java 泛型json解析_用Gson实现泛型解析
用Gson(URL:https://github.com/google/gson)解析Json方便之处我就不多说了,不过,要是针对没有实体类都写对应的解析方式是不是就有点晕了呢?代码一样就是解析的对象不一样,那么为什么不使用泛型呢?你会说我用了呀,会报错呀(java.lang.ClassCastException: com.google.gson.internal.LinkedTreeMap ca
用Gson(URL:https://github.com/google/gson)解析Json方便之处我就不多说了,不过,要是针对没有实体类都写对应的解析方式是不是就有点晕了呢?代码一样就是解析的对象不一样,那么为什么不使用泛型呢?你会说我用了呀,会报错呀(java.lang.ClassCastException: com.google.gson.internal.LinkedTreeMap cannot be cast to xxx)?是的,因为你没有写好,所以报错。
下面来看一下,我调通的代码吧,希望能帮助你。
ParserBase.java
package com.aoaoyi.gson.parser;
import android.text.TextUtils;
import com.google.gson.Gson;
import com.google.gson.reflect.TypeToken;
import java.util.List;
/**
* 解析对象基类
*
* Created by yuzhenbei on 2015/7/25.
*/
public class ParserBase {
/**
* 从JSON字符串中反序列化T对象
*
* @param pJsonStr JSON字符串
* @param pClass 对象的Class
* @param 将要反序列化成的T对象
* @return T对象
*/
public static T parserTFromJson(String pJsonStr, final Class pClass){
T _T;
try{
if (!TextUtils.isEmpty(pJsonStr)){
Gson _Gson = new Gson();
_T = _Gson.fromJson(pJsonStr, pClass);
}else {
_T = null;
}
}catch (Exception e){
e.printStackTrace();
_T = null;
}
return _T;
}
/**
* 序列化T对象为JSON字符串
*
* @param pT T对象
* @param 将要序列化的T对象
* @return JSON字符串
*/
public static String parserTToJson(T pT){
String _JosnStr;
try{
if (pT != null) {
Gson _Gson = new Gson();
_JosnStr = _Gson.toJson(pT);
}else {
_JosnStr = "";
}
}catch (Exception e){
e.printStackTrace();
_JosnStr = "";
}
return _JosnStr;
}
/**
* 从JSON字符串中反序列化List集合对象
*
* @param pJsonData JSON字符串
* @param pClass T对象的Class
* @param 对象类型
* @return List集合对象
*/
public static List parserListTFromJson(final String pJsonData, final Class pClass){
List _TList;
try{
if (!TextUtils.isEmpty(pJsonData)){
Gson _Gson = new Gson();
_TList = _Gson.fromJson(pJsonData, new ListOfJson(pClass));
}else {
_TList = null;
}
}catch (Exception e){
e.printStackTrace();
_TList = null;
}
return _TList;
}
/**
* 序列化List集合对象为JSON字符串
*
* @param pListT List集合对象
* @param 对象类型
* @return JSON字符串
*/
public static String parserListTToJson(List pListT){
String _JosnStr;
try{
if (pListT != null) {
Gson _Gson = new Gson();
_JosnStr = _Gson.toJson(pListT);
}else {
_JosnStr = "";
}
}catch (Exception e){
e.printStackTrace();
_JosnStr = "";
}
return _JosnStr;
}
}
其中用到的ListOfJson.class
package com.aoaoyi.gson.parser;
import java.lang.reflect.ParameterizedType;
import java.lang.reflect.Type;
import java.util.List;
/**
* class can store the exactly type of list
*
* Created by yuzhenbei on 2015/7/25.
*/
public class ListOfJson implements ParameterizedType {
private Class> mType;
public ListOfJson(Class pType) {
this.mType = pType;
}
/**
* Returns an array of the actual type arguments for this type.
*
* If this type models a non parameterized type nested within a
* parameterized type, this method returns a zero length array. The generic
* type of the following {@code field} declaration is an example for a
* parameterized type without type arguments.
*
*
* A<String>.B field;
*
* class A<T> {
* class B {
* }
* }
*
* @return the actual type arguments
* @throws TypeNotPresentException if one of the type arguments cannot be found
* @throws MalformedParameterizedTypeException if one of the type arguments cannot be instantiated for some
* reason
*/
@Override
public Type[] getActualTypeArguments() {
return new Type[] {mType};
}
/**
* Returns the parent / owner type, if this type is an inner type, otherwise
* {@code null} is returned if this is a top-level type.
*
* @return the owner type or {@code null} if this is a top-level type
* @throws TypeNotPresentException if one of the type arguments cannot be found
* @throws MalformedParameterizedTypeException if the owner type cannot be instantiated for some reason
*/
@Override
public Type getOwnerType() {
return null;
}
/**
* Returns the declaring type of this parameterized type.
*
* The raw type of {@code Set field;} is {@code Set}.
*
* @return the raw type of this parameterized type
*/
@Override
public Type getRawType() {
return List.class;
}
}
相关阅读:
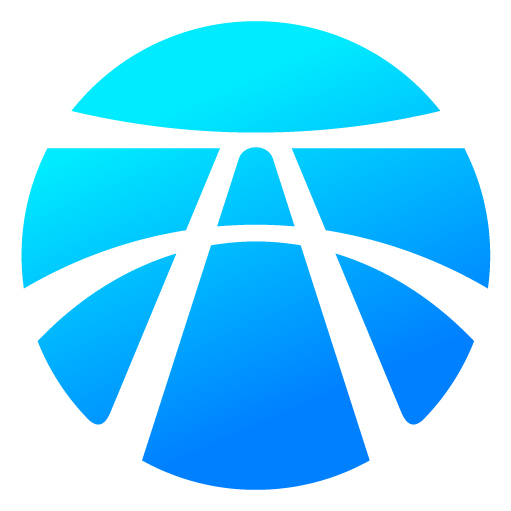
开放原子开发者工作坊旨在鼓励更多人参与开源活动,与志同道合的开发者们相互交流开发经验、分享开发心得、获取前沿技术趋势。工作坊有多种形式的开发者活动,如meetup、训练营等,主打技术交流,干货满满,真诚地邀请各位开发者共同参与!
更多推荐
所有评论(0)