React -- mobx5
Mobx 简介Mobx 介绍简单,可扩展的状态管理库Mobx 是由 Mendix , Coinbase, Facebook 开源和众多个人赞助商所赞助的React 和 Mobx 是一对强力组合,React 负责渲染应用的状态,Mobx负责管理应用状态供 React 使用Mobx 浏览器支持Mobx 5 版本运行在任何支持 ES6 proxy 的浏览器, 不支持 IE11,node.js 6Mobx
Mobx 简介
Mobx 介绍
- 简单,可扩展的状态管理库
- Mobx 是由 Mendix , Coinbase, Facebook 开源和众多个人赞助商所赞助的
- React 和 Mobx 是一对强力组合,React 负责渲染应用的状态,Mobx负责管理应用状态供 React 使用
Mobx 浏览器支持
- Mobx 5 版本运行在任何支持 ES6 proxy 的浏览器, 不支持 IE11,node.js 6
- Mobx 4 可以运行在任何支持 ES5 的浏览器上
- Mobx 4 和 5的 API 是相同的
开发前准备
启用装饰器语法支持(方式一)
- 弹射项目底层配置:npm run eject
- 下载装饰器语法babel插件:npm install @babel/plugin-proposal-decorators
- 在package.json文件中加入配置
"babel": { "plugins": [ [ "@babel/plugin-proposal-decorators", { "legacy": true } ] ] }
启用装饰器语法支持(方式二)
-
npm install react-app-rewired @babel/plugin-proposal-decorators
customize-cra -
在项目根目录下创建 config-overrides.js 并加入配置
const { override, addDecoratorsLegacy } = require("customize-cra"); module.exports = override(addDecoratorsLegacy());
-
在package.json文件中加入配置
"scripts": { "start": "react-app-rewired start", "build": "react-app-rewired build", "test": "react-app-rewired test", }
解决vscode编辑器关于装饰器语法的警告
- 修改配置:“javascript.implicitProjectConfig.experimentalDecorators”: true
Mobx 使用
下载 Mobx
npm install mobx mobx-react
Mobx 工作流程
Mobx + React 基本使用
-
定义 Store 类
import { observable } from 'mobx'; class CounterStore { @observable count = 0; increment = () => { this.count = this.count + 1; } } export default CounterStore ;
-
创建 Store 对象,通过 Provider 组件将 Store 对象放置在全局
import { Provider } from 'mobx-react'; import App from './App'; import Counter from './stores/counterStore'; const counter = new Counter() ReactDOM.render( <Provider counter={counter}><App /></Provider>, document.getElementById('root') );
-
将 Store 注入组件,将组件变成响应式组件
import { inject, observer } from "mobx-react"; @inject("counter") @observer class App extends Component { render() { const { counter } = this.props; return ( <div> <button onClick={counter.increment}>+</button> <span>{counter.count}</span> <button onClick={counter.decrement}>-</button> </div> ); } } export default App;
-
禁止普通方法更改可观察的状态
-
更正 Action 函数中 this 指向问题
在类中定义方法时,使用非箭头函数定义的方式 this 指向 undefinedimport { observable,configure, action} from 'mobx'; // 通过配置强制程序使用action函数更改应用程序中的状态 configure({enforceActions: 'observed'}); class CounterStore { @observable count = 0; @action.bound increment () { this.count = this.count + 1; } } export default CounterStore ;
-
异步更新状态 — runInAction
在 action 函数中如果存在异步代码,更新状态的代码就需要包裹在 runInAction 方法中import { observable, action, runInAction } from 'mobx'; import axios from 'axios'; class CounterStore { @observable users = []; @action.bound async getData () { let { data } = await axios.get('https://api.github.com/users'); runInAction(() => this.users = data); } }
-
异步更新状态 — flow
flow 方法中可以执行异步操作,可以直接进行状态的更改import { observable, action, flow} from 'mobx'; import axios from 'axios'; class CounterStore { @observable users = []; getData = flow(function* () { let { data } = yield axios.get('https://api.github.com/users'); this.users = data }).bind(this) }
-
数据监测 – computed 计算值
import { observable, computed } from 'mobx'; class CounterStore { @observable count = 0; @computed get getResult () { return this.count * 10 } } export default CounterStore ;
-
数据监测 – autorun 方法
当监测的状态发生变化时,你想根据状态产生 “效果”,请使用 autoran。
aotorun会在初始的时候执行一次,会在状态每次发生变化时执行autorun (asnyc () => { let response = await uniqueUsername(this.username) }, { delay: 2000 })
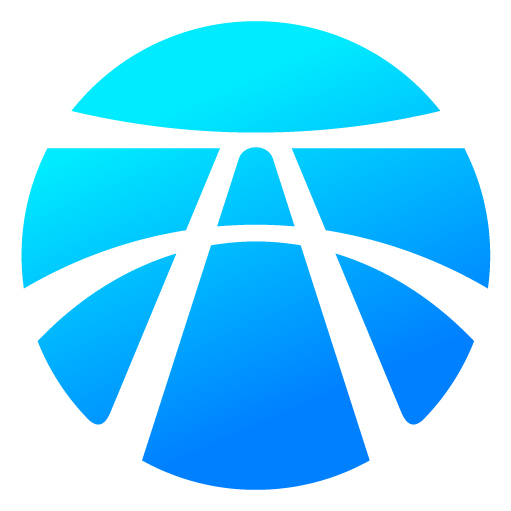
开放原子开发者工作坊旨在鼓励更多人参与开源活动,与志同道合的开发者们相互交流开发经验、分享开发心得、获取前沿技术趋势。工作坊有多种形式的开发者活动,如meetup、训练营等,主打技术交流,干货满满,真诚地邀请各位开发者共同参与!
更多推荐
所有评论(0)