handy:daemon.cc
https://github.com/yedf/handyExitCallernamespace handy {namespace {struct ExitCaller {~ExitCaller() { functor_(); }ExitCaller(std::function<void()>&& functor): functor_(std::move(functor)) {
daemon.cc
ExitCaller
namespace handy {
namespace {
struct ExitCaller {
~ExitCaller() { functor_(); }
ExitCaller(std::function<void()>&& functor): functor_(std::move(functor)) {}
private:
std::function<void()> functor_;
};
}
...
}
ExitCaller是一个RAII类,利用栈上对象离开作用域时析构来“搞事情”。它使用了右值引用和std::move。(那个没有名字的namespace有什么作用?是用来代码归类?)
思考:是否可以去掉std::move?
答案是不可以的,因为右值引用本身是一个左值,如果不使用move,传递给functor_的参数是一个左值,会调用std::function的拷贝构造函数,而不是移动拷贝构造函数。Example:
class T {
public:
T(int n) : n_(n) {}
T(const T& t) : n_(t.n_) {
std::cout << "copy ctor" << std::endl;
}
T(T&& t) noexcept: n_(t.n_) {
std::cout << "move copy ctor" << std::endl;
}
private :
int n_;
};
struct ExitCaller {
~ExitCaller() {}
ExitCaller(T&& t) : t_(std::move(t)) {} // output: move copy ctor
//ExitCaller(T&& t) : t_(t) {} // output: copy ctor
private:
T t_;
};
int main()
{
{
T t(0x10);
ExitCaller ec(std::move(t));
}
return 0;
}
可以参考std::move的帮助文档:
http://en.cppreference.com/w/cpp/utility/move
Names of rvalue reference variables are lvalues and have to be converted to xvalues to be bound to the function overloads that accept rvalue reference parameters, which is why move constructors and move assignment operators typically use std::move:
// Simple move constructor A(A&& arg) : member(std::move(arg.member)) // the expression "arg.member" is lvalue {} // Simple move assignment operator A& operator=(A&& other) { member = std::move(other.member); return *this; }
此外可以使用typedef或using简化functor的类型:
using ExitFunc = std::function<void()>;
typedef std::function<void()> ExitFunc;
Signal
namespace {
map<int, function<void()>> handlers;
void signal_handler(int sig) {
handlers[sig]();
}
}
void Signal::signal(int sig, const function<void()>& handler) {
handlers[sig] = handler;
::signal(sig, signal_handler);
}
signal是对信号的封装。使用map容器来管理信号与处理函数的对应关系。
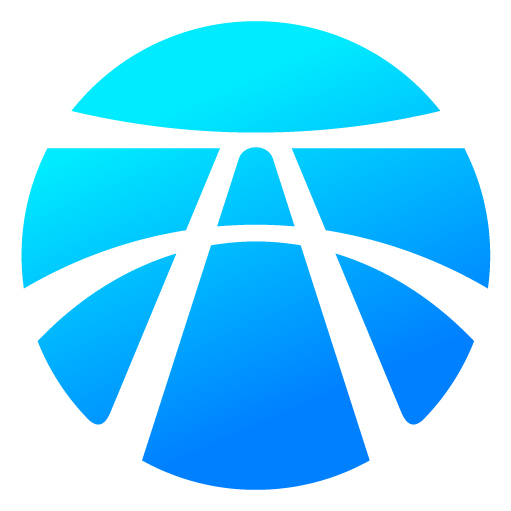
开放原子开发者工作坊旨在鼓励更多人参与开源活动,与志同道合的开发者们相互交流开发经验、分享开发心得、获取前沿技术趋势。工作坊有多种形式的开发者活动,如meetup、训练营等,主打技术交流,干货满满,真诚地邀请各位开发者共同参与!
更多推荐
所有评论(0)