leetcode---Additive Number
Additive number is a string whose digits can form additive sequence.A valid additive sequence should contain at least three numbers. Except for the first two numbers, each subsequent number in the seq
Additive number is a string whose digits can form additive sequence.
A valid additive sequence should contain at least three numbers. Except for the first two numbers, each subsequent number in the sequence must be the sum of the preceding two.
For example:
“112358” is an additive number because the digits can form an additive sequence: 1, 1, 2, 3, 5, 8.
1 + 1 = 2, 1 + 2 = 3, 2 + 3 = 5, 3 + 5 = 8
“199100199” is also an additive number, the additive sequence is: 1, 99, 100, 199.
1 + 99 = 100, 99 + 100 = 199
Note: Numbers in the additive sequence cannot have leading zeros, so sequence 1, 2, 03 or 1, 02, 3 is invalid.
Given a string containing only digits ‘0’-‘9’, write a function to determine if it’s an additive number.
Follow up:
How would you handle overflow for very large input integers?
class Solution {
public:
bool isAdditiveNumber(string num)
{
int n = num.size();
for(int i=0; i<n; i++)
{
string s1 = num.substr(0, i);
for(int j=i+1; j<n; j++)
{
string s2 = num.substr(i, j-i);
if((s1.size() > 1 && s1[0] == '0') || (s2.size() > 1 && s2[0] == '0'))
continue;
long a1 = atoll(s1.c_str());
long a2 = atoll(s2.c_str());
long r = a1 + a2;
string rs = to_string(r);
string cur = s1 + s2 + rs;
while(cur.size() < n)
{
a1 = a2;
a2 = r;
r = a1 + a2;
rs = to_string(r);
cur += rs;
}
if(cur == num)
return true;
}
}
return false;
}
};
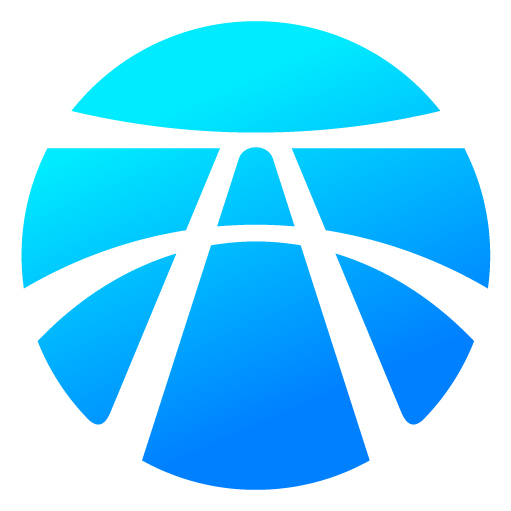
开放原子开发者工作坊旨在鼓励更多人参与开源活动,与志同道合的开发者们相互交流开发经验、分享开发心得、获取前沿技术趋势。工作坊有多种形式的开发者活动,如meetup、训练营等,主打技术交流,干货满满,真诚地邀请各位开发者共同参与!
更多推荐
所有评论(0)