System.Reflection 反射技术实例.
项目结构:ReflectionExample.csproj-HelloWorld.cs-Program.cs源代码:HelloWorld.csusing System;using System.Collections.Generic;using System.Text;namespace ReflectionExample{ class HelloWorld
项目结构:
ReflectionExample.csproj
-HelloWorld.cs
-Program.cs
源代码:
HelloWorld.cs
using System;
using System.Collections.Generic;
using System.Text;
namespace ReflectionExample
{
class HelloWorld
{
string myName = null;
public HelloWorld(string name)
{
myName = name;
}
public HelloWorld()
: this(null)
{
}
public string Name
{
get { return myName; }
}
public void SayHello()
{
if (myName == null)
System.Console.WriteLine("Hello World");
else
System.Console.WriteLine("Hello," + myName);
}
}
}
Program.cs
using System;
using System.Collections.Generic;
using System.Text;
using System.Reflection;
namespace ReflectionExample
{
class Program
{
static void Main(string[] args)
{
BindingFlags flags = (BindingFlags.Public | BindingFlags.Static | BindingFlags.Instance | BindingFlags.DeclaredOnly);
System.Console.WriteLine("列出程序集中的所有类型");
Assembly a = Assembly.LoadFrom("ReflectionExample.exe");
Type[] mytypes = a.GetTypes();
foreach (Type t in mytypes)
{
System.Console.WriteLine(t.Name);
}
System.Console.ReadLine();
System.Console.WriteLine("列出HelloWorld类中的所有方法");
Type ht = typeof(HelloWorld);
MethodInfo[] mif = ht.GetMethods(flags);
foreach (MethodInfo mf in mif)
{
System.Console.WriteLine(mf.Name);
}
System.Console.ReadLine();
System.Console.WriteLine("实例化HelloWorld,并调用SayHello方法");
Object obj = Activator.CreateInstance(ht);
String[] s ={ "ZhenLei" };
Object objName = Activator.CreateInstance(ht, s);
MethodInfo msayhello = ht.GetMethod("SayHello",flags);
msayhello.Invoke(obj, null);
msayhello.Invoke(objName,null);
System.Console.ReadLine();
}
}
}
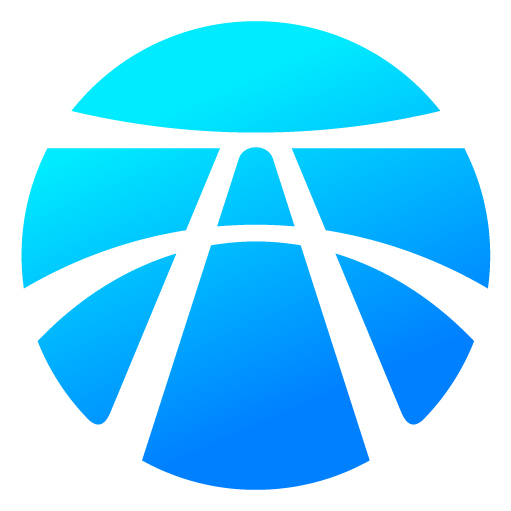
开放原子开发者工作坊旨在鼓励更多人参与开源活动,与志同道合的开发者们相互交流开发经验、分享开发心得、获取前沿技术趋势。工作坊有多种形式的开发者活动,如meetup、训练营等,主打技术交流,干货满满,真诚地邀请各位开发者共同参与!
更多推荐
所有评论(0)