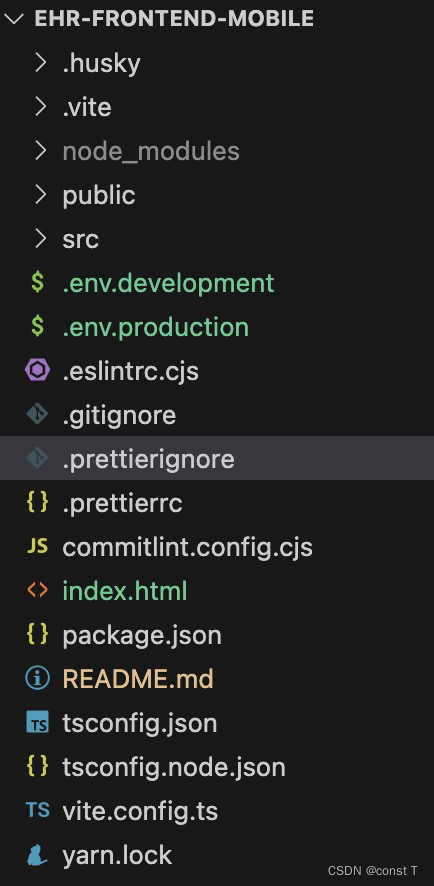
前端项目git、ESLint、prettier基本配置(前端工程化)
在 Git 中分为客户端钩子和服务端钩子,客户端钩子被用户操作触发,例如 commit,服务端钩子被网络动作触发,例如 pushed。
一、githooks 前端工程化
在 Git 中分为客户端钩子和服务端钩子,客户端钩子被用户操作触发,例如 commit,服务端钩子被网络动作触发,例如 pushed
https://juejin.cn/post/7174992870313574456#heading-7
客户端常用 hook
1.pre-commit
在 commit 动作完成前触发此 hook,可以用此 hook 检查代码风格、代码规范,或者运行自动化测试。当返回非零值时 Git 会放弃本次提交,也可以使用 git commit --no-verify 忽略,跳过本次 hook 的执行。
2.prepare-commit-msg
当 commit 时需要输入 message 前会触发,可以用此 hook 来定制自己的 default message 信息。该 hook 接收一个包含提交信息的文件名的参数,当返回非零值时 Git 会放弃本次提交。
3.commit-msg
当用户输入 commit 的 message 后被触发,可以用此 hook 校验 message 的信息,比如格式是否符合规范等。该 hook 接收一个包含提交信息的文件名的参数,当返回非零值时 Git 会放弃本次提交。
4.post-commit
在 commit 动作完成后被触发,可以用此 hook 推送消息等。
服务端常用 hook
1.pre-receive
当服务端收到 push 动作之前会被执行。
2.post-receive
当服务端收到 push 动作并且已经完成的时候会被触发,可以用此 hook 来推送消息,比如发邮件,通知持续构建服务器等。
配置 githooks
githooks 存放在 .git/hooks 目录,没有配置 githooks 的仓库默认会有很多 .sample 结尾的示例文件,去掉 .sample 重命名文件可以使 hooks 生效。在我们手动配置 githooks 的过程中,需要在对应名字的 hook 文件中编写逻辑,并且 .git 文件夹不能上传到远程仓库,配置无法共享,需要在根目录创建新的文件夹存放 hooks 并配置给 git 运行,比较繁琐,所以使用 Husky 来更加方便的配置 githooks,参考 Husky 官网。
1. 创建项目 (记得初始化自己的git仓库)
yarn create vite my-app --template react-ts
2.安装 husky
yarn add husky -D
3.启用 hook
npx husky install
要在添加新的 hook 后自动启用,请编辑 package.json。
npm pkg set scripts.prepare="husky install"
执行以上命令你将得到下边的内容,如果 npm 版本过低不支持此命令也可以手动填写。
// package.json
{
"scripts": {
"prepare": "husky install"
}
}
4.创建一个 hook
要创建 hook 或者添加新命令,请使用husky add [cmd](在此之前需要 husky install 获得 .husky 目录),例如创建一个 pre-commit hook:
npx husky add .husky/pre-commit ""
此时会创建一个执行逻辑为空的 pre-commit hook,可以根据需求添加不同的 [cmd]
作者:昭昭日月明
链接:https://juejin.cn/post/7174992870313574456
二、ESLint基本规范以及相关规范插件使用
1.安装 eslint
yarn add eslint -D
2.初始化 eslint 并生成配置文件
npx eslint --init
执行此命令后会进行初始化配置,根据自己项目的情况和使用习惯选择,例如:
✔ How would you like to use ESLint? · syntax
✔ What type of modules does your project use? · esm
✔ Which framework does your project use? · react
✔ Does your project use TypeScript? · No / Yes
✔ Where does your code run? · browser
✔ What format do you want your config file to be in? · JavaScript
完成后会在项目根目录生成 .eslintrc 文件,可以在这里写具体规则,如果需要更加详细的配置可以参考 eslint 配置。
例如:.eslintrc.cjs
module.exports = {
env: {
browser: true,
es2021: true,
},
extends: ["eslint:recommended", "plugin:react/recommended", "plugin:@typescript-eslint/recommended"],
overrides: [],
parser: "@typescript-eslint/parser",
parserOptions: {
ecmaVersion: "latest",
sourceType: "module",
},
plugins: ["react", "@typescript-eslint"],
rules: {
"react/react-in-jsx-scope": "off",
"@typescript-eslint/no-explicit-any": ["off"],
},
settings: {
react: {
version: "detect",
// React version. "detect" automatically picks the version you have installed.
// You can also use `16.0`, `16.3`, etc, if you want to override the detected value.
// It will default to "latest" and warn if missing, and to "detect" in the future
},
},
};
(如果cv不管用,就把之前的文件删了重新新建)
3.使用 lint-staged
lint-staged 为了实现每次提交只检查本次提交(暂存区)所修改的文件,避免在遗留项目中使用 eslint 时面对成千上万的 lint error
安装 lint-staged
yarn add lint-staged -D
在 package.json 中配置,只检查暂存区的 .ts,.tsx 文件
"scripts": {
........
},
//只检查暂存区的 .ts,.tsx 文件
"lint-staged": {
"*.{ts,tsx}": [
"eslint --fix"
]
},
在 文件.husky/pre-commit 中关联 lint-staged 命令:
#!/usr/bin/env sh
. "$(dirname -- "$0")/_/husky.sh"
yarn lint-staged
4. 规范提交日志
安装 commitlint 插件
yarn add @commitlint/cli @commitlint/config-conventional -D
初始化 commitlint 配置(注意根据CommonJS和ES选择生成 .js/.cjs 格式文件)
echo "module.exports = {extends: ['@commitlint/config-conventional']}" > commitlint.config.cjs
创建 commit-msg hook
npx husky add .husky/commit-msg 'npx --no -- commitlint --edit ${1}'
填写不符合规范的 message 时:
git commit -m "123"
⧗ input: 123
✖ subject may not be empty [subject-empty]
✖ type may not be empty [type-empty]
✖ found 2 problems, 0 warnings
ⓘ Get help: https://github.com/conventional-changelog/commitlint/#what-is-commitlint
如果遇到使用 nvm 等工具管理 node 会遇到 command not found 的问题,需要在用户目录创建 .huskyrc 文件:
# ~/.huskyrc
# This loads nvm.sh and sets the correct PATH before running hook
export NVM_DIR="$HOME/.nvm"
[ -s "$NVM_DIR/nvm.sh" ] && \. "$NVM_DIR/nvm.sh"
5. .gitignore文件
在一些项目中,我们不想让本地仓库的所有文件都上传到远程仓库中,而是有选择的上传,比如:一些依赖文件(node_modules下的依赖)、bin 目录下的文件、测试文件等。一方面将一些依赖、测试文件都上传到远程传输量很大,另一方面,一些文件对于你这边是可用的,在另一个人那可能就不可用了,比如:本地配置文件。
例如:
# Logs
logs
*.log
npm-debug.log*
yarn-debug.log*
yarn-error.log*
pnpm-debug.log*
lerna-debug.log*
node_modules
dist
dist-ssr
*.local
# Editor directories and files
.vscode/*
!.vscode/extensions.json
.idea
.DS_Store
*.suo
*.ntvs*
*.njsproj
*.sln
*.sw?
三、Prettier基本配置
下载
yarn add --dev --exact prettier
然后,创建一个空的配置文件,让编辑器和其他工具知道你正在使用Prettier:
echo {}> .prettierrc.json
接下来,创建一个.prettierignore文件,让Prettier CLI和编辑器知道哪些文件不需要格式化。例如:
# Ignore artifacts:
node_modules
dist
现在,用Prettier格式化所有文件:
yarn prettier . --write
前端工程化基本配置文件:
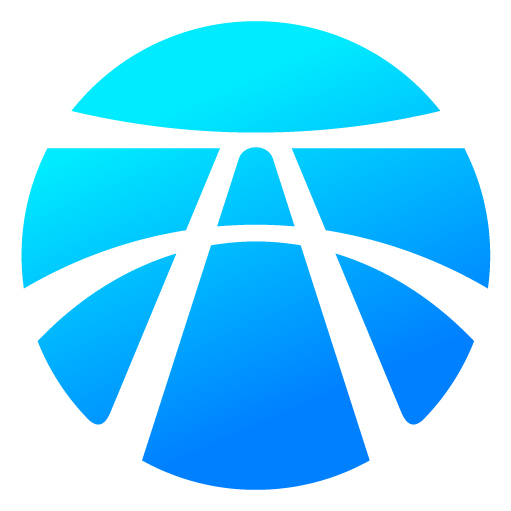
开放原子开发者工作坊旨在鼓励更多人参与开源活动,与志同道合的开发者们相互交流开发经验、分享开发心得、获取前沿技术趋势。工作坊有多种形式的开发者活动,如meetup、训练营等,主打技术交流,干货满满,真诚地邀请各位开发者共同参与!
更多推荐
所有评论(0)