer getSort() {
return sort;
}
public void setSort(Integer sort) {
this.sort = sort;
}
public List<EsProductAttributeValue> getAttrValueList() {
return attrValueList;
}
public void setAttrValueList(List<EsProductAttributeValue> attrValueList) {
this.attrValueList = attrValueList;
}
public String getKeywords() {
return keywords;
}
public void setKeywords(String keywords) {
this.keywords = keywords;
}
}
- 搜索中的商品属性信息
package com.wnx.mall.tiny.nosql.document;
import org.springframework.data.elasticsearch.annotations.Field;
import org.springframework.data.elasticsearch.annotations.FieldType;
import java.io.Serializable;
/**
* 搜索中的商品属性信息
* Created by macro on 2018/6/27.
*/
public class EsProductAttributeValue implements Serializable {
private static final long serialVersionUID = 1L;
private Long id;
private Long productAttributeId;
//属性值
@Field(type = FieldType.Keyword)
private String value;
//属性参数:0->规格;1->参数
private Integer type;
//属性名称
@Field(type=FieldType.Keyword)
private String name;
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public Long getProductAttributeId() {
return productAttributeId;
}
public void setProductAttributeId(Long productAttributeId) {
this.productAttributeId = productAttributeId;
}
public String getValue() {
return value;
}
public void setValue(String value) {
this.value = value;
}
public Integer getType() {
return type;
}
public void setType(Integer type) {
this.type = type;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
2.2、接口
package com.wnx.mall.tiny.nosql.repository;
import com.wnx.mall.tiny.nosql.document.EsProduct;
import org.springframework.data.domain.Page;
import org.springframework.data.domain.Pageable;
import org.springframework.data.elasticsearch.repository.ElasticsearchRepository;
/**
* 商品ES操作类
* Created by macro on 2018/6/19.
*/
public interface EsProductRepository extends ElasticsearchRepository<EsProduct, Long> {
/**
* 搜索查询
*
* @param name 商品名称
* @param subTitle 商品标题
* @param keywords 商品关键字
* @param page 分页信息
* @return
*/
Page<EsProduct> findByNameOrSubTitleOrKeywords(String name, String subTitle, String keywords, Pageable page);
}
3、Service
package com.wnx.mall.tiny.service;
import com.wnx.mall.tiny.nosql.document.EsProduct;
import org.springframework.data.domain.Page;
import java.util.List;
public interface EsProductService {
/**
* 从数据库中导入所有商品到ES
*/
int importAll();
/**
* 根据id删除商品
*/
void delete(Long id);
/**
* 根据id创建商品
*/
EsProduct create(Long id);
/**
* 批量删除商品
*/
void delete(List<Long> ids);
/**
* 根据关键字搜索名称或者副标题
*/
Page<EsProduct> search(String keyword, Integer pageNum, Integer pageSize);
}
3.1、ServiceImpl
package com.wnx.mall.tiny.service.impl;
import cn.hutool.core.collection.CollUtil;
import com.wnx.mall.tiny.dao.EsProductDao;
import com.wnx.mall.tiny.nosql.document.EsProduct;
import com.wnx.mall.tiny.nosql.repository.EsProductRepository;
import com.wnx.mall.tiny.service.EsProductService;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.data.domain.Page;
import org.springframework.data.domain.PageRequest;
import org.springframework.data.domain.Pageable;
import org.springframework.stereotype.Service;
import javax.annotation.Resource;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
@Service
public class EsProductServiceImpl implements EsProductService {
@Resource
private EsProductDao productDao;
@Resource
private EsProductRepository productRepository;
private static final Logger LOGGER = LoggerFactory.getLogger(EsProductServiceImpl.class);
@Override
public int importAll() {
List<EsProduct> allEsProductList = productDao.getAllEsProductList(null);
Iterable<EsProduct> iterable = productRepository.saveAll(allEsProductList);
Iterator<EsProduct> iterator = iterable.iterator();
int result = 0;
while (iterator.hasNext()){
result++;
iterator.next();
}
return result;
}
@Override
public void delete(Long id) {
productRepository.deleteById(id);
}
@Override
public EsProduct create(Long id) {
EsProduct result = null;
List<EsProduct> esProductList = productDao.getAllEsProductList(id);
if (esProductList.size()>0){
EsProduct esProduct = esProductList.get(0);
result = productRepository.save(esProduct);
}
return result;
}
@Override
public void delete(List<Long> ids) {
if (CollUtil.isNotEmpty(ids)){
List<EsProduct> esProductList = new ArrayList<>();
ids.forEach(id->{
EsProduct esProduct = new EsProduct();
esProduct.setId(id);
esProductList.add(esProduct);
});
productRepository.deleteAll(esProductList);
}
}
@Override
public Page<EsProduct> search(String keyword, Integer pageNum, Integer pageSize) {
Pageable pageable = PageRequest.of(pageNum,pageSize);
return productRepository.findByNameOrSubTitleOrKeywords(keyword,keyword,keyword,pageable);
}
}
4、DAO
package com.wnx.mall.tiny.dao;
import com.wnx.mall.tiny.nosql.document.EsProduct;
import org.apache.ibatis.annotations.Param;
import java.util.List;
/**
* 搜索系统中的商品管理自定义Dao
* Created by macro on 2018/6/19.
*/
public interface EsProductDao {
List<EsProduct> getAllEsProductList(@Param("id") Long id);
}
4.1、Mapper.xml
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.wnx.mall.tiny.dao.EsProductDao">
<resultMap id="esProductListMap" type="com.wnx.mall.tiny.nosql.document.EsProduct" autoMapping="true">
<id column="id" property="id"/>
<collection property="attrValueList" columnPrefix="attr_" ofType="com.wnx.mall.tiny.nosql.document.EsProductAttributeValue">
<id column="id" property="id"/>
<result column="product_attribute_id" property="productAttributeId"/>
<result column="value" property="value"/>
<result column="type" property="type"/>
<result column="name" property="name"/>
</collection>
</resultMap>
<select id="getAllEsProductList" resultMap="esProductListMap">
select
p.id as id,
p.product_sn as productSn,
p.brand_id as brandId,
p.brand_name as brandName,
p.product_category_id as productCategoryId,
p.product_category_name as productCategoryName,
p.pic as pic,
p.name as name,
p.sub_title as subTitle,
p.price as price,
p.sale as sale,
p.new_status as newStatus,
p.recommand_status as recommandStatus,
p.stock as stock,
p.promotion_type as promotionType,
p.keywords as keywords,
p.sort as sort,
pav.id as attr_id,
pav.value as attr_value,
pav.product_attribute_id as attr_product_attribute_id,
pa.type as attr_type,
pa.name as attr_name
from pms_product p
left join pms_product_attribute_value pav on p.id =pav.product_id
left join pms_product_attribute pa on pav.product_attribute_id = pa.id
where delete_status = 0 and publish_status = 1
<if test="id !=null">
and p.id = #{id}
</if>
</select>
</mapper>
5、Controller
package com.wnx.mall.tiny.controller;
import com.wnx.mall.tiny.common.api.CommonPage;
import com.wnx.mall.tiny.common.api.CommonResult;
import com.wnx.mall.tiny.nosql.document.EsProduct;
import com.wnx.mall.tiny.service.EsProductService;
import io.swagger.annotations.Api;
import io.swagger.annotations.ApiOperation;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.domain.Page;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.*;
import java.util.List;
/**
* 搜索商品管理Controller
* Created by macro on 2018/6/19.
*/
@Controller
@Api(tags = "EsProductController", description = "搜索商品管理")
@RequestMapping("/esProduct")
public class EsProductController {
@Autowired
private EsProductService esProductService;
@ApiOperation(value = "导入所有数据库中商品到ES")
@RequestMapping(value = "/importAll", method = RequestMethod.POST)
@ResponseBody
public CommonResult<Integer> importAllList() {
int count = esProductService.importAll();
return CommonResult.success(count);
}
@ApiOperation(value = "根据id删除商品")
@RequestMapping(value = "/delete/{id}", method = RequestMethod.GET)
@ResponseBody
public CommonResult<Object> delete(@PathVariable Long id) {
esProductService.delete(id);
return CommonResult.success(null);
}
@ApiOperation(value = "根据id批量删除商品")
@RequestMapping(value = "/delete/batch", method = RequestMethod.POST)
@ResponseBody
public CommonResult<Object> delete(@RequestParam("ids") List<Long> ids) {
esProductService.delete(ids);
return CommonResult.success(null);
}
@ApiOperation(value = "根据id创建商品")
@RequestMapping(value = "/create/{id}", method = RequestMethod.POST)
@ResponseBody
public CommonResult<EsProduct> create(@PathVariable Long id) {
EsProduct esProduct = esProductService.create(id);
if (esProduct != null) {
return CommonResult.success(esProduct);
} else {
return CommonResult.failed();
}
}
@ApiOperation(value = "简单搜索")
@RequestMapping(value = "/search/simple", method = RequestMethod.GET)
@ResponseBody
public CommonResult<CommonPage<EsProduct>> search(@RequestParam(required = false) String keyword,
@RequestParam(required = false, defaultValue = "0") Integer pageNum,
@RequestParam(required = false, defaultValue = "5") Integer pageSize) {
Page<EsProduct> esProductPage = esProductService.search(keyword, pageNum, pageSize);
return CommonResult.success(CommonPage.restPage(esProductPage));
}
}
5.1、接口测试
接口地址:http://localhost:8080/swagger-ui.html
5.2、客户端操作ES
- 访问http://localhost:5601 即可打开Kibana的用户界面
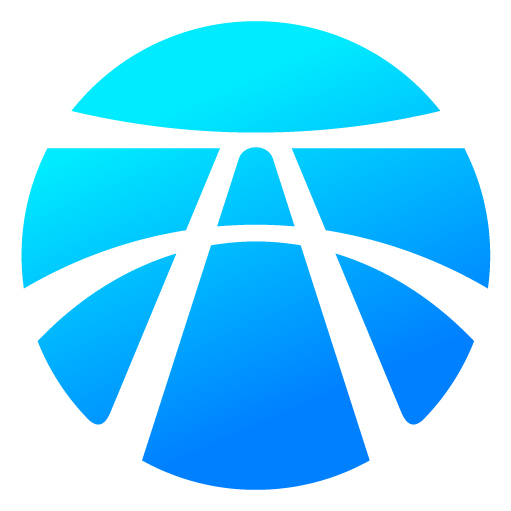
开放原子开发者工作坊旨在鼓励更多人参与开源活动,与志同道合的开发者们相互交流开发经验、分享开发心得、获取前沿技术趋势。工作坊有多种形式的开发者活动,如meetup、训练营等,主打技术交流,干货满满,真诚地邀请各位开发者共同参与!
更多推荐
所有评论(0)