Rails源代码分析(16):ActionController::Cookies
1 如何使用Cookies 从controller中调用直接调用cookies方法就能得到cookies, 读取的cookies是从request中读取的时候读取的, 读取的时候不会把cookie对象取回来,只会把它包含的值取回来 写入的cookies将会和response一起发送出去, # Examples for writing: # # # Sets a simple
·
1 如何使用Cookies
从controller中调用直接调用cookies方法就能得到cookies,
读取的cookies是从request中读取的时候读取的,
读取的时候不会把cookie对象取回来,只会把它包含的值取回来
写入的cookies将会和response一起发送出去,
可以看到提供了一个cookies方法,并通过helper_method开放给view访问
再看看CGI::Cookie本身
Examples of use
- # Examples for writing:
- #
- # # Sets a simple session cookie.
- cookies[:user_name] = "david"
- #
- # # Sets a cookie that expires in 1 hour.
- cookies[:login] = { :value => "XJ-122", :expires => 1.hour.from_now }
- #
- # Examples for reading:
- #
- cookies[:user_name] # => "david"
- cookies.size # => 2
- #
- # Example for deleting:
- #
- cookies.delete :user_name
支持的symbol包括::value, :path, :domain, :expires, :secure, :http_only
2 实现
- module Cookies
- def self.included(base)
- base.helper_method :cookies
- end
- protected
- # Returns the cookie container, which operates as described above.
- def cookies
- CookieJar.new(self)
- end
- end
下面主要看CookieJar的实现:
- class CookieJar < Hash #:nodoc:
- def initialize(controller)
- @controller, @cookies = controller, controller.request.cookies
- # 这个controller.request.cookies实际上是通过@cgi.cookies.freeze进行获取的
- super()
- update(@cookies)
- end
- # Returns the value of the cookie by +name+, or +nil+ if no such cookie exists.
- def [](name)
- # 到@cookies里面获取
- cookie = @cookies[name.to_s]
- # 如果包含value方法,如果里面大于1,那么就返回整个列表,否则返回第一个值
- if cookie && cookie.respond_to?(:value)
- cookie.size > 1 ? cookie.value : cookie.value[0]
- end
- end
- # Sets the cookie named +name+. The second argument may be the very cookie
- # value, or a hash of options as documented above.
- def []=(name, options)
- # 赋值操作
- if options.is_a?(Hash)
- # 如果是一个hash,那么下面这句话就是将所有的key都转化为String类型
- # 将这个值的key设置为 name.to_s,
- options = options.inject({}) { |options, pair| options[pair.first.to_s] = pair.last; options }
- options["name"] = name.to_s
- else
- options = { "name" => name.to_s, "value" => options }
- end
- set_cookie(options)
- end
- # Removes the cookie on the client machine by setting the value to an empty string
- # and setting its expiration date into the past. Like <tt>[]=</tt>, you can pass in
- # an options hash to delete cookies with extra data such as a <tt>:path</tt>.
- def delete(name, options = {})
- options.stringify_keys!
- # 如果删除将这个key对应的值清空,并且将cookie设置为过期以更新
- set_cookie(options.merge("name" => name.to_s, "value" => "", "expires" => Time.at(0)))
- end
- private
- # Builds a CGI::Cookie object and adds the cookie to the response headers.
- #
- # The path of the cookie defaults to "/" if there's none in +options+, and
- # everything is passed to the CGI::Cookie constructor.
- def set_cookie(options) #:doc:
- options["path"] = "/" unless options["path"] #默认路径设置为'/'
- cookie = CGI::Cookie.new(options)
- @controller.logger.info "Cookie set: #{cookie}" unless @controller.logger.nil?
- @controller.response.headers["cookie"] << cookie #将cookie设置到response里面
- end
- end
CGI::Cookie的Attributes包括:
domain | [RW] | |
expires | [RW] | |
name | [RW] | |
path | [RW] | |
secure | [R] | |
value | [RW] |
name:the name of the cookie. Required.
value:the cookie‘s value or list of values.
domain:the domain for which this cookie applies.
secure:whether this cookie is a secure cookie or not (default to false). Secure cookies are only transmitted to HTTPS servers.
Examples of use
- cookie1 = CGI::Cookie::new("name", "value1", "value2", ...)
- cookie1 = CGI::Cookie::new("name" => "name", "value" => "value")
- cookie1 = CGI::Cookie::new('name' => 'name',
- 'value' => ['value1', 'value2', ...],
- 'path' => 'path', # optional
- 'domain' => 'domain', # optional
- 'expires' => Time.now, # optional
- 'secure' => true # optional
- )
- cgi.out("cookie" => [cookie1, cookie2]) { "string" }
- name = cookie1.name
- values = cookie1.value
- path = cookie1.path
- domain = cookie1.domain
- expires = cookie1.expires
- secure = cookie1.secure
- cookie1.name = 'name'
- cookie1.value = ['value1', 'value2', ...]
- cookie1.path = 'path'
- cookie1.domain = 'domain'
- cookie1.expires = Time.now + 30
- cookie1.secure = true
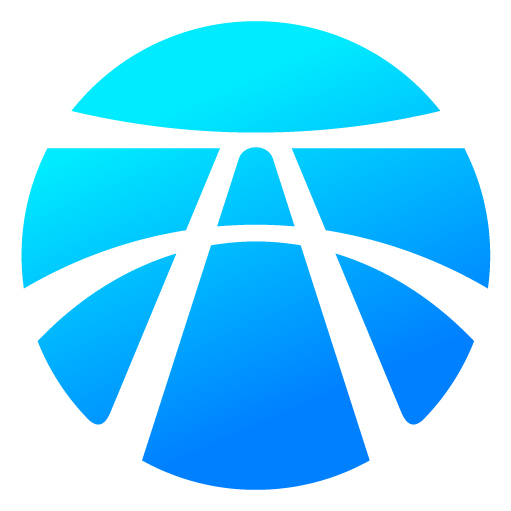
开放原子开发者工作坊旨在鼓励更多人参与开源活动,与志同道合的开发者们相互交流开发经验、分享开发心得、获取前沿技术趋势。工作坊有多种形式的开发者活动,如meetup、训练营等,主打技术交流,干货满满,真诚地邀请各位开发者共同参与!
更多推荐
所有评论(0)