个人博客网站记录 3
文章目录1、富文本编辑器:1、富文本编辑器:下载ue,地址:https://github.com/fex-team/ueditor下载的慢可以下载网盘的,链接:https://pan.baidu.com/s/1jZhsBzNmkkUjQ3PuIDjfCg提取码:7fjz下载完解压,把文件夹放到项目中:http://www.miss168.net/detail/science/63125256758
文章目录
1、< a >跳转方式:
_blank //在新窗口中打开被链接文档。
_self //默认。在相同的框架中打开被链接文档。
_parent //在父框架集中打开被链接文档。
_top //在整个窗口中打开被链接文档。
framename //在指定的框架中打开被链接文档。
2、thymeleaf结合substring截取:
后端字符串可以直接使用substring
,前端可以像下面这样:
th:text = "${#strings.substring(articleComment1.ip, 0, 3)}+'.xxx'"
3、修改alert样式:
参考自:https://www.cnblogs.com/st-leslie/articles/5279864.html
<script>
//做了一些修改
window.alert = function(data) {
var alert1 = document.createElement("div"),
p = document.createElement("p"),
textNode = document.createTextNode(data ? data : "");
// 控制样式
css(alert1, {
"position" : "fixed",
"left" : "0",
"right" : "0",
"top" : "40%",
"font-family":"宋体,Georgia,Serif",
"width" : "100px",
"font-weight":"bold",
"height" : "40px",
"margin" : "0 auto",
"color" : "#ffffff",
"background-color" : "#1f1f1f",
"border-radius" : "4px",
"font-size" : "15px",
"text-align" : "center"
});
p.appendChild(textNode);
alert1.appendChild(p);
document.getElementsByTagName("body")[0].appendChild(alert1);
//显示1.5秒消失
setTimeout(function()
{alert1.parentNode.removeChild(alert1);},1500)
}
function css(targetObj, cssObj) {
var str = targetObj.getAttribute("style") ? targetObj.getAttribute("style") : "";
for(var i in cssObj) {
str += i + ":" + cssObj[i] + ";";
}
targetObj.style.cssText = str;
}
</script>
添加代码:
alert("请输入评论");
alert("评论成功");
4、局部刷新div:
刷新id
为comment_div
的标签:
function()
{$("#comment_div").load(location.href + " #comment_div");} //这里留个空格
5、按钮跳转浏览器顶部和底部:
<script>
document.getElementById('to_top').onclick = function() {
$('html, body').animate({ scrollTop: 0 }, 'slow');
}
document.getElementById('to_bottom').onclick = function() {
$('html, body').animate({ scrollTop: document.body.scrollHeight }, 'slow');
}
</script>
以下来自:https://www.cnblogs.com/mengshenshenchu/p/6666300.html
网页可见区域宽: document.body.clientWidth
网页可见区域高: document.body.clientHeight
网页可见区域宽: document.body.offsetWidth (包括边线的宽)
网页可见区域高: document.body.offsetHeight (包括边线的高)
网页正文全文宽: document.body.scrollWidth
网页正文全文高: document.body.scrollHeight
网页被卷去的高: document.body.scrollTop
网页被卷去的左: document.body.scrollLeft
网页正文部分上: window.screenTop
网页正文部分左: window.screenLeft
屏幕分辨率的高: window.screen.height
屏幕分辨率的宽: window.screen.width
屏幕可用工作区高度: window.screen.availHeight
屏幕可用工作区宽度: window.screen.availWidth
6、ajax的一些使用:
(1)ajax get:
如点击按钮对photoGraph
发起请求:
document.getElementById('up_button').onclick=function (e){ //点击按钮up_button时触发
$.ajax({ //ajax对同域的photoGraph发起请求
url: "/photoGraph" , // 提交get请求的地址
type: "GET", //方法类型
dataType: "text", //返回的数据类型
success: function (data, statusText, xmlHttpRequest) {
console.log(data); //控制台打印请求数据
}
});
}
接着f12
打开控制台,并点击按钮,控制台就会输出内容了:
如果有报错信息也会在控制台显示,如404
等。当我把url
改为https://www.baidu.com
时会提示:
表明这个请求跨域了,请求不在同一个IP
下,需要跨域访问才行。
(2)ajax post:
由于ajax
的post
请求是通过XMLHttpRequest
来向服务器发送异步请求的,它从服务器获取的数据是纯文本流,所以即使获取到得后端程序中return
了某个html
页面,也只是以文本流的形式发给了ajax
来进行处理,所以并不会产生页面的跳转,要实现跳转,则需要使用ajax
在js
中实现。
修改ajax
中的代码:
$.ajax({
url: "/article" , //post的目的地址,该url表示ajax post将会执行后端的article.html的postMapping方法
type: "POST", //方法类型
dataType: "html", //返回的数据类型
success: function () { //post成功后执行
alert("SUCCESS"); //抛出success
},
complete: function(XMLHttpRequest, textStatus){ //post结束后执行
alert(XMLHttpRequest.responseText); //抛出post回显的内容
}
})
后端修改article
的postMapping
方法:
@RequestMapping(value = "/article", method = RequestMethod.POST)
public String tt1(ModelMap map) {
//其他操作,如数据库控制
return "article"; //返回 article.html
}
再次点击网页中的按钮,先是抛出success
,然后就会把回显的内容抛出了:
(3)向后端提交内容:
使用data
向后端提交内容,如果有多个ajax
的post
的请求就可以使用提交的内容来区分。
通过data
传参的几种方式百度上有,我就不细写了,下面我列举了json
方式。
修改ajax
中的代码:
$.ajax({
url: "/article" ,
type: "POST",
//传递的参数
data:{"action":"up","content":"none"},
dataType: "html",
success: function () {
}
});
修改后端代码:
@RequestMapping(value = "/article", method = RequestMethod.POST)
@ResponseBody
//这里的参数名一定要和前端的json中的键的名字一样才能接收到
public void tt1(String action,String content) {
System.out.println(action);
System.out.println(content);
}
多个表单提交,然后根据form
表单内容来传参可以参考:https://blog.csdn.net/fukaiit/article/details/89097530
(4)评论点赞的实现:
- 点赞:
继续之前的,由于没找到js
怎么直接使用map.addAttribute
的方法,所以我创建了一个标签<p>
用来存储文章id
,然后用js来通过它获取:
<p id="articleId" style="display: none" th:text="${articleId}"></p>
修改点赞按钮的点击事件:
var up_signal = false; //用来标记点赞状态
//获取标签中存储的文章Id
var articleIdP = document.getElementById('articleId');
let articleId = articleIdP .innerText;
document.getElementById('up_button').onclick=function (e){
var upbutton = document.getElementById('up_button');
if(up_signal){ //取消点赞
//点赞按钮变灰色
upbutton.style.backgroundImage="url('../source/up1.png')";
up_signal=~up_signal; //状态取反
$.ajax({
url: "/article" , //url
type: "POST", //方法类型
data:{"action":"up","articleId":`${articleId}`,"up_signal":0,"content":"none"},
dataType: "html",//预期服务器返回的数据类型
success: function () {
}
});
}
else { //点赞
//点赞按钮变红色
upbutton.style.backgroundImage="url('../source/up2.png')";
up_signal=~up_signal;
//以下代码表示每点击一下点赞按钮,鼠标旁会出现感谢点赞的字样,然后慢慢消失
var a = new Array("感谢点赞");
var $i = $("<span/>").text(a[0]);
var x = e.pageX,y = e.pageY;
$i.css(
{"z-index": 100000000,"top": y - 20,"left": x,"position": "absolute","font-size":"12px","color": "#ff6651"}
);
$("body").append($i);
$i.animate(
{"top": y - 180,"opacity": 0}
,1500
,function()
{$i.remove();}
);
$.ajax({
url: "/article" , //url
type: "POST", //方法类型
//传递给后端
data:{"action":"up","articleId":`${articleId}`,"content":"none"},
dataType: "html",
success: function () {
}
});
}
}
后端响应代码:
@RequestMapping(value = "/article", method = RequestMethod.POST)
@ResponseBody
public void up_Comment_Add(String action,String articleId,int up_signal,String content) {
if (action.equals("up")){
if (up_signal==1){ //点赞
articleMapper.addUp(Integer.parseInt(articleId)); //数据库中该文章的点赞数+1
}
else
{ //取消赞
articleMapper.minusUp(Integer.parseInt(articleId)); //数据库中该文章的点赞数-1
}
}
else //评论
{
}
}
- 评论:
填写评论的框我选择的是textarea
:
<!-- 评论输入框 -->
<label for="commentContent"></label>
<textarea id="commentContent" placeholder="留下你的评论吧" style="outline: none;width: 80%;height: 50px;resize: none;float: left;border: 2px solid rgb(220,220,220);" maxlength="100">
</textarea>
<!-- 评论提交按钮 -->
<button id="releaseComment" style="width:70px;height: 25px;float: left;margin-left: 40px;cursor: pointer;outline: none;background-color: #3b97d7;border-radius: 3px;border: 0;color:white;">发布评论</button>
提交评论按钮响应的js
代码:
<script>
//发表评论按钮
document.getElementById('releaseComment').onclick=function (){
//textarea中填写的评论内容
var value1 = document.getElementById('commentContent').value;
if (value1.length === 0) //未填写评论内容
{
alert("请输入评论");
}
else
{
//将填写好的文章内容提交给后端
$.ajax({
url: "/article" ,
type: "POST",
data:{"action":"comment","articleId":`${articleId}`,"up_signal":0,"content":value1},
dataType: "html",
success: function () {
document.getElementById('commentContent').value = "";
alert("评论成功");
},
error: function (){
alert("出错啦");
}
});
//刷新评论区的div,由于使用时发现刷新后第一次不显示,设置了定时器后正常显示
setTimeout(function()
{$("#comment_div").load(location.href + " #comment_div");},500)
}
</script>
由于我点赞和评论的后端写在同一个方法里了,所以一起放上来:
@RequestMapping(value = "/article", method = RequestMethod.POST)
@ResponseBody
public void up_Comment_Add(String action,String articleId,int up_signal,String content,HttpServletRequest request) { //,HttpServletRequest request
if (action.equals("up")){
if (up_signal==1){ //点赞
articleMapper.addUp(Integer.parseInt(articleId)); //数据库中该文章的点赞数+1
}
else
{ //取消赞
articleMapper.minusUp(Integer.parseInt(articleId)); //数据库中该文章点赞数-1
}
}
else //评论
{
articleMapper.addComment(Integer.parseInt(articleId)); //评论数+1
//将评论id、文章id、ip地址、当天日期、填写的评论内容加入到数据库
articleCommentMapper.addComment(new articleComment(articleCommentMapper.articleCommentList().size()+1
,Integer.parseInt(articleId),getCliectIp(request),LocalDate.now().toString(),content));
}
}
评论区我是使用的table
来显示的:
<table th:each="articleComment1,status:${articleComment}" style="border-bottom: 1px solid rgb(200,200,200);width: 98%;padding-left: 2%;">
<tr>
<!-- 评论内容 -->
<td colspan="3" th:text = "${articleComment1.commentContent}" ></td>
</tr>
<tr>
<!-- IP地址 -->
<td th:text = "'ip:'+${#strings.substring(articleComment1.ip, 0, 9)}+'.xxx'"></td>
<!-- 评论日期 -->
<td th:text = "'日期:'+${articleComment1.createdDate}"></td>
<!-- 楼层数 -->
<td th:text = "${status.size}-${status.index}+'楼'"></td>
</tr>
</table>
到这里,文章的显示切页读取,点赞评论等都已经有了个大概了,文章内容上,现在使用的是富文本写完文章后,把html
内容插入到数据库中,显示时用utext
来解析。关于富文本的内容我没有记录在这里,主要是插入图片的功能好像由于配置问题一直无法使用,所以我现在都是自己手敲把图片的位置代码插入进去的。等功能再完善完善以后,我会着力于增加前端的特效。
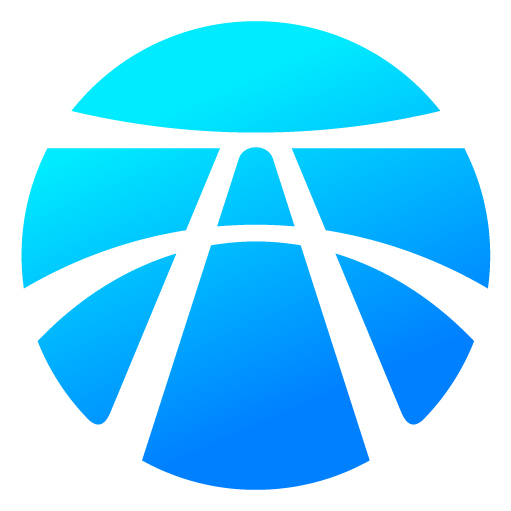
开放原子开发者工作坊旨在鼓励更多人参与开源活动,与志同道合的开发者们相互交流开发经验、分享开发心得、获取前沿技术趋势。工作坊有多种形式的开发者活动,如meetup、训练营等,主打技术交流,干货满满,真诚地邀请各位开发者共同参与!
更多推荐
所有评论(0)