你的第一个单机Java商城订单系统
鑫亿数码背景说明:为了方便用户购买网店商品,并且对用户购买的信息进行统一管理,可以把所有商品信息录入系统,通过数据库来保存相关录入的商品数据。主要功能包括浏览商品信息模块;购买商品模块;查询模块,按编号或者名称查询物品;订单管理模块。(无数据库、导出订单文件、纯eclipse控制台操作、附带操作结果说明文档)工具及环境:eclipse-jee-2018JDK11tomcat9源代码下载地址:鑫亿数
·
鑫亿数码
背景说明:
为了方便用户购买网店商品,并且对用户购买的信息进行统一管理,可以把所有商品信息录入系统,通过数据库来保存相关录入的商品数据。
主要功能包括浏览商品信息模块;购买商品模块;查询模块,按编号或者名称查询物品;订单管理模块。
(无数据库、导出订单文件、纯eclipse控制台操作、附带操作结果说明文档)
工具及环境:
- eclipse-jee-2018
- JDK11
- tomcat9
源代码下载地址:鑫亿数码
文件目录:
User实体类:
代码:
public class User implements Serializable {
private static final long serialVersionUID = 5666219877199167391L;
private String username;//用户名
private long code;//电话号码
private String address;//地址
private Date date;//下单时间
private int ways;//邮寄方式
private int card=1000;//订单默认后半部分编号
public User() {
super();
}
public User(String username, long code, String address) {
super();
this.username = username;
this.code = code;
this.address = address;
this.date =new Date();
this.ways=ways;
}
public String getUsername() {
return username;
}
public void setUsername(String username) {
this.username = username;
}
public long getCode() {
return code;
}
public void setCode(long code) {
this.code = code;
}
public String getAddress() {
return address;
}
public void setAddress(String address) {
this.address = address;
}
public Date getDate() {
return date;
}
public void setDate(Date date) {
this.date = date;
}
public int getWays() {
return ways;
}
public void setWays(int ways) {
this.ways = ways;
}
@Override
public String toString() {
//"订单编号\t\t创建时间\t\t创建人\t\t联系方式\t\t联系地址\t\t派送方式"
SimpleDateFormat sdf=new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");//获取时间
SimpleDateFormat sdf1=new SimpleDateFormat("yyyyMMdd");//获取时间字段作为订单前半部分
String way1;
if (ways==1) {
way1="自提";
} else {
way1="邮寄";
}
card++;//编号自增
StringBuilder builder=new StringBuilder();
String card1=card+"";//转字符串
builder.append(sdf1.format(date)).append(card1);//字符拼接
return builder+"\t"+sdf.format(date)+"\t"+username + "\t\t" + code + "\t"+address+"\t"+way1 ;
}
}
Order实体类:
代码:
public class Order {
private String gname;
private String id;
private int num;//购买数量
private double price;//单价
private double sumPrice;//总价
public Order() {
super();
}
public Order(String id,int num, double price) {
super();
this.gname = gname;
this.id=id;
this.num = num;
this.price = price;
this.sumPrice = price*num;
}
public String getGname() {
return gname;
}
public void setGname(String gname) {
this.gname = gname;
}
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public int getNum() {
return num;
}
public void setNum(int num) {
this.num = num;
}
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
}
public double getSumPrice() {
return sumPrice;
}
public void setSumPrice(double sumPrice) {
this.sumPrice = sumPrice;
}
@Override
public String toString() {
//商品\t\t价格\t\t购买数量\t\t总价
return gname+ "\t" + price + "\t\t" + num + "\t\t" + sumPrice;
}
}
Goods实体类:
代码:
public class Goods {
private String id;//编号
private String gname;//商品名
private double price;//价格
public Goods() {
super();
}
public Goods(String id, String gname, double price) {
super();
this.id = id;
this.gname = gname;
this.price = price;
}
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getGname() {
return gname;
}
public void setGname(String gname) {
this.gname = gname;
}
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
}
@Override
public String toString() {
return id + "\t\t" + gname + "\t\t" + price;
}
}
Customer实体类:
代码:
public class Customer {
private static Scanner scanner=new Scanner(System.in);
private static List<Goods> goods=new ArrayList<Goods>();
private static List<User> users=new ArrayList<User>();
private static List<Order> orders=new ArrayList<Order>();
//目标存放文件夹位置
private final String filePath="D:\\digitual.db";
public Customer() {
load();
}
private void load() {
//创建对象流 反序列化
ObjectInputStream ois=null;
File file=new File(filePath);
try {
if(!file.exists()||file.isDirectory()) {
System.out.println("订单文件不存在");
return;
}else {
ois=new ObjectInputStream(new FileInputStream(file));
//读取文件数据
users=(List<User>)ois.readObject();
}
} catch (FileNotFoundException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}catch(EOFException e) {
}
catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
catch (ClassNotFoundException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}finally {
if(ois!=null) {
try {
ois.close();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
}
//序列化,整个集合对象写到文件中
//退出时保存修改过后的集合
public void save() {
ObjectOutputStream oos=null;
try {
oos=new ObjectOutputStream(new FileOutputStream(filePath));
//写出数据
oos.writeObject(users);
oos.flush();
} catch (FileNotFoundException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}finally {
if(oos!=null) {
try {
oos.close();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
}
//商品信息
public static void putaway() {
//gname后为格式好看加了空格
Goods g1=new Goods("1001", "iPhoneX ", 9988);
Goods g2=new Goods("1002", "MacBook ", 12888);
Goods g3=new Goods("1003", "iPad-mini", 3688);
//添加数据
goods.add(g1);
goods.add(g2);
goods.add(g3);
}
//主菜单商品展示
public static void show() {
System.out.println("今日上架:");
System.out.println("编号\t\t商品\t\t\t价格");
for (Goods goods2 : goods) {
System.out.println(goods2);
}
}
//打印菜单
public static void printMenu() {
System.out.println("请选择:");
System.out.println("[1.我要下单]");
System.out.println("[2.我的订单]");
System.out.println("[3.查询商品]");
System.out.println("[4.退出]");
}
//购买
public void shopping() {
System.out.println("********************欢迎订购********************");
System.out.println("请输入商品编号:");
String id=scanner.next();
System.out.println("请输入购买数量:");
int num=scanner.nextInt();
for (Goods goods2 : goods) {
if (id.equals(goods2.getId())) {
Order order=new Order(id,num,goods2.getPrice());
order.setGname(goods2.getGname());
System.out.println("您当前购买的是:【"+goods2.getGname().trim()+"】,单价是:【"+goods2.getPrice()+"元】,购买数量:【"+num+"个】,合计:【"+order.getSumPrice()+"元】");
System.out.println("是否确定下单(Y/N?)");
String result=scanner.next();
if("y".equalsIgnoreCase(result)) {
orders.add(order);
System.out.println("请输入姓名:") ;
String username=scanner.next();
System.out.println("请输入联系方式:");
long code=scanner.nextLong();
System.out.println("请选择派送方式:【1、自提 2、邮寄】");
int way=scanner.nextInt();
System.out.println("请输入收货地址:");
String address=scanner.next();
System.out.println("恭喜下单成功,请耐心等待收货!");
User user=new User(username, code, address);
users.add(user);
user.setWays(way);
return ;
}else {
System.out.println("谢谢使用,欢迎下次再来!");
return;
}
}
}
System.out.println("您要购买的商品不存在");
}
//我的订单
public void history() {
if (users.size()>0) {
System.out.println("当前位置>>我的订单");
System.out.println("订单编号\t\t创建时间\t\t\t创建人\t\t联系方式\t\t联系地址\t\t派送方式");
for (User user : users) {
System.out.println(user);
}
} else {
System.out.println("还没有订单信息!");
}
}
//二级菜单
public void moreMenu() {
System.out.println("[1、查看订单][2、返回上一级][3、退出]");
int choice=scanner.nextInt();
switch (choice) {
case 1:
myOrder();
break;
case 2:
break;
case 3:
System.out.println("确认退出?Y/N");
String result=scanner.next();
//equalsIgnoreCase 忽略大小写 比较值
if("y".equalsIgnoreCase(result)) {
System.out.println("谢谢使用");
//信息保存
save();
}
System.exit(0);
break;
default:
break;
}
}
//订单详情
public static void myOrder() {
if (orders.size()>0) {
System.out.println("当前位置>>我的订单>>订单详情");
System.out.println("商品\t\t价格\t\t购买数量\t\t\t总价");
for (Order order : orders) {
System.out.println(order);
System.out.println("当前订单合计:"+order.getSumPrice()+"元");
}
} else {
System.out.println("还没购买商品!");
}
}
//查询商品
public static void find() {
System.out.println("请输入商品编号或名称:");
String gs1=scanner.next();
for (Goods goods3 : goods) {
//输入名称时有问题????若创建的gname中间有空格,30行出现异常java.util.InputMismatchException
//.trim() 去掉首位空格
if (gs1.equals(goods3.getId())||gs1.equals(goods3.getGname().trim())) {
System.out.println("编号\t\t商品\t\t\t价格");
System.out.println(goods3);
//putaway();
show();
return;
}
}
System.out.println("抱歉无此商品,我们会努力添加的哦!");
}
}
测试实体类:
代码:
public class Test {
private static Scanner scanner=new Scanner(System.in);
public static void main(String[] args) {
Customer customer=new Customer();
System.out.println("********************新毅数码********************");
customer.putaway();
customer.show();
//键盘输入选择
while (true) {
customer.printMenu();
int choices=scanner.nextInt();
//功能选项判断
switch (choices) {
case 1:
customer.shopping();//下单
break;
case 2:
customer.history();//查看订单
customer.moreMenu();//二级菜单
break;
case 3:
customer.find();//查询订单
//printMenu();
break;
case 4:
System.out.println("确认退出?Y/N");
String result=scanner.next();
//equalsIgnoreCase 忽略大小写 比较值
if("y".equalsIgnoreCase(result)) {
System.out.println("谢谢使用");
//信息保存
customer.save();
}
System.exit(0);
break;
default:
break;
}
}
}
}
系统运行:
- 初次运行界面:
初次运行,由于还未生成订单文件,所以提示订单文件不存在。 - 选择【我要下单】:
选择输入【1】后回车,控制台窗口出现提示信息,根据步骤进行下单,可以看到现在我下单成功,系统给与提示。 - 查看【我的订单】:
选择输入【2】后回车,系统显示刚才下单的订单列表,由于输入的字符长度不够,导致没有对齐表头,哈哈,你们将就着看吧! - 查看【订单详情】:
选择输入【1】后回车,控制台窗口显示当前位置以及订单的详情列表信息,来来来,66台电脑随便拿哈,不收大家的钱。 - 【查询商品】:
分别从三种情况去查询系统的商品信息,可以看到控制台窗口显示的信息都是符合的。 - 最后【退出】:
还不快去开动你充满智慧的脑袋去写出一个属于自己的系统~
注:能力有限,目前基本无干货,还请谅解,争取早日能够写出有质量的文章!
我是皮蛋布丁,一位爱吃皮蛋的热爱运动的废铁程序猿。
感谢各位大佬光临寒舍~
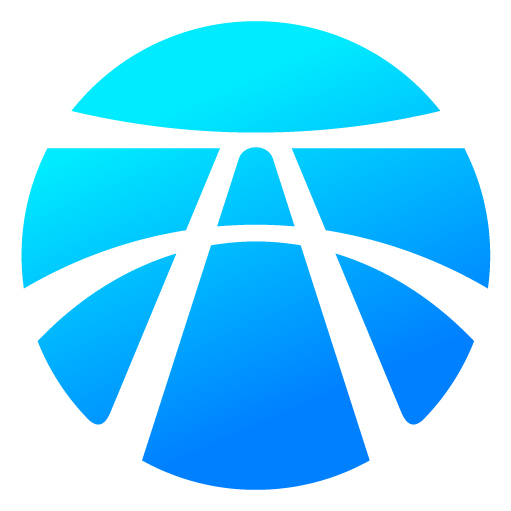
开放原子开发者工作坊旨在鼓励更多人参与开源活动,与志同道合的开发者们相互交流开发经验、分享开发心得、获取前沿技术趋势。工作坊有多种形式的开发者活动,如meetup、训练营等,主打技术交流,干货满满,真诚地邀请各位开发者共同参与!
更多推荐
所有评论(0)