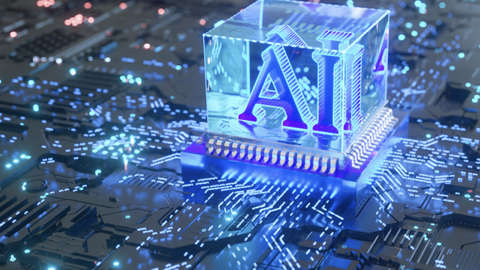
考研真题C语言
在上述代码中,我们定义了 `countCharacters` 函数来统计输入字符串中的每种字符类型的个数。(3)算法的时间复杂度是 O(n),其中 n 是输入字符串的长度。2. 判断字符的类型,根据字符的ASCII码范围来判断是否为英文字母、数字、空格或其他字符。printf("英文字母个数:%d\n", letters);printf("其他字符个数:%d\n", others);printf(
【2015年山西大学考研真题】输入一行字符,分别统计其中英文字母、空格、数字和其他字符的
个数。
(1)算法的基本思想如下:
1. 遍历输入的字符串,依次判断每个字符的类型。
2. 判断字符的类型,根据字符的ASCII码范围来判断是否为英文字母、数字、空格或其他字符。
3. 统计每种字符类型的个数。
(2)下面是使用C语言描述这个算法的代码:
```c
#include <stdio.h>
void countCharacters(char* str, int* letters, int* spaces, int* digits, int* others) {
char ch;
int i = 0;
while (str[i]) {
ch = str[i];
if ((ch >= 'a' && ch <= 'z') || (ch >= 'A' && ch <= 'Z')) {
(*letters)++;
} else if (ch == ' ') {
(*spaces)++;
} else if (ch >= '0' && ch <= '9') {
(*digits)++;
} else {
(*others)++;
}
i++;
}
}
int main() {
char str[100];
int letters = 0, spaces = 0, digits = 0, others = 0;
printf("请输入一行字符:");
gets(str);
countCharacters(str, &letters, &spaces, &digits, &others);
printf("英文字母个数:%d\n", letters);
printf("空格个数:%d\n", spaces);
printf("数字个数:%d\n", digits);
printf("其他字符个数:%d\n", others);
return 0;
}
```
在上述代码中,我们定义了 `countCharacters` 函数来统计输入字符串中的每种字符类型的个数。在 `main` 函数中,我们读取输入字符串,并调用 `countCharacters` 来进行统计。
(3)算法的时间复杂度是 O(n),其中 n 是输入字符串的长度。我们需要遍历每个字符判断其类型,并累加对应的计数器。
更多推荐
所有评论(0)