数据增强 python代码_图像数据增强python代码
安装安装请参考https://github.com/aleju/imgaug 在安装时,如果Shapely这个库安装不上,请到https://pypi.org/project/Shapely/#history下载whl文件,然后使用命令pip install xxx_shaplely.whl进行安装施加单种变换from imgaug import augmenters as iaaimport i
安装
安装请参考https://github.com/aleju/imgaug
在安装时,如果Shapely这个库安装不上,请到https://pypi.org/project/Shapely/#history下载whl文件,然后使用命令
pip install xxx_shaplely.whl
进行安装
施加单种变换
from imgaug import augmenters as iaa
import imgaug as ia
import numpy as np
from scipy import misc
import os
def createdir(path):
if not os.path.exists(path):
os.mkdir(path)
def apply_aug(image):
images = []
flipper = iaa.Fliplr(1.0) # always horizontally flip each input image
images.append(flipper.augment_image(image)) # horizontally flip image 0
vflipper = iaa.Flipud(1) # vertically flip each input image with 90% probability
images.append(vflipper.augment_image(image)) # probably vertically flip image 1
blurer = iaa.GaussianBlur(sigma=(0, 3.0))
images.append(blurer.augment_image(image)) # blur image 2 by a sigma of 3.0
crop_and_pad = iaa.CropAndPad(px=(0, 30)) # crop images from each side by 0 to 16px (randomly chosen)
images.append(crop_and_pad.augment_image(image))
# inverter = iaa.Invert(0.05)
# images.append(inverter.augment_image(image))
contrast_normalization = iaa.ContrastNormalization((0.5, 2.0))
images.append(contrast_normalization.augment_image(image))
add_process = iaa.Add((-10, 10), per_channel=0.5)
images.append(add_process.augment_image(image))
sharpen_process = iaa.Sharpen(alpha=(0, 1.0), lightness=(0.75, 1.5))
images.append(sharpen_process.augment_image(image))
emboss_process = iaa.Emboss(alpha=(0, 1.0), strength=(0, 2.0)) # emboss images
images.append(emboss_process.augment_image(image))
rot_process_1 = iaa.Rot90(1)
images.append(rot_process_1.augment_image(image))
rot_process_2 = iaa.Rot90(2)
images.append(rot_process_2.augment_image(image))
rot_process_3 = iaa.Rot90(3)
images.append(rot_process_3.augment_image(image))
elastic_transformation_process = iaa.ElasticTransformation(alpha=(0.5, 3.5), sigma=0.25)
images.append(elastic_transformation_process.augment_image(image))
perspectivetransform_process = iaa.PerspectiveTransform(scale=(0.01, 0.1))
images.append(perspectivetransform_process.augment_image(image))
averageblur_process = iaa.AverageBlur(k=(2, 7))
images.append(averageblur_process.augment_image(image))
medianblur_process = iaa.MedianBlur(k=(3, 11))
images.append(medianblur_process.augment_image(image))
return np.array(images, dtype=np.uint8)
'''
文件夹格式:
根文件夹"D:\smj\data"
类别文件夹"D:\smj\data\dog"和"D:\smj\data\cat"
图片文件夹"D:\smj\data\dog\1.png"和"D:\smj\data\cat\1.png"
'''
root_path = r"D:\smj\data"
root_save_path = r"D:\smj\data\aug"
class_dirs = os.listdir(root_path)
for class_dir in class_dirs:
class_dir_path = root_path + "\\" + class_dir
img_names = os.listdir(class_dir_path)
class_save_dir_path = root_save_path + "\\" + class_dir
createdir(class_save_dir_path)
for img_name in img_names:
img_path = class_dir_path + "\\" + img_name
img = misc.imread(img_path)
images = apply_aug(np.array(img, dtype=np.uint8))
for i in range(images.shape[0]):
save_path = class_save_dir_path + "\\" + img_name.split(".")[0] + "====" + str(i) + ".png"
misc.imsave(save_path, images[i,:,:,:])
单个图片同时施加多种变换
from imgaug import augmenters as iaa
import imgaug as ia
import numpy as np
from scipy import misc
import os
seq = iaa.Sequential([
iaa.CropAndPad(
px=(0, 30)), # crop images from each side by 0 to 16px (randomly chosen)
iaa.Fliplr(1), # horizontally flip 50% of the images
iaa.Flipud(1),
iaa.GaussianBlur(sigma=(0, 3.0)), # blur images with a sigma of 0 to 3.0
# # iaa.Affine(
# # scale={"x": (0.8, 1.2), "y": (0.8, 1.2)}, # scale images to 80-120% of their size, individually per axis
# # translate_percent={"x": (-0.2, 0.2), "y": (-0.2, 0.2)}, # translate by -20 to +20 percent (per axis)
# # rotate=(-45, 45), # rotate by -45 to +45 degrees
# # shear=(-16, 16), # shear by -16 to +16 degrees
# # order=[0, 1], # use nearest neighbour or bilinear interpolation (fast)
# # cval=(0, 255), # if mode is constant, use a cval between 0 and 255
# # mode=ia.ALL # use any of scikit-image's warping modes (see 2nd image from the top for examples)
# # ),
iaa.Invert(0.05, per_channel=True),
iaa.ContrastNormalization((0.5, 2.0), per_channel=0.5),
# iaa.AddToHueAndSaturation((-20, 20)), #############commonly error
iaa.Add((-10, 10), per_channel=0.5),
iaa.Sharpen(alpha=(0, 1.0), lightness=(0.75, 1.5)),
iaa.Emboss(alpha=(0, 1.0), strength=(0, 2.0)), # emboss images
iaa.Rot90(1),
iaa.ElasticTransformation(alpha=(0.5, 3.5), sigma=0.25),
iaa.Rot90(2),
iaa.Rot90(3),
])
root_path = r"F:\data"
class_dirs = os.listdir(root_path)
for class_dir in class_dirs:
class_dir_path = root_path + "\\" + class_dir
img_names = os.listdir(class_dir_path)
for img_name in img_names:
img_path = class_dir_path + "\\" + img_name
img = misc.imread(img_path)
img = img[np.newaxis,:,:,:]
img = np.array(img, dtype=np.uint8)
images_aug = seq.augment_images(img) # done by the library
print("img_name")
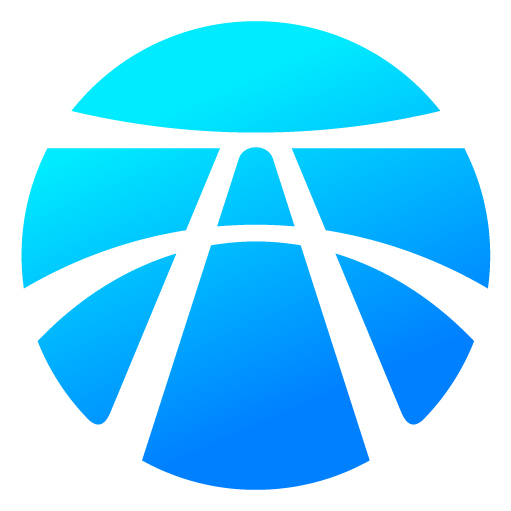
开放原子开发者工作坊旨在鼓励更多人参与开源活动,与志同道合的开发者们相互交流开发经验、分享开发心得、获取前沿技术趋势。工作坊有多种形式的开发者活动,如meetup、训练营等,主打技术交流,干货满满,真诚地邀请各位开发者共同参与!
更多推荐
所有评论(0)