雪花算法
package mainimport (“fmt”“github.com/bwmarrin/snowflake”“os”“reflect”“time”)//Aa、Bb、Cc、Dd、Ee、Ff、Gg、Hh、Ii、Jj、Kk、Ll、Mm、Nn、Oo、Pp、Qq、Rr、Ss、Tt、Uu、Vv、Ww、Xx、Yy、Zz//IJjkKLMNO5//67PQX12RVW//3YZaDEFGbc//defghiA
·
package main
import (
"fmt"
"github.com/bwmarrin/snowflake"
"os"
"reflect"
"time"
)
//Aa、Bb、Cc、Dd、Ee、Ff、Gg、Hh、Ii、Jj、Kk、Ll、Mm、Nn、Oo、Pp、Qq、Rr、Ss、Tt、Uu、Vv、Ww、Xx、Yy、Zz
//IJjkKLMNO5
//67PQX12RVW
//3YZaDEFGbc
//defghiABCH
//lSTUmnopqr
//xyz04stuvw
//89+/
var (
EncodeMapping = map[string]string{
"A": "I", "B": "J", "C": "j", "D": "k", "E": "K", "F": "L", "G": "M", "H": "N", "I": "O", "J": "5", "K": "6",
"L": "7", "M": "P", "N": "Q", "O": "X", "P": "1", "Q": "2", "R": "R", "S": "V", "T": "W", "U": "3", "V": "Y",
"W": "Z", "X": "a", "Y": "D", "Z": "E", "a": "F", "b": "G", "c": "b", "d": "c", "e": "d", "f": "e", "g": "f",
"h": "g", "i": "h", "j": "i", "k": "A", "l": "B", "m": "C", "n": "H", "o": "l", "p": "S", "q": "T", "r": "U",
"s": "m", "t": "n", "u": "o", "v": "p", "w": "q", "x": "r", "y": "x", "z": "y", "0": "z", "1": "0", "2": "4",
"3": "s", "4": "t", "5": "u", "6": "v", "7": "w", "8": "8", "9": "9", "=": "-", "|": "%", "_": "@",
}
DecodeMapping = map[string]string{
"I": "A", "J": "B", "j": "C", "k": "D", "K": "E", "L": "F", "M": "G", "N": "H", "O": "I", "5": "J", "6": "K",
"7": "L", "P": "M", "Q": "N", "X": "O", "1": "P", "2": "Q", "R": "R", "V": "S", "W": "T", "3": "U", "Y": "V",
"Z": "W", "a": "X", "D": "Y", "E": "Z", "F": "a", "G": "b", "b": "c", "c": "d", "d": "e", "e": "f", "f": "g",
"g": "h", "h": "i", "i": "j", "A": "k", "B": "l", "C": "m", "H": "n", "l": "o", "S": "p", "T": "q", "U": "r",
"m": "s", "n": "t", "o": "u", "p": "v", "q": "w", "r": "x", "x": "y", "y": "z", "z": "0", "0": "1", "4": "2",
"s": "3", "t": "4", "u": "5", "v": "6", "w": "7", "8": "8", "9": "9", "-": "=", "%": "|", "@": "_",
}
)
func GetEncodeLeads(e string) (LeadsStr string) {
for _, val := range e {
LeadsStr += EncodeMapping[string(val)]
}
return
}
func GetDecodeLeads(e string) (LeadsinfoStr string) {
for _, val := range e {
LeadsinfoStr += DecodeMapping[string(val)]
}
return
}
type aa struct {
a string
b int64
c string
d int64
}
type A struct {
name string
age int
}
func (a A) IsEmpty() bool {
return reflect.DeepEqual(a, A{})
}
func main() {
times := "2020-09-18 15:04:05"
s, _ := time.Parse("2006-01-02 15:04:05", times)
fmt.Println(s.Year())
fmt.Println(int(s.Month()))
fmt.Println(s.Day())
fmt.Println(s.Date())
os.Exit(12)
var a A
a.name = "aa"
if a == (A{}) { // 括号不能去
fmt.Println("a == A{} empty")
}
if a.IsEmpty() {
fmt.Println("reflect deep is empty")
}
os.Exit(1)
as := map[int64]*aa{
11: &aa{
a: "A",
b: 1,
c: "",
d: 0,
},
}
for index, v := range as {
//type sf map[int64]aa
//var aaa sf = map[int64]aa{
// index: aa{
// c: "nn",
// },
//}
v.c = "nn"
fmt.Printf("%#v", as[index])
}
//fmt.Println(as)
//os.Exit(12)
for _, v := range as {
fmt.Println(v)
}
os.Exit(124)
//a := 15
//b := 45
//
//fmt.Println(a & b)
//os.Exit(12)
// Create a new Node with a Node number of 1
//创建一个节点号为1的新节点
node, err := snowflake.NewNode(1)
//fmt.Println(node)
if err != nil {
fmt.Println(err)
return
}
// Generate a snowflake ID.
//生成 一个雪花ID
//id := node.Generate()
//fmt.Println(id)
Print out the ID in a few different ways.
//fmt.Printf("Int64 ID: %d\n", id)
//fmt.Printf("String ID: %s\n", id)
//1001000001
//1001111110
//0111000011
//1101000100
//0000000010
//0000000000
//0
//fmt.Printf("Base2 ID: %s\n", id.Base2())
MTMwMDY5Mj
QzOTYwMTc3
ODY4OA== 28位
//fmt.Printf("Base64 ID: %s\n", id.Base64())
//
Print out the ID's timestamp
//fmt.Printf("ID Time : %d\n", id.Time())
//
Print out the ID's node number
//fmt.Printf("ID Node : %d\n", id.Node())
//
Print out the ID's sequence number
//fmt.Printf("ID Step : %d\n", id.Step())
//
Generate and print, all in one.
fmt.Printf("ID : %d\n", node.Generate().IntBytes())
fmt.Printf("ID1 : %d\n", node.Generate().Base64())
fmt.Printf("ID2 : %d\n", node.Generate().Base32())
fmt.Printf("ID2 : %d\n", node.Generate().Base2())
//fmt.Printf("ID3 : %d\n", node.Generate().Int64())
//IJjkKLMNO567PQX12RVW3YZaDEFGbcde*fghiABCHlSTUmnopqrxyz04stuvw89+/
e := node.Generate().Base64()
//转码
e += "|leads_public2020"
fmt.Println("原始data", e)
str := GetEncodeLeads(e)
fmt.Println("加密data", str)
l := GetDecodeLeads(str)
fmt.Println("解密data", l)
//go GetEncodeLeads(e)
//go GetEncodeLeads(e)
}
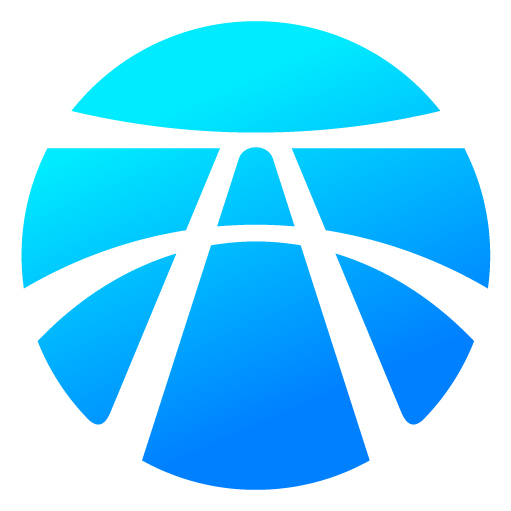
开放原子开发者工作坊旨在鼓励更多人参与开源活动,与志同道合的开发者们相互交流开发经验、分享开发心得、获取前沿技术趋势。工作坊有多种形式的开发者活动,如meetup、训练营等,主打技术交流,干货满满,真诚地邀请各位开发者共同参与!
更多推荐
所有评论(0)